Common Pitfalls in One-to-Many XML Mapping with Hibernate
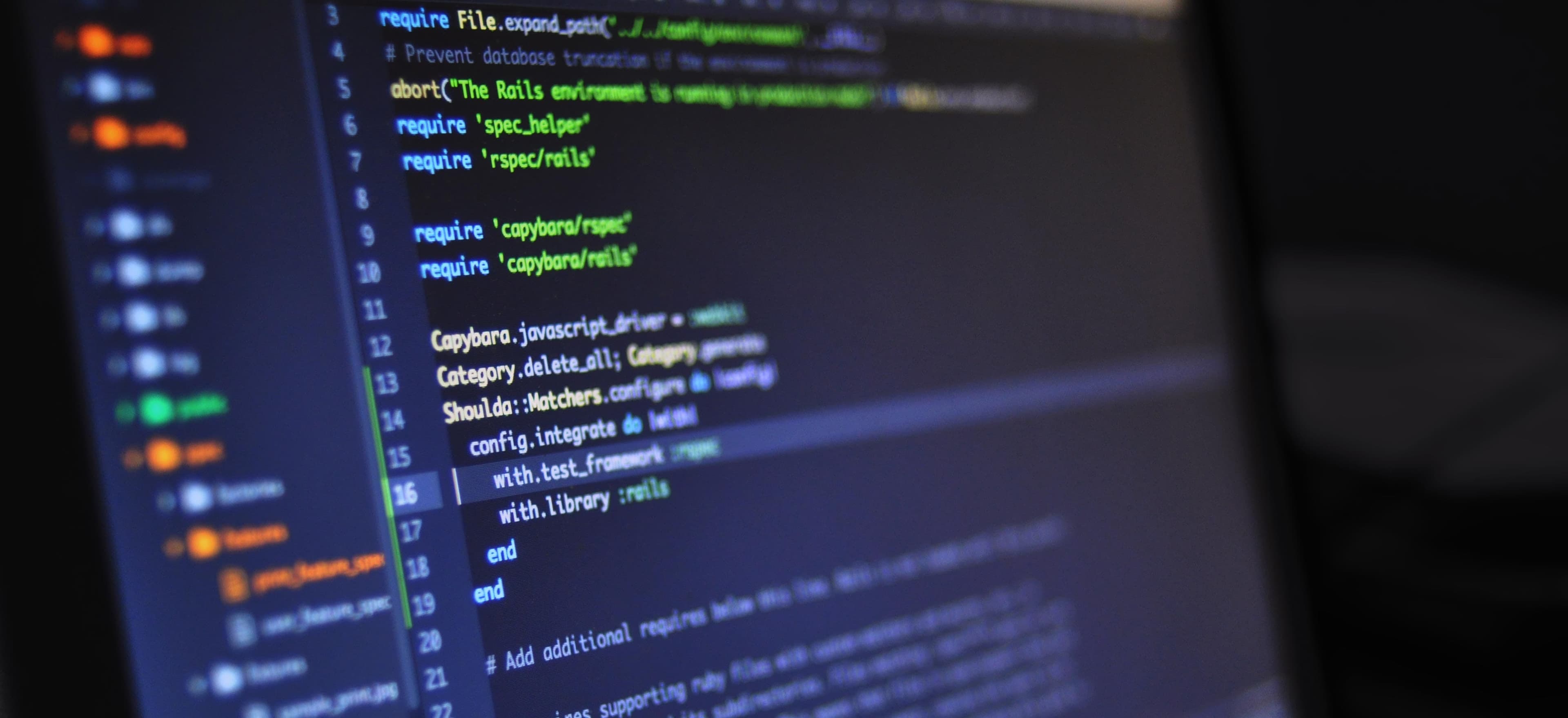
- Published on
Common Pitfalls in One-to-Many XML Mapping with Hibernate
Hibernate is a powerful Object-Relational Mapping (ORM) framework in Java that simplifies database interactions. However, working with complex relationships such as one-to-many mappings using XML configuration can lead to several pitfalls. In this blog post, we will discuss common mistakes developers make when implementing one-to-many mappings with Hibernate through XML and provide insights on how to avoid these issues.
Understanding One-to-Many Relationships
A one-to-many relationship means that one entity (the ‘one’ side) can be associated with multiple instances of another entity (the ‘many’ side). For example, consider a Customer
entity that can have multiple Order
entities.
Here’s a simplified representation of this relationship:
- Customer (1)
- Order (1)
- Order (2)
- Order (3)
Importance of XML Mapping
Unlike annotations, XML mapping provides a more centralized configuration, making it easier to manage complex relationships and configurations in larger applications. However, leveraging XML for one-to-many relationships can create complications if not handled correctly.
Common Pitfalls and Their Solutions
Below are frequently encountered issues during one-to-many XML mapping in Hibernate, along with recommended solutions.
1. Improper Element Mapping
Pitfall: Many developers mistakenly map the collection in the wrong manner, especially when defining the child entity. This can lead to runtime exceptions, such as MappingException
.
Solution: Ensure that you are mapping the collection correctly. Here’s an example:
<class name="com.example.Customer">
<id name="id" column="customer_id">
<generator class="increment"/>
</id>
<property name="name" column="customer_name"/>
<bag name="orders" table="orders" inverse="true" cascade="all">
<key column="customer_id"/>
<one-to-many class="com.example.Order"/>
</bag>
</class>
Commentary:
- bag: The
bag
element is used for collections where duplicates are allowed, and order is not significant, making it a suitable choice forOrder
entities belonging to aCustomer
. - inverse="true": This setting indicates that the child entity
Order
manages the foreign key. - cascade:
cascade="all"
ensures that when aCustomer
is saved or deleted, all associatedOrder
entities are also handled accordingly.
2. Failure to Configure Fetch Type
Pitfall: Not specifying the fetch type can lead to performance issues when loading related entities lazily, especially in large collections.
Solution: Explicitly define your fetch strategy. For instance, you can emphasize that you want the orders to be fetched eagerly:
<bag name="orders" fetch="select" table="orders" inverse="true" cascade="all">
Commentary:
- fetch="select": The
select
strategy fetches the collection when the parent entity is loaded. This can lead to performance hits if not managed correctly. For many scenarios, you may want to adhere to lazy loading (fetch="join"
), depending on your use cases.
3. Improper Transaction Management
Pitfall: Not managing transactions properly often results in inconsistent data, especially when cascades are involved.
Solution: Always ensure that your transaction boundaries are clearly defined. Here’s an example snippet demonstrating proper transaction usage:
Session session = sessionFactory.openSession();
Transaction transaction = null;
try {
transaction = session.beginTransaction();
Customer customer = new Customer();
customer.setName("John Doe");
Order order1 = new Order(...);
Order order2 = new Order(...);
// Set orders
customer.getOrders().add(order1);
customer.getOrders().add(order2);
session.save(customer);
transaction.commit();
} catch (Exception e) {
if (transaction != null) {
transaction.rollback();
}
} finally {
session.close();
}
Commentary:
- transaction management: Ensure every database interaction is wrapped in a transaction block to maintain integrity. Failing to do so can lead to partial updates or corrupted data states.
4. Neglecting to Use the Correct Collection Type
Pitfall: Using inappropriate collection types leads to problems with performance and efficacy.
Solution: Choose the right type of collection based on your use case. For example, using a Set
instead of a Bag
can offer better performance and ensure uniqueness.
Here’s an example with a Set
:
<set name="orders" table="orders" inverse="true" cascade="all">
<key column="customer_id"/>
<one-to-many class="com.example.Order"/>
</set>
Commentary:
- set: Enforces uniqueness within the collection. This is crucial when duplicate orders are not acceptable in the context of a customer.
5. Ignoring Updates to Child Entities
Pitfall: Failing to properly manage state transitions of child entities can lead to confusion and unexpected behavior.
Solution: Always maintain references to your owned entities. When modifying a child's state (like the status of an order), ensure you reflect those changes in both the child and the parent entities.
Order order = session.get(Order.class, orderId);
order.setStatus("Completed");
session.update(order);
Commentary:
- state change awareness: Managing synchronous updates between parent and child entities is essential to immutable relationships, avoiding stale data references.
6. Combining Annotations and XML Configuration
Pitfall: Mixing XML and annotation-based configuration can lead to conflicts and confusion.
Solution: Stick to one mapping strategy. If you start with XML, continue with it. If annotations are preferable, migrate fully to annotations for consistency.
In Conclusion, Here is What Matters
Implementing one-to-many relationships in Hibernate using XML mapping can indeed be tricky. By recognizing these common pitfalls and following the solutions outlined, you can navigate through your ORM tasks more effectively.
As developers, growing our understanding of these challenges not only enhances our coding efficiency but also contributes to a more stable application environment.
For further exploration on Hibernate mappings, consider checking out Hibernate Documentation.
With these insights, you're now equipped to handle one-to-many XML mappings while steering clear of common mistakes. Happy coding!
Feel free to leave your comments or ask questions regarding your experiences with Hibernate!
Checkout our other articles