Common Command Line Pitfalls When Handling JARs and WARs
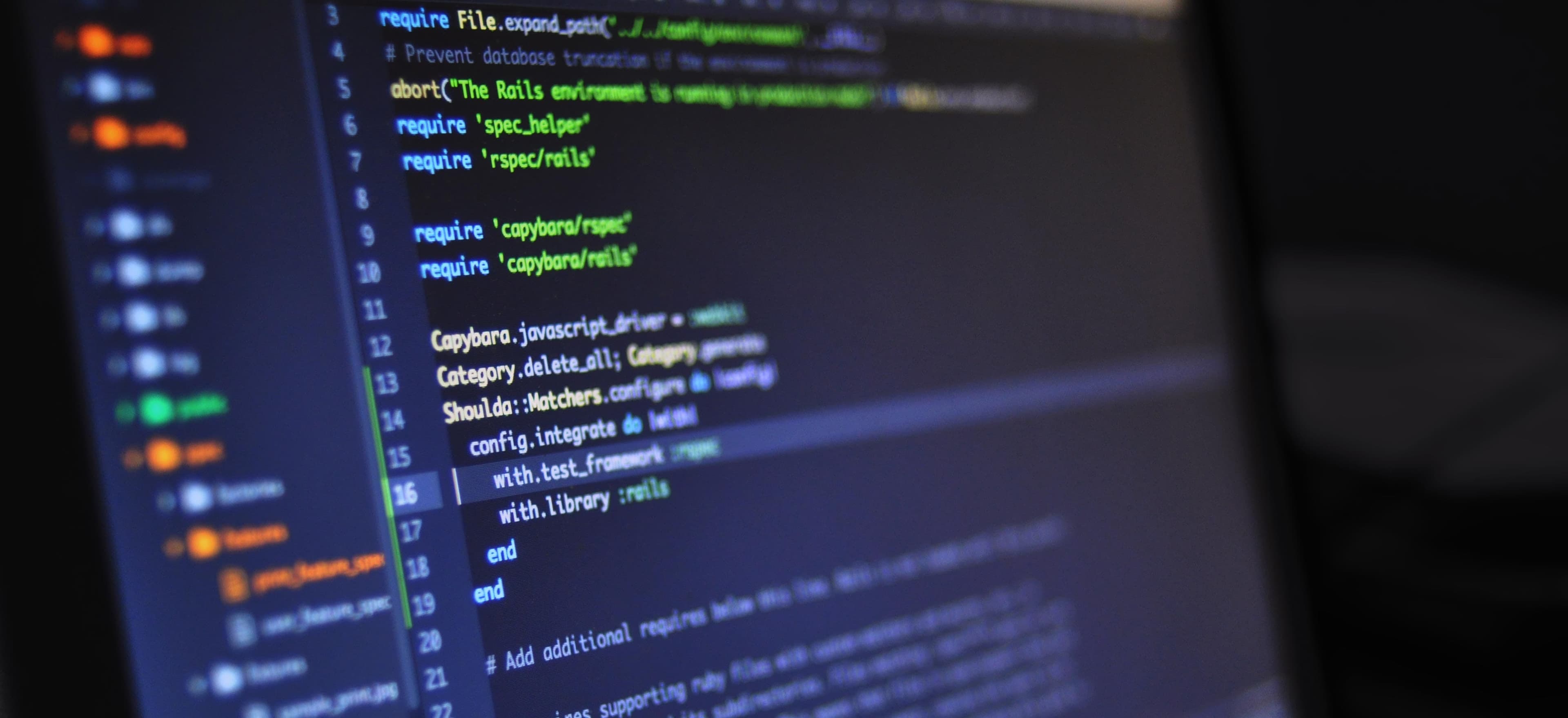
- Published on
Common Command Line Pitfalls When Handling JARs and WARs in Java
Java Archive (JAR) and Web Application Archive (WAR) files are essential components of Java applications, but like any powerful tool, they come with their own set of challenges. This blog post will explore common command line pitfalls developers encounter while working with JARs and WARs, along with best practices to avoid them.
Understanding JAR and WAR Files
Before diving into pitfalls, let’s clarify what JARs and WARs are:
- JAR (Java Archive): A bundle of Java classes, metadata, and resources, packaged together into a single file for distribution.
- WAR (Web Application Archive): A specialized JAR file used for packaging web applications that can be deployed onto a servlet container or application server.
Now, let’s address some common errors, tips, and best practices.
Pitfall #1: Incorrect JAR and WAR File Structure
The Problem:
A common mistake is using the wrong directory structure when creating a JAR or WAR file. This can lead to runtime exceptions or the application failing to start.
The Solution:
Here's a standard structure for a WAR file:
my-webapp/
└── WEB-INF/
├── classes/
│ └── com/
│ └── example/
│ └── MyServlet.class
├── lib/
│ └── some-dependency.jar
└── web.xml
And for a JAR file:
my-library/
├── com/
│ └── example/
│ └── MyLibrary.class
└── META-INF/
└── MANIFEST.MF
Code Snippet: Creating a JAR
When creating a JAR file, ensure you specify the correct paths. Here's how to do it using the command line:
javac -d out src/com/example/MyLibrary.java
cd out
jar cf my-library.jar com/example/MyLibrary.class META-INF/MANIFEST.MF
Why this matters: The -d out
option specifies the output directory where compiled classes will go, preventing structure issues later.Being mindful of structure helps the JVM and web servers locate resources without confusion.
Pitfall #2: Not Including Required Libraries
The Problem:
When packaging a JAR or WAR, it's easy to overlook the required libraries. Forgetting to include dependencies can lead to ClassNotFoundException
during runtime.
The Solution:
Use the -cp
option when running your Java applications to specify additional classpath resources. Also, ensure dependencies are correctly included in the lib
directory for WAR files.
Code Snippet: Running a JAR with Dependencies
When running a JAR file that relies on external libraries, use:
java -cp "my-library.jar:lib/*" com.example.MyLibrary
Why this matters: The -cp
argument specifies all required libraries in your classpath. This ensures that all dependencies are accessible to the Java Runtime Environment, avoiding runtime errors.
Pitfall #3: Failing to Set Execution Permissions on Unix Systems
The Problem:
On Unix-like systems, packaging a JAR file does not automatically make it executable. This could lead to confusion if you try to execute a JAR without the necessary permissions.
The Solution:
After creating a JAR file, update its permissions as follows:
chmod +x my-application.jar
Code Snippet: Running a JAR File
You can run an executable JAR file using:
java -jar my-application.jar
Why this matters: Setting the file as executable allows you to run it directly, enhancing usability for developers transitioning from other platforms.
Pitfall #4: Misconfigured Manifest Files
The Problem:
The manifest file (MANIFEST.MF
) serves crucial roles, such as defining the main class or specifying classpath entries. Misconfiguration can lead to runtime issues.
The Solution:
Ensure your MANIFEST.MF
file is correctly formatted. Here’s an example:
Manifest-Version: 1.0
Main-Class: com.example.Main
Class-Path: lib/some-dependency.jar
Code Snippet: Including a Manifest in Your JAR
You can package your JAR with a specified manifest like this:
jar cfm my-application.jar MANIFEST.MF -C out/ .
Why this matters: A correctly configured manifest enables the JVM to locate the main class and any dependencies, allowing your application to start correctly without additional configuration.
Pitfall #5: Incomplete Cleanup After Deployment
The Problem:
After deploying a WAR file, many developers forget to clean up the target environment. This oversight can lead to version clashes or resource leaks, affecting application performance.
The Solution:
Regularly clean your deployment directories. For example:
rm -rf /path/to/tomcat/webapps/my-webapp*
Code Snippet: Redeploying a WAR File
When redeploying, ensure old versions are cleared:
cp my-webapp.war /path/to/tomcat/webapps/
Why this matters: Keeping deployment environments clean helps avoid conflicts and ensures you're always working with the correct version of your application.
Closing the Chapter
Working with JAR and WAR files via the command line can be straightforward if you avoid common pitfalls. By adhering to recommended practices regarding structure, dependencies, execution permissions, and manifests, you'll find greater efficiency and fewer headaches.
For more information on JAR and WAR management, consider checking the official Java documentation. Happy coding and deploying!