Streamlining JDBC ResultSet Processing: Common Pitfalls
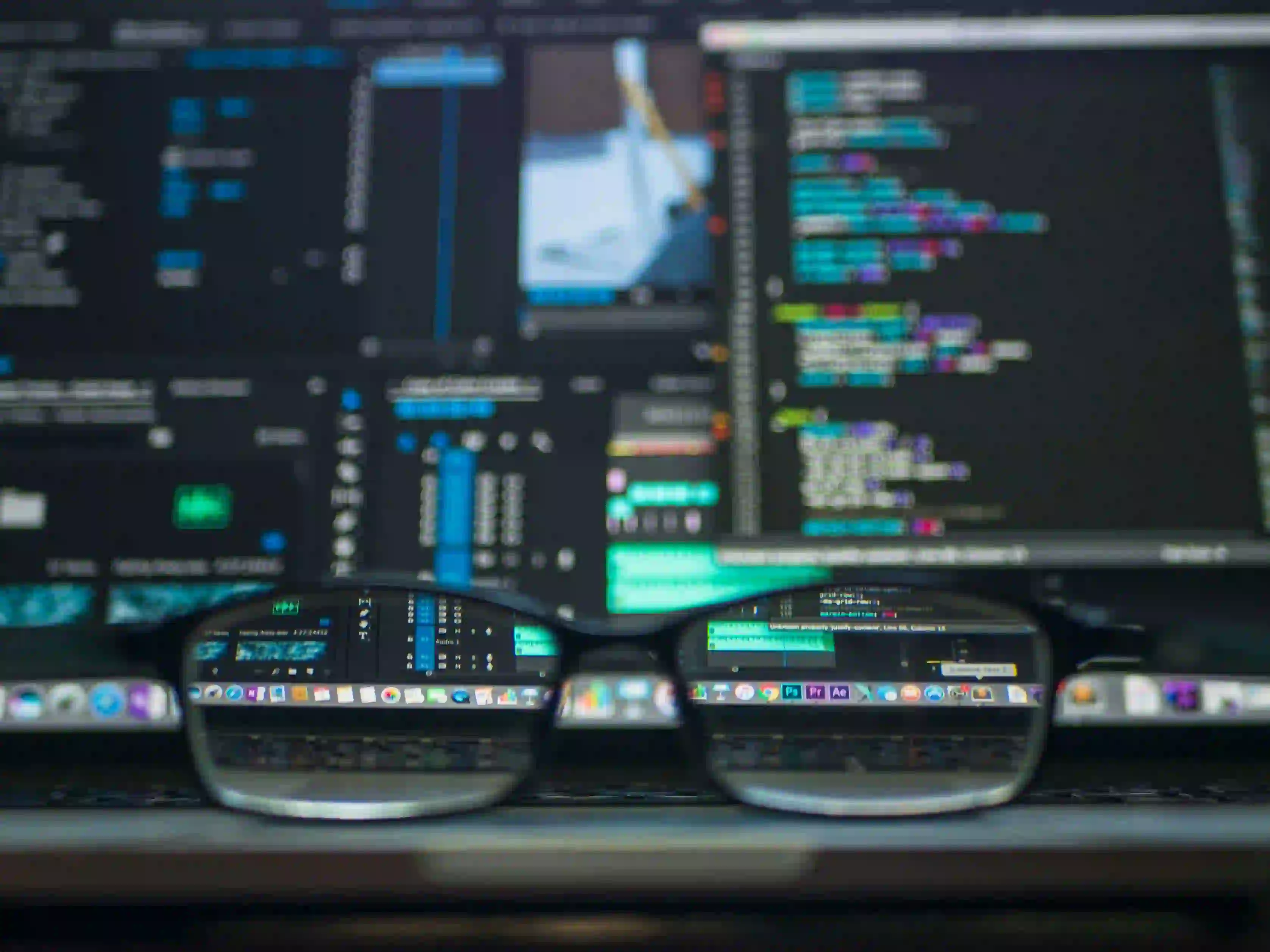
Streamlining JDBC ResultSet Processing: Common Pitfalls
Many Java developers interact with databases using JDBC (Java Database Connectivity). While JDBC is a powerful and flexible API, it comes with its share of challenges, especially when processing ResultSet
objects. In this post, we will explore common pitfalls in ResultSet
processing, and provide strategies to streamline your database operations effectively.
Understanding ResultSet Basics
ResultSet
is an interface that represents the result set of a query. It provides methods to read data from the query results. Here’s a basic outline of how you typically create a ResultSet
:
Connection connection = DriverManager.getConnection(DB_URL, USER, PASS);
Statement statement = connection.createStatement();
ResultSet resultSet = statement.executeQuery("SELECT * FROM users");
In this example, we connect to a database and execute a simple SQL query to retrieve all users.
Important Note on Resource Management
Always remember to close your ResultSet
, Statement
, and Connection
objects to prevent memory leaks. You can do this in a finally
block or use the try-with-resources statement available since Java 7.
try (Connection connection = DriverManager.getConnection(DB_URL, USER, PASS);
Statement statement = connection.createStatement();
ResultSet resultSet = statement.executeQuery("SELECT * FROM users")) {
// Process the ResultSet
} catch (SQLException e) {
e.printStackTrace();
}
Common Pitfalls in ResultSet Processing
1. Ignoring ResultSet Metadata
One of the most commonly overlooked aspects of handling a ResultSet
is the metadata it provides. The metadata allows you to understand the structure of the result set without hard-coding column names and indexes.
Example:
Instead of assuming the order of columns, leverage ResultSetMetaData
:
ResultSetMetaData metaData = resultSet.getMetaData();
int columnCount = metaData.getColumnCount();
for (int i = 1; i <= columnCount; i++) {
String columnName = metaData.getColumnName(i);
System.out.println("Column " + i + ": " + columnName);
}
Why this matters: Relying on hard-coded indexes or column names can lead to errors if the underlying database changes. Using metadata makes your code more robust and flexible.
2. ResultSet Not Iterated Properly
Another pitfall is not properly iterating through the ResultSet
. Developers sometimes forget that the cursor starts before the first row and needs to be explicitly moved to the first row.
Correct Iteration Process:
if (resultSet.next()) { // Moves the cursor to the first row
do {
// Read data from the ResultSet
} while (resultSet.next()); // Continue until no more rows
}
Why this matters: Failing to call next()
can lead to skipping all rows or throwing exceptions when trying to read data.
3. Not Using Appropriate Data Types
Java's type system is strong, and using incorrect data types while retrieving values can lead to SQLException
. Each column in your ResultSet
should be accessed with the appropriate method.
Example of Proper Data Type Handling:
String username = resultSet.getString("username"); // For VARCHAR
int age = resultSet.getInt("age"); // For INTEGER
Why this matters: Using the correct types ensures that your application behaves as expected and reduces the chance of runtime exceptions.
4. Not Handling Null Values
Null values in databases need special handling. If you try to retrieve a value directly without checking for null, you can end up with a NullPointerException
.
Null Handling:
String phoneNumber = resultSet.getString("phone_number");
if (resultSet.wasNull()) {
phoneNumber = "N/A"; // or handle it accordingly
}
Why this matters: Proper null handling ensures that your application can gracefully deal with database records containing missing values.
5. Inefficient ResultSet Processing
Sometimes, developers process results inefficiently. For example, calling getString()
multiple times for the same column in a single loop iteration is redundant.
Example of Streamlined Processing:
while (resultSet.next()) {
String username = resultSet.getString("username");
String email = resultSet.getString("email");
// Process results
}
Why this matters: Reading the same column multiple times can impact performance, particularly with large datasets. Caching values locally can help mitigate this.
Best Practices for JDBC ResultSet
Processing
- Use Prepared Statements: Always favor
PreparedStatement
overStatement
to avoid SQL