Unlocking the Secrets of Java Flight Recorder in Java 11
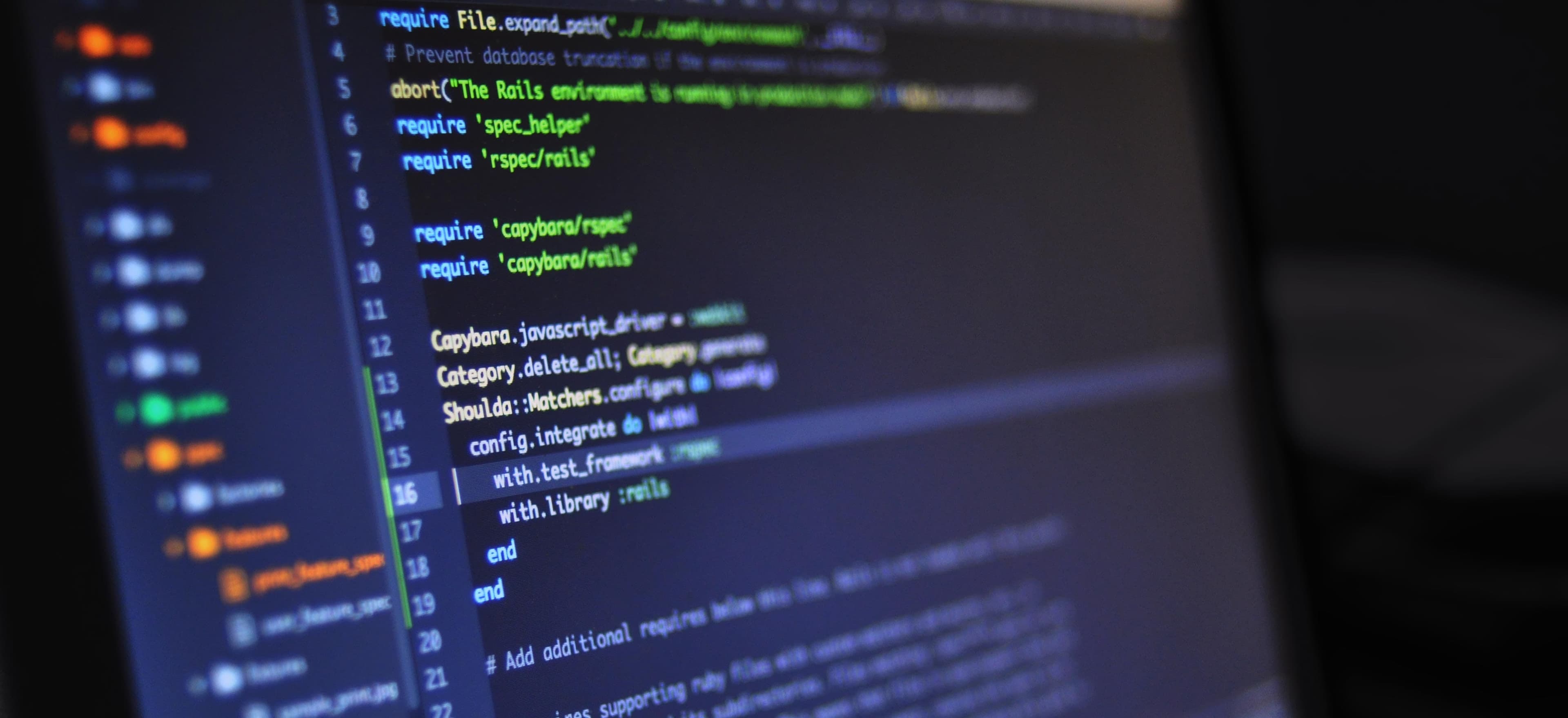
- Published on
Unlocking the Secrets of Java Flight Recorder in Java 11
Java Flight Recorder (JFR) is a powerful tool that allows developers to collect and analyze performance data from Java applications. Introduced in Java 11, it provides insight into application behavior, resource consumption, and helps to troubleshoot performance issues. In this blog post, we will explore what JFR is, how to use it, and its benefits, along with practical code examples to illustrate its capabilities.
What is Java Flight Recorder?
Java Flight Recorder is an advanced profiling tool that captures detailed information about the state of a Java application. It allows developers to understand runtime behavior, analyze CPU and memory usage, and diagnose performance bottlenecks. Unlike traditional profilers, JFR has a minimal impact on application performance, making it ideal for production environments.
Key Features of Java Flight Recorder
- Low Overhead: JFR is designed for production, ensuring that the performance overhead while capturing data is minimal, typically a few percent.
- Event-Based Model: JFR captures a series of events that occur during application execution, which can be analyzed for various metrics.
- Integration with Java Virtual Machine (JVM): JFR integrates seamlessly with the JVM, allowing for easy data collection without requiring additional instrumentation.
- Flexibility: You can configure JFR to collect only specific events, reducing the amount of data collected and focusing on relevant metrics.
Setting Up Java Flight Recorder
To start using JFR, ensure you have at least Java 11 installed on your machine. You can download it from the official Oracle website.
Starting a Java Application with JFR
To enable JFR when launching your application, you can use the -XX:StartFlightRecording
option alongside other parameters. Here’s an example command to start a simple Java application with JFR enabled:
java -XX:StartFlightRecording=duration=60s,filename=myrecording.jfr -jar myapp.jar
In this command:
duration=60s
specifies that the recording should last for 60 seconds.filename=myrecording.jfr
designates the output file for the recorded data.
Example: Java Application with JFR Enabled
public class HelloJFR {
public static void main(String[] args) {
for (int i = 0; i < 1_000_000; i++) {
compute(i);
}
}
private static void compute(int value) {
// Simple computation to simulate work
double result = Math.sqrt(value);
System.out.println("Computed value: " + result);
}
}
Run the application with the command provided earlier, and it will generate a JFR file named myrecording.jfr
after the execution completes.
Analyzing JFR Data
To analyze the recorded data, you can use Java Mission Control (JMC), a suite of tools designed for this purpose. JMC provides a graphical interface through which you can view various metrics.
The JMC Interface
- Overview Tab: Displays high-level metrics such as CPU usage, memory consumption, and thread activity.
- Event Browser: Allows you to explore detailed recordings and filter specific events related to garbage collection, method calls, and exceptions.
- Memory Leak Analysis: Offers tools to identify potential memory leaks by monitoring object retention and allocation patterns.
Example Analysis Workflow
After running your Java application and generating a JFR file, follow these steps:
- Open JMC.
- Load your
.jfr
file. - Navigate to the Overview tab for insights into application performance.
- Use the Event Browser to dig deeper into specific areas of interest, such as garbage collection events or time spent in methods.
Using JFR in Production
One of the most compelling features of JFR is its usability in production environments. Capturing performance data without significant overhead can help track down issues live without impacting user experience.
Recording Events of Interest
Java Flight Recorder allows you to specify which events to capture. For example, you could opt only to record CPU, memory, or I/O-related events. To do this, adjust the -XX:StartFlightRecording
parameters like so:
java -XX:StartFlightRecording=duration=120s,filename=myrecording.jfr,settings=profile -jar myapp.jar
The settings=profile
configuration will collect a predefined set of events that give a clear picture of application performance.
Best Practices for Using JFR in Production
- Start Small: Commonly, it's beneficial to begin recording only essential data and incrementally capture additional events as needed.
- Use with Diagnostics: Combine JFR with other application diagnostic tools for a holistic view of performance metrics.
- Automate Analysis: Establish regular analysis sessions to ensure performance monitoring isn't a one-time task.
Advanced Features of Java Flight Recorder
As you become more comfortable with JFR, consider leveraging these advanced capabilities.
Custom Events
JFR allows you to create custom events that can be logged during specific operations. This provides a tailored profiling experience. Example code for a custom event might look like this:
import jdk.jfr.Event;
import jdk.jfr.OnError;
import jdk.jfr.Recording;
public class PerformanceMonitor {
@OnError
public void onError(Throwable error) {
System.out.println("Error captured: " + error.getMessage());
}
public void criticalOperation() {
Event event = new Event("CriticalOperationEvent");
event.begin();
try {
// Perform operation
} finally {
event.end();
}
}
}
Here, the Event
class represents a custom event, which starts when a critical operation begins and ends when the operation concludes. By adding this enhancement, you can gain profound insight into vital application functionalities.
Continuous Monitoring
You can configure JFR to run continuously, capturing data over a longer period to identify trends or recurring issues. This can be useful during high-load periods or when deploying new features.
java -XX:StartFlightRecording=filename=myrecording.jfr -jar myapp.jar
By omitting the duration
parameter, you enable continuous recording until the application stops or the recording is manually stopped.
To Wrap Things Up
Java Flight Recorder is an invaluable asset for Java developers seeking to enhance their applications' performance. By providing detailed insight into runtime behavior with minimal overhead, JFR allows for efficient troubleshooting and performance optimization.
Next time you face performance challenges or need to understand your Java application better, consider utilizing Java Flight Recorder. Start by enabling it in your environment, employing JMC for analysis, and experimenting with custom events for additional insight.
For further resources on Java Flight Recorder and performance tuning in Java, check out the following links:
- Official Java Flight Recorder Documentation
- Java Mission Control Guide
Happy profiling!