Overcoming Common Pitfalls in CompletableFuture Usage
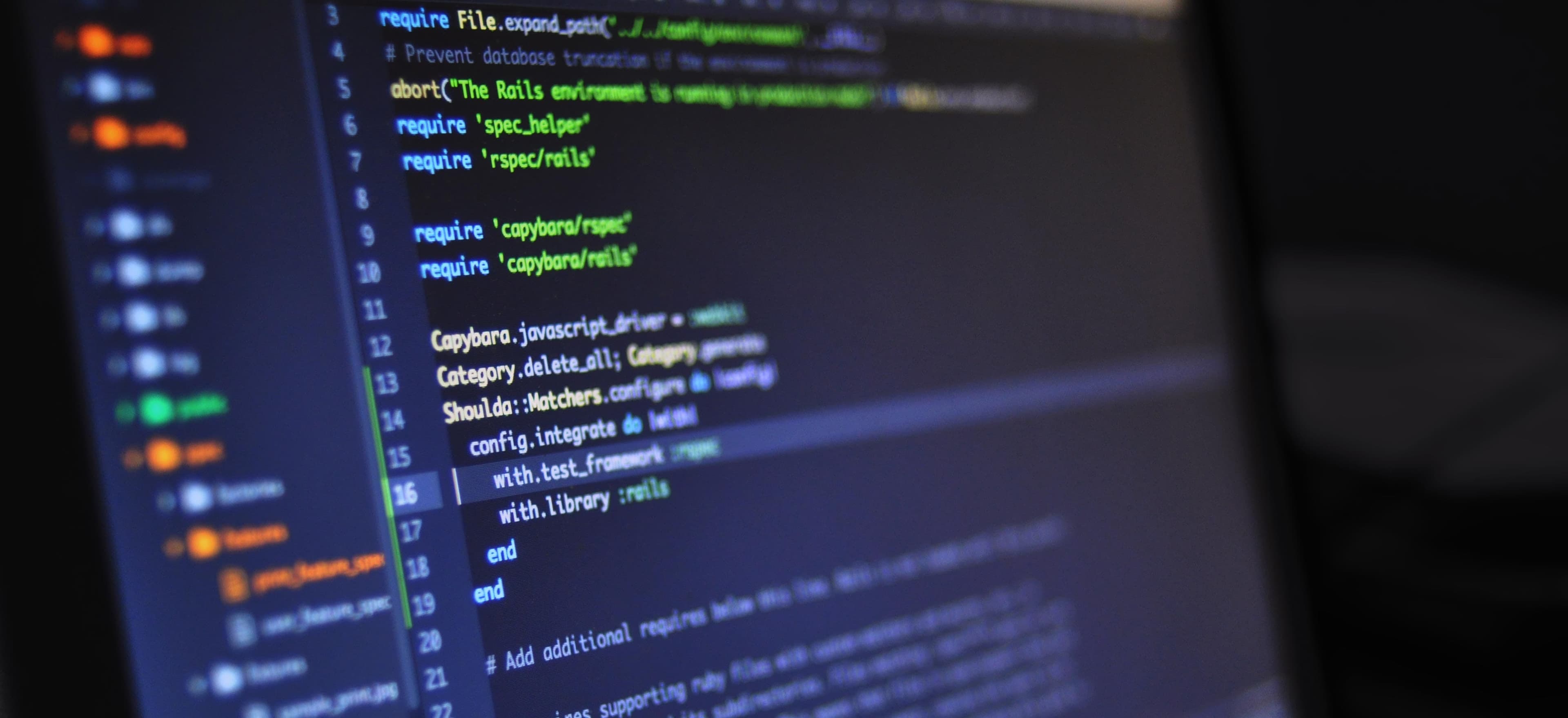
- Published on
Overcoming Common Pitfalls in CompletableFuture Usage
In the world of Java programming, asynchronous programming has become increasingly vital as applications require scalability and responsiveness. The CompletableFuture
class, introduced in Java 8, makes it easier to write non-blocking code. However, despite its powerful capabilities, many developers encounter pitfalls that can lead to subtle bugs and performance issues.
In this blog post, we’ll examine common pitfalls associated with CompletableFuture
usage and explore solutions to ensure that your asynchronous programming is both effective and efficient.
What is CompletableFuture?
Before we dive into the pitfalls, let’s quickly review what CompletableFuture
is. It enables the creation of asynchronous, non-blocking computations with the ability to manage and combine multiple futures. It can manage tasks that are performed in parallel and allows the threading model of your application to be more flexible.
Basic Example of CompletableFuture
Here's a simple example of a CompletableFuture
in action:
import java.util.concurrent.CompletableFuture;
public class CompletableFutureExample {
public static void main(String[] args) {
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> {
// Simulating long-running task
try {
Thread.sleep(2000);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
return "Hello from CompletableFuture!";
});
// Do something else while waiting for the result
System.out.println("Doing something while waiting...");
// Block and get the result
future.thenAccept(result -> System.out.println(result));
// Keep the main thread alive to see the result.
future.join();
}
}
In this example, we create a simple CompletableFuture
, simulate a long-running task using sleep, and print the result after it's complete. The thenAccept
method allows us to perform actions on the result without blocking.
Common Pitfalls and Solutions
While CompletableFuture
can simplify writing asynchronous code, there are several common pitfalls that developers should be aware of. Let’s explore these in detail.
1. Not Handling Exceptions Properly
One major pitfall is neglecting to manage exceptions that may arise during asynchronous computation. If not handled, exceptions can lead to silent failures.
Example of Exception Handling
In this code, we’ll see how to handle exceptions properly in a CompletableFuture
.
import java.util.concurrent.CompletableFuture;
public class ExceptionHandlingExample {
public static void main(String[] args) {
CompletableFuture<Integer> future = CompletableFuture.supplyAsync(() -> {
// Simulating an exception
if (true) {
throw new RuntimeException("Simulated Exception");
}
return 42;
});
future
.exceptionally(ex -> {
System.out.println("Caught exception: " + ex.getMessage());
return -1; // Fallback value
})
.thenAccept(result -> {
System.out.println("Result: " + result);
});
// Keep the main thread alive to see the result.
future.join();
}
}
In this example, the exceptionally
method catches exceptions thrown in the computation, allowing for error recovery while ensuring the program continues running.
2. Blocking the Main Thread
Another common mistake is blocking the main thread by invoking methods like get()
or join()
without considering the implications.
Non-blocking Approach
Instead of blocking the main thread, use asynchronous callbacks and methods like thenApply()
, thenAccept()
, etc. Here’s an improved code sample:
CompletableFuture<Integer> future = CompletableFuture.supplyAsync(() -> {
// Perform some long-running task
return 42;
});
// Use thenApply to process the result asynchronously rather than blocking the main thread
future.thenApply(result -> result * 2)
.thenAccept(result -> System.out.println("Result: " + result));
// It's good practice not to block unless necessary.
In this revised example, thenApply()
processes the result of the original computation asynchronously, keeping the main thread free.
3. Creating too Many CompletableFutures
Creating an excessive number of CompletableFuture
instances can lead to resource exhaustion or overwhelming the thread pool. This is a common concern when dealing with high-frequency tasks.
Managing Executors
To manage this situation effectively, consider using a custom Executor
to limit the number of concurrent threads.
import java.util.concurrent.*;
public class LimitedFutureExample {
public static void main(String[] args) {
ExecutorService executor = Executors.newFixedThreadPool(4); // Limit to 4 concurrent threads
CompletableFuture<Void> future1 = CompletableFuture.supplyAsync(() -> {
// Simulate task
return "Task 1 done";
}, executor).thenAccept(System.out::println);
CompletableFuture<Void> future2 = CompletableFuture.supplyAsync(() -> {
// Simulate task
return "Task 2 done";
}, executor).thenAccept(System.out::println);
// Wait for all futures to complete before shutting down the executor
CompletableFuture.allOf(future1, future2).join();
executor.shutdown();
}
}
Using a limited thread pool allows you to control the resource consumption of your application better.
4. Chained Futures Leading to Confusion
Sometimes, developers create complex chains of futures, which can become difficult to read and debug. As the number of chained stages increases, the control flow can become complicated.
Simplifying with Helper Methods
To mitigate this issue, consider breaking down complex tasks into smaller, manageable methods that can be called sequentially.
public class ChainedFutureExample {
public static void main(String[] args) {
CompletableFuture<Integer> future = CompletableFuture.supplyAsync(() -> computeValue())
.thenApply(value -> addTen(value))
.thenAccept(result -> System.out.println("Result: " + result));
future.join();
}
private static int computeValue() {
// Long running task
return 32;
}
private static int addTen(int value) {
return value + 10;
}
}
This approach makes your code cleaner, more readable, and easier to maintain.
5. Not Using CompletableFuture Combinators
Failing to leverage CompletableFuture
methods like thenCombine()
, thenCompose()
, and allOf()
can lead to redundant code and missed opportunities for concurrent execution.
Example of Using Combinators
Here's an example of combining futures effectively:
import java.util.concurrent.CompletableFuture;
public class CombineFutureExample {
public static void main(String[] args) {
CompletableFuture<Integer> future1 = CompletableFuture.supplyAsync(() -> 10);
CompletableFuture<Integer> future2 = CompletableFuture.supplyAsync(() -> 20);
CompletableFuture<Integer> combinedFuture = future1.thenCombine(future2, Integer::sum);
combinedFuture.thenAccept(result -> {
System.out.println("Combined Result: " + result);
});
combinedFuture.join();
}
}
In this example, thenCombine()
allows you to aggregate results from two asynchronous tasks easily and efficiently.
The Bottom Line
CompletableFuture
can greatly enhance the performance of your Java applications when harnessed correctly. By being mindful of the common pitfalls discussed in this blog post, you can write cleaner, more efficient asynchronous code.
For a deeper dive into asynchronous programming in Java, consider exploring the official Java Documentation on CompletableFuture
.
Take the time to analyze your current implementations and ensure you’re leveraging CompletableFuture
in the best possible manner. Happy coding!
Checkout our other articles