Mastering JUnit Parameters: Common Pitfalls and Solutions
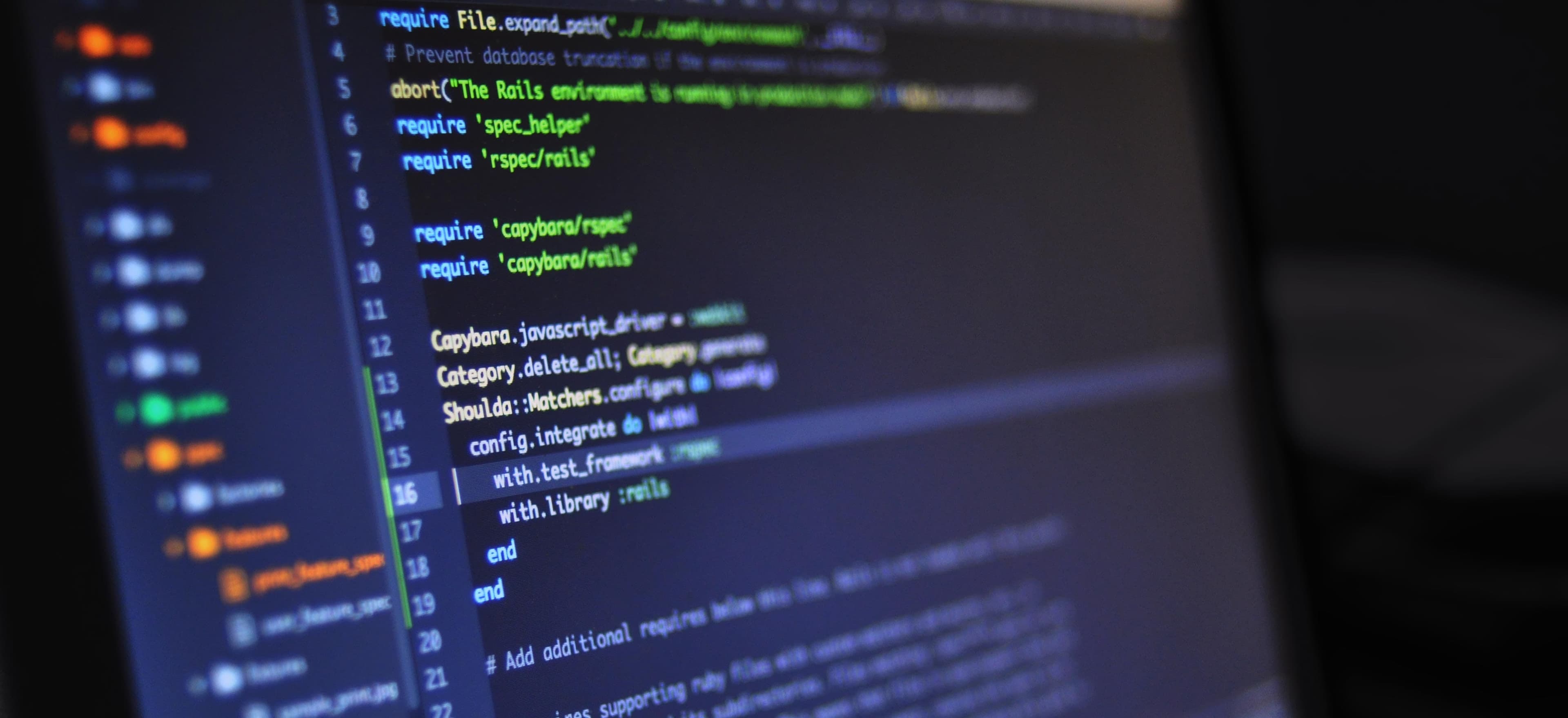
- Published on
Mastering JUnit Parameters: Common Pitfalls and Solutions
JUnit is widely recognized as the go-to testing framework for Java applications. With the increasing complexity of software systems, the need for effective testing becomes paramount. One of the powerful features that JUnit provides is parameterized tests. These allow developers to run the same test with multiple sets of data, making it a valuable asset to ensure robust test coverage.
However, working with parameterized tests can sometimes lead to confusion. In this blog post, we'll explore some common pitfalls associated with JUnit parameterized tests and provide practical solutions to overcome them.
Understanding Parameterized Tests
A parameterized test is a type of test that can be executed multiple times with different parameters. In JUnit, this is achieved using the @RunWith(Parameterized.class)
annotation along with a class that defines the test data.
Example of a Basic Parameterized Test
Before we delve into common pitfalls, let's look at a simple example. Below is a parameterized test that checks if a number is even.
import static org.junit.Assert.assertTrue;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.junit.runners.Parameterized;
import java.util.Arrays;
import java.util.Collection;
@RunWith(Parameterized.class)
public class EvenNumberTest {
private Integer number;
private Boolean expectedResult;
public EvenNumberTest(Integer number, Boolean expectedResult) {
this.number = number;
this.expectedResult = expectedResult;
}
@Parameterized.Parameters
public static Collection<Object[]> data() {
return Arrays.asList(new Object[][] {
{ 2, true },
{ 3, false },
{ 4, true },
{ 5, false }
});
}
@Test
public void testIsEven() {
assertTrue(isEven(number) == expectedResult);
}
private boolean isEven(Integer number) {
return number % 2 == 0;
}
}
Explanation of the Code
In this sample code, we define a EvenNumberTest
class that tests whether numbers are even. The constructor takes the number and the expected result. The data
method provides the sets of inputs. Each set is executed as a separate test case. This is an effective way to minimize redundancy in tests.
Common Pitfalls and Solutions
While parameterized tests can be beneficial, developers frequently encounter specific pitfalls. Let's identify some of these and suggest suitable solutions.
1. Failure to Run with a Parameterized Runner
When using parameterized tests, remember to include the @RunWith(Parameterized.class)
annotation on your test class header. Failing to do so will cause your tests to run as normal JUnit tests, causing unexpected results or failures.
Solution
Always ensure that the class is correctly annotated.
@RunWith(Parameterized.class) // Make sure to add this annotation
public class YourTestClass {
// Your test methods here
}
2. Passing Invalid Parameter Types
JUnit requires that the parameter types match the expected types in the test. Mismatched types can throw errors during the test execution.
Solution
Double-check the expected types in the data()
method and the constructor parameters.
@Parameterized.Parameters
public static Collection<Object[]> data() {
return Arrays.asList(new Object[][] {
{ "invalid", true } // This will fail type-checking
});
}
3. Not Understanding the Implications of State Sharing
Each test case runs in the same instance of the test class, which means shared state can lead to unexpected behaviors.
Solution
Minimize reliance on instance variables. Use method-local variables or provide data through constructor parameters.
@Parameterized.Parameters
public static Collection<Object[]> data() {
return Arrays.asList(new Object[][] {
{ 2 }, { 4 }, { 5 }
});
}
4. Overloading the Parameterized Test with Too Many Parameters
While it’s tempting to test multiple attributes in one parameterized test, it can lead to increased complexity and debugging difficulty.
Solution
Whenever possible, create separate parameterized tests for different functionality.
@RunWith(Parameterized.class)
public class MultipleAttributesTest {
// Break these down into separate tests
}
5. Not Using Descriptive Test Names
If you rely solely on the numeric index generated by JUnit in the test report, the results will be less clear. This can obfuscate which parameter set failed.
Solution
Override the toString()
method to provide a more descriptive name for your test cases:
@Override
public String toString() {
return "Number: " + number + ", Expected: " + expectedResult;
}
Advanced Parameterization Techniques
Using Method Sources for Parameterization
JUnit 5 enhanced parameterized tests by introducing @MethodSource
, which allows developers to use methods to supply arguments.
import org.junit.jupiter.params.ParameterizedTest;
import org.junit.jupiter.params.provider.MethodSource;
import java.util.stream.Stream;
public class EvenNumberTest {
@ParameterizedTest
@MethodSource("numberProvider")
void testIsEven(Integer number, Boolean expectedResult) {
assertTrue(isEven(number) == expectedResult);
}
static Stream<Arguments> numberProvider() {
return Stream.of(
Arguments.of(2, true),
Arguments.of(3, false),
Arguments.of(4, true),
Arguments.of(5, false)
);
}
private boolean isEven(Integer number) {
return number % 2 == 0;
}
}
With @MethodSource
, you can generate parameters directly from streams, making your tests cleaner and more flexible.
Bringing It All Together
Parameterized tests provide an effective means to validate the behavior of your code across a spectrum of inputs. While they offer an array of benefits, like reducing code duplication, improper use can lead to significant challenges.
By being aware of common pitfalls and adhering to best practices, you can harness the full potential of JUnit parameterized tests for your projects. For further reading on JUnit, check out JUnit 5 Documentation and Effective Java Testing.
Now go forth and master your testing suite with the improved understanding of parameterized tests! Happy coding!
Checkout our other articles