Mastering Nested Collections: Filter with Java Streams
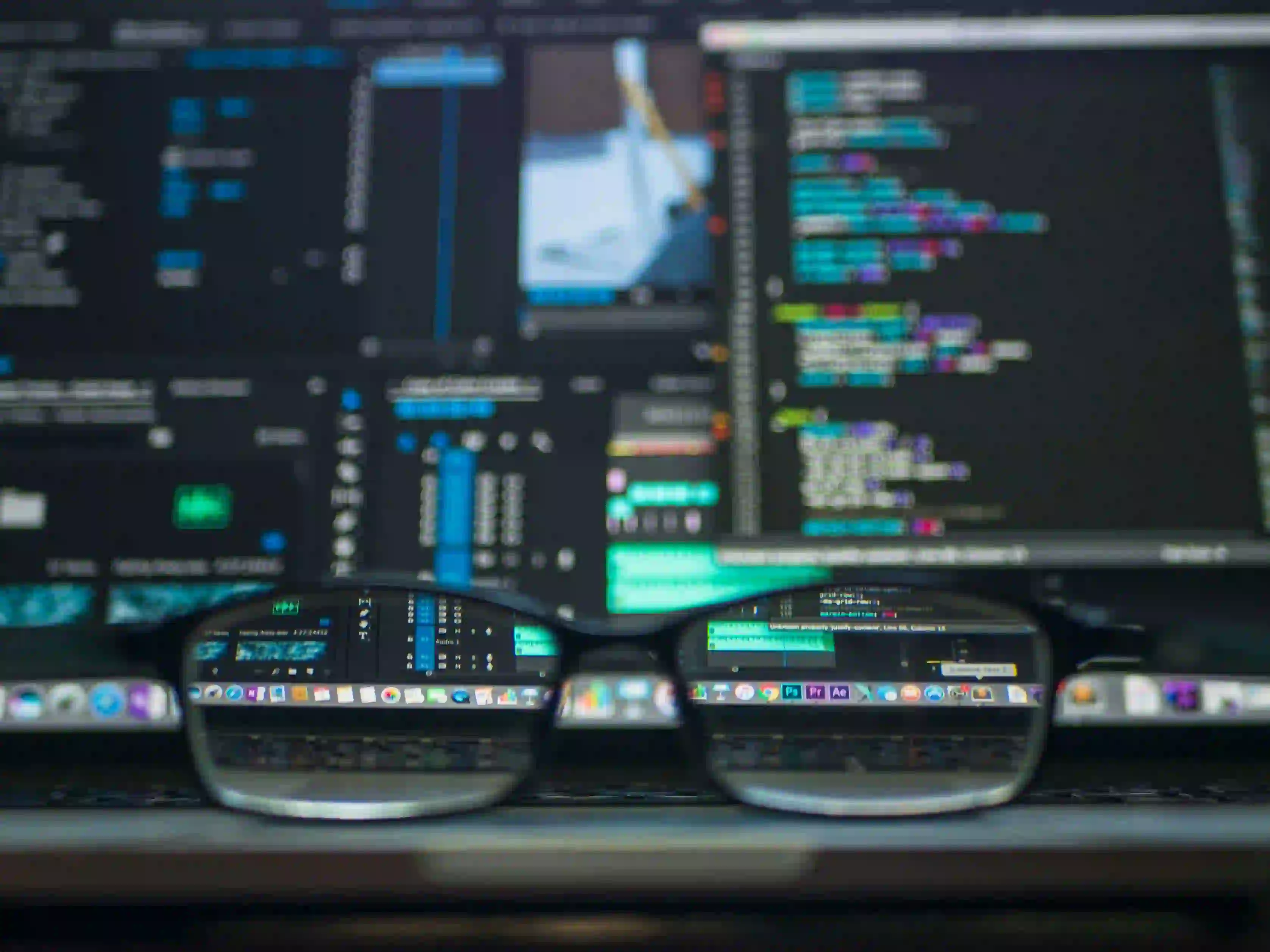
Mastering Nested Collections: Filter with Java Streams
Java Streams have revolutionized the way we handle collections in Java. They allow developers to process sequences of elements in a functional style, making code more readable and concise. In this blog post, we will delve into filtering nested collections using Java Streams, understand why this technique is powerful, and see some practical examples that demonstrate its utility.
Table of Contents
- Introduction to Java Streams
- Understanding Nested Collections
- Filtering with Java Streams
- Code Examples
- Conclusion
Setting the Scene to Java Streams
Java Streams, introduced in Java 8, offer a functional approach to processing collections of data. They enable developers to perform complex data manipulation tasks in a clear and efficient way. The main benefits of using Java Streams include:
- Declarative code: Focus on what to achieve rather than how to achieve it.
- Chaining operations: Combine multiple operations in a single statement.
- Lazy evaluation: Stream operations are performed only when necessary, improving efficiency.
To kick things off, let’s create a simple data model representing a Student
and a Classroom
.
Code Example: Defining the Data Model
import java.util.List;
public class Student {
private String name;
private int grade;
public Student(String name, int grade) {
this.name = name;
this.grade = grade;
}
public String getName() {
return name;
}
public int getGrade() {
return grade;
}
}
public class Classroom {
private String classroomName;
private List<Student> students;
public Classroom(String classroomName, List<Student> students) {
this.classroomName = classroomName;
this.students = students;
}
public String getClassroomName() {
return classroomName;
}
public List<Student> getStudents() {
return students;
}
}
Commentary on the Code
- The
Student
class models an individual student withname
andgrade
properties. - The
Classroom
class represents a collection of students, allowing easy access to each student's details.
Understanding Nested Collections
In many applications, data is not just flat but organized in a nested structure. For example, a Classroom
consists of multiple Student
objects, leading to a nested collection scenario where you might want to filter students based on various criteria.
Why Use Nested Collections?
Nested collections allow for complex data organization. In the educational system, for instance, classrooms contain students, which can represent grades and other relevant data. Manipulating these data structures efficiently is crucial for maintaining performance and clarity in your code.
Filtering with Java Streams
Using Java Streams to filter nested collections is both powerful and expressive. By leveraging methods such as filter
and flatMap
, you can succinctly express your data-processing intentions.
Basic Filtering Example
Let’s start with a simple example where we have a list of classrooms, and we want to filter students with grades above a certain threshold.
Code Example: Filtering Students
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class SchoolManagement {
public static void main(String[] args) {
Student john = new Student("John", 85);
Student alice = new Student("Alice", 92);
Student bob = new Student("Bob", 76);
List<Student> class1Students = Arrays.asList(john, alice);
List<Student> class2Students = Arrays.asList(bob);
Classroom class1 = new Classroom("Class 1", class1Students);
Classroom class2 = new Classroom("Class 2", class2Students);
List<Classroom> classrooms = Arrays.asList(class1, class2);
List<Student> highAchievers = classrooms.stream()
.flatMap(classroom -> classroom.getStudents().stream())
.filter(student -> student.getGrade() > 80)
.collect(Collectors.toList());
highAchievers.forEach(student -> System.out.println(student.getName()));
}
}
Commentary on the Code
- Creating a List of Classrooms: We model our
Student
andClassroom
with instances. - Flattening the Structure: Using
flatMap
, we convert theClassroom
structure into a flat stream of students. - Filtering: The
filter
method allows us to apply a condition (in our case, grades above 80). - Collecting Results: Finally, we collect the filtered students into a new list.
Advanced Filtering Scenarios
Example 1: Finding Students by Name
Imagine we need to find a student by name from various classrooms. Here's how you can accomplish that with Java Streams.
Code Example: Finding a Student by Name
public class SchoolManagement {
public static void main(String[] args) {
// Student and Classroom initialization code goes here...
String searchName = "Alice";
List<Student> foundStudents = classrooms.stream()
.flatMap(classroom -> classroom.getStudents().stream())
.filter(student -> student.getName().equals(searchName))
.collect(Collectors.toList());
foundStudents.forEach(student -> System.out.println(student.getName()));
}
}
Commentary on the Code
- The filtering condition checks the student's name.
- This pattern effectively retrieves a list of matched students, demonstrating the flexibility of Streams.
Example 2: Counting High Achievers
If we wanted to count the number of students with grades above a certain threshold, we could simplify this with the count()
method.
Code Example: Counting High Achievers
long highAchieverCount = classrooms.stream()
.flatMap(classroom -> classroom.getStudents().stream())
.filter(student -> student.getGrade() > 80)
.count();
System.out.println("Number of high achievers: " + highAchieverCount);
Commentary on the Code
- Here, we directly obtain the count of students meeting our criteria.
My Closing Thoughts on the Matter
Filtering nested collections with Java Streams is a powerful technique for building efficient and readable code. By effectively employing methods like flatMap
and filter
, we can navigate complex data structures with ease.
The examples discussed here lay a foundational understanding of manipulating nested collections. As you develop your applications, remember that tools like Java Streams can greatly enhance your workflow.
To explore more about Java Streams and their capabilities, you can check the official Java Documentation.
By mastering these techniques, you elevate your Java programming skills, making your data manipulation tasks more elegant and efficient. Happy coding!
This blog post provides insights and code snippets to help you grasp the concept of filtering nested collections using Java Streams. The combination of explanation and practical examples aims to solidify your understanding and serve as a useful reference in your programming journey.