Common Misconceptions About Java Garbage Collection
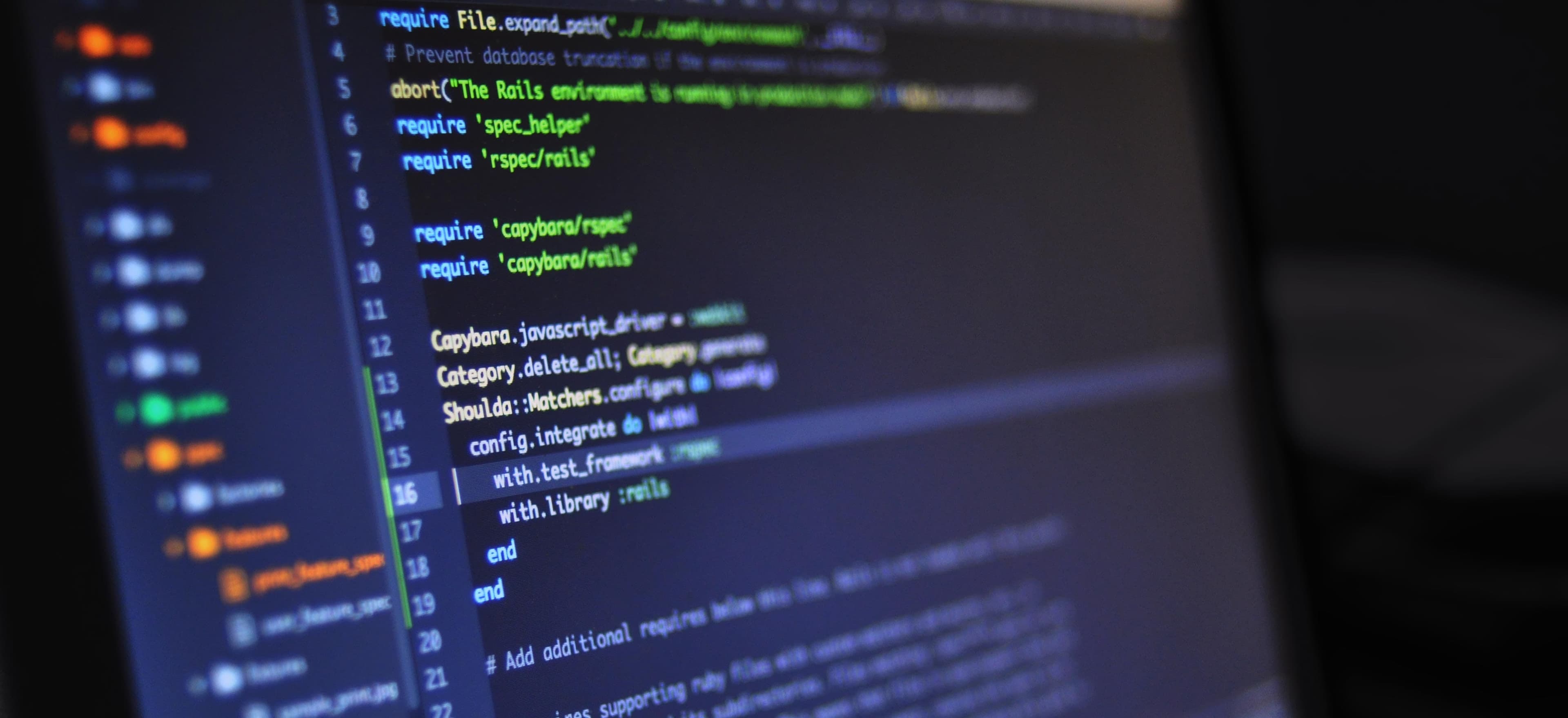
- Published on
Common Misconceptions About Java Garbage Collection
Java developers often encounter garbage collection (GC) during their programming journey. While garbage collection is a powerful feature, it also comes with a lot of misunderstanding. In this blog post, we will explore common misconceptions, clarify how Java's garbage collection works, and allow you to write better code by leveraging this automated memory management system.
What is Garbage Collection?
Garbage collection is the process of automatically recycling memory by reclaiming space occupied by objects that are no longer needed by the application. In Java, this process is handled by the Java Virtual Machine (JVM). It allows developers to focus on application logic instead of memory management.
Key Points to Understand:
- Automatic Memory Management: Developers do not need to manually allocate and deallocate memory.
- Non-Deterministic: The timing of garbage collection is unpredictable.
- Improves Performance: It can help maintain a stable environment by automatically managing memory.
Misconception 1: Garbage Collection Means No Memory Leaks
One of the most persistent myths is that garbage collection in Java eliminates memory leaks entirely. While garbage collection helps reclaim memory, it doesn't eradicate the possibility of memory leaks.
Why This is a Misconception:
Memory leaks occur when objects are no longer needed but are still referenced, preventing the garbage collector from reclaiming that memory.
import java.util.HashSet;
import java.util.Set;
public class MemoryLeakExample {
private Set<String> mySet = new HashSet<>();
public void addString(String str) {
mySet.add(str);
}
public void clearSet() {
// Intended to remove references, but objects remain in memory
// for the lifetime of this instance if this method is ignored.
// mySet.clear(); // Uncommenting here would fix the leak.
}
}
In the above example, if clearSet()
is ignored after adding strings to mySet
, it can lead to a memory leak. The HashSet
retains references to the strings, which the garbage collector cannot clean up even if the strings are no longer needed.
Prevention Tips:
- Regularly examine your application's memory and performance.
- Use tools like VisualVM for monitoring.
- Avoid holding onto references longer than necessary.
Misconception 2: Garbage Collection Guarantees Memory Release
It is common for developers to assume that after an object becomes unreachable, the memory will be released immediately. However, this is not true.
Why This is a Misconception:
Garbage collection in Java operates under indeterministic timing. Although an object may be unreachable, there is no guarantee when memory will be reclaimed.
public class GCExample {
private Object obj;
public void createObject() {
obj = new Object(); // Object reference is created
// obj becomes unreachable after this scope
}
public void someMethod() {
createObject();
// obj may remain in memory for an unpredictable amount of time.
}
}
In the example, the object referenced by obj
becomes eligible for garbage collection when createObject()
exits. However, the memory used by that object won't be freed immediately.
Best Practices:
- Use
System.gc()
judiciously; this suggests that the JVM run garbage collection but does not guarantee it. - Understand that JVM will run GC based on its own algorithms and resource availability.
Misconception 3: Garbage Collection Only Happens on the Heap
Many believe garbage collection is limited strictly to the heap memory. In reality, this isn't entirely accurate.
Why This is a Misconception:
Garbage collection primarily addresses objects created on the heap. However, it does interact with other areas such as the stack.
- Heap: For dynamically allocated objects.
- Stack: For simple values and method call frames. When stack memory is freed, it doesn't invoke GC.
public class StackExample {
public void stackMethod() {
int localVariable = 10; // Local variables' memory is managed via the stack.
// When this method completes, memory for localVariable is released automatically.
}
}
In the code above, localVariable
exists in stack memory and is automatically reclaimed without GC.
Conclusion:
- Understand the different memory areas and how they interact.
- Focus on managing your heap memory effectively.
Misconception 4: Garbage Collection Is Always Slow
A common fear among developers is that garbage collection will slow down their applications significantly. This concern often stems from misconceptions about the GC process.
Why This is a Misconception:
While it is true that certain types of garbage collectors can introduce pauses, modern JVMs have made significant strides in performance.
- Generational Garbage Collection: Most JVMs divide the heap into generations—young, old, and permanent. This categorization allows for optimized memory management.
- Concurrent and Incremental Collection: Techniques like concurrent GC allow applications to continue running while garbage collection takes place.
public class GCPerformanceExample {
public void createManyObjects() {
for (int i = 0; i < 1000000; i++) {
new Object(); // Creating many objects quickly, initially filling the young generation.
}
}
}
In this scenario, even though many objects are created rapidly, the use of generational garbage collection will optimize the reclaiming of memory from short-lived objects without a significant impact on performance.
Performance Improvement Strategies:
- Choose the correct garbage collector based on your application's needs (e.g., G1, ZGC).
- Use JVM flags to fine-tune GC behavior, such as setting the size of the young generation.
Misconception 5: You Should Avoid Objects All Together to Prevent Garbage Collection
Some developers might get the impression that they can achieve better performance by deliberately avoiding object creation to minimize garbage collection. This belief can lead to poor design practices.
Why This is a Misconception:
Object-oriented programming principles make it beneficial to encapsulate functionality in classes.
- Avoiding object creation compromises code clarity.
- Modern JVMs are optimized for garbage collection, making it a non-issue in many scenarios.
public class Employee {
private String name;
private int id;
public Employee(String name, int id) {
this.name = name;
this.id = id;
}
// Other methods...
}
Creating objects like in the example above is perfectly fine. The JVM is designed to manage the lifecycle of these objects effectively.
Balanced Approach:
- Use proper data structures based on your needs rather than avoiding object creation out of fear of garbage collection.
- Refer to the Java Performance Guide for efficient programming practices.
The Bottom Line
Garbage collection in Java is both powerful and sophisticated, providing valuable automated memory management. However, misconceptions can lead developers astray, resulting in inefficient coding practices and performance issues.
By understanding the real capabilities, limitations, and appropriate usage of garbage collection, you can harness its full potential while optimizing your Java applications. Always keep learning, and don't hesitate to explore further into Java's garbage collection mechanisms and best practices.
For more information on garbage collection algorithms, I recommend checking out Oracle's Garbage Collection Tuning Guide.
Further Reading:
- Understanding Java Garbage Collection
- Java Performance Tuning
Happy coding, and may your Java applications run smoothly with efficient garbage collection!
Checkout our other articles