Common Pitfalls in Automation Testing: Avoid These Mistakes!
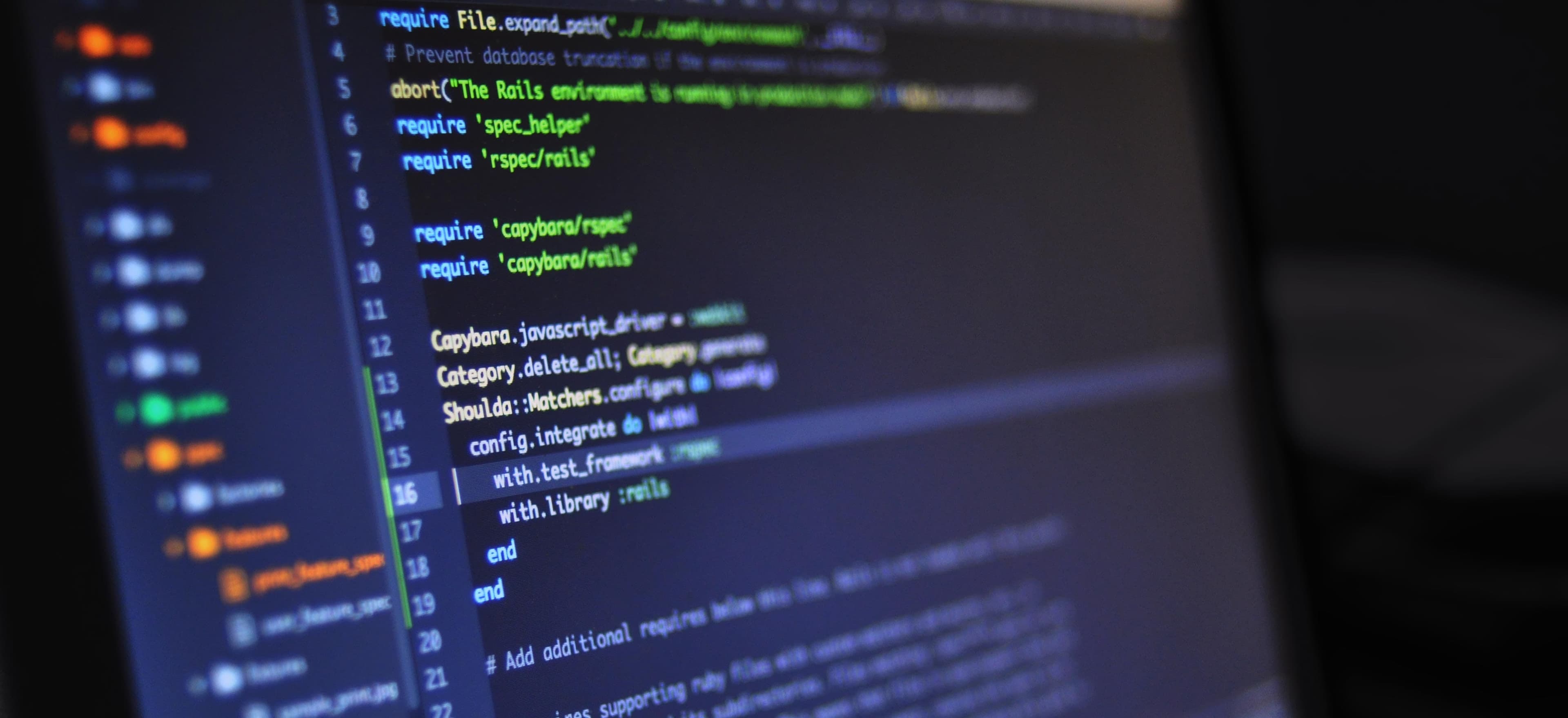
- Published on
Common Pitfalls in Automation Testing: Avoid These Mistakes!
Automation testing is an essential part of modern software development. It helps test applications for quality and performance with speed and accuracy. However, it is not without its challenges. In this post, we’ll explore some common pitfalls in automation testing and discuss ways to avoid them. Understanding these pitfalls can save you time, resources, and frustration in your testing efforts.
1. Lack of a Clear Strategy
One of the biggest mistakes teams make is diving into automation without a solid strategy. A lack of a clear plan can lead to chaos, inefficient tests, and unmet expectations.
Solution
Start with a well-defined strategy that outlines your goals. Determine which tests to automate based on factors like:
- Repetitiveness: Tests that are run frequently.
- Complexity: Tests that require significant manual effort.
- Critical paths: Scenarios essential for business functionality.
Example
If you're testing a login page, prioritize automating scenarios like logging in with valid and invalid credentials, as these are common and critical operations.
@Test
public void testValidLogin() {
driver.get("http://yourapp.com/login");
driver.findElement(By.id("username")).sendKeys("validUser");
driver.findElement(By.id("password")).sendKeys("validPass");
driver.findElement(By.id("loginButton")).click();
String expectedUrl = "http://yourapp.com/dashboard";
assertEquals(expectedUrl, driver.getCurrentUrl(), "Login did not redirect to dashboard.");
}
Why: This test case checks a common user flow and is fundamental to the application's functionality.
2. Failing to Keep Tests Maintainable
Automation tests can become brittle if they are not designed for maintainability. Changes in the user interface or application logic often lead to test failures.
Solution
Write modular and reusable test scripts. Implement the Page Object Model (POM) design pattern to create a separation of concerns between test logic and application structure.
Example
Instead of embedding locators directly within your test, create a page object class.
public class LoginPage {
private WebDriver driver;
public LoginPage(WebDriver driver) {
this.driver = driver;
}
public void enterUsername(String username) {
driver.findElement(By.id("username")).sendKeys(username);
}
public void enterPassword(String password) {
driver.findElement(By.id("password")).sendKeys(password);
}
public void clickLogin() {
driver.findElement(By.id("loginButton")).click();
}
}
Using the Page Object in Tests
@Test
public void testValidLogin() {
LoginPage loginPage = new LoginPage(driver);
loginPage.enterUsername("validUser");
loginPage.enterPassword("validPass");
loginPage.clickLogin();
assertEquals("http://yourapp.com/dashboard", driver.getCurrentUrl(), "Login did not redirect to dashboard.");
}
Why: This reduces the potential for disruption when the UI changes. Updates are localized to the page object, making your tests easier to maintain.
3. Ignoring Test Data Management
Not having an efficient strategy for managing test data can lead to inconsistent results and increased test maintenance overhead.
Solution
Implement a robust test data management strategy. Use tools or services that allow you to create, read, and update test data systematically.
Example
For tests that require a user account, consider using a factory pattern or a mock data service to generate user credentials instead of hardcoding them.
public class UserFactory {
public static User createUser(String username, String password) {
// Logic to create a user instance
return new User(username, password);
}
}
// In your test
User testUser = UserFactory.createUser("testUser", "testPass");
Why: This aids in maintaining clean data and allows for easy updates, saving time and reducing errors in the testing process.
4. Over-Automating Tests
Automation is powerful, but not everything should be automated. Attempting to automate every test can lead to wasted resources and complex management.
Solution
Prioritize tests that provide the most value to automate. Manual testing is still required for exploratory testing, usability, and new features.
When Not to Automate
- If a test runs infrequently.
- If the business value is minimal.
- If the tests require significant manual intervention.
Why: Effective test automation involves a balance. Focus on automating the tests that have a significant impact on overall application health and user experience.
5. Neglecting to Review and Maintain Tests
Automation tests are not set-it-and-forget-it. Failing to regularly review and maintain them will result in outdated tests that do not accurately reflect application behavior.
Solution
Establish a regular review process. Schedule periodic meetings to discuss and analyze test results, identify flaky tests, and update cases as necessary.
Feedback Loop
Create a feedback mechanism with developers to ensure tests are up-to-date and relevant to the current codebase.
Why: Continuous assessment enables you to identify potential issues early and keeps your test suite relevant and effective.
6. Not Leveraging the Right Tools
Using the wrong tools can severely hamper your automation process. Each tool comes with its strengths and weaknesses.
Solution
Evaluate the tools you use against your specific testing needs. Consider factors like:
- Ease of use
- Integration capabilities
- Support and community
Popular Tools
- Selenium: For web application testing.
- TestNG: For managing complex test suites.
- Jenkins: For continuous integration.
Example
A basic Selenium setup for Java can look like this:
WebDriver driver = new ChromeDriver();
driver.get("http://yourapp.com");
Why: Using the right tools not only improves productivity but also enhances the robustness of your testing strategy.
7. Insufficient Reporting and Metrics
Without proper reporting and metrics, it's difficult to gauge the effectiveness of your automation testing efforts.
Solution
Integrate reporting tools like Allure or ExtentReports. This allows you to visualize results and track progress over time.
Example
A simple setup with TestNG reporting:
<listeners>
<listener class-name="org.uncommons.reportng.HTMLReporter"/>
<listener class-name="org.uncommons.reportng.JUnitXMLReporter"/>
</listeners>
Why: Effective reporting helps teams make informed decisions, understand test coverage, and ultimately improve the quality of the software.
Closing Remarks
Automation testing brings tremendous benefits to software development, but pitfalls lurk around every corner. By avoiding these common mistakes, you can streamline your automation efforts, improve test quality, and deliver a better product.
Regular reviews, a solid strategy, and proper metrics will help you navigate the intricacies of automation testing. Invest in your testing framework, and you'll reap the rewards in efficiency and reliability.
For further reading, you may find these resources valuable:
- The Page Object Model
- TestNG Official Documentation
- Continuous Integration with Jenkins
Embrace the power of automation while being wary of its complexities, and your testing journey will be much smoother. Happy testing!
Checkout our other articles