Troubleshooting Common Spring Hibernate Integration Issues
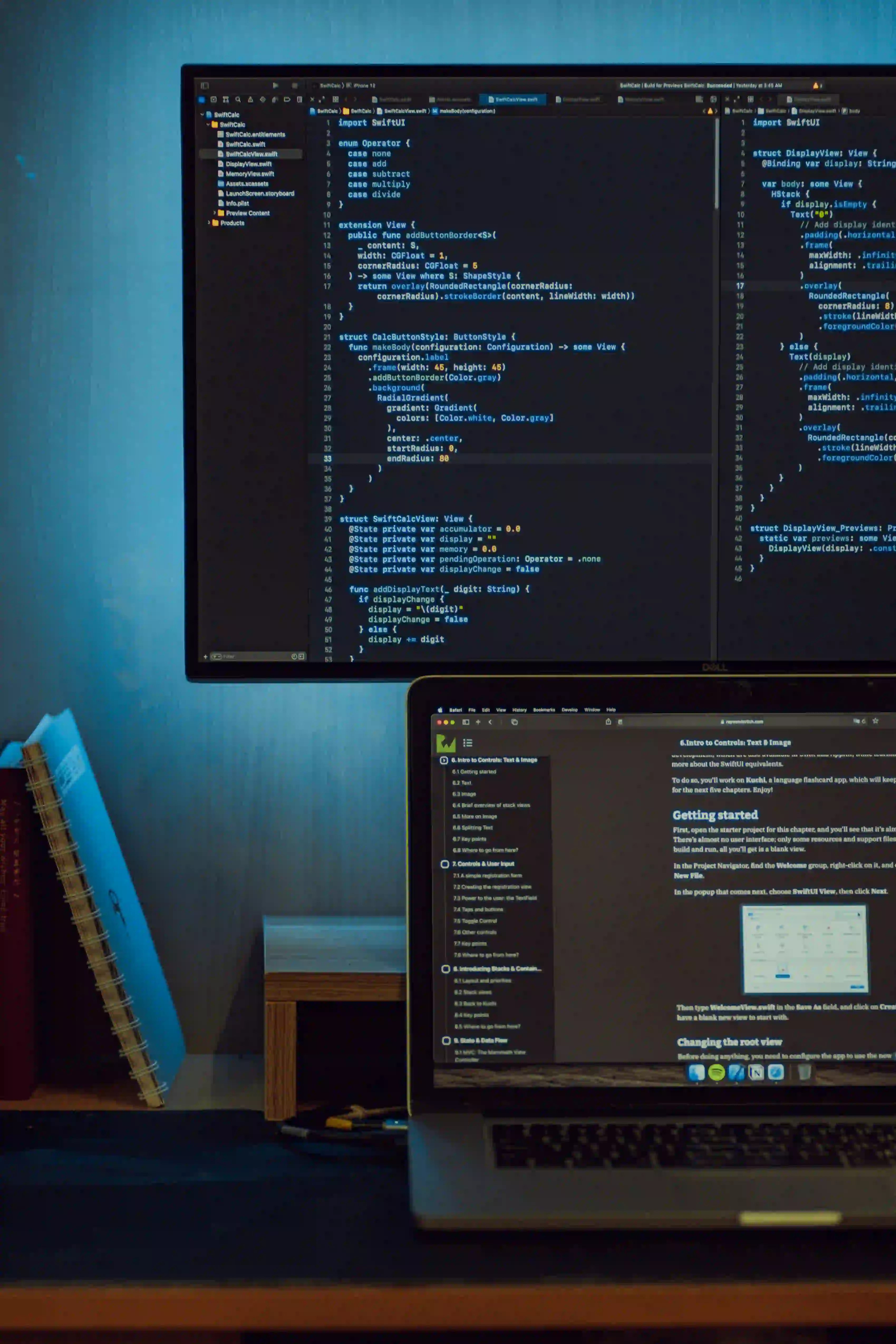
Troubleshooting Common Spring Hibernate Integration Issues
Integrating Spring with Hibernate can lead to a seamless and powerful application development experience. However, with great power comes great responsibility, and many developers face challenges during this integration. In this blog post, we will discuss common issues, their symptoms, and effective troubleshooting steps to resolve them. We'll also provide code snippets to clarify important concepts.
Understanding Spring and Hibernate
Spring Framework is a powerful framework focused on building enterprise-level applications. It offers various modules, including Spring Data, that simplify database operations.
Hibernate is a popular Object-Relational Mapping (ORM) tool that maps Java classes to database tables. It handles the complexities of database interactions, allowing developers to focus on business logic.
Common Spring Hibernate Integration Issues
1. Transaction Management Issues
Symptoms:
- Data not persisted in the database.
- Exceptions related to transactions.
Understanding the Issue
Spring manages transactions via the @Transactional
annotation. If it is not properly configured, transactions may not work as expected.
Troubleshooting Steps:
- Check the Transaction Manager:
Ensure that you have configured the appropriate transaction manager. For Hibernate, this is oftenHibernateTransactionManager
.
@Bean
public HibernateTransactionManager transactionManager(SessionFactory sessionFactory) {
HibernateTransactionManager transactionManager = new HibernateTransactionManager();
transactionManager.setSessionFactory(sessionFactory);
return transactionManager;
}
In the snippet above, we define a transaction manager for Hibernate. Always ensure a session factory is injected.
- Enable Transaction Management:
Make sure transaction management is enabled in your configuration.
@EnableTransactionManagement
public class AppConfig {
// Configuration beans
}
The @EnableTransactionManagement
annotation activates Spring's annotation-driven transaction management.
2. SessionFactory Bean Configuration
Symptoms:
- LazyInitializationException when accessing entities outside the session.
- Data fetch issues from the database.
Understanding the Issue
The SessionFactory
bean must be properly defined to manage Hibernate sessions. If it isn't set up correctly, operations like fetching or saving entities can fail.
Troubleshooting Steps:
- Check SessionFactory Creation:
@Bean
public SessionFactory sessionFactory(DataSource dataSource) {
LocalSessionFactoryBuilder factoryBuilder = new LocalSessionFactoryBuilder(dataSource);
factoryBuilder.scanPackages("com.example.model")
.addProperties(hibernateProperties());
return factoryBuilder.buildSessionFactory();
}
Ensure you provide correct package scanning and properties in your SessionFactory
setup.
- Review Hibernate Properties:
Make sure your properties are correctly set, especiallyshow_sql
andhbm2ddl.auto
, which can provide useful logs during development.
hibernate.show_sql=true
hibernate.dialect=org.hibernate.dialect.MySQLDialect
hibernate.hbm2ddl.auto=update
3. Entity Configuration Issues
Symptoms:
- Data retrieval returns null.
- Invalid mapping errors during runtime.
Understanding the Issue
Each entity must be marked with appropriate annotations such as @Entity
, and the primary key must be defined with @Id
.
Troubleshooting Steps:
- Check Entity Annotations:
@Entity
@Table(name = "users")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(name = "name")
private String name;
// getters and setters
}
In this example, ensure all fields are correctly annotated.
- Validate Relationships:
If your entity has relationships, ensure cascading and fetching strategies are properly defined.
@OneToMany(mappedBy = "user", cascade = CascadeType.ALL, fetch = FetchType.LAZY)
private List<Post> posts;
Cascade types control the lifecycle of related entities. Incorrect settings can lead to unintentional data loss.
4. Incorrectly Configuring DataSource
Symptoms:
- Unable to establish a connection to the database.
- SQL exceptions thrown during runtime.
Understanding the Issue
The DataSource is a critical component of your Spring and Hibernate setup. Errors here can prevent your application from communicating with the database.
Troubleshooting Steps:
- Check Configuration Properties:
spring.datasource.url=jdbc:mysql://localhost:3306/exampledb
spring.datasource.username=root
spring.datasource.password=password
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
Ensure the JDBC URL, username, and password are correctly set.
- Driver Dependency:
Ensure the database driver is included in your Maven or Gradle dependencies.
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.29</version>
</dependency>
5. Performance Issues
Symptoms:
- Slow application response times.
- Delayed query execution.
Understanding the Issue
Inefficient queries or poor mappings can lead to performance degradation.
Troubleshooting Steps:
- Use Fetch Joins Instead of Separate Queries:
If you are often accessing related entities, using fetch joins can improve performance:
@Query("SELECT u FROM User u JOIN FETCH u.posts")
List<User> findAllUsersWithPosts();
Be mindful of the potential N+1 query problem if lazy fetching is misused.
- Optimize Queries:
Use query hints or indexes on the database to enhance performance. Additionally, make use of the Hibernate second-level cache.
Final Considerations
Troubleshooting Spring and Hibernate integration issues can be daunting, but with the right approach, you can effectively resolve the problems encountered. Keep your configurations well-documented and regularly test your application during development to catch issues early.
By understanding the root causes of these common issues and employing the troubleshooting steps outlined in this post, you can ensure a seamless integration between Spring and Hibernate, leading to a robust and efficient application.
For more advanced discussions, consider referring to the following resources:
- Spring Data JPA Documentation
- Hibernate Documentation
Feel free to explore and post your queries or experiences in the comments below. Happy coding!