Understanding the Pitfalls of Java Numeric Type Comparison
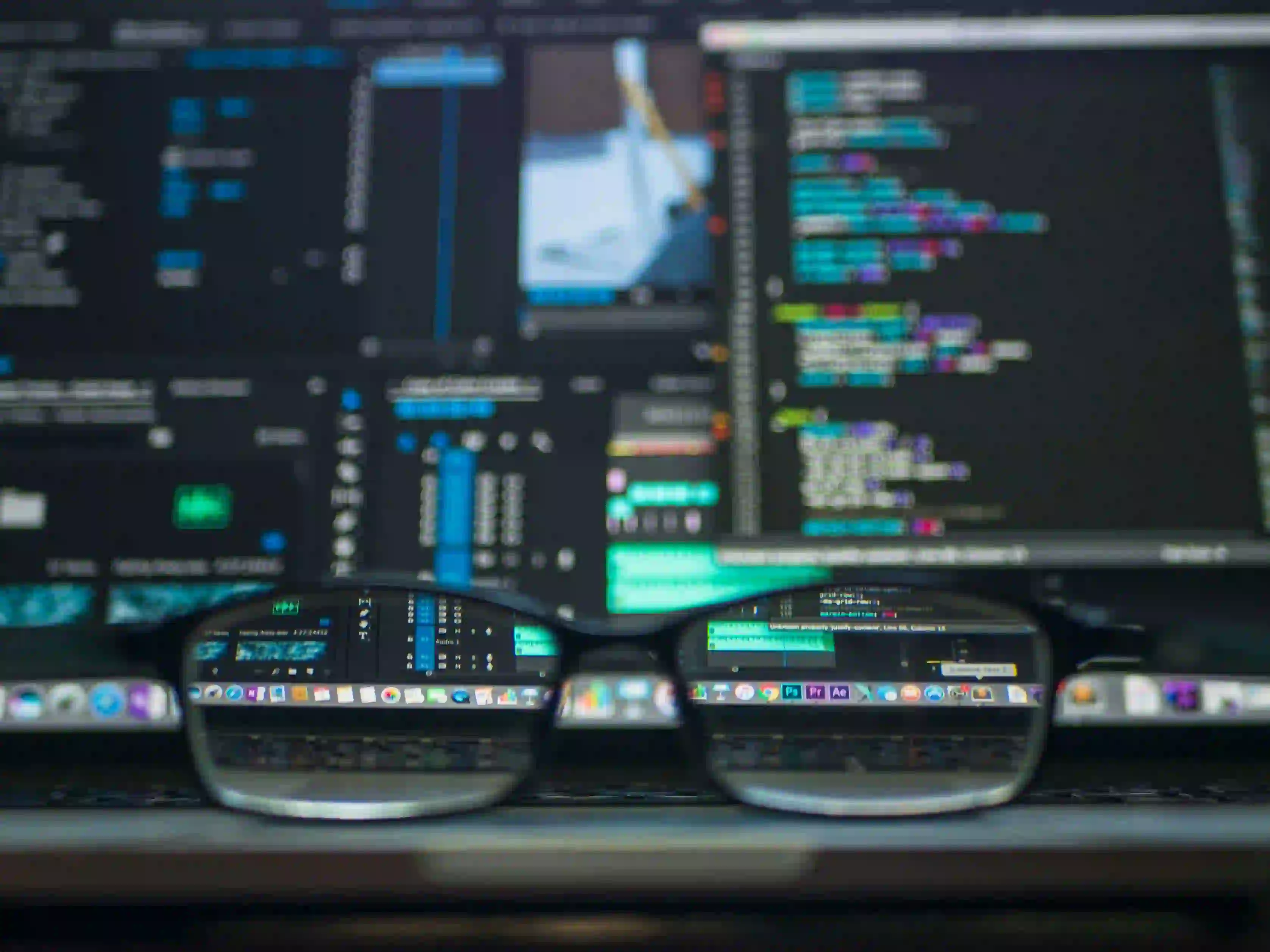
Understanding the Pitfalls of Java Numeric Type Comparison
Java is a strongly typed language known for its strict adherence to data types, which enhances reliability and reduces errors in application development. However, with this strength comes the challenge of comparing different numeric types. In this post, we will dive into the nuances of Java numeric type comparison, unveil potential pitfalls, and provide best practices to avoid common mistakes.
Numeric Types in Java
Java provides several numeric types that developers frequently use:
- byte: An 8-bit signed integer.
- short: A 16-bit signed integer.
- int: A 32-bit signed integer.
- long: A 64-bit signed integer.
- float: A single-precision 32-bit floating point.
- double: A double-precision 64-bit floating point.
These types vary not only in size but also in how they represent numbers, especially when it comes to comparisons.
Implicit and Explicit Type Conversion
Java handles type conversion through implicit and explicit conversions. Implicit conversions occur automatically when compatible types are assigned. Explicit conversions (or type casting), on the other hand, require the developer to specify the desired type.
Here's a simple example demonstrating both:
int intValue = 10;
// Implicit conversion from int to double
double doubleValue = intValue;
System.out.println("Double value: " + doubleValue); // Output: 10.0
In this example, intValue
is implicitly converted to a double
. However, care must be taken with explicit conversions as they can lead to data loss.
double largeDoubleValue = 9.87654321;
int intConversion = (int) largeDoubleValue;
System.out.println("Int value after casting: " + intConversion); // Output: 9
The code above demonstrates how casting a double
to an int
results in the truncation of decimals. Understanding these conversions is key in numeric type comparisons.
Common Pitfalls in Numeric Comparisons
There are several common pitfalls when comparing numeric types in Java. Let's explore these challenges to help you navigate them effectively.
Comparing Different Types
One common error occurs when comparing different numeric types either implicitly or explicitly. Java automatically promotes smaller types (e.g., byte
, short
, char
) to int
. This can lead to unexpected results if you're not careful.
Consider the following code:
byte b1 = 10;
byte b2 = 20;
int result = b1 - b2;
System.out.println("Result: " + result); // Output: -10
Even though b1
and b2
are of type byte
, the expression b1 - b2
is promoted to int
, thus changing the expected type.
Floating-Point Precision Issues
Comparing floating-point numbers also has its pitfalls due to precision. The IEEE 754 standard, which Java follows for floating-point arithmetic, cannot precisely represent most decimal numbers, leading to rounding errors. This means that two numbers that visually seem equal may not be:
double a = 0.1 + 0.2;
double b = 0.3;
if (a == b) {
System.out.println("Equal"); // May not output as expected
} else {
System.out.println("Not equal"); // Likely output
}
Instead of direct comparison, consider using a tolerance:
double epsilon = 0.0001; // Define a tolerance level
if (Math.abs(a - b) < epsilon) {
System.out.println("Approximately equal");
} else {
System.out.println("Not approximately equal");
}
This method allows for more reliable comparisons of floating-point numbers.
Integer Overflow and Underflow
When performing arithmetic operations on integers, watch out for overflow and underflow. If the result exceeds the maximum or minimum value that a data type can hold, it wraps around to the opposite end of its range.
int maxValue = Integer.MAX_VALUE;
int overflowedValue = maxValue + 1; // This wraps around to the negative side
System.out.println("Overflow value: " + overflowedValue); // Output: -2147483648
To mitigate these concerns, use types that provide a wider range (e.g., long
) or leverage mathematical libraries that handle larger values.
Use of Wrapper Classes
Java provides wrapper classes for primitive types (e.g., Integer
, Double
) which introduce additional functionalities, like nullability and methods for comparing values. However, be careful after autoboxing (automatic conversion between primitives and their corresponding wrapper classes).
Integer x = 127;
Integer y = 127;
System.out.println(x == y); // Output: true (due to caching of small integers)
Integer a = 128;
Integer b = 128;
System.out.println(a == b); // Output: false (different objects!)
In this case, Integer
values between -128 and 127 are cached, which means x
and y
point to the same object. Once you exceed this range, a
and b
are treated as separate instances, leading to surprising results if you're not aware of this behavior. Always use .equals
for checking equality of wrapper objects:
System.out.println(a.equals(b)); // Output: true
Best Practices for Numeric Type Comparisons
To effectively navigate numeric comparisons in Java, follow these best practices:
-
Understand Type Promotion: Always be aware of how Java promotes types in expressions to avoid unintentional data loss or misinterpretation.
-
Use Tolerance for Floating-Point Comparisons: Implement a tolerance level for comparing floating-point numbers to mitigate precision errors.
-
Watch for Overflow/Underflow: Always check the range of values your operations might produce, especially when dealing with integers.
-
Prefer
equals
for Wrapper Class Comparisons: Use theequals
method for comparing instances of wrapper classes, rather than the==
operator. -
Leverage Libraries: Utilize third-party libraries, like Apache Commons Math, which provide robust numerical methods and handle potential pitfalls.
For more insights into Java numeric comparisons and best practices, check out the Oracle Documentation on Java Primitive Types and What is IEEE 754? for an in-depth understanding.
Closing Remarks
Understanding the pitfalls of numeric type comparisons in Java is vital for writing robust and error-free applications. By grasping the nuances of implicit and explicit conversions, accounting for precision errors, and adhering to best practices, developers can mitigate common issues and enhance code reliability.
Armed with this knowledge, you can approach numeric comparisons with confidence, paving the way for effective Java application development. Happy coding!