Common Pitfalls When Migrating Spring Microservices to AWS
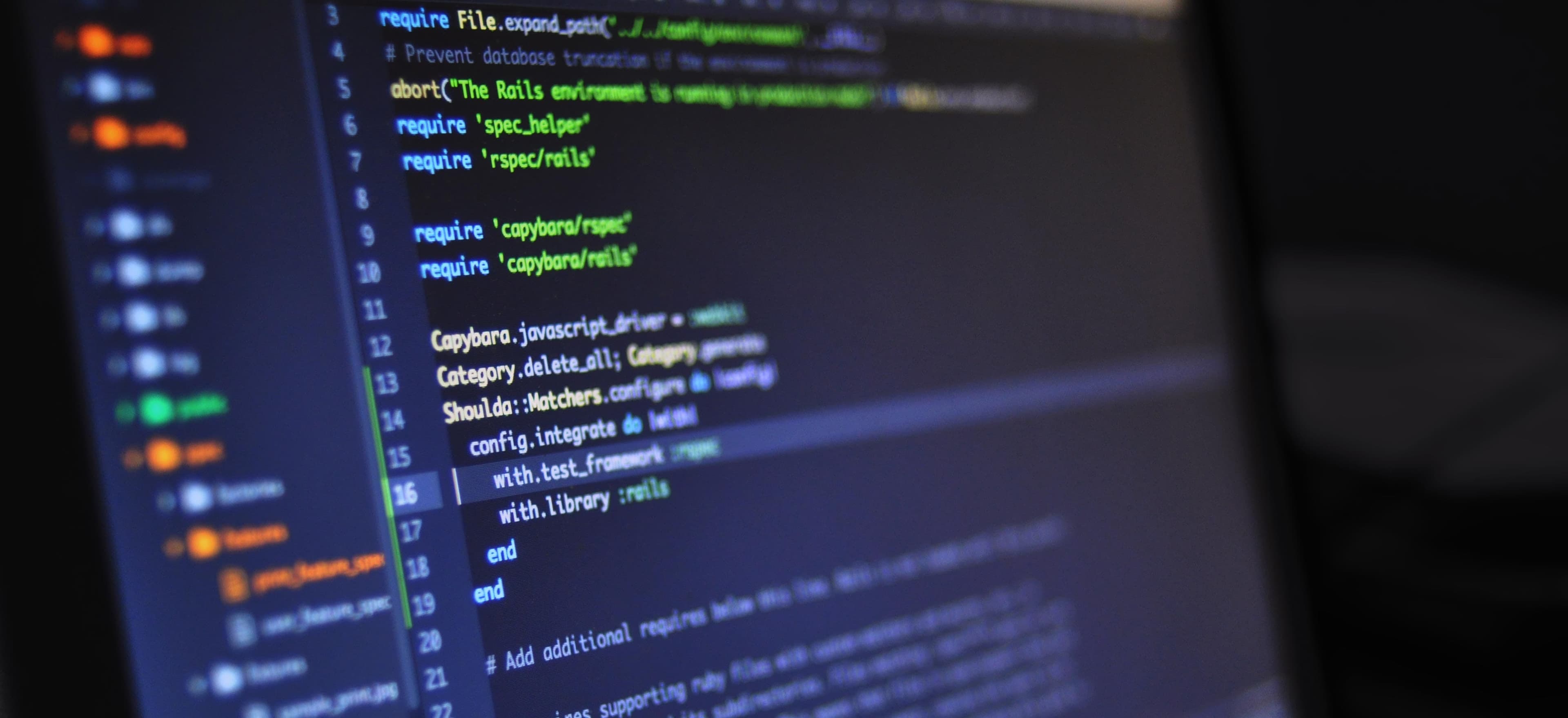
- Published on
Common Pitfalls When Migrating Spring Microservices to AWS
Migrating an application—especially a complex one like a Spring microservice—can be a daunting task. This blog post will discuss common pitfalls developers encounter when migrating Spring microservices to AWS (Amazon Web Services). We will explore the nuances of such a migration, share code snippets, and provide recommendations for a smoother transition.
Understanding Microservices Architecture
Microservices architecture allows developers to build applications as a collection of loosely coupled services. Each service is independently deployable, scalable, and can communicate via lightweight APIs, often in the form of REST.
Why Spring Framework?
Spring is a powerful framework for building Java applications, particularly microservices. It offers:
- Dependency injection and aspect-oriented programming support.
- Integration with various data access technologies and message brokers.
- An extensive ecosystem with Spring Boot and Spring Cloud, especially for building microservices.
Key Reasons for Migration to AWS
- Scalability: AWS provides auto-scaling capabilities.
- Cost-effectiveness: Pay only for the resources used.
- Global Reach: AWS infrastructure spans across multiple regions.
Nevertheless, several pitfalls can hinder a smooth migration. Let’s explore these common challenges.
Common Pitfalls in Migration to AWS
1. Ignoring Dependency Management
When migrating microservices, managing dependencies is crucial. Different services may rely on specific versions of libraries or tools. Without careful planning, you might face compatibility issues post-migration.
Code Snippet
Managing dependencies in Spring Boot requires listing them in the pom.xml
(for Maven) or build.gradle
(for Gradle). Here's a basic example:
<!-- pom.xml -->
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
</dependencies>
Why: This configuration pulls in the necessary dependencies for creating a web application with JPA support, which is essential for most microservices that need to interact with a database.
2. Lack of Infrastructure as Code (IaC)
Failing to implement Infrastructure as Code can lead to inconsistencies in deployment environments. Utilizing IaC ensures that environments remain reproducible and manageable.
Example using AWS CloudFormation
Resources:
MyMicroservice:
Type: AWS::ElasticBeanstalk::Application
Properties:
ApplicationName: MyMicroserviceApp
Why: This YAML snippet defines an AWS Elastic Beanstalk application, allowing for automated, repeatable deployments. For more on IaC, AWS CloudFormation is an excellent starting point.
3. Not Considering Cloud-Native Services
AWS offers a plethora of cloud-native services designed to optimize your application, but neglecting to leverage these can lead to sub-optimal performance.
Using AWS RDS for Database Management
Instead of managing your own database instance, consider utilizing AWS RDS:
@Bean
public DataSource dataSource() {
return DataSourceBuilder.create()
.driverClassName("com.mysql.cj.jdbc.Driver")
.url("jdbc:mysql://your-instance.c7d9h4e8klmn.us-west-2.rds.amazonaws.com:3306/yourdb")
.username("youruser")
.password("yourpassword")
.build();
}
Why: This bean configuration allows Spring to connect seamlessly to the RDS instance, enabling you to offload management tasks like backups, scaling, and patching to AWS.
4. Not Using a CI/CD Pipeline
Continuous integration and continuous deployment (CI/CD) are critical for any modern development process. Not establishing a CI/CD pipeline will slow down your deployment process and may introduce bugs.
Example of a Basic CI/CD Pipeline using AWS CodePipeline
version: '1.0'
resources:
- name: CodePipeline
type: AWS::CodePipeline::Pipeline
properties:
RoleArn: !GetAtt CodePipelineRole.Arn
Artifacts:
- Name: SourceOutput
Stages:
- Name: Source
Actions:
- Name: SourceAction
ActionTypeId:
Category: Source
Owner: ThirdParty
Provider: GitHub
Version: '1'
OutputArtifacts:
- Name: SourceOutput
Why: This CI/CD configuration integrates GitHub as your source repository, which is essential for automating your pipeline from code commit to deployment.
5. Poor Monitoring and Logging
Post-deployment, not setting up robust monitoring and logging can lead to significant operational blind spots. AWS CloudWatch can help here.
Setting Up Log Streams
aws logs create-log-group --log-group-name MyMicroserviceLogs
aws logs create-log-stream --log-group-name MyMicroserviceLogs --log-stream-name $(date +"%Y-%m-%d")
Why: This command creates a log group and a log stream in CloudWatch, making it easier to track application behavior and performance.
6. Not Leveraging AWS Security Measures
Security should be a priority; ignoring AWS's robust security features like IAM roles can expose your application to vulnerabilities. Understand how to manage permissions effectively.
Assigning Role to EC2 Instance
{
"Version": "2012-10-17",
"Statement": [
{
"Effect": "Allow",
"Action": "s3:GetObject",
"Resource": "arn:aws:s3:::your-bucket-name/*"
}
]
}
Why: This IAM policy gives your EC2 instance permission to access specific S3 resources, thus following the principle of least privilege.
7. Failing to Optimize Costs
AWS operates on a pay-as-you-go model that can also lead to surprises if you're not monitoring usage closely.
Using the AWS Cost Explorer
Utilize the AWS Cost Explorer to monitor and analyze your spending. Implement budgets to ensure you do not exceed allocated resources.
Closing Remarks
Migrating Spring microservices to AWS can provide significant benefits but also introduces various challenges.
To summarize:
- Manage Dependencies: Keep careful track of all service dependencies.
- Adopt Infrastructure as Code: Ensure environments are consistent.
- Leverage Cloud-Native Services: Optimize performance using AWS offerings.
- Implement CI/CD: Automate your deployment process.
- Set Up Monitoring and Logging: Maintain operational awareness.
- Use AWS Security Measures: Protect your application with IAM roles.
- Monitor Costs: Use AWS tools to keep expenses in check.
By being aware of these pitfalls, you can significantly increase your chances of a successful migration and unleashing the full potential of your Spring microservices in AWS.
For more details on specific AWS services, check the AWS documentation. Happy coding!
Checkout our other articles