Mastering Spring Data: Common Pitfalls to Avoid
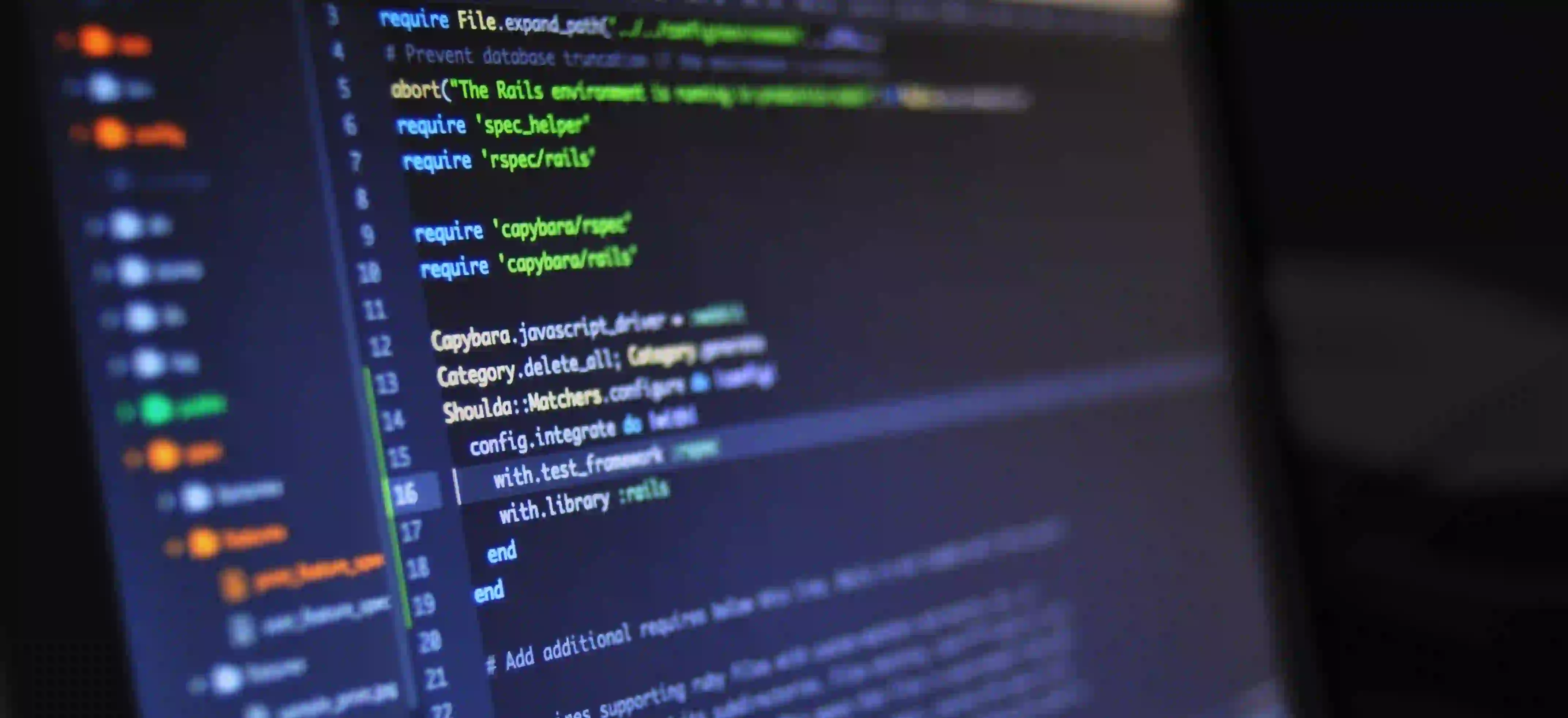
Mastering Spring Data: Common Pitfalls to Avoid
Spring Data is a powerful framework that simplifies database access and manipulation in Java applications. With its numerous features, developers can easily interact with various data stores. However, like any technology, it comes with its own set of challenges. In this blog post, we will discuss common pitfalls to avoid when using Spring Data, ensuring you can leverage its full potential in your projects.
1. Ignoring the Repository Pattern
One of the core principles of Spring Data is the repository pattern. The repository pattern helps to abstract and encapsulate the data access logic.
Why It Matters
Ignoring the repository pattern can lead to tightly coupled code, making it challenging to manage and test. By using repositories, you gain the following advantages:
- Separation of Concerns: Business logic is separated from data access logic.
- Testability: Easier to mock dependencies during unit tests.
Example Code Snippet
Here is a simple example of defining a repository for a User
entity:
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.stereotype.Repository;
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
User findByUsername(String username);
}
Commentary
In this example, the UserRepository
extends JpaRepository
, inheriting essential CRUD operations. This separation allows your service layer to focus on business logic, while the repository layer handles database interactions.
2. Overly Complex Queries
Spring Data provides a neat way to define queries using method names. However, complex queries can lead to maintenance nightmares.
Why It Matters
Overly complex queries can:
- Reduce readability and make your code harder to understand.
- Create performance bottlenecks.
Example Code Snippet
Instead of creating a complex method name, consider using a custom query:
@Query("SELECT u FROM User u WHERE u.age > 18 AND u.city = ?1")
List<User> findAdultsInCity(String city);
Commentary
Using the @Query
annotation allows you to define a clear and concise query. This method enhances readability and keeps the method names clean, making it easier to understand the underlying logic at a glance.
3. Neglecting Caching Mechanisms
Caching is essential for application performance. Spring Data can integrate with caching technologies but is often overlooked.
Why It Matters
Neglecting caching can lead to:
- Increased database load.
- Slower responses for frequently accessed data.
Example Code Snippet
Here is how you can enable caching with Spring Data:
import org.springframework.cache.annotation.Cacheable;
import org.springframework.stereotype.Service;
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
@Cacheable("users")
public User findUserById(Long id) {
return userRepository.findById(id).orElse(null);
}
}
Commentary
In this example, the findUserById
method is annotated with @Cacheable
. When called, the method's result will be cached, which reduces load on the database for subsequent calls with the same ID.
4. Poor Exception Handling
Spring Data implementations can throw various exceptions. Handling these exceptions poorly can result in unwieldy applications.
Why It Matters
Neglecting proper exception handling can lead to:
- Application crashes.
- Uninformative error messages for users.
Example Code Snippet
Here’s an approach to handle data access exceptions:
import org.springframework.dao.DataAccessException;
import org.springframework.stereotype.Service;
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
public User getUserById(Long id) {
try {
return userRepository.findById(id).orElseThrow(() -> new UserNotFoundException("User not found"));
} catch (DataAccessException e) {
// Log the exception
throw new DatabaseAccessException("Failed to access the database", e);
}
}
}
Commentary
In this code, DataAccessException
is caught, allowing you to log the error or handle it gracefully while providing a more meaningful response to the client.
5. Misusing Lazy Loading
Lazy loading can boost performance but can also lead to the infamous LazyInitializationException
.
Why It Matters
Misusing lazy loading can cause:
- Unintended database calls.
- Performance degradation if not managed properly.
Example Code Snippet
Consider using FetchType.LAZY
wisely:
@Entity
public class User {
@OneToMany(fetch = FetchType.LAZY, mappedBy = "user")
private List<Post> posts;
}
Commentary
In this setup, posts are not loaded until explicitly accessed. Ensure that your service layer is aware of when to access these posts to avoid lazy initialization exceptions, typically when the session is closed.
6. Forgetting to Use Projection
Working with large entities can be resource-intensive, slowing down your application.
Why It Matters
Not employing projections can lead to:
- Loading unnecessary data into memory.
- Increased processing time on database queries.
Example Code Snippet
Here's how to create a projection:
public interface UserProjection {
String getUsername();
String getEmail();
}
public interface UserRepository extends JpaRepository<User, Long> {
List<UserProjection> findAllProjectedBy();
}
Commentary
By defining an interface for your projections, you ensure that only necessary fields are loaded, enhancing performance and reducing resource allocation.
Lessons Learned
Adopting Spring Data can significantly enhance application development, but it is essential to avoid the common pitfalls outlined above. By adhering to best practices such as using the repository pattern, managing exceptions, leveraging caching, and avoiding complex queries, you can set yourself up for success.
For further reading, consider exploring the official Spring Data documentation and community-driven tutorials on repositories and projections.
By mastering these concepts and avoiding common pitfalls, you can develop efficient, maintainable, and high-performance applications with Spring Data. Happy coding!