Mastering Java 9: The Pitfalls of Default Private Methods
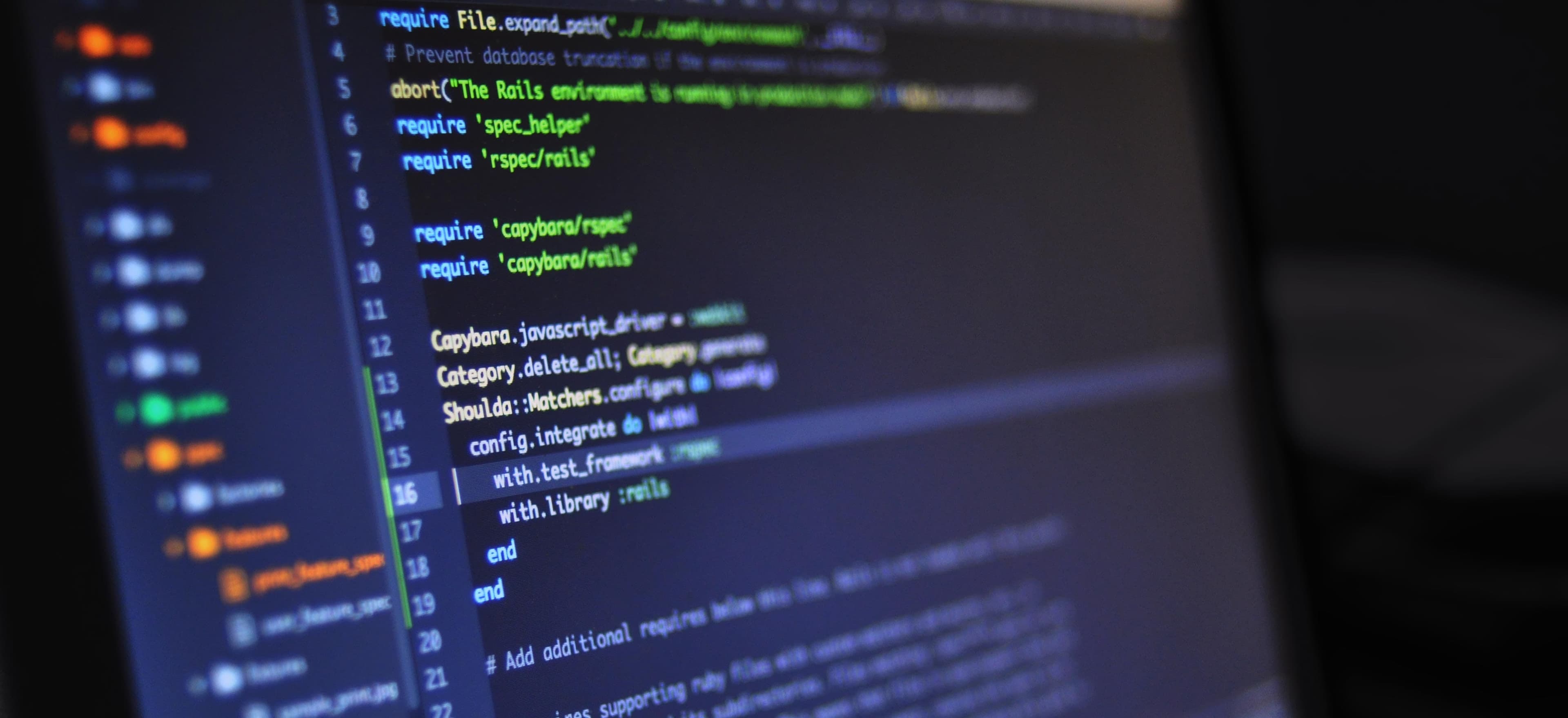
- Published on
Mastering Java 9: The Pitfalls of Default Private Methods
Java 9 introduced several enhancements that improved performance, interface design, and developer experience. Among these enhancements, one of the most significant changes was the ability to declare private methods within interfaces. These default private methods provided a new level of abstraction and code reuse that was previously unattainable in Java interfaces. While this feature has its benefits, it also comes with potential pitfalls that every Java developer should be aware of.
In this blog post, we will delve into the concept of default private methods, exploring their advantages while highlighting their pitfalls. By the end, you will have a solid understanding of how to leverage this feature effectively and avoid common mistakes.
Understanding Default Private Methods
First, let’s clarify what default private methods are in the context of Java interfaces. A default method is a specific type of method that allows developers to add new functionality to interfaces without breaking existing implementations. However, with Java 9, private methods were introduced, meaning developers can now create helper methods that are only accessible within the interface itself.
Code Example
Consider the following interface with a default private method:
public interface Shape {
default void draw() {
prepareCanvas();
System.out.println("Drawing the shape");
}
private void prepareCanvas() {
System.out.println("Preparing the canvas");
}
}
Why Use Default Private Methods?
-
Code Reusability: By encapsulating common logic within private methods, code duplication is minimized. This is particularly useful when you have multiple default methods requiring similar operations or calculations.
-
Encapsulation: These private methods are hidden from the outer world, keeping the interface clean and protecting its internal mechanics from users.
-
Facilitating Future Changes: If you change the implementation of a private method, it automatically affects all default methods relying on it. This decoupling makes future modifications easier.
The Pitfalls of Default Private Methods
Despite the advantages of using default private methods, they carry certain pitfalls that developers must navigate carefully.
1. Complexity and Readability
Adding private methods can lead to increased complexity within an interface. When interfaces grow complicated, they can detract from the simplicity that interfaces are designed to maintain.
Example:
public interface OrderProcessor {
default void processOrder(Order order) {
validateOrder(order);
if (order.isValid()) {
chargeCustomer(order);
}
}
private void validateOrder(Order order) {
System.out.println("Validating order...");
// complex validation logic
}
private void chargeCustomer(Order order) {
System.out.println("Charging customer for order...");
// charging logic
}
}
While the private methods provide essential functionality, they make it less obvious what the processOrder
method does at a glance.
2. Overhead in Future Maintenance
Over time, interfaces can become bloated with numerous default methods and private helpers. Introducing new developers to the project becomes more challenging as they try to grasp the functionality encapsulated in complex private methods.
Advice: Always strive for clarity. Keep private methods focused on a single responsibility, and ensure naming conventions convey their purpose effectively.
3. Unintended Side Effects
Since private methods are only accessible within the interface, their modification can have unintended side effects on all public or default methods that utilize them. This could lead to bugs that are difficult to track down.
4. Break with Liskov Substitution Principle
The Liskov Substitution Principle states that objects of a superclass should be replaceable with objects of a subclass without affecting the correctness of the program. When using default private methods, any implementation of the interface is coupled with the internal implementation details that might not be relevant or needed.
Code Example:
Consider extending an interface with a new class:
public class Circle implements Shape {
// Circle implementation here, but draw() behavior is inherited
}
If the Shape
interface changes because of internal changes to private methods, the behavior in Circle
will also change, potentially breaking existing functionality.
Best Practices
1. Use Sparingly
Only use private methods when the code benefits significantly from code reuse or encapsulation. Do not create private methods for small sections of code that are unlikely to be reused.
2. Emphasize Documentation
Ensure that each private method has clear comments describing its purpose and any important functionality. This saves time for developers who might be debugging or extending the codebase later on.
3. Consider Composition Over Inheritance
Whenever possible, favor composition to handle shared logic instead of using private methods in interfaces. Composition usually results in cleaner, more maintainable code.
public class ShapeUtilities {
public static void prepareCanvas() {
System.out.println("Preparing the canvas");
}
}
4. Unit Testing
While private methods in interfaces can’t be tested directly, ensure that the default methods which use them are well-tested. This way, the integrity of your logic remains intact.
Lessons Learned
Default private methods in Java 9 provide a powerful tool for interface design and implementation. They facilitate code reuse and enhance encapsulation. However, they are not without their complexities and risks.
Adopting best practices, maintaining clarity, and considering the overall design when integrating default private methods can significantly mitigate these pitfalls. Always remember that while it’s tempting to utilize new features, understanding when and how to use them is crucial for maintaining robust Java applications.
For further reading on Java 9 features, check out Oracle's Official Documentation on Java 9 and explore community insights on Java 9 Enhancements by Baeldung.
By mastering these concepts and being aware of potential pitfalls, you can effectively harness the power of Java 9 and elevate your programming skills to new heights. Happy coding!
Checkout our other articles