Unlocking a Warm JVM: Speed Up Your Application Startup
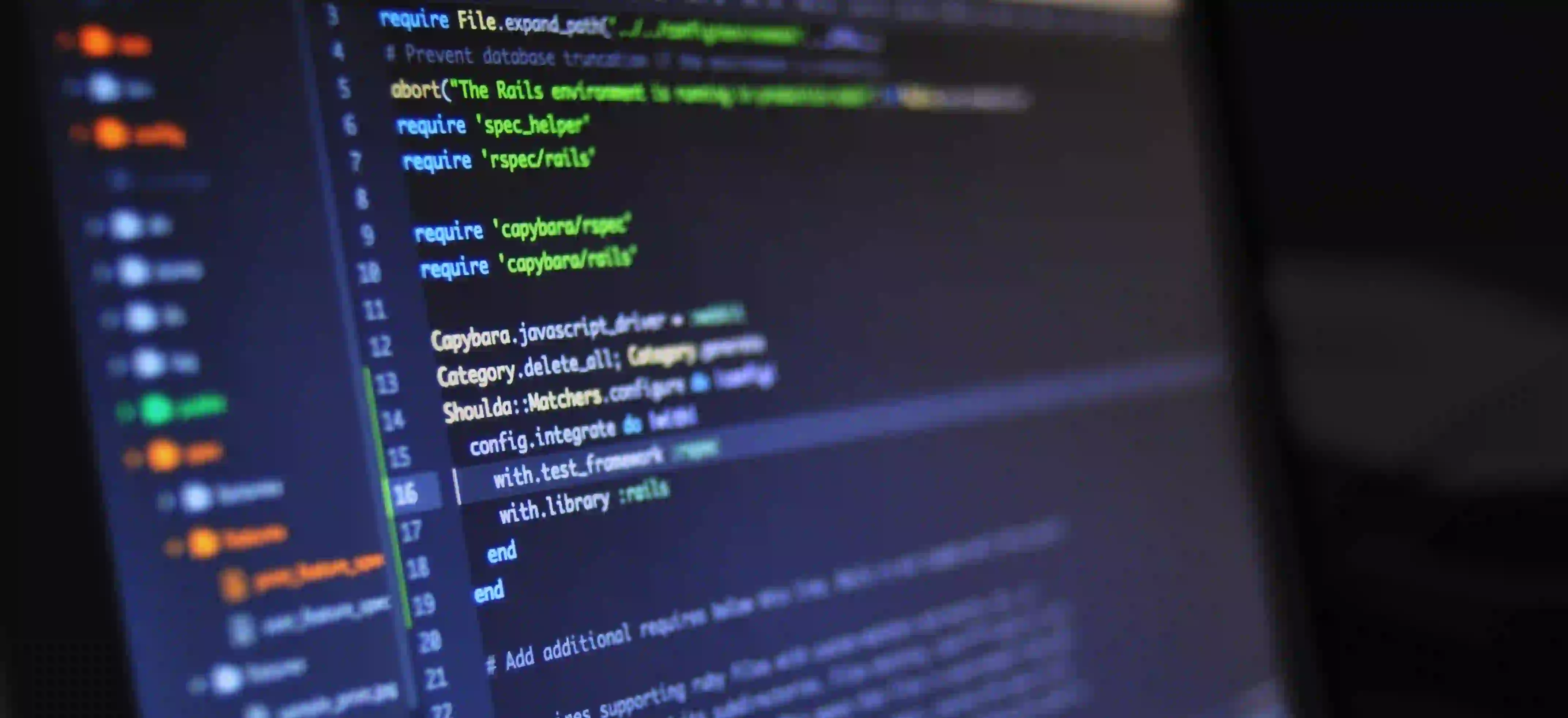
Unlocking a Warm JVM: Speed Up Your Application Startup
Java is a powerful, portable programming language that boasts strong performance, scalability, and ease of use. However, one of the common pain points developers encounter is the slow startup time associated with Java applications. While garbage collection (GC) tuning, JIT compilation, and other performance optimizations come into play during runtime, startup time remains pivotal, especially for microservices and serverless applications where quick deployment is critical.
In this blog post, we will explore the concept of a "warm" JVM and discuss strategies to speed up your application's startup time. By the end, you should have a solid understanding of practical techniques to optimize your Java applications, enhancing both user experience and operational efficiency.
What is a Warm JVM?
A "warm" JVM refers to a Java Virtual Machine that has already loaded and optimized the necessary classes and methods for execution. In contrast, a "cold" JVM is one that has just started up, necessitating the loading of classes, the execution of static initializers, and the compilation of Java bytecode to native code through the Just-In-Time (JIT) compiler.
When the JVM is warm, it can execute code significantly faster since it skips many of the setup steps involved in starting from a cold state. Consequently, the focus should be on strategies that help the JVM jump directly into the "warm" state.
Why Startup Time is Important
The importance of startup time can be summarized as follows:
-
User Experience: In interactive applications, users expect fast responses. Long startup times can frustrate users and lead to disengagement.
-
Scalability: Microservices and serverless architectures require rapid scaling. Quick startups are essential for handling spikes in user demand.
-
Development Efficiency: During development, quick iterations are vital. Reduced startup times lead to shorter debugging cycles and increased productivity.
Strategies to Speed Up Application Startup
Let's dive into some effective methods to warm up your JVM and reduce startup time.
1. Use Ahead-of-Time Compilation (AOT)
Ahead-of-Time (AOT) compilation allows you to compile Java bytecode into native machine code before the application is run. This can significantly reduce startup time as native code can be executed directly without the JIT compilation step.
Using GraalVM, a high-performance runtime that provides AOT capabilities, you can leverage the native-image
tool. Below is an illustrative example of compiling a simple Java application to a native image:
native-image -cp myapp.jar com.example.MyApp
Why AOT? By eliminating the JIT compilation phase, you make it possible for the JVM to start executing your application immediately, resulting in faster startup times. Check out GraalVM's official documentation for more details.
2. Reduce Classpath Scanning
Java applications often utilize third-party libraries, leading to extensive classpath scanning. This can hinder startup performance. To mitigate this, take the following actions:
- Use a minimal set of libraries.
- Avoid including unnecessary packages within your dependencies.
Using the -Xbootclasspath/a
option can help load essential libraries early, bypassing the need to scan unnecessary classes that are not intended for immediate use.
Example Usage:
java -Xbootclasspath/a:myLibrary.jar -jar myApp.jar
Why Reduce Classpath? By limiting the number of classes loaded at startup, you significantly lower initialization time and reduce the time taken by the JVM to prepare the runtime environment.
3. Lazy Initialization
Avoid performing heavy computations and resource allocations at the application startup. Instead, use lazy initialization. With lazy initialization, resources are created only when they are needed.
Here's a simple example that demonstrates lazy initialization for a database connection:
public class DatabaseConnection {
private Connection connection;
public Connection getConnection() {
if (connection == null) {
connection = createNewConnection();
}
return connection;
}
private Connection createNewConnection() {
// Implement logic to create a database connection
}
}
Why Lazy Initialization? This technique conserves resources and reduces startup time by delaying resource-heavy operations until they are absolutely necessary.
4. Use Initialization Hooks (for Spring Boot applications)
If you're working with Spring Boot, leverage the application initialization hooks to delay certain operations until after the application context has been created.
@Component
public class AppStartupRunner implements CommandLineRunner {
@Override
public void run(String... args) throws Exception {
// Perform startup logic here
System.out.println("Application is starting...");
}
}
Why Use Initialization Hooks? By managing the initialization logic smartly, you can defer operations that are not crucial for the initial startup, allowing your application to be ready to respond to user requests more quickly.
5. JVM Options and Flags
Utilizing specific JVM options can lead to performance improvements during application startup. Some relevant flags include:
-Xms
and-Xmx
: These flags set the initial and maximum heap size. Set them to appropriate values to avoid resizing during startup.-XX:+UseCompressedOops
: Enables compressed pointers, which can save memory and lead to faster start times.
Example:
java -Xms256m -Xmx512m -XX:+UseCompressedOops -jar myApp.jar
Why JVM Options? Tuning these options can lead to enhancements in functional memory allocation, reducing the time taken during the startup phase.
6. Profile and Optimize Hot Code Paths
Use profiling tools to identify slow parts in your code that significantly impact startup times. You can use Java profilers like VisualVM, JProfiler, or YourKit to visualize this information.
Once you identify hotspots, focus on optimizing these methods or restructuring your codebase to speed up the startup process.
Why Profile? Profiling allows you to see, in real-time, where your application is lagging. Targeted optimizations can drastically improve startup time when you address the right parts of your code.
7. Explore Java Modules
Java 9 introduced the module system (Project Jigsaw) to help developers encapsulate packages. Using modules allows you to load only the necessary parts of your application, minimizing the startup overhead for applications comprised of numerous libraries.
To create a module, define it with a module-info.java
file:
module com.example.myapp {
requires java.logging;
exports com.example.myapp;
}
Why Use Modules? By isolating dependencies and minimizing the package footprint, you can load only what’s necessary during startup. This ensures a leaner application and faster loading times.
The Closing Argument
Optimizing application startup time is a multifaceted challenge that requires careful consideration of architecture, coding practices, and JVM settings. By implementing AOT compilation, reducing classpath, using lazy initialization, and tuning JVM options, you can significantly enhance startup performance.
As technology continues to evolve with solutions like GraalVM and more robust JVM tuning parameters, developers have many opportunities to improve Java application performance. Remember that each application is unique, and profiling your specific use cases is crucial for identifying the right optimizations.
For additional insight, consider exploring the following resources:
By unlocking the secrets to a warm JVM, you're not just enhancing performance — you're paving the way for a more seamless user experience that can handle the demands of today's fast-paced digital landscape. Happy coding!