Struggling with Java Heap Size? Here’s Your Ultimate Guide!
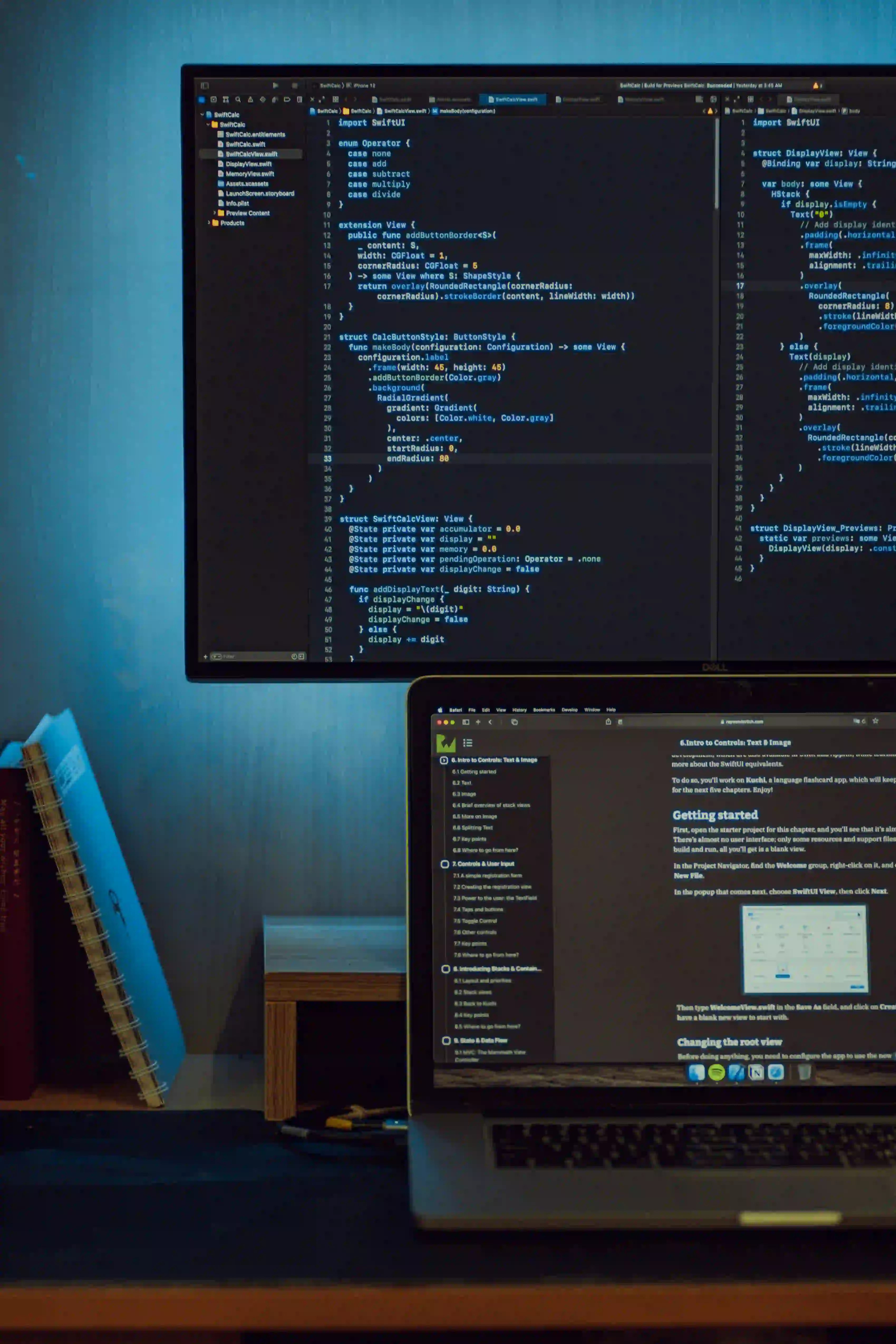
Struggling with Java Heap Size? Here’s Your Ultimate Guide!
Java is an immensely popular programming language, renowned for its versatility and robustness. However, developers often encounter challenges regarding memory management, particularly with heap size. If you find yourself grappling with heap size issues, you've come to the right place. This ultimate guide will provide insights into Java heap memory, how to optimize it, and code snippets to illustrate key concepts.
Understanding Java Heap Memory: The Basics
The Java Virtual Machine (JVM) uses a specific memory area called the heap for dynamic memory allocation. The heap is where Java Objects reside, and it plays a critical role in the performance of Java applications.
Why is HEAP Memory Important?
Heap memory in Java is crucial for several reasons:
-
Object Storage: Java objects are created and stored in the heap. This means that the more objects you create, the more memory you need.
-
Garbage Collection: The JVM automatically manages memory through a process called garbage collection (GC). If there is not enough heap space for new objects, a GC process will kick in.
-
Performance: Inefficient heap management can lead to performance issues, including slow response times and memory-related errors.
The Structure of the Java Heap
The heap in the JVM is divided into several generations to optimize memory management:
-
Young Generation: This is where all new objects are allocated. It includes Eden space and Survivor spaces.
-
Old Generation: Objects that survive garbage collection in the young generation are promoted to the old generation.
-
Permanent Generation (Metaspace in Java 8 and later): This area stores metadata about classes and methods.
Code Insight: Analyzing Java Heap Usage
To understand how memory is used, we can visualize the allocation of Java heap.
import java.util.ArrayList;
import java.util.List;
public class HeapAnalysis {
public static void main(String[] args) {
List<String> longStrings = new ArrayList<>();
// Creating thousands of Strings to fill heap memory
for (int i = 0; i < 100000; i++) {
longStrings.add("This is a long string " + i);
}
// At this point, be cautious of the heap size.
System.out.println("Heap memory filled with string objects.");
}
}
Why this works: The program creates a list of long strings and fills the heap memory rapidly. If your application crashes or shows an "OutOfMemoryError," it indicates the heap is filling up.
Common Heap Size Problems
1. OutOfMemoryError
This is the most common issue you might encounter. It typically occurs when there is not enough memory in the heap for new objects.
There are various types of OutOfMemoryError
:
- Java heap space: Indicates a shortage of memory in the heap.
- GC overhead limit exceeded: Suggests that the garbage collector is spending too much time cleaning up but is not reclaiming sufficient memory.
2. Memory Leaks
A memory leak happens when objects that are no longer needed are still referenced in the application. This prevents the garbage collector from freeing that memory, leading to memory exhaustion over time.
Tuning Java Heap Size
One of the most effective ways to manage heap memory is by tuning its size. Here’s how to do it:
Setting Heap Size Parameters
You can set the minimum and maximum heap size using the following JVM arguments:
java -Xms512m -Xmx2048m YourApp
-Xms
sets the initial heap size (in this case, 512 MB).-Xmx
sets the maximum heap size (in this case, 2048 MB).
Code Insight: Monitoring Heap Usage
To monitor heap memory during execution, we can use the following code snippet alongside JVM parameters.
public class HeapMonitor {
public static void main(String[] args) {
Runtime runtime = Runtime.getRuntime();
// Initial heap size
System.out.println("Initial Heap Size: " + (runtime.totalMemory() / 1024 / 1024) + "MB");
// Allocating memory
int[] array = new int[10000000];
// Heap size after allocation
System.out.println("Heap Size After Allocation: " + (runtime.totalMemory() / 1024 / 1024) + "MB");
}
}
Why monitor: This code allows you to see how much memory is being consumed before and after the allocation. Observing these changes can help in optimizing your application's heap usage.
Analyzing and Optimizing Heap Usage
Effective heap analysis can inform necessary adjustments. Here are some tools and techniques to help:
Tools for Heap Analysis
-
VisualVM: This powerful tool can provide insights into memory usage, CPU usage, and thread activity.
-
Java Mission Control: It allows developers to monitor and manage Java applications at runtime, providing profiling, monitoring, and diagnostics features.
-
Eclipse Memory Analyzer (MAT): This tool helps in analyzing memory leaks and reducing memory consumption.
Garbage Collection Strategies
Different garbage collection algorithms can be employed depending on your application's needs.
- Serial GC: Suitable for smaller applications with limited memory.
- Parallel GC: Aimed at multi-threaded applications.
- G1 GC: The default garbage collector since Java 9, designed for larger heaps and multi-core systems.
Lessons Learned
Understanding and optimizing Java heap size is crucial for successful Java application development. By monitoring heap usage, tuning JVM parameters, and utilizing the appropriate tools, you can improve application performance and prevent memory-related issues.
For more information on Java memory management, check out this detailed guide on garbage collection in Java.
Further Reading
- Java Performance Tuning - Oracle provides insights into best practices for Java performance.
By implementing these practices and paying careful attention to heap management, you can ensure your Java applications run smoothly, efficiently, and free of memory-related issues. Happy coding!