Common Pitfalls in Manifold JSON Schema Parsing
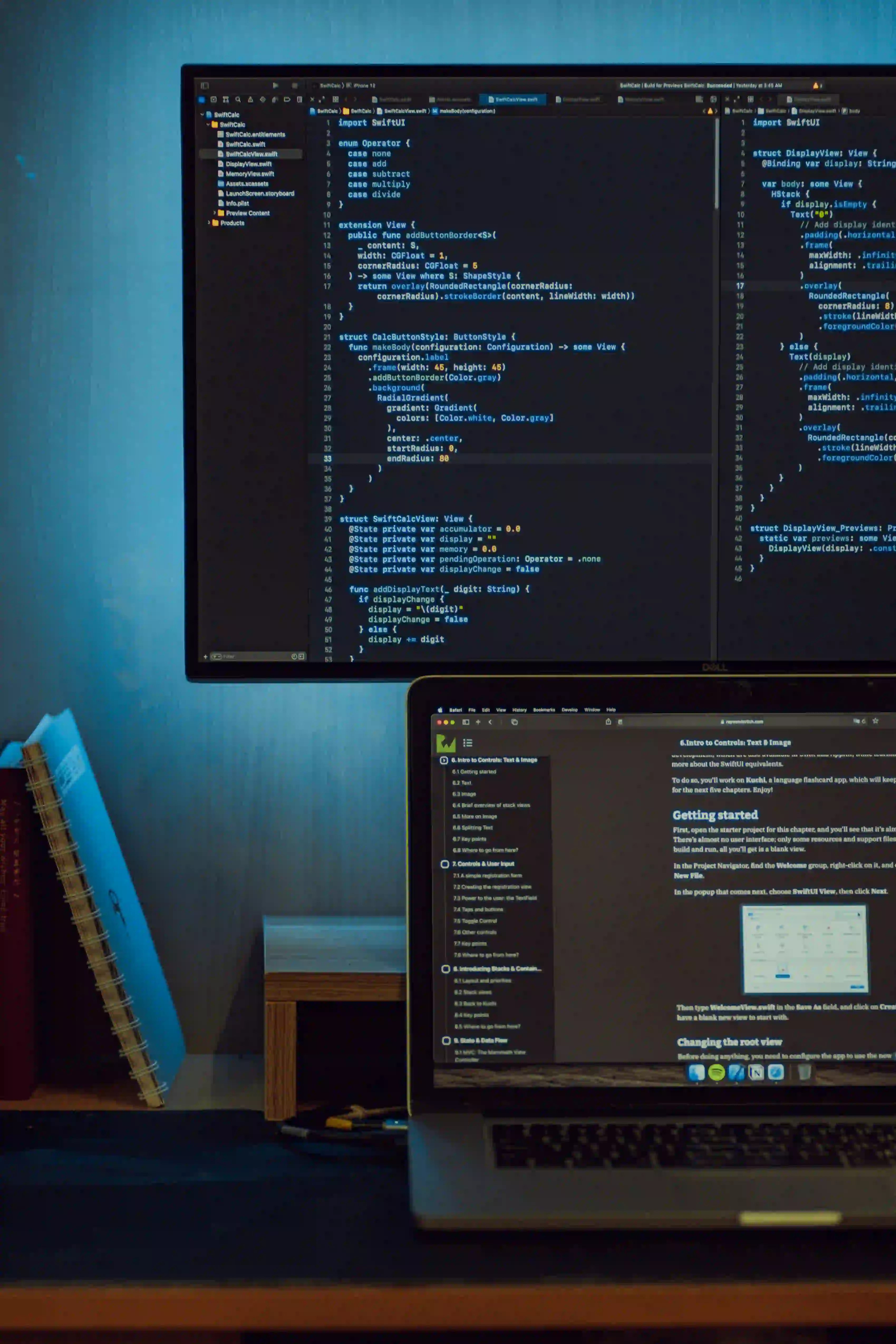
Common Pitfalls in Manifold JSON Schema Parsing
JSON Schema is a powerful tool for validating the structure of JSON data. It’s widely used to define data formats, ensuring compliance and correctness. However, parsing JSON schemas can sometimes lead developers down a rocky path filled with pitfalls. This blog post will explore some of the most common issues encountered when working with JSON schema in Manifold—a dynamic data language—while providing strategies and code examples to help you effectively navigate these challenges.
Understanding JSON Schema
Before diving into the common pitfalls, let’s briefly review what JSON Schema is. JSON Schema defines the structure of JSON documents through a contract. The schema specifies the expected data types, required fields, and other rules. For example, a simple JSON schema could look like this:
{
"$schema": "http://json-schema.org/draft-07/schema#",
"type": "object",
"properties": {
"name": {
"type": "string"
},
"age": {
"type": "integer"
}
},
"required": ["name", "age"]
}
In this schema, we define an object that requires a name
of type string and an age
of type integer.
Why Using Manifold for JSON Schema Parsing?
Manifold provides a rich set of functionalities for parsing schemas because of its emphasis on immutability and functional programming principles. This makes it a popular choice in the Java ecosystem. Manifold allows you to parse and manipulate JSON schema dynamically, aligning neatly with Java's strong type system.
Common Pitfalls
1. Ignoring Schema Versions
One of the biggest misunderstandings is the $schema
keyword. JSON Schema has several drafts with significant differences. Ignoring schema versions can lead to unexpected behaviors and validation failures.
Solution: Always specify the correct $schema
URI in your schema definition.
Example:
{
"$schema": "http://json-schema.org/draft-07/schema#",
"type": "object"
}
2. Neglecting Type Definitions
JSON Schema has specific types, including string
, integer
, boolean
, and more. By failing to define types accurately, you run the risk of legalising incorrect data formats.
Solution: Always validate your data types against the schema.
Example:
import manifold.json.JsonSchema;
JsonSchema schema = JsonSchema.loadFromURL("http://my-schema-url").parse();
String validJson = "{ \"name\": \"John\", \"age\": 30 }";
schema.validate(validJson); // This will validate type correctly
3. Assuming All Fields Are Optional
In JSON Schemas, fields are optional by default unless specified in the required
array. This can result in validation errors that are difficult to track.
Solution: Always check which fields are marked as required.
Example:
{
"type": "object",
"required": ["email"],
"properties": {
"email": {
"type": "string"
}
}
}
4. Misunderstanding Object vs. Array Types
Mixing up between object
and array
types is a common mistake. They have different constraints and rules.
Solution: Clearly understand the distinction. Objects are unordered collections of key/value pairs, while arrays are ordered lists of values.
Example:
{
"type": "array",
"items": {
"type": "string"
}
}
This designates an array of strings, while:
{
"type": "object",
"properties": {
"items": {
"type": "array"
}
}
}
defines an object containing an array under the items
property.
5. Ignoring Conditional Schemas
JSON Schema supports conditional elements through keywords like if
, then
, and else
. Ignoring these features can compromise the validation process.
Solution: Implement conditional logic in your schema as needed.
Example:
{
"if": {
"properties": { "type": { "const": "car" } }
},
"then": {
"required": ["model"]
}
}
6. Failing to Handle Additional Properties
By default, JSON Schema allows additional properties that are not specified in the properties
section. This can lead to unpredicted behavior if the incoming data structure is not controlled.
Solution: Use additionalProperties
to explicitly control this behavior.
Example:
{
"type": "object",
"properties": {
"name": { "type": "string" }
},
"additionalProperties": false
}
In this case, no properties other than name
will be allowed.
7. Complex Validation Logic
As schemas grow more complex, understanding how to handle nested structures gets trickier. Developers may fail to adequately handle deep validations or interdependencies.
Solution: Use a systematic approach to validate nested structures.
Example:
{
"type": "object",
"properties": {
"user": {
"type": "object",
"properties": {
"name": { "type": "string" }
},
"required": ["name"]
}
}
}
8. Relying Solely on Tools for Validation
Many developers assume that tools and libraries will handle all schema validations automatically. This may lead to missing essential data integrity checks.
Solution: Always augment library validations with manual checks, especially when leveraging Manifold, to ensure that data quality meets your application's standards.
The Bottom Line
Navigating JSON Schema parsing in Manifold can be a breeze if you understand these common pitfalls. By keeping an eye on schema versions, defining types correctly, and implementing validation mechanisms thoughtfully, you can significantly enhance the robustness of your applications.
Further reading on JSON Schema can be found at the official JSON Schema documentation. Embrace the challenges of schema validation with confidence, and ensure high-quality data structures in your projects.
With these strategies and examples, you will be better equipped to tackle JSON schema parsing in Manifold. Keep experimenting and learning; the journey towards mastery is continuous!