Mastering Recipe Null Errors: A Developer's Guide
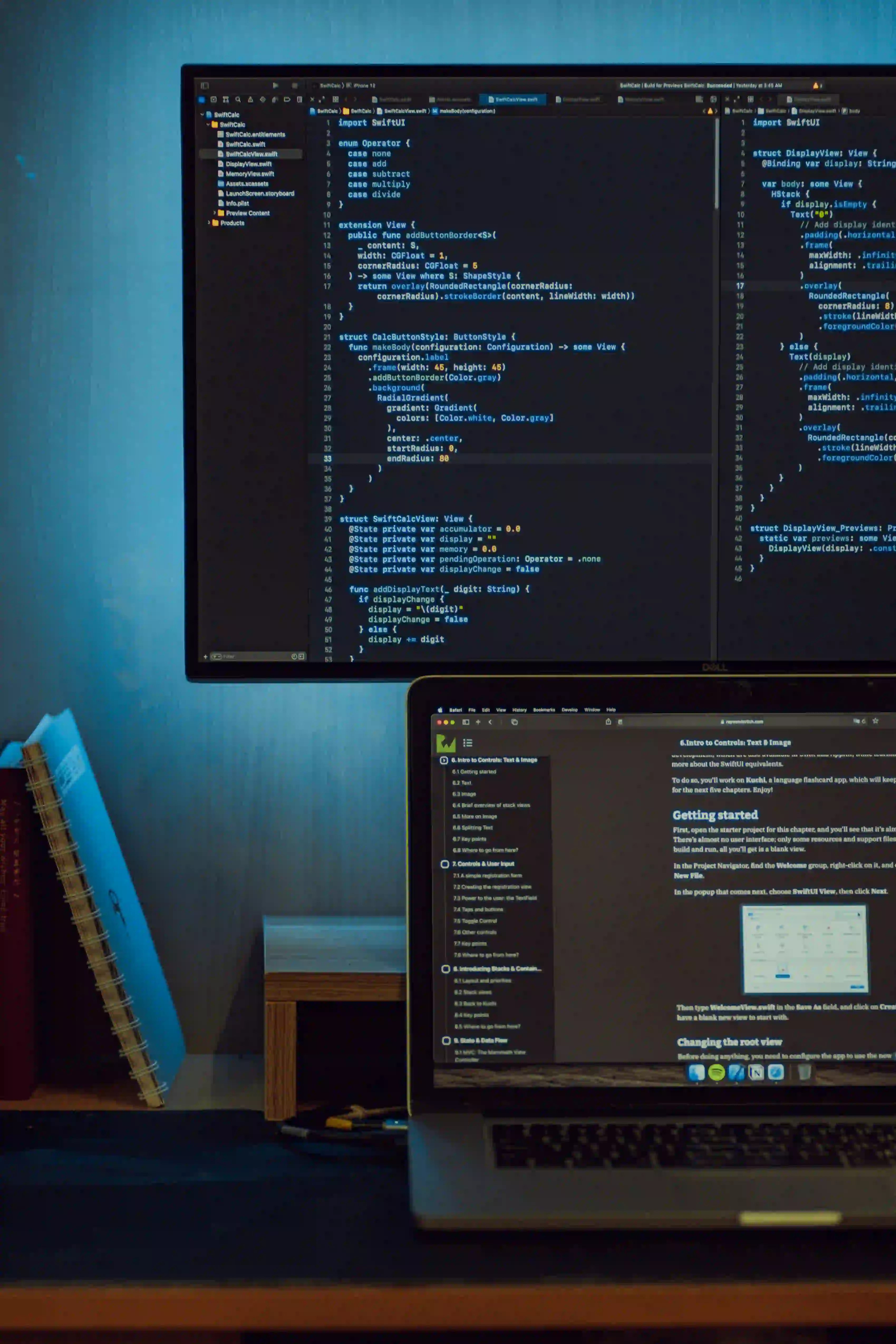
Mastering Recipe Null Errors: A Developer's Guide
In the world of Java programming, error handling is an essential skill for developers. Among the myriad of exceptions that can occur, null pointer exceptions stand out as a common annoyance, often arising at the least expected time. In this blog post, we are going to dive deep into the nuances of "recipe null errors," a term I use to describe the frustrating scenarios when developers encounter null values in their code. Along the way, we'll explore strategies to prevent these errors, best practices for handling them, and how to write cleaner, more reliable Java code.
Understanding Null Pointer Exceptions
Before we get into the strategies for mastering null pointer exceptions, let’s clarify what they are. A null pointer exception occurs when the Java Virtual Machine (JVM) attempts to use an object reference that has not been initialized—meaning it points to null. This can often happen due to:
- Not initializing an object.
- Mismanaging object lifecycle.
- Incorrectly assuming that a method will not return null.
Code Snippet: Basic Null Pointer Exception
Consider the following simple Java code snippet that demonstrates a null pointer exception:
public class Recipe {
private String name;
public Recipe(String name) {
this.name = name;
}
public String getName() {
return name.toUpperCase();
}
public static void main(String[] args) {
Recipe recipe = null;
System.out.println(recipe.getName());
}
}
Why This Happens:
In this example, recipe
is initialized to null. When we call getName()
on a null reference, it throws a NullPointerException
. It's important to understand that this error interrupts the program flow and can lead to frustrating debugging sessions.
Best Practices to Avoid Null Pointer Exceptions
1. Use Optional<?>
One of the most effective strategies in modern Java (Java 8 and above) is to leverage the Optional
class. This container allows you to represent a value that may or may not be present, thus reducing the chances of encountering null.
Code Snippet: Using Optional
import java.util.Optional;
public class Recipe {
private Optional<String> name;
public Recipe(String name) {
this.name = Optional.ofNullable(name);
}
public String getName() {
return name.map(String::toUpperCase).orElse("No name");
}
public static void main(String[] args) {
Recipe recipe = new Recipe(null);
System.out.println(recipe.getName());
}
}
Why Use Optional:
By using Optional
, we avoid null reference checks directly and provide a default response if no value is present. This makes your API cleaner and reduces unchecked exceptions.
2. Defensive Programming
Another key strategy is to adopt defensive programming. This involves validating your inputs as an early defense against nulls.
Code Snippet: Defensive Programming
public class Recipe {
private String name;
public Recipe(String name) {
if (name == null || name.trim().isEmpty()) {
throw new IllegalArgumentException("Recipe name cannot be null or empty");
}
this.name = name;
}
public String getName() {
return name.toUpperCase();
}
public static void main(String[] args) {
try {
Recipe recipe = new Recipe(null);
} catch (IllegalArgumentException e) {
System.err.println(e.getMessage());
}
}
}
Why Validate Inputs:
By throwing an IllegalArgumentException
, we catch the issue early in the lifecycle, making it evident where the mistake lies. This proactive approach reduces debugging time significantly.
3. Initializing Defaults
When defining class attributes, you can set default values to eliminate null issues entirely.
Code Snippet: Setting Defaults
public class Recipe {
private String name = "Unnamed Recipe"; // Default value
public Recipe(String name) {
if (name != null && !name.trim().isEmpty()) {
this.name = name;
}
}
public String getName() {
return name.toUpperCase();
}
public static void main(String[] args) {
Recipe recipe = new Recipe(null);
System.out.println(recipe.getName());
}
}
Why Default Values:
This approach allows the object to always have a usable state, reducing the chances of null pointer exceptions significantly.
Using Annotations for Documentation
Java provides several annotations that can help document methods and parameters regarding nullability. Notably, the @NonNull
and @Nullable
annotations can clarify which values can be null.
Code Snippet: Using Annotations
import javax.annotation.Nullable;
public class Recipe {
private String name;
public Recipe(@Nullable String name) {
this.name = name;
}
public String getName() {
return name != null ? name.toUpperCase() : "No name";
}
public static void main(String[] args) {
Recipe recipe = new Recipe(null);
System.out.println(recipe.getName());
}
}
Why Use Annotations:
Annotations like @Nullable
are very useful in generating documentation and can be processed by static analysis tools to alert developers about potential null pointer exceptions upstream.
Handling Exceptions Gracefully
Even with the best strategies, there may be situations where you cannot avoid all null references. Instead of letting the program crash, handle them gracefully.
Code Snippet: Try-Catch Block
public class Recipe {
private String name;
public Recipe(String name) {
this.name = name;
}
public String getName() {
return name.toUpperCase();
}
public static void main(String[] args) {
Recipe recipe = new Recipe(null);
try {
System.out.println(recipe.getName());
} catch (NullPointerException e) {
System.err.println("Caught a null pointer exception: " + e.getMessage());
}
}
}
Why Catch Exceptions:
This approach keeps the application running and allows you to provide meaningful feedback to users. Logging the error can also help maintain transparency regarding issues that arise.
Closing the Chapter
Mastering null pointer exceptions is vital for Java developers seeking to write robust and maintainable code. By employing strategies such as using Optional
, engaging in defensive programming, initializing defaults, and documenting code with annotations, you can effectively mitigate the risks associated with null values. Finally, handling exceptions gracefully protects your application's integrity, ensuring a better user experience.
To recap:
- Use
Optional
to avoid nulls. - Validate inputs with defensive programming.
- Set defaults for class attributes.
- Document nullability with annotations.
- Gracefully handle exceptions.
Further Learning Resources:
- Java Optional Documentation
- Effective Java - Second Edition by Joshua Bloch
- Java Exception Handling Guide
Master these concepts, and soon null pointer exceptions will be a minor inconvenience rather than a major roadblock in your Java journey. Happy coding!