Mastering Equality Checks in Java's 2D Arrays
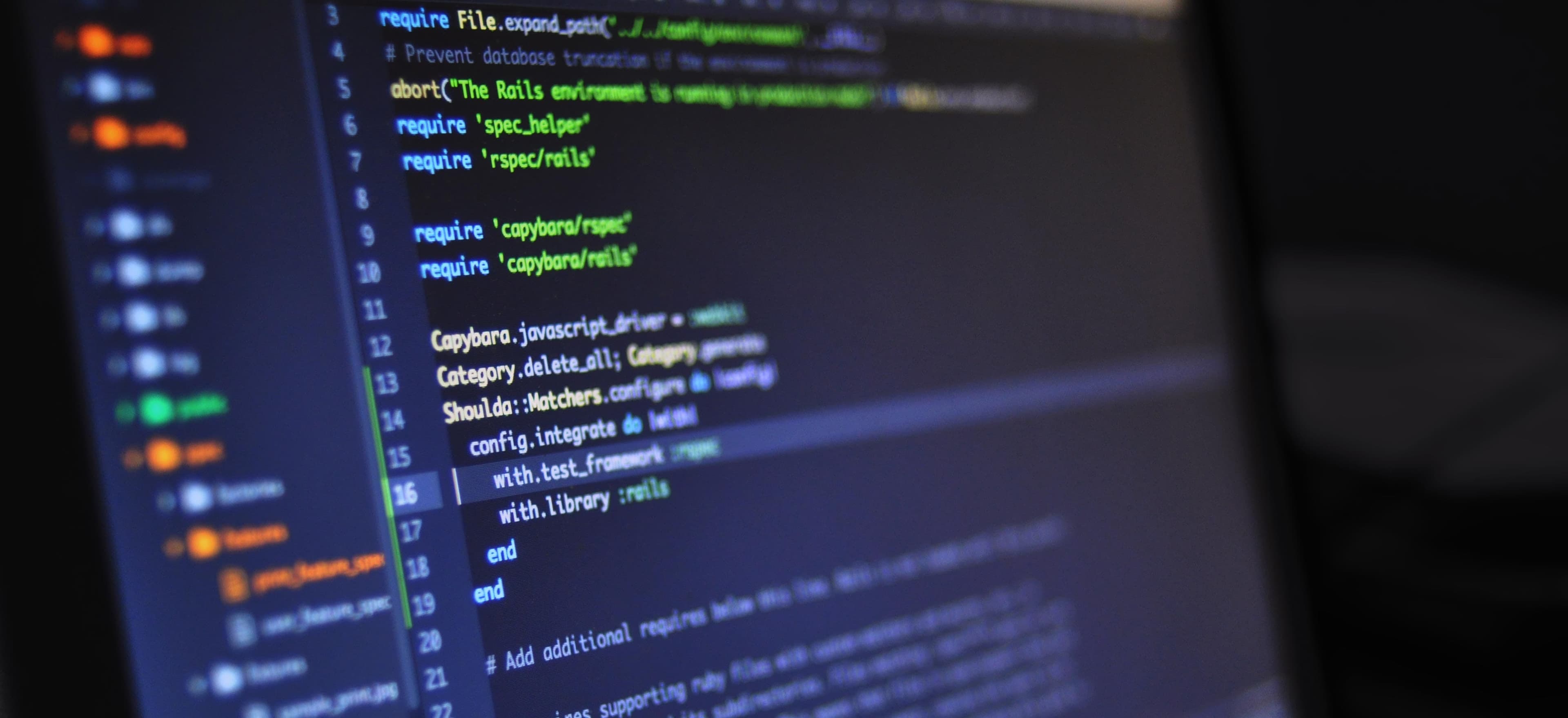
- Published on
Mastering Equality Checks in Java's 2D Arrays
When working with data structures in Java, arrays are a fundamental aspect of the language. Among them, two-dimensional arrays (2D arrays) hold a special place due to their capability to represent data in a grid-like format. Whether you are building complex applications or simply managing data, understanding how to perform equality checks in these structures is essential. This blog post will guide you through the intricacies of equality checks in Java's 2D arrays, outlining best practices and common pitfalls.
Table of Contents
- Understanding 2D Arrays in Java
- Equality Check Basics
- Using Arrays.deepEquals
- Custom Equality Checks
- Performance Considerations
- Conclusion
Understanding 2D Arrays in Java
In Java, a 2D array is essentially an array of arrays. This means that each element of a 2D array is itself an array. The syntax for declaring a 2D array looks like this:
int[][] matrix = new int[3][3]; // A 3x3 matrix
This declaration creates an array that can hold three arrays, each containing three integer values. If you were to assign values, you might end up with something like this:
matrix[0][0] = 1;
matrix[0][1] = 2;
matrix[0][2] = 3;
matrix[1][0] = 4;
matrix[1][1] = 5;
matrix[1][2] = 6;
matrix[2][0] = 7;
matrix[2][1] = 8;
matrix[2][2] = 9;
Equality Check Basics
When checking equality in Java, it's important to first understand the difference between reference equality and value equality:
- Reference equality: Uses the
==
operator, which checks if both references point to the same object in memory. - Value equality: Utilizes the
.equals()
method, which checks if two objects are logically equivalent.
For 2D arrays, using ==
will only determine if two array references point to the same array object. To compare the contents, a more robust approach is required.
int[][] array1 = { {1, 2}, {3, 4} };
int[][] array2 = { {1, 2}, {3, 4} };
System.out.println(array1 == array2); // false
Using Arrays.deepEquals
Java provides a convenient utility to handle equality checks with multi-dimensional arrays. The java.util.Arrays
class contains the deepEquals
method, specifically designed to compare nested arrays.
Here is a code snippet that demonstrates Arrays.deepEquals
:
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
int[][] array1 = { {1, 2}, {3, 4} };
int[][] array2 = { {1, 2}, {3, 4} };
int[][] array3 = { {1, 2}, {4, 3} };
boolean isEqual1 = Arrays.deepEquals(array1, array2);
boolean isEqual2 = Arrays.deepEquals(array1, array3);
System.out.println("Array1 equals Array2: " + isEqual1); // true
System.out.println("Array1 equals Array3: " + isEqual2); // false
}
}
Why Use deepEquals
?
The deepEquals
method compares the contents of two arrays recursively. This means it checks the equality of nested arrays as well, which is crucial for a 2D array.
In this method, if two corresponding elements are arrays themselves, deepEquals
will recursively check if these sub-arrays are equal.
Custom Equality Checks
While Arrays.deepEquals
is a powerful utility, there may be cases where you need a custom equality check tailored to your specific requirements. This can be essential if your arrays contain complex objects.
Here's an example of how one might construct a custom equality check:
public class CustomObject {
private int id;
private String name;
public CustomObject(int id, String name) {
this.id = id;
this.name = name;
}
// Override equals to compare CustomObject instances
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (!(obj instanceof CustomObject)) return false;
CustomObject other = (CustomObject) obj;
return this.id == other.id && this.name.equals(other.name);
}
}
public class Main {
public static void main(String[] args) {
CustomObject[][] array1 = {
{ new CustomObject(1, "A"), new CustomObject(2, "B") },
{ new CustomObject(3, "C"), new CustomObject(4, "D") }
};
CustomObject[][] array2 = {
{ new CustomObject(1, "A"), new CustomObject(2, "B") },
{ new CustomObject(3, "C"), new CustomObject(4, "D") }
};
boolean isEqual = Arrays.deepEquals(array1, array2);
System.out.println("Custom Objects equality: " + isEqual); // true
}
}
Why Override Equals?
Overriding the equals
method allows you to define what makes instances of your class equivalent. This is especially important in complex data structures like 2D arrays, where the automatic reference comparison would not suffice.
Performance Considerations
When dealing with large 2D arrays, performance can become a crucial issue. The deepEquals
method performs an extensive comparison, traversing each element in the arrays recursively. This can lead to performance hits if the arrays are particularly large.
Here are a few tips to optimize performance:
-
Short-Circuiting: If the reference checks (via
==
) are false, you can avoid callingdeepEquals
. This is done internally, but being mindful can help improve performance. -
Avoid Unnecessary Checks: If you already know certain rows or columns are different, skip checking the entire 2D array.
-
Use Hashing: For large datasets, consider using a hash function to create a unique identifier for the array's values. Compare these identifiers before resorting to a full equality check.
To Wrap Things Up
Mastering equality checks in Java's 2D arrays is crucial for developers aiming to build efficient and effective applications. With tools like Arrays.deepEquals
and the ability to implement custom equality checks, you have a robust toolbox at your disposal.
By understanding when to use each method and being aware of performance implications, you can create well-structured and efficient code that meets your application's needs. For further reading on arrays in Java, you can explore Oracle's documentation.
By embracing these techniques, you can ensure that your applications remain performant while handling complex data structures with ease. Happy coding!