Common Pitfalls in Spring Reactive Programming You Should Avoid
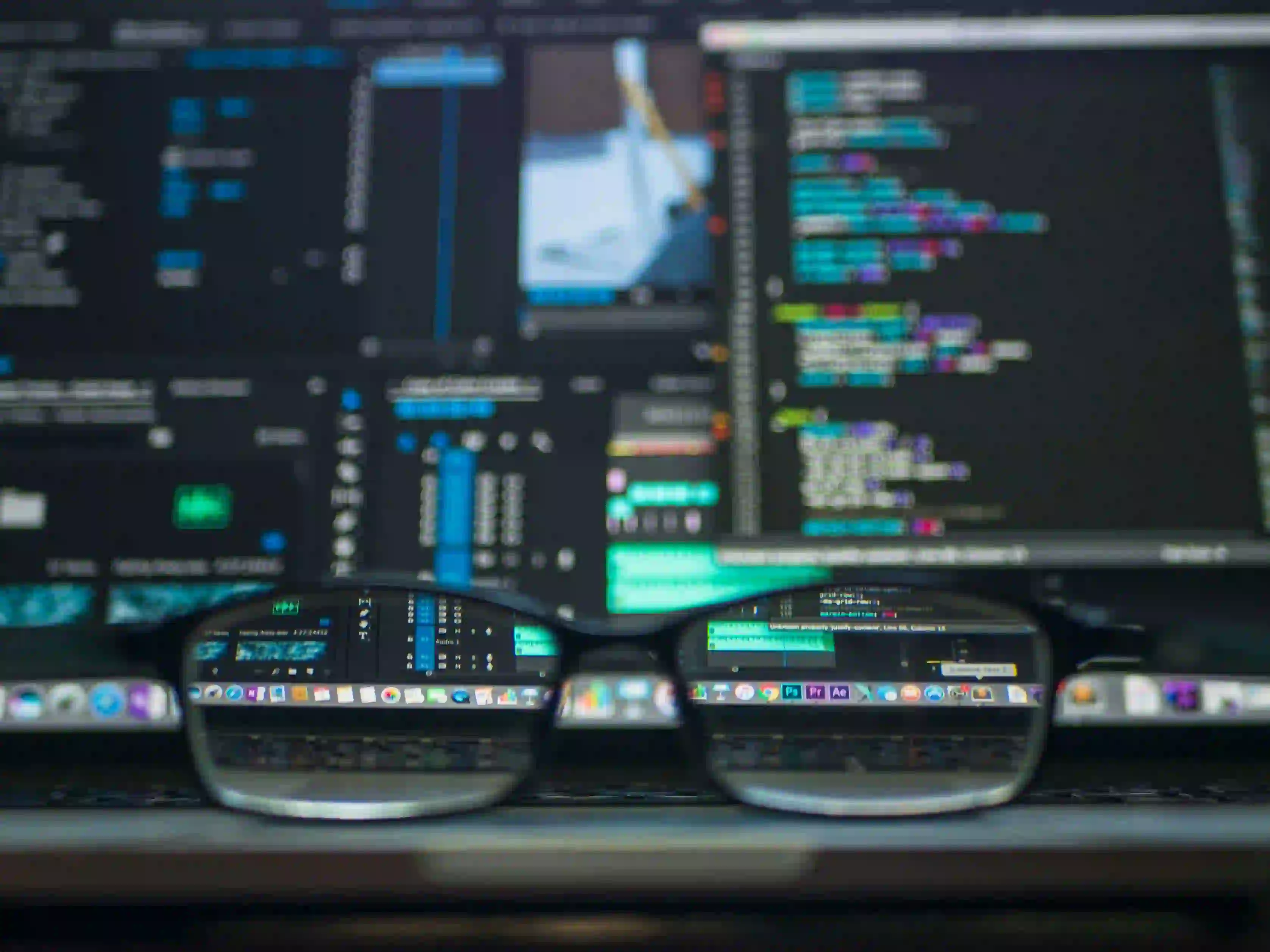
Common Pitfalls in Spring Reactive Programming You Should Avoid
Spring Reactive programming has gained traction thanks to its ability to provide non-blocking, asynchronous data streams. It allows developers to build applications that are efficient and responsive. However, with great power comes great responsibility. Many developers encounter common pitfalls that can lead to performance issues, bugs, and reactive programming confusion.
In this blog post, we will explore some of these common pitfalls and summarize how to avoid them. By understanding these challenges, you'll be in a better position to leverage the full potential of Spring Reactive programming.
1. Ignoring Backpressure
Backpressure is an essential concept in reactive programming. It is a mechanism that helps manage the flow of data between producers and consumers. When a producer generates data faster than a consumer can process it, the consumer faces overwhelming data loads.
How to Properly Handle Backpressure
Spring WebFlux (the web framework for reactive programming in Spring) provides operators like onBackpressureBuffer()
and onBackpressureDrop()
that help manage backpressure effectively.
Here is an example:
Flux<Integer> flux = Flux.range(1, 100)
.onBackpressureBuffer(10, dropped -> System.out.println("Dropped: " + dropped));
Why This Matters: By properly handling backpressure, you ensure that your application doesn’t become slow or crash under high loads.
Learn more about Backpressure in Reactive Programming.
2. Blocking Calls in Reactive Code
One of the foundational principles of reactive programming is non-blocking calls. Developers sometimes unknowingly use blocking operations, which can negate the advantages of reactive programming.
Consider the following example:
Mono<String> mono = Mono.fromCallable(() -> {
Thread.sleep(1000); // Blocking the thread
return "Hello, World!";
});
Why This is a Problem:
The code above introduces blocking behavior. This can cause thread pool exhaustion when many such calls are made concurrently. Aim to use reactive alternatives, like subscribeOn()
and publishOn()
, which help keep the flow non-blocking.
Avoiding Blocking Behavior
Always prefer non-blocking methods when working within a Reactive Pipeline. If you need to make blocking calls, ensure you do it on a different thread, such as:
Mono<String> nonBlockingMono = Mono.fromCallable(() -> {
// Perform your blocking call here
})
.subscribeOn(Schedulers.boundedElastic());
By isolating blocking logic, you can maintain overall responsiveness.
3. Overusing Thread Management Operators
Using the wrong threading strategy in a reactive application can lead to performance bottlenecks. Developers sometimes overuse operators like subscribeOn
and publishOn
, which can introduce unnecessary context switching.
Correct Thread Handling
Instead of scattering your application with various thread management calls, decide on a consistent threading strategy. Use them primarily when you need a specific thread context.
For example:
Flux.range(1, 10)
.subscribeOn(Schedulers.elastic()) // Use elastic only once where necessary
.map(i -> heavyComputation(i))
.publishOn(Schedulers.parallel())
.subscribe(System.out::println);
Why It Matters: Clear and intentional thread management leads to optimized resource use, reducing context switching that can lead to CPU overhead and latency issues.
4. Not Using Project Reactor Properly
Project Reactor is the foundation for building reactive applications in Spring. If not used properly, it can lead to unexpected issues. For instance, using the wrong types (like Flux
instead of Mono
) might not raise compile-time errors but can lead to runtime errors.
Example of Inappropriate Use
Imagine you’re dealing with a single value but instead, opt for a Flux:
Flux<String> flux = Flux.just("Single Value");
// This can lead to confusion regarding expected behaviors.
Recommended Practice
Use Mono
for single values and Flux
for multiple values to clarify your intention.
Mono<String> mono = Mono.just("Single Value"); // Clear context
Avoid Confusion: Understanding and using Project Reactor's types correctly enhances both readability and maintainability of your code.
5. Ignoring Error Handling
Error handling is crucial in any programming paradigm, and the reactive approach is no exception. It is easy to overlook error scenarios in the reactive flow, which can screw up the entire chain.
Error Handling Example
Here is how you can manage errors gracefully in a reactive stream:
Mono<String> mono = Mono.just("Hello")
.map(value -> {
if (true) throw new RuntimeException("An error occurred!"); // Intentional error
return value;
})
.doOnError(e -> System.out.println("Caught error: " + e.getMessage()))
.onErrorReturn("Fallback Value"); // Providing a fallback
Why Error Handling is Critical: Implementing robust error handling ensures that your application remains resilient. Without handling potential errors, you risk causing disruptions in your application flow.
6. Forgetting to Release Resources
Reactive programming often deals with resource management, such as database connections or HTTP requests. Not releasing resources can lead to memory leaks.
Resource Management Example
Utilize operators like doFinally()
or using()
from Reactor:
Mono<String> resourceMono = Mono.using(
() -> acquireResource(), // Acquire resource
resource -> processResource(resource),
resource -> releaseResource(resource) // Ensure resource release
);
Why Resource Management Matters: Proper resource lifecycle management keeps your application lightweight and efficient. This practice significantly mitigates the risk of memory-related issues.
A Final Look
Spring Reactive programming offers a powerful paradigm for building scalable applications, but it comes with its own set of challenges. By being aware of these common pitfalls—from managing backpressure to handling errors and resources—you can avoid potential issues and create efficient, responsive applications.
For further reading, consider diving into the official Spring documentation and Project Reactor Guides.
Reactive programming is an exciting field with plenty of room for growth. As you continue your journey in mastering Spring Reactive, keep these pitfalls in mind to ensure a smoother development experience. Happy coding!