Overcoming UI Challenges in Codename One Chat Apps
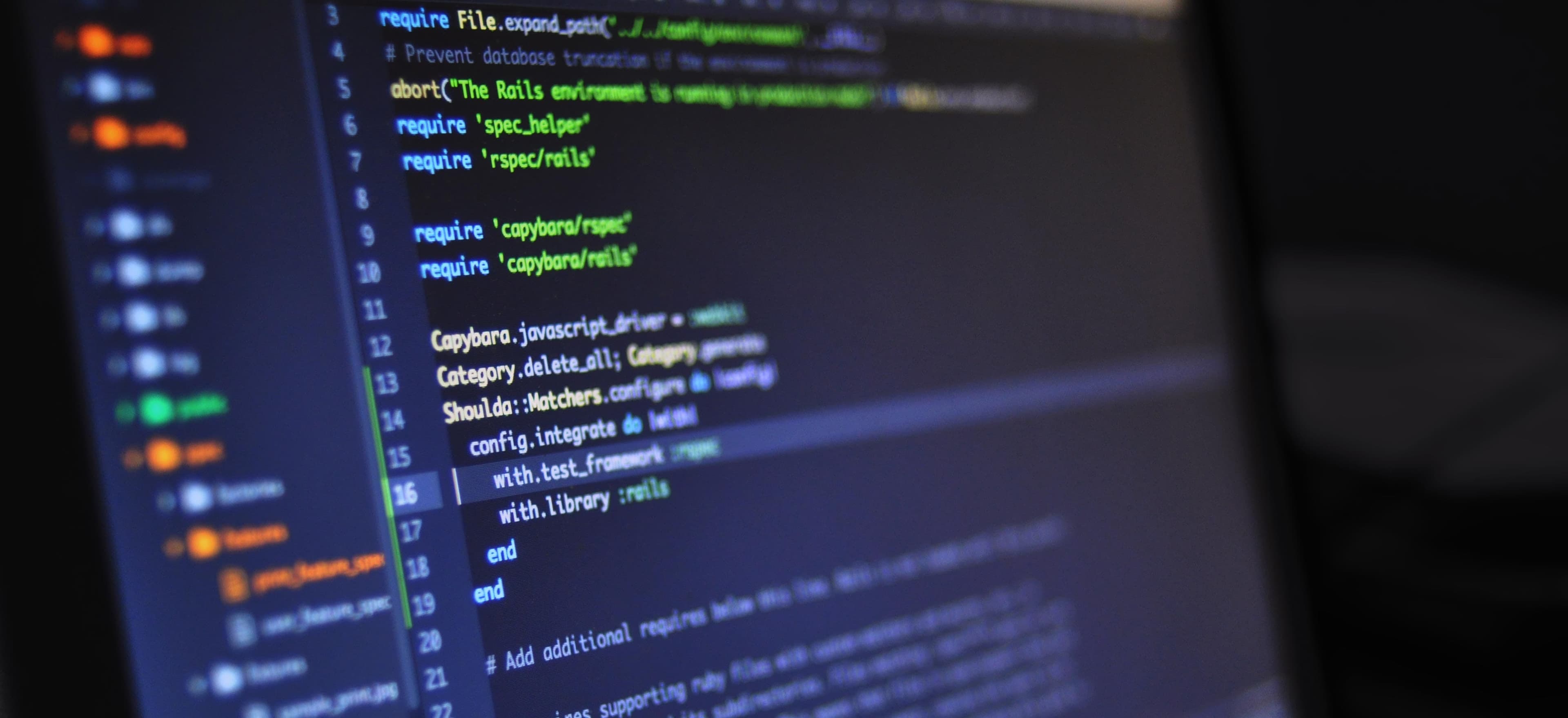
- Published on
Overcoming UI Challenges in Codename One Chat Apps
In today's digital landscape, chat applications have become fundamental tools for communication. Building a chat app with Codename One, a popular cross-platform mobile application development framework, can be a rewarding yet challenging endeavor. One of the most significant hurdles developers face is User Interface (UI) design and functionality. In this blog post, we will explore common UI challenges encountered in developing chat apps with Codename One, along with practical solutions and code snippets to help streamline the process.
Why Choose Codename One for Chat Apps?
Before diving into specific UI issues, let's briefly highlight the advantages of using Codename One:
- Cross-Platform Compatibility: Write once, run anywhere. Codename One enables you to develop applications for various platforms including iOS, Android, and desktops seamlessly.
- Rich UI Components: It offers a variety of UI components that can be easily customized.
- High Performance: Codename One applications are known for their performance, thanks to optimizations that occur under the hood.
Common UI Challenges in Chat Applications
When creating chat applications, developers often face various UI-related challenges, such as:
- Dynamic loading of messages
- Conversations with numerous participants
- User engagement through notifications
- Designing an intuitive input field for text messages
In the sections below, we will delve into these challenges and explore effective solutions with code examples.
1. Dynamic Loading of Messages
In chat apps, users expect messages to appear in real-time. Handling dynamic data can be tricky. Here's how you can refresh the chat list efficiently:
Code Snippet: Updating Messages Dynamically
private List<Message> messages = new ArrayList<>();
private Container messageListContainer;
// Method to load messages
private void loadMessages() {
messageListContainer.removeAll();
for (Message msg : messages) {
Label messageLabel = new Label(msg.getText());
messageListContainer.add(messageLabel);
}
messageListContainer.revalidate();
}
Explanation of the Code:
- We initialize a list of messages and a Container to hold those messages.
- The
loadMessages
method clears the existing messages, iterates through the list of messages, and adds them to themessageListContainer
. - The
revalidate
method updates the UI to reflect the new state. This method is critical for ensuring your UI reflects the most current data.
2. Handling Conversations with Multiple Participants
In group chats, managing conversations can become complex. Codename One offers flexible container layouts that can help in visually categorizing participants.
Code Snippet: Group Chat Layout
private Container createGroupChatUI(List<Participant> participants) {
Container groupChatContainer = new Container(new BoxLayout(BoxLayout.Y_AXIS));
for (Participant participant : participants) {
Button participantButton = new Button(participant.getName());
participantButton.addActionListener(e -> showParticipantDetails(participant));
groupChatContainer.add(participantButton);
}
return groupChatContainer;
}
private void showParticipantDetails(Participant participant) {
Dialog.show("Participant Details", "Name: " + participant.getName(), "OK", null);
}
Explanation of the Code:
- Here, we create a
Container
for the group chat UI using a vertical BoxLayout. - Each participant is represented as a button, and clicking the button displays their details in a dialog.
- This structure provides a user-friendly way to interact with multiple participants without overwhelming the user.
3. User Engagement through Notifications
In a chat app, timely notifications are critical for user engagement. Codename One allows for notifications that can alert users of new messages even when the app is not in use.
Code Snippet: Sending Notifications
private void sendNotification(String message) {
Display.getInstance().callSerially(() -> {
Notification n = new Notification("New Message", message);
n.show();
});
}
Explanation of the Code:
- We utilize the
Notification
class in Codename One, which allows for straightforward alerts. - The
callSerially
method ensures that the notification is shown on the UI thread, which is a good practice to avoid any threading issues.
4. Designing an Intuitive Input Field
A user-friendly text input field is the cornerstone of any chat app. A clean design helps users send messages effortlessly.
Code Snippet: Input Field UI
private void createInputField() {
TextField inputField = new TextField();
inputField.setHint("Type a message...");
Button sendButton = new Button("Send");
sendButton.addActionListener(e -> {
String text = inputField.getText();
if (!text.isEmpty()) {
sendMessage(text);
inputField.clear();
}
});
Container inputContainer = new Container(new BorderLayout());
inputContainer.add(BorderLayout.CENTER, inputField);
inputContainer.add(BorderLayout.EAST, sendButton);
add(inputContainer);
}
Explanation of the Code:
- We create a
TextField
to allow users to input their messages, accompanied by a "Send" button. - On clicking the send button, if the text is not empty, it proceeds to send the message and clears the input field. This fosters a cleaner user interface and enhances usability.
The Closing Argument
Building a chat application in Codename One is an exciting venture filled with challenges, particularly related to user interface design. However, with careful planning and the right approach, you can deliver a polished and user-friendly application. We discussed dynamic message loading, managing group conversations, engaging users through notifications, and designing intuitive input fields—all crucial aspects of a successful chat app.
As you continue to develop your chat application, remember to focus on the user experience. Test your UI thoroughly with actual users to gather feedback, and iterate your design accordingly. For additional resources, consider checking the Codename One Documentation or exploring the Codename One GitHub repository.
Happy coding, and may your chat apps foster meaningful connections!
Checkout our other articles