Unlocking the Mystery of Java 8's Target Type Inference
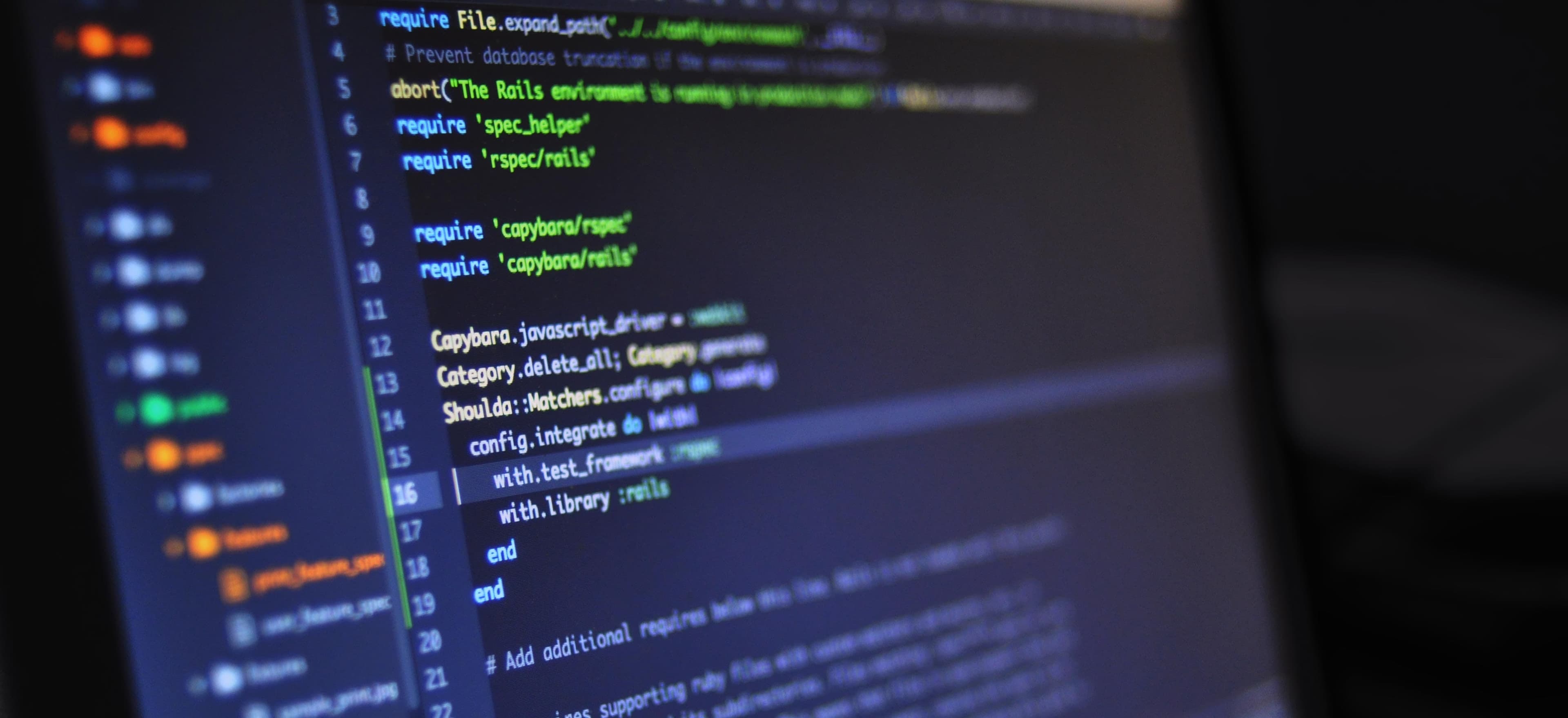
- Published on
Unlocking the Mystery of Java 8's Target Type Inference
Java has consistently evolved over the years, with each version introducing features that enhance developer productivity and improve overall code quality. One of the most notable enhancements in Java 8 was the introduction of target type inference, a concept that simplifies the use of generics in Java. In this blog post, we will dive into the intricacies of target type inference, explore its significance, and provide practical examples to help you harness its capabilities in your projects.
What is Target Type Inference?
Target type inference refers to the Java compiler's ability to deduce the type of a generic expression based on the context in which it is used. This becomes particularly powerful when dealing with lambda expressions and method references introduced in Java 8. The compiler analyzes the expected type of the expression from the surrounding context, thus reducing the need for explicit type declarations.
Why Use Target Type Inference?
The traditional Java approach required developers to specify types explicitly, often leading to verbose code. With target type inference, you can write cleaner, more concise code while still maintaining type safety. This makes your code easier to read and maintain, which is a significant advantage in both team environments and personal projects.
A Brief Example
Let’s start with a simple example of using target type inference with a functional interface.
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class TargetTypeInferenceExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
// Using method reference instead of a lambda expression
List<String> upperCaseNames = names.stream()
.map(String::toUpperCase) // target type inference in action
.collect(Collectors.toList());
System.out.println(upperCaseNames);
}
}
In the code above, we are using a method reference (String::toUpperCase
) instead of a traditional lambda expression (name -> name.toUpperCase()
). The compiler infers the expected type String
from the context of the map
method, where it knows it should return an uppercased String
.
Target Type Inference in Detail
Let's break down how target type inference works under the hood.
Situations Where Target Type Inference is Applicable
Target type inference is generally applicable in the following scenarios:
-
Lambdas: When passing a lambda expression as an argument to a method.
-
Method References: Using method references where the compiler can deduce the target type.
-
Generics: When dealing with generic collections or classes.
Example of Lambda Expressions
Consider this example that demonstrates the use of lambdas with type inference:
import java.util.function.Function;
public class LambdaInferenceExample {
public static void main(String[] args) {
// Target type inference through functional interface
Function<Integer, String> intToString = integer -> Integer.toString(integer);
String result = intToString.apply(42);
System.out.println(result); // Output: "42"
}
}
In the above code, the type Function<Integer, String>
is explicitly defined for clarity. However, if we were to omit it, the compiler would still infer the types based on context.
Using Target Type Inference with Collections
Often, target type inference shines in collection operations:
import java.util.ArrayList;
import java.util.List;
public class CollectionInferenceExample {
public static void main(String[] args) {
// Inferring types without explicit declaration
List<Integer> numbers = new ArrayList<>();
numbers.add(1);
numbers.add(2);
numbers.add(3);
// Using a lambda expression with target type inference
List<Integer> doubled = numbers.stream()
.map(n -> n * 2) // inferred as Integer -> Integer
.toList();
System.out.println(doubled); // Output: [2, 4, 6]
}
}
In this example, the compiler infers that n
in the lambda expression is of type Integer
due to the context of the map
method on a List<Integer>
.
Limitations and Risks
While target type inference makes coding easier, it also has its limitations:
-
Ambiguous Types: When the context is not clear, the compiler may throw errors or produce unexpected behavior. It is often better to be explicit in cases where types can lead to ambiguity.
-
Readability: Over-reliance on inference may lead to code that is harder for others (or yourself) to read. Balancing explicit declarations with inferred types is key.
-
Version Compatibility: Target type inference is supported only in Java 8 and later. For older versions of Java, you must define types explicitly.
Best Practices for Target Type Inference
-
Use Explicit Types When Necessary: If a method's expected type is complex, consider specifying the type explicitly to aid in readability.
-
Practice Consistency: Strive for a consistent coding style among your team. Using target type inference can enhance brevity, but clarity should not be sacrificed.
-
Familiarize with Functional Interfaces: Understanding Java's built-in functional interfaces, such as
Predicate
,Function
, andConsumer
, will help you leverage target type inference more effectively.
Learning More
If you want to deepen your understanding of Java 8 and functional programming concepts, consider checking out the following resources:
- Java SE 8 for the Really Impatient by Cay S. Horstmann
- Oracle’s Official Java Documentation for an overview of Java 8 features.
- Functional Programming in Java by Venkat Subramaniam.
Key Takeaways
Java 8's target type inference is a powerful feature that can enhance the way you write Java code. By allowing the compiler to deduce type information based on context, you can create cleaner, more concise code. As you practice and implement these principles in your own projects, remember to balance clarity with convenience.
Harness the power of target type inference, and let your Java programming become more intuitive and efficient than ever before. Happy coding!
Checkout our other articles