Troubleshooting Camel's Twitter and WebSocket Integration
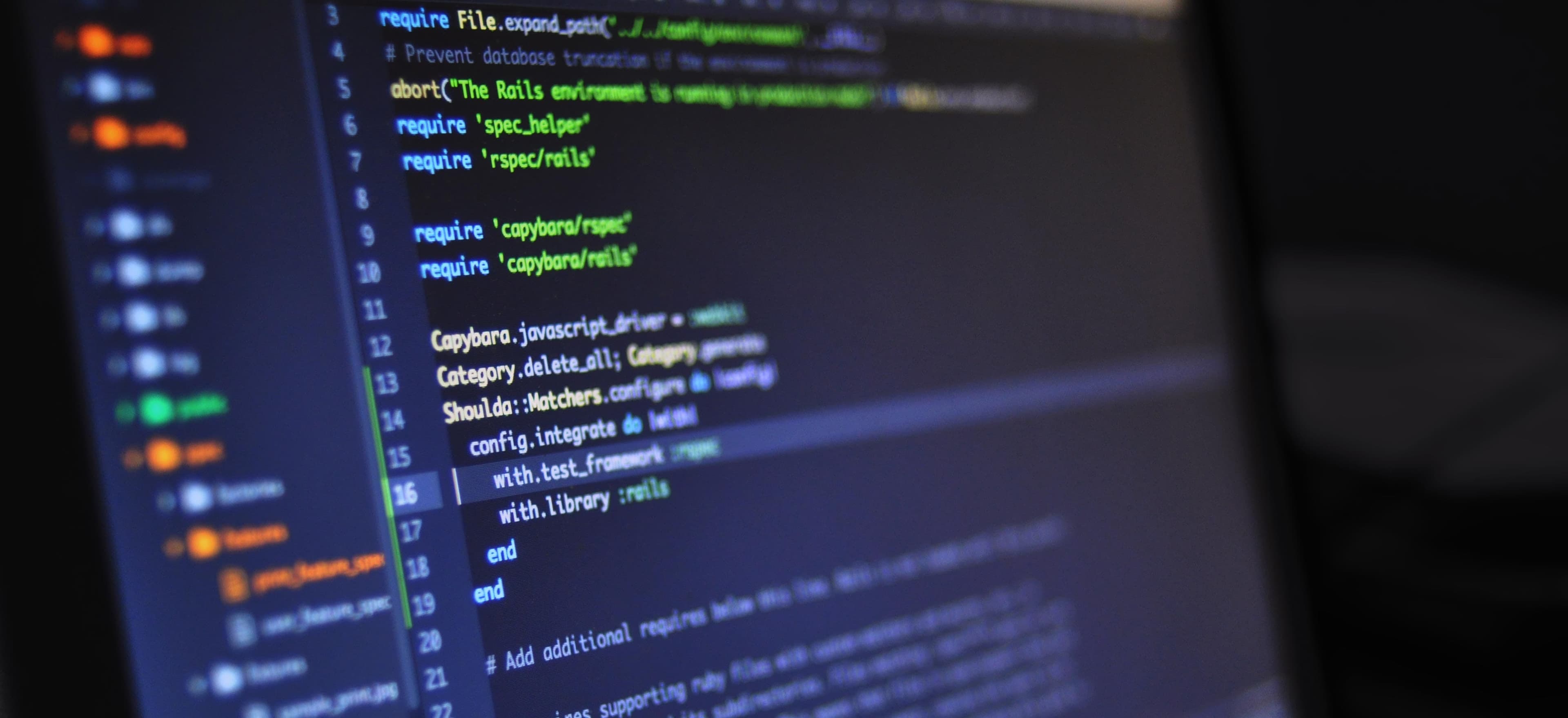
- Published on
Troubleshooting Camel's Twitter and WebSocket Integration
Apache Camel is a powerful integration framework that provides extensive capabilities to connect various applications and services. One of the most robust features of Camel is its ability to handle real-time data streaming via Twitter and WebSocket. This blog post will explore common problems you might encounter while integrating Twitter with WebSocket through Apache Camel and provide solutions to troubleshoot them effectively.
Table of Contents
- Overview of Apache Camel
- Understanding the Camel Twitter Component
- Understanding the Camel WebSocket Component
- Common Issues in Twitter and WebSocket Integration
- Debugging and Troubleshooting Techniques
- Example Code Snippet
- Additional Resources
1. Overview of Apache Camel
Apache Camel is an open-source integration framework that allows you to connect different systems seamlessly. With its "Enterprise Integration Patterns" (EIP), Camel simplifies the integration of various components, such as databases, messaging queues, and APIs, making development easier and more efficient.
This integration framework is designed to be lightweight and flexible. It supports multiple programming languages and allows developers to create custom routes that dictate how data flows between sources and destinations.
2. Understanding the Camel Twitter Component
Camel's Twitter component enables developers to interact with Twitter's API effortlessly. You can retrieve tweets, follow users, and even send tweets directly from your application. Some key benefits include:
- Easy access to Twitter's streaming API.
- Integration with other components for comprehensive data workflows.
Key Configurations
To get started, you'll need Twitter API credentials. You must set these up in your Camel route:
from("twitter://streaming/filter?keywords=camel")
.to("log:twitter");
This configuration streams tweets containing the keyword "camel" to the log.
3. Understanding the Camel WebSocket Component
The WebSocket component in Camel allows for real-time web communication, making it possible to push data to clients instantly. This is incredibly beneficial when working with dynamic data sources like Twitter where you want to keep clients updated in real time.
Key Configurations
A simple WebSocket route might look like this:
from("websocket://localhost:8080/tweets")
.to("log:websocket");
This configuration listens for WebSocket connections on port 8080
and logs any incoming messages.
4. Common Issues in Twitter and WebSocket Integration
While integrating Twitter and WebSocket using Camel, you might encounter several common issues:
4.1 Authentication Errors
Authentication errors often occur when your API keys are incorrect or have expired. Verify that you have the correct credentials in your Camel configuration and ensure they are up-to-date.
4.2 Connection Issues
If your WebSocket client cannot connect to the server, check the following:
- Make sure the WebSocket server is running.
- Verify that there are no firewall rules blocking access to the WebSocket port.
4.3 Data Format Issues
Incompatibilities in data format can cause data transmission errors. Ensure that the data sent from the Twitter stream is in a format that can be parsed and understood by the WebSocket client.
4.4 High Traffic Handling
Handling a large volume of Tweets and WebSocket connections can overwhelm your application. Ensure that you implement proper throttling and manage concurrency effectively.
5. Debugging and Troubleshooting Techniques
Debugging Camel applications efficiently can save a significant amount of time. Here are several techniques that can help:
5.1 Enable Debug Logs
Apache Camel allows you to set log levels for better debugging. Set a debug log level in your log4j.properties
file:
log4j.logger.org.apache.camel=DEBUG
This will give you deeper insights into what's happening during data flow.
5.2 Use the Dashboards
Use tools like Hawtio for monitoring Camel routes. It provides a user-friendly UI to visualize your routes and identify bottlenecks in real-time.
5.3 Unit Testing
Unit testing your Camel routes can help catch issues before going live. Use Camel's testing framework to write unit tests for your routes. A simple test could look like:
public class MyRouteTest extends CamelTestSupport {
@Override
protected RouteBuilder createRouteBuilder() {
return new MyRoute();
}
@Test
public void testTwitterIntegration() throws Exception {
getMockEndpoint("mock:websocket").expectedMessageCount(1);
template.sendBody("twitter://streaming/filter?keywords=camel", "Test Tweet");
assertMockEndpointsSatisfied();
}
}
This helps ensure that your route behaves as expected.
6. Example Code Snippet
Here’s a complete example that illustrates how to pull tweets from Twitter and push them to WebSocket clients.
import org.apache.camel.builder.RouteBuilder;
import org.apache.camel.main.Main;
public class TwitterWebSocketIntegration {
public static void main(String[] args) throws Exception {
Main main = new Main();
main.addRoutes(new RouteBuilder() {
@Override
public void configure() {
from("twitter://streaming/filter?keywords=camel")
.log("New Tweet: ${body}")
.to("websocket://localhost:8080/tweets");
}
});
main.start();
}
}
Explanation of the Code
- The Twitter component retrieves tweets containing "camel" and logs them.
- The tweets are then pushed via WebSocket, allowing real-time updates to connected clients.
7. Additional Resources
To dive deeper into these technologies and troubleshoot effectively, you can check the following resources:
- Apache Camel Documentation
- Apache Camel Twitter Component
- WebSocket Protocol Overview
The Closing Argument
Integrating Twitter and WebSocket with Apache Camel allows for innovative real-time applications, but it comes with its challenges. Authentication issues, connection problems, data format mismatches, and high traffic can arise.
By employing various debugging strategies and utilizing the provided code snippets, you can overcome common pitfalls and enhance your integration projects.
If you have questions or further insights about working with Apache Camel, feel free to leave your comments below!
Checkout our other articles