Resolving Dependency Injection Issues in Play Framework with Guice
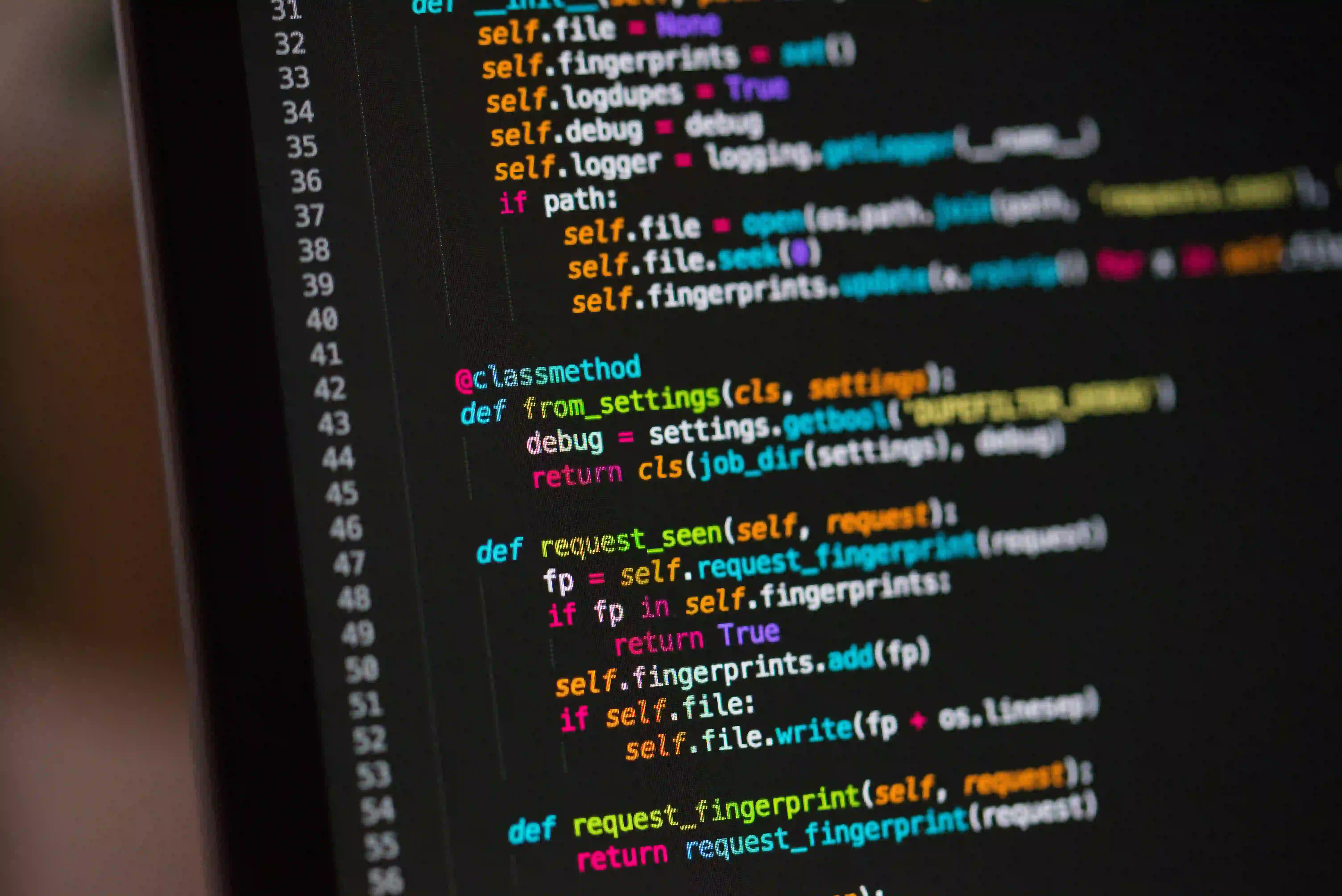
Resolving Dependency Injection Issues in Play Framework with Guice
Dependency Injection (DI) is a critical concept in software development, particularly in modern frameworks. It promotes loose coupling, enhances testability, and fosters better code organization. If you're working with the Play Framework and leveraging Guice for Dependency Injection, you may encounter various issues along the journey. This blog post will highlight common problems, provide solutions, and guide you through best practices when tackling DI-related problems in the Play Framework with Guice.
Understanding Dependency Injection in Play Framework
Play Framework is a powerful tool for building web applications in Java and Scala. At its core, it supports Dependency Injection to manage the components of your application more efficiently. Guice, created by Google, is a popular DI framework that integrates seamlessly with Play.
DI allows you to define your dependencies outside of your classes, which helps to manage the lifecycle of objects. This leads to cleaner, more maintainable code.
Why Guice?
Guice offers several advantages:
- Reduced Boilerplate Code: Guice reduces the amount of code you write for instantiating objects.
- Scalability: The approach easily scales with your application as new components are added.
- Testing: Dependency Injection facilitates testing by allowing for mock implementations.
Common Dependency Injection Issues in Play Framework with Guice
Using DI with Guice is powerful but can sometimes lead to complications if not managed correctly. Let’s discuss some of the most common issues and their solutions.
1. Circular Dependencies
Issue: Circular dependencies occur when two or more classes depend on each other. This can lead to runtime errors, as Guice cannot instantiate classes that reference each other.
Solution: To resolve circular dependencies, you can use constructor injection for one class and setter injection for the other.
Example:
// Class A depends on Class B
public class A {
private B b;
@Inject
public A(B b) {
this.b = b;
}
}
// Class B depends on Class A
public class B {
private A a;
@Inject
public void setA(A a) {
this.a = a;
}
}
In this example, Class A uses constructor injection, while Class B uses setter injection to avoid a direct circular reference during instantiation.
2. Missing Bindings
Issue: Sometimes, you might forget to bind a class or interface in the Guice module, leading to a ProvisionException
.
Solution: Ensure that all dependencies are properly bound in the Guice module.
Example:
public class Module extends AbstractModule {
@Override
protected void configure() {
bind(MyService.class).to(MyServiceImpl.class);
}
}
In this code snippet, we bind the MyService
interface to its implementation MyServiceImpl
. If this binding is omitted, any attempt to inject MyService
will throw a runtime exception.
3. Scoping Issues
Issue: Scoping defines the lifespan of a binding. For instance, if you mistakenly bind a singleton service within a request scope, it can lead to unexpected behavior.
Solution: Be clear about the scope of your services. Use @Singleton
for singleton services and @RequestScoped
for request-dependent services.
Example:
public class Module extends AbstractModule {
@Override
protected void configure() {
bind(MyRequestScopedService.class).in(RequestScoped.class);
bind(MySingletonService.class).in(Singleton.class);
}
}
Ensure you are aware of how scope affects the lifespan of your dependencies.
4. Constructor Injection vs. Field Injection
Issue: Field injection is simpler but can introduce hard-to-test code. When classes are test-agnostic, it can lead to complications in unit tests.
Solution: Prefer constructor injection over field injection wherever possible.
Example:
Instead of:
public class MyService {
@Inject
private MyDependency dep;
// Do something with dep
}
Use:
public class MyService {
private final MyDependency dep;
@Inject
public MyService(MyDependency dep) {
this.dep = dep;
}
// Do something with dep
}
This approach makes your classes easier to test, as you can pass mocks and spies into the constructor.
5. Missing Annotations
Issue: You may forget to annotate your constructor or method with @Inject
, leading to DI failures.
Solution: Always ensure that your constructors or setter methods intended for DI are annotated with @Inject
.
Example:
public class Service {
private final Repository repository;
@Inject // Required to be recognized by Guice
public Service(Repository repository) {
this.repository = repository;
}
}
For Guice to recognize the dependency, it's imperative to use the @Inject
annotation.
Best Practices for Dependency Injection with Guice
Now that we’ve covered common issues, let’s discuss a few best practices:
1. Keep Scope Clear
Always define scopes clearly based on service requirements. This ensures that your components behave as expected throughout various lifecycle phases of your application.
2. Limit the Use of Singleton
Singletons can become a bottleneck if not used wisely. Use them for stateless services or shared resources, but avoid them where mutable state exists.
3. Favor Interfaces over Concrete Classes
Binding interfaces to implementations allows for greater flexibility and promotes cleaner code. This practice enhances testability by allowing dependency injection of mock objects in tests.
4. Use Provider Interfaces
In certain cases where bindings can’t be resolved immediately, consider using the Provider
interface.
Example:
public class MyService {
private final Provider<MyDependency> dependencyProvider;
@Inject
public MyService(Provider<MyDependency> dependencyProvider) {
this.dependencyProvider = dependencyProvider;
}
public void performAction() {
MyDependency dep = dependencyProvider.get(); // Lazily injected
dep.doSomething();
}
}
This defers the creation of MyDependency
, allowing for lazy instantiation when needed, reducing overhead.
5. Monitor and Optimize Performance
Be sure to monitor and optimize your dependency injection configurations. Overhead can occur with complex graphs of dependencies. Use tools like VisualVM or JProfiler for profiling your application.
Closing Remarks
Resolving Dependency Injection issues in Play Framework with Guice can be daunting but rewarding. Implementing the right strategies for binding, scoping, annotations, and constructor injection can lead to a vastly improved codebase.
By adhering to best practices and staying aware of pitfalls, your application will be more maintainable, scalable, and easier to test. As you work more with Guice and Play, these principles will solidify the robustness of your work.
By following the guidelines provided in this blog post, you can navigate through common DI challenges effectively and remain focused on delivering high-quality applications.
Feel free to check out resources like the Play Framework Documentation and Guice Documentation for in-depth knowledge.
Happy coding!