Avoid These 10 SQL Mistakes Every Java Developer Makes
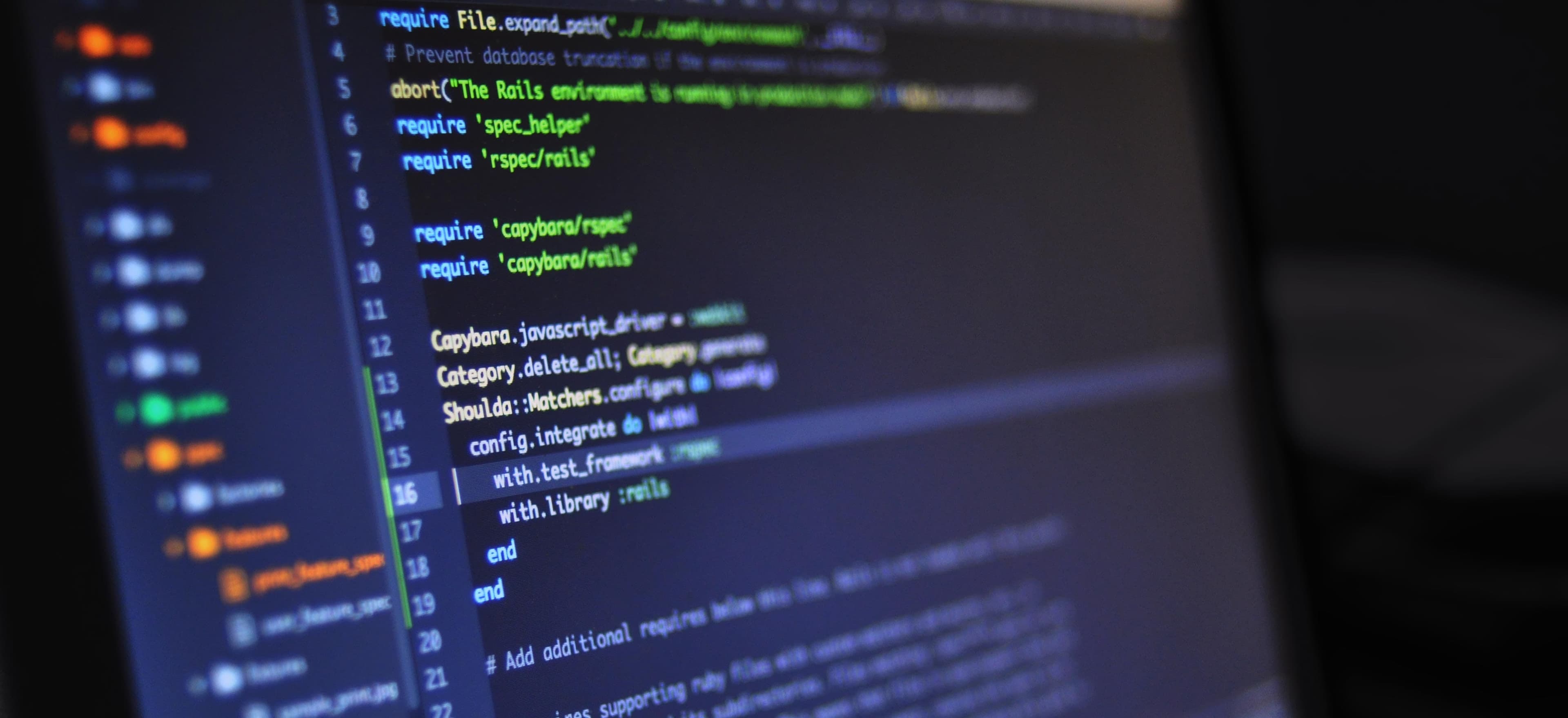
- Published on
Avoid These 10 SQL Mistakes Every Java Developer Makes
SQL and Java are a powerful combination for many developers. However, missteps can lead to inefficient queries, security vulnerabilities, and application bugs. In this blog post, we will explore ten common SQL mistakes that Java developers often make and how to avoid them.
1. Not Using Prepared Statements
The Mistake
One of the most prevalent pitfalls is the use of string concatenation to build queries. Not only is this inefficient, but it also exposes your application to SQL injection attacks.
Solution
Utilizing prepared statements is essential to improve security and performance.
Example
String query = "SELECT * FROM users WHERE username = ? AND password = ?";
PreparedStatement preparedStatement = connection.prepareStatement(query);
preparedStatement.setString(1, username);
preparedStatement.setString(2, password);
Prepared statements ensure that the SQL engine can parse the statement before it's executed, significantly reducing the risk of SQL injection.
Why It Matters
Prepared statements help to protect the integrity of your database and improve performance by allowing the SQL engine to cache the execution plan.
2. Ignoring ResultSet Closing
The Mistake
Failing to close the ResultSet can result in memory leaks and connection exhaustion.
Solution
Always close your ResultSet in a finally block to ensure it closes regardless of whether an exception occurs.
Example
ResultSet resultSet = null;
try {
resultSet = preparedStatement.executeQuery();
while (resultSet.next()) {
// Process the result set
}
} finally {
if (resultSet != null) {
resultSet.close();
}
}
This approach guarantees that resources are released efficiently.
Why It Matters
Closing the ResultSet promptly helps to free up database connections and resources, leading to better application performance and reliability.
3. Not Using Transactions
The Mistake
Performing multiple database operations without managing transactions is risky. Incomplete operations can leave your database in an inconsistent state.
Solution
Use transaction management to handle commits and rollbacks effectively.
Example
try {
connection.setAutoCommit(false);
// Execute multiple SQL commands
connection.commit();
} catch (SQLException e) {
connection.rollback();
} finally {
connection.setAutoCommit(true);
}
By wrapping your database operations in a transaction, you ensure atomicity.
Why It Matters
Transactions guarantee that either all operations succeed or none do, promoting data integrity.
4. Overlooking Indexing
The Mistake
Not creating indices on frequently queried columns can drastically slow down query performance.
Solution
Analyze your queries and establish appropriate indexes.
Example
CREATE INDEX idx_username ON users (username);
This index will allow fast lookups based on the username column.
Why It Matters
Indexes can significantly boost query performance, especially on larger datasets. Understanding when and where to use them is crucial for efficient database interactions.
5. Using SELECT *
The Mistake
Utilizing SELECT *
retrieves all columns, which can result in unnecessary data transfer and processing.
Solution
Explicitly specify the columns you need.
Example
String query = "SELECT id, username FROM users WHERE active = ?";
This results in a more efficient query by limiting the amount of data processed.
Why It Matters
By selecting only necessary columns, you reduce data transfer times and memory usage.
6. Forgetting to Handle Exceptions
The Mistake
Poorly managed exceptions can obscure errors and lead to unexpected application behavior.
Solution
Implement robust error handling.
Example
try {
// Database operations
} catch (SQLException e) {
System.err.println("SQL Exception: " + e.getMessage());
}
Captured exceptions can guide you in diagnosing issues effectively.
Why It Matters
Clear exception handling leads to easier debugging and a more stable application.
7. Not Normalizing Data
The Mistake
Storing redundant data within the same table leads to anomalies and increased storage requirements.
Solution
Normalize your database schema.
Example
Instead of:
CREATE TABLE orders (
order_id INT,
customer_name VARCHAR(100),
customer_address VARCHAR(255)
);
Instead, normalize it:
CREATE TABLE customers (
customer_id INT PRIMARY KEY,
name VARCHAR(100),
address VARCHAR(255)
);
CREATE TABLE orders (
order_id INT,
customer_id INT,
FOREIGN KEY (customer_id) REFERENCES customers(customer_id)
);
Normalization eliminates redundancy and improves data integrity.
Why It Matters
A normalized database design enhances data consistency and manageability.
8. Inadequate Use of Joins
The Mistake
Using too many joins or not understanding their types can lead to performance issues or incorrect results.
Solution
Understand different types of joins and use them appropriately for your queries.
Example
SELECT o.order_id, c.name FROM orders o
INNER JOIN customers c ON o.customer_id = c.customer_id;
This inner join retrieves only records that have corresponding entries in both tables.
Why It Matters
Knowing how to effectively use joins optimizes data retrieval and reduces complexity in data handling.
9. Assuming Single-threaded Database Access
The Mistake
Java applications often have multiple threads working with the database. Assuming single-threaded access can lead to concurrency issues.
Solution
Implement appropriate concurrency controls when needed, such as optimistic or pessimistic locking.
Example
// Optimistic locking using a version field
String query = "UPDATE users SET version = version + 1 WHERE id = ? AND version = ?";
Why It Matters
Concurrency controls prevent data inconsistencies and ensure smooth database interactions across multiple threads.
10. Not Analyzing Query Performance
The Mistake
Neglecting to analyze how your SQL queries perform can lead to slow applications.
Solution
Use tools like EXPLAIN
statement for performance insights.
Example
EXPLAIN SELECT * FROM users WHERE username = 'testUser';
This informs you about how the SQL engine processes your query and where optimizations can be implemented.
Why It Matters
Regular analysis ensures that your queries remain efficient as your dataset grows.
To Wrap Things Up
SQL interaction is an essential component of Java development, yet many overlook best practices. From leveraging prepared statements to managing transactions and indexing, small adjustments can yield significant improvements in application performance and security.
To learn more about optimizing your SQL skills and avoiding common pitfalls, check out SQL Performance Tuning.
By being mindful of these ten SQL mistakes, you can enhance your Java applications' efficiency, security, and reliability. Remember, a well-structured database and appropriately optimized queries are cornerstones of successful software development. Happy coding!
Checkout our other articles