Overcoming Common Challenges in Mutation Testing
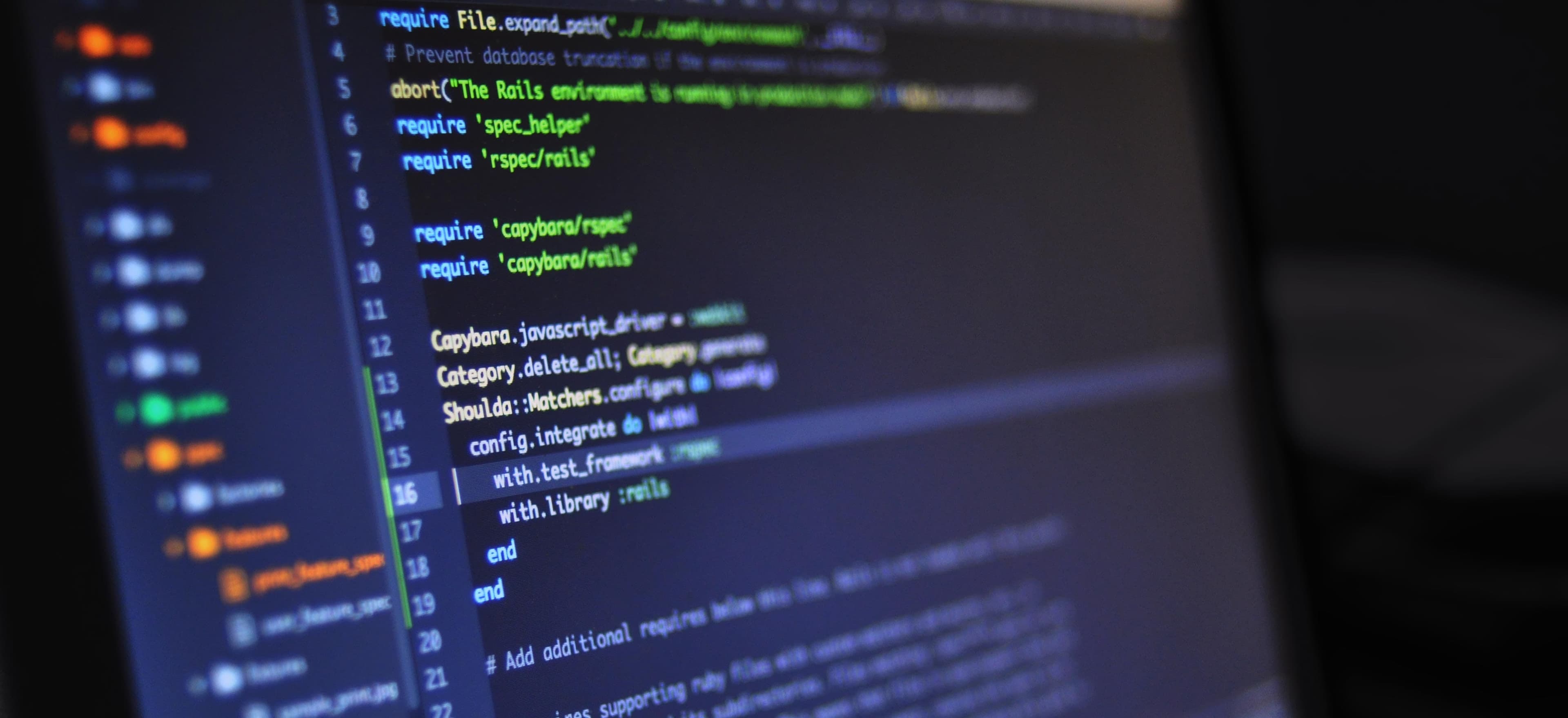
- Published on
Overcoming Common Challenges in Mutation Testing
Mutation testing is a powerful technique for evaluating and improving the quality of your software tests. By intentionally introducing changes (mutations) to your code, you can check whether your test suite is robust enough to catch these modifications. While it offers significant advantages, mutation testing presents certain challenges that developers must overcome. In this blog post, we'll delve into these challenges and provide practical solutions, complemented by Java examples.
What is Mutation Testing?
Mutation testing creates small, systematic changes to the source code to assess the effectiveness of test cases. If your tests can detect the changes (or "mutants"), they are deemed effective. If they cannot, it indicates a gap in your tests, prompting you to refine them.
For further reading on the fundamentals of mutation testing, you can refer to this introduction.
Common Challenges in Mutation Testing
- Performance Issues
- Complexity in Test Case Design
- High Number of Mutants
- Integration with Existing Test Frameworks
- Value of the Mutation Score
Let's explore each challenge and how to overcome it.
1. Performance Issues
The Challenge
One of the primary hurdles in mutation testing is performance. Running tests against millions of mutants can drastically slow down the testing process. This can become untenable as codebases grow.
The Solution
Employ selective mutation strategies. Instead of testing against all mutants, focus on those most likely to be significant or relevant to your current code changes. Tools like PIT (a popular mutation testing tool for Java) allow you to configure the mutations you want to focus on.
public class Calculator {
public int add(int a, int b) {
return a + b; // Mutate '+' to '-' to create a mutant
}
}
In this example, if you suspect that the addition method is well-covered, you could prioritize the mutation of the add operation.
2. Complexity in Test Case Design
The Challenge
Creating effective test cases that account for every possible mutation can be intricate and time-consuming. For instance, consider edge cases that might not be initially obvious.
The Solution
Utilize Test-Driven Development (TDD) to help design your test cases. Starting with a failing test case lets you understand the minimum requirements your tests must meet.
import static org.junit.jupiter.api.Assertions.assertEquals;
public class CalculatorTest {
@org.junit.jupiter.api.Test
public void testAddition() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3)); // Helps identify errors resulting from mutations
}
}
In this example, a simple addition test will help in identifying whether the mutant successfully fails the test if we change the operation from +
to -
.
3. High Number of Mutants
The Challenge
As mentioned earlier, mutation testing can generate a high number of mutants, leading to confusion about the relevance of results. Tracking and organizing a large number of mutants can be daunting.
The Solution
Use integration with coverage tools. Tools like JaCoCo can help track which parts of your code are exercised and give you insights into where to concentrate your testing efforts. This often makes it easier to ignore trivially failed mutants that don't add value.
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
public class CoverageExample {
@Test
public void shouldReturnPositiveWhenInputIsPositive() {
assertTrue(Calculator.isPositive(5)); // Only test relevant scenarios
}
}
Here, the test focuses only on relevant scenarios. Using coverage tools will help track which mutants relate to different coverage levels.
4. Integration with Existing Test Frameworks
The Challenge
Integrating mutation testing with existing test frameworks could require significant adjustments. The overhead can deter developers from implementing mutation testing.
The Solution
Choose mutation testing tools that are compatible with popular frameworks like JUnit and TestNG. PIT, for example, seamlessly integrates with these frameworks, allowing you to run mutation tests with minimal configuration.
Example configuration in Maven:
<plugin>
<groupId>org.pitest</groupId>
<artifactId>pitest-maven</artifactId>
<version>1.6.8</version>
<configuration>
<targetClasses>com.myapp.*</targetClasses>
<mutators>
<mutator>DEFAULTS</mutator> <!-- Leverage default mutators -->
</mutators>
</configuration>
</plugin>
This configuration directly runs mutation tests alongside your standard Maven builds, reducing integration friction.
5. Value of the Mutation Score
The Challenge
Determining the value of a mutation score can be ambiguous. High mutation scores don't guarantee high quality, and conversely, low scores can exist in quality codebases.
The Solution
Treat mutation scores as one of many metrics when assessing test effectiveness. Combine mutation testing insights with traditional testing metrics, code reviews, and static code analysis to get a more holistic view.
public class ExampleClass {
public int divide(int a, int b) {
if(b == 0) {
throw new IllegalArgumentException("Cannot divide by zero");
}
return a / b; // Pay attention to tests that cover exceptions
}
}
@Test
public void testDivideByZero() {
Exception exception = assertThrows(IllegalArgumentException.class, () -> {
exampleClass.divide(1, 0);
});
assertEquals("Cannot divide by zero", exception.getMessage());
}
In this snippet, we ensure exception handling is covered. Your mutation score can reveal gaps, but the goal should always be well-rounded test coverage, including exception handling.
Final Considerations
Mutation testing is a valuable practice that can significantly improve your software testing infrastructure. While it comes with its set of challenges, understanding and actively implementing solutions can help you reap the rewards of robust test suites.
Additional Resources
- If you're interested in further enhancing your understanding of mutation testing, check out Mutation Testing: A Tutorial and a Framework.
- For in-depth exploration of tools related to mutation testing in Java, you can visit PIT Mutation Testing.
Implement these strategies, evolve your testing practices, and ensure your software remains resilient in a changing landscape! Happy coding!
Checkout our other articles