Common Pitfalls When Setting Up Java EE 6 Development Environment
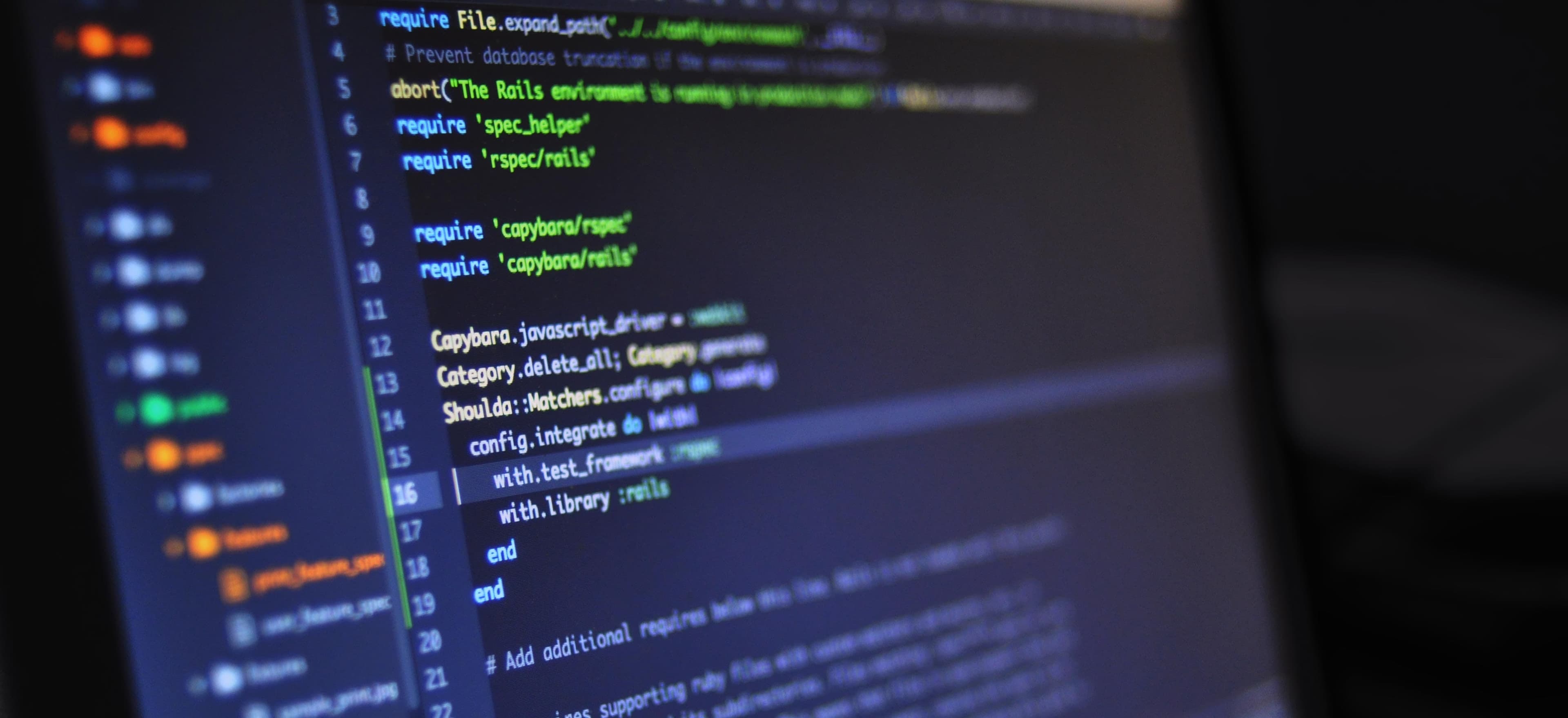
- Published on
Common Pitfalls When Setting Up Java EE 6 Development Environment
Setting up a Java EE 6 development environment can be daunting, especially for those new to Java Enterprise Edition. However, the right knowledge can help you avoid common pitfalls and streamline your development process. In this post, we'll explore some frequent mistakes and offer guidance on how to overcome them.
Understanding Java EE 6
Java EE (Enterprise Edition) is a set of specifications that extends the Java platform with specifications for enterprise features such as distributed computing and web services. Java EE 6, released in 2009, introduced some significant changes from previous versions, including simplified development with annotations and the introduction of new APIs. Understanding the core components of Java EE is essential for setting up your environment effectively.
Common Pitfall #1: Ignoring System Requirements
Before embarking on your journey, ensure you meet the system requirements. Java EE 6 applications typically run on robust application servers like GlassFish or JBoss.
Key Requirements:
- Java Development Kit (JDK): Ensure you are using JDK 6 or later. You can download it from the Oracle website.
- IDE: A good Integrated Development Environment (IDE) can simplify the setup process. Popular choices include Eclipse, IntelliJ IDEA, and NetBeans.
- Database: Make sure you have a compatible database such as MySQL, PostgreSQL, or Oracle installed.
Example Code: Check Java Version
public class JavaVersionCheck {
public static void main(String[] args) {
String version = System.getProperty("java.version");
System.out.println("Java version: " + version);
}
}
Why this Matters: Checking the Java version ensures your environment is compatible with Java EE 6, preventing issues during development.
Common Pitfall #2: Misconfiguring the Application Server
Setting up your application server incorrectly can lead to frustrating errors later in the development process.
Steps for Correct Configuration:
- Download and Install the Server: For instance, GlassFish can be downloaded from its official website.
- Configure Java EE: Ensure that the Java EE libraries are correctly linked to your server.
- Adjust Server Settings: Modify settings related to memory, threading, and the JDBC data source to suit your application’s requirements.
Example Configuration
In GlassFish, configuring a data source can be as simple as navigating to the admin console and selecting Resources > JDBC > JDBC Resources. Here, you can define data source properties such as JNDI name and connection pool settings.
Why this Matters: Proper configuration of the application server can significantly impact the performance and reliability of your applications.
Common Pitfall #3: Neglecting Dependency Management
Managing dependencies in a Java EE application is critical, particularly when using libraries and frameworks. Many developers overlook this aspect and end up with "Jar Hell" – conflicts arising from missing or incompatible libraries.
Solutions:
- Use a Build Tool: Tools like Maven or Gradle simplify dependency management. For example, Maven automatically downloads libraries declared in your
pom.xml
.
Example Maven Dependency:
<dependency>
<groupId>javax</groupId>
<artifactId>javaee-api</artifactId>
<version>6.0</version>
<scope>provided</scope>
</dependency>
Why this Matters: Using a build tool ensures all required libraries are available and up-to-date, significantly reducing conflicts and errors.
Common Pitfall #4: Overlooking Configuration for JavaServer Faces (JSF)
JavaServer Faces (JSF) simplifies the development of user interfaces for Java web applications. However, misconfiguring JSF can lead to runtime issues.
Key Configuration Steps:
- faces-config.xml File: Ensure that this file is correctly set up within the
WEB-INF
directory and defines your managed beans. - Add JSF Library: Ensure the JSF library is included in your project dependencies.
Sample faces-config.xml
<faces-config xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee
http://xmlns.jcp.org/xml/ns/javaee/web-facesconfig_2_1.xsd"
version="2.1">
<managed-bean>
<managed-bean-name>userBean</managed-bean-name>
<managed-bean-class>com.example.UserBean</managed-bean-class>
<managed-bean-scope>session</managed-bean-scope>
</managed-bean>
</faces-config>
Why this Matters: Properly configuring JSF ensures that user interfaces function correctly, providing a seamless user experience.
Common Pitfall #5: Forgetting About Security
When developing Java EE applications, security should not be an afterthought. Neglecting security can expose your application to vulnerabilities.
Key Security Considerations:
- Use Security Annotations: Leverage Java EE security annotations such as
@RolesAllowed
to secure your methods. - Define Roles in web.xml: Specify user roles in your
web.xml
file to control access.
Example web.xml Snippet
<security-constraint>
<web-resource-collection>
<web-resource-name>Protected Area</web-resource-name>
<url-pattern>/secure/*</url-pattern>
</web-resource-collection>
<auth-constraint>
<role-name>admin</role-name>
</auth-constraint>
</security-constraint>
Why this Matters: Implementing security measures from the outset helps protect your application from unauthorized access.
Bringing It All Together
Setting up a Java EE 6 development environment can be complex, but avoiding common pitfalls can make the process smoother. By paying attention to system requirements, properly configuring your application server, managing dependencies, correctly setting up components like JSF, and prioritizing security, you will create a robust development environment that allows for efficient coding and deployment.
For more insights on Java EE, consider checking out resources on Oracle Java EE Learning and additional tutorials available on Java Brains.
By being aware of these potential challenges and following the outlined strategies, you can improve your development experience and focus on building great applications.
Happy coding!
Checkout our other articles