Mastering Java Concurrency: Overcoming Lock Contention Issues
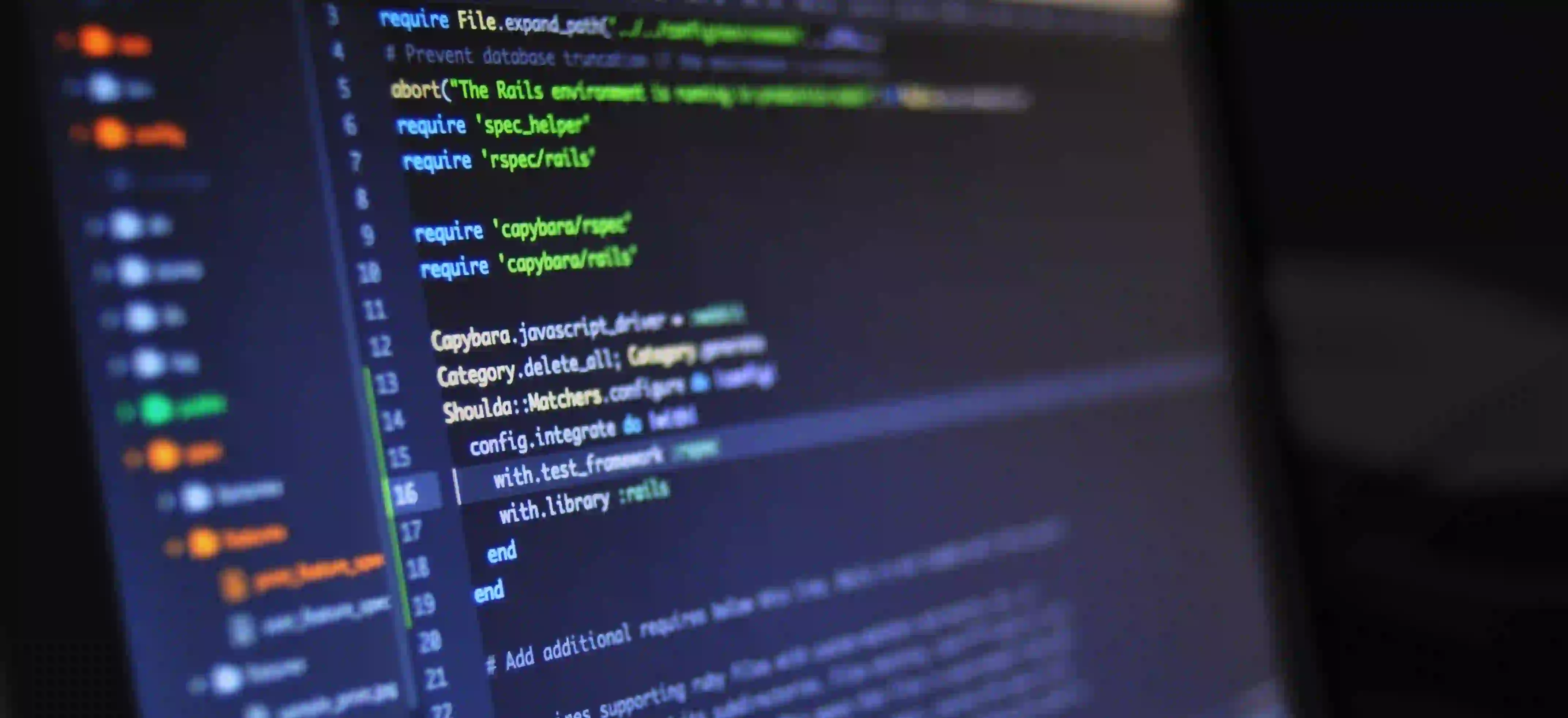
Mastering Java Concurrency: Overcoming Lock Contention Issues
Concurrency in Java is a fundamental aspect that allows developers to execute multiple threads simultaneously. However, as beneficial as concurrency can be, it also introduces complexities, particularly around the issue of lock contention. In this blog post, we will explore what lock contention is, why it occurs, and techniques to overcome it.
Understanding Lock Contention
Lock contention occurs when multiple threads vie for the same lock. Imagine a conference room where several employees want to enter to attend a meeting but can only enter one at a time. When one is inside, others must wait. Similarly, when your application uses synchronized blocks or methods, only one thread can hold the lock for that block or method. If other threads try to access the same synchronized resource, they must wait, leading to performance bottlenecks.
Lock contention can significantly degrade performance especially in multi-threaded applications. Consequently, developers must adopt efficient strategies to minimize it.
Why Lock Contention Happens
To better grasp lock contention, consider the following reasons:
-
Inadequate Granularity of Locks: Using coarse-grained locks (locking large sections of code) leads to high contention as many threads compete for the same lock.
-
Long Holding Times: The longer a thread holds a lock, the more likely others will contend for it. This is especially true for lengthy or blocking operations within synchronized blocks.
-
High Thread Count: A large number of threads increases the chances of contention as more threads will compete for the limited resources.
-
Synchronization Overhead: Java's intrinsic locking mechanism incurs a performance penalty, especially when contention exists.
Strategies to Overcome Lock Contention
1. Use More Granular Locks
Instead of using a single lock for an entire method or data structure, consider breaking your locks down into more manageable parts.
public class Account {
private final Object balanceLock = new Object();
private double balance;
public void deposit(double amount) {
synchronized (balanceLock) {
balance += amount;
}
}
public void withdraw(double amount) {
synchronized (balanceLock) {
balance -= amount;
}
}
public double getBalance() {
synchronized (balanceLock) {
return balance;
}
}
}
Why This Works: By creating a separate lock for the balance, we reduce the contention that might arise if multiple methods were synchronized on the same lock.
2. Opt for ReadWriteLock
In scenarios where reads occur more frequently than writes, using a ReadWriteLock
can improve throughput. This allows multiple threads to read simultaneously while still ensuring exclusive access for writing.
import java.util.concurrent.locks.ReadWriteLock;
import java.util.concurrent.locks.ReentrantReadWriteLock;
public class ReadWriteCounter {
private final ReadWriteLock lock = new ReentrantReadWriteLock();
private int count = 0;
public void increment() {
lock.writeLock().lock();
try {
count++;
} finally {
lock.writeLock().unlock();
}
}
public int getCount() {
lock.readLock().lock();
try {
return count;
} finally {
lock.readLock().unlock();
}
}
}
Why This Works: By allowing concurrent access during read operations, we alleviate bottlenecks when multiple threads are merely reading the count.
3. Leverage the Atomic Classes
Java provides a range of atomic classes that can help in implementing lock-free algorithms. For instance, AtomicInteger
can be used to safely update variables without explicit locking.
import java.util.concurrent.atomic.AtomicInteger;
public class SafeCounter {
private final AtomicInteger count = new AtomicInteger(0);
public void increment() {
count.incrementAndGet();
}
public int getCount() {
return count.get();
}
}
Why This Works: The atomic classes utilize low-level atomic operations and offer thread-safe behavior with reduced overhead compared to traditional locking mechanisms.
4. Utilize Concurrent Collections
Java provides several concurrent collections, such as ConcurrentHashMap
, which are designed to minimize contention. These collections ensure that operations are performed without requiring synchronized blocks on the entire collection.
import java.util.concurrent.ConcurrentHashMap;
public class UserSessions {
private ConcurrentHashMap<String, String> sessions = new ConcurrentHashMap<>();
public void addSession(String userId, String sessionId) {
sessions.put(userId, sessionId);
}
public String getSession(String userId) {
return sessions.get(userId);
}
}
Why This Works: ConcurrentHashMap
allows concurrent access by dividing the map into segments and requiring locks only on individual segments, thus minimizing the chances of contention.
5. Rethink Your Application Design
Sometimes the way an application is architected can lead to contention. Consider using practices such as message queuing or adopting a microservices architecture where different services can operate independently without needing to contend for shared resources.
To Wrap Things Up
Lock contention is a significant challenge in Java concurrency, but it is manageable with the right strategies. By utilizing more granular locks, embracing ReadWriteLock
, leveraging atomic classes, and utilizing concurrent collections, you can minimize potential bottlenecks effectively.
Staying informed and continuously improving your concurrency strategies will ultimately lead to a better-performing application. For those interested in further exploring concurrency in Java, the Java Concurrency in Practice book by Brian Goetz offers a wealth of knowledge.
By understanding and applying these techniques, you can master Java concurrency and ensure your applications run smoothly, even under high loads. If you have any experiences or additional tips to share, feel free to leave a comment below!