How to Ensure Zero Downtime During Database Migrations
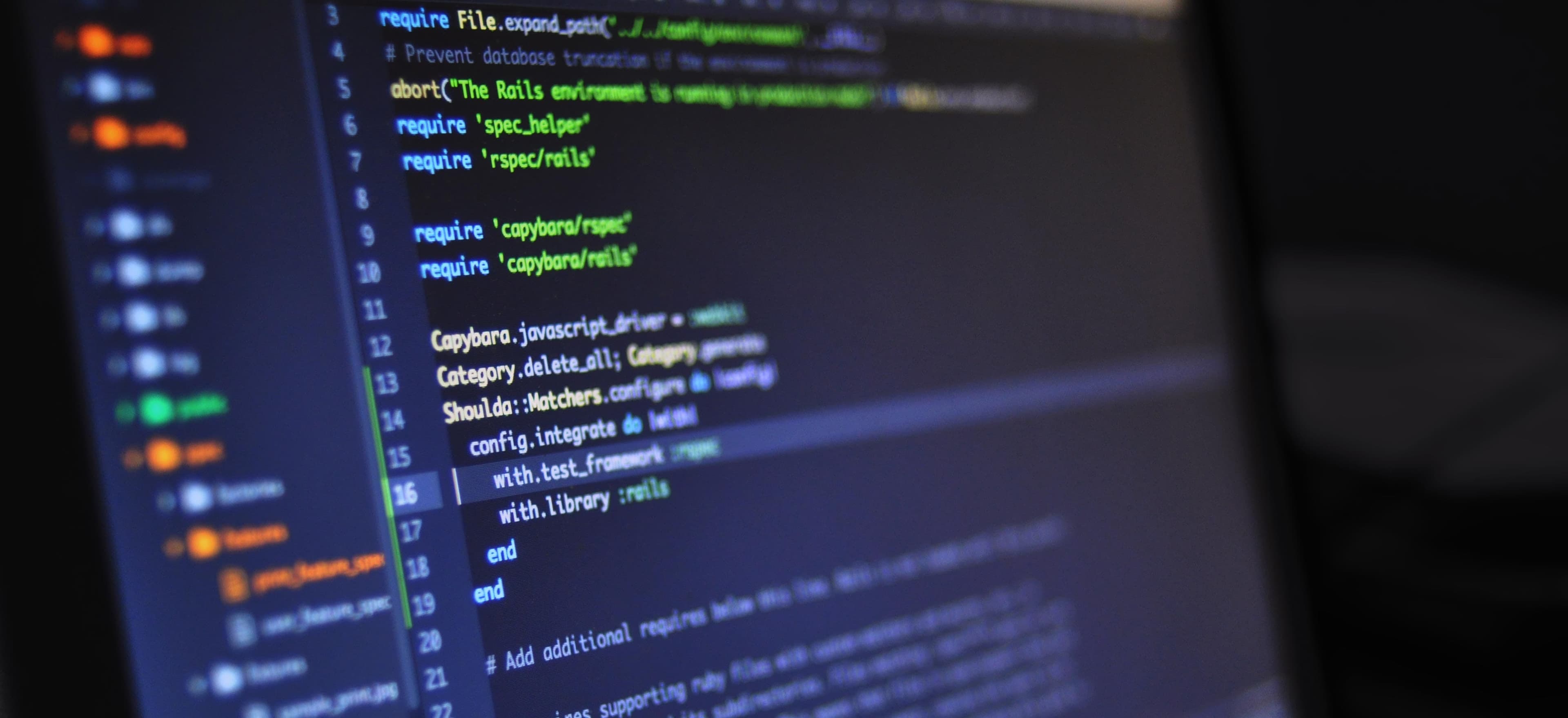
- Published on
How to Ensure Zero Downtime During Database Migrations
Database migrations are a crucial part of software development, allowing developers to evolve their databases seamlessly alongside code changes. However, traditional database migration techniques can lead to downtime, which may disrupt user experience and impact business operations. In this article, we’ll explore strategies to ensure zero downtime during database migrations, enhancing reliability and user satisfaction.
Understanding the Importance of Zero Downtime
Before diving into specific methodologies, it’s essential to grasp why zero downtime is vital for your application:
- User Experience: An uninterrupted service keeps your users happy and engaged.
- Revenue Impact: Downtime can directly affect sales, especially for e-commerce applications.
- Business Reputation: Consistent performance fosters trust and credibility in your brand.
Now, let’s look at effective strategies and practices to achieve zero downtime during database migrations.
1. Blue-Green Deployment
Blue-Green Deployment is a technique where two identical environments, dubbed Blue and Green, are maintained. During a migration, one environment serves production traffic while the other is updated.
Advantages
- Smooth traffic switching
- Immediate rollback option
Implementation Steps
- Clone Your Production Database: Create a replica of your production database to the Green environment.
- Run Migrations on Green: Apply the database changes and test thoroughly.
- Switch Traffic: Once validated, shift user traffic to the Green environment.
- Update the Blue Environment: After migration, you can upgrade the Blue environment without affecting users.
Code Example
# Clone your database schema to the new version
pg_dump -U username -h localhost -Fc dbname > dbname_backup.dump
# Run your migration on the new database
knex migrate:latest --env green
Explanation
This code demonstrates the process of backing up using Postgres and applying migrations using Knex (a SQL query builder). By creating backups, you ensure that you can roll back if anything goes wrong.
2. Rolling Updates with Versioned Migrations
Versioned migrations allow you to handle incremental changes in your database schema, minimizing the need for extensive changes that can trigger downtime.
Advantages
- Lesser risk of outages
- Easier code reviews
Implementation Steps
- Version Control Your Migrations: Use a versioning system (like timestamping) to track migration scripts.
- Deploy Non-Destructive Changes First: Start by adding new columns or tables but avoid removing or renaming during the application runtime.
- Gradually Deploy Code Changes: Once migrations are in place, you can update your application code to use the new columns/tables.
- Clean-Up Old Code: Finally, after confirming everything works well, you can remove old columns or tables.
Code Example
-- Add a new column in a non-destructive manner
ALTER TABLE users ADD COLUMN last_login TIMESTAMP NULL;
-- Update all necessary existing rows
UPDATE users SET last_login = CURRENT_TIMESTAMP WHERE condition_met = true;
-- Ensure the application can handle both old and new column names
Explanation
In this SQL migration, we added a new column, last_login
, without impacting existing functionality. By doing this first, we can transition the application logic comfortably and avoid breaking existing operations.
3. Feature Toggles
Feature toggles allow developers to enable or disable features without deploying new code. This practice enables code changes to be released without being fully operational.
Advantages
- Control the feature rollout
- Reduce risk in deployments
Implementation Steps
- Implement a Feature Toggle Framework: Use tools like LaunchDarkly or Optimizely to manage feature flags.
- Deploy Database Changes: Migrate the database to support new features while toggling them off in the application.
- Gradually Enable Features: Once confirmed working, enable features incrementally to gather feedback.
Code Example
// Pseudo-code for a feature toggle
if (featureToggle.isEnabled("newFeature")) {
// Execute code for the new feature
} else {
// Execute legacy code
}
Explanation
This conditional checks the status of a feature toggle. If the toggle is enabled, the new code executes; otherwise, the application continues using the existing code.
4. Data Backfilling and Shadow Reads
Data backfilling allows you to update existing data to fit new schema requirements. Shadow reads involve creating a parallel data read mechanism to validate the new schema without affecting current users.
Advantages
- Verify new schema functionality in real-time.
- Test migrations against live data without affecting performance.
Implementation Steps
- Introduce a Shadow Database: Duplicate the data into a shadow database that reflects changes.
- Backfill Data: Update existing records to comply with the new schema.
- Conduct Real-time Testing: Use the shadow reads to ensure the application works correctly with the updated data.
Code Example
-- Backfill the shadow database
INSERT INTO shadow_users SELECT *, DEFAULT(last_login) FROM users;
-- Example of querying changed data
SELECT * FROM shadow_users WHERE created_at >= NOW() - INTERVAL '1 month';
Explanation
In this example, we create a shadow version of the users
table, updating any records that need it. Queries against the shadow database allow for testing without risking production data integrity.
5. Read-Replica for Zero Downtime
Using read-replicas can create a buffer during migrations. The goal is to redirect read requests to replicas, allowing writes to continue on the primary database.
Advantages
- Mitigate performance impact
- Handle read-heavy traffic
Implementation Steps
- Set Up a Read-Replica: Use database features like AWS RDS or Azure SQL Databases to create replicas.
- Direct Reads to the Replica: Configure your application to read data from the replica.
- Perform Migrations with Writes on Primary: Apply changes to the primary while read traffic uses the replica.
Code Example
// Java code sample for read-replica
public ResultSet fetchUserData(int userId) {
Connection conn = readReplicaDataSource.getConnection();
PreparedStatement stmt = conn.prepareStatement("SELECT * FROM users WHERE id = ?");
stmt.setInt(1, userId);
return stmt.executeQuery();
}
Explanation
In this Java snippet, the application is redirected to use a read-replica for fetching user data. During migrations, this ensures that read operations are unaffected even when the primary database is undergoing changes.
Closing Remarks
Achieving zero downtime during database migrations entails careful planning and implementation. By leveraging techniques like Blue-Green Deployment, versioned migrations, feature toggles, data backfilling, and read-replicas, businesses can ensure a seamless user experience and protect their revenue streams.
When applying these techniques, it is vital to maintain a strong testing environment that allows for verifying changes before they reach production. Ensuring robust monitoring solutions will also help detect issues quickly, minimizing the potential impact on users.
Ultimately, proactive database management enables developers to scale applications effectively without the headache of downtime. Happy coding and migrating!
For more insights into database management and migrations, consider checking out PostgreSQL Migrations and AWS RDS Read Replicas.
Checkout our other articles