Unlocking the Power of Data Over Database Management
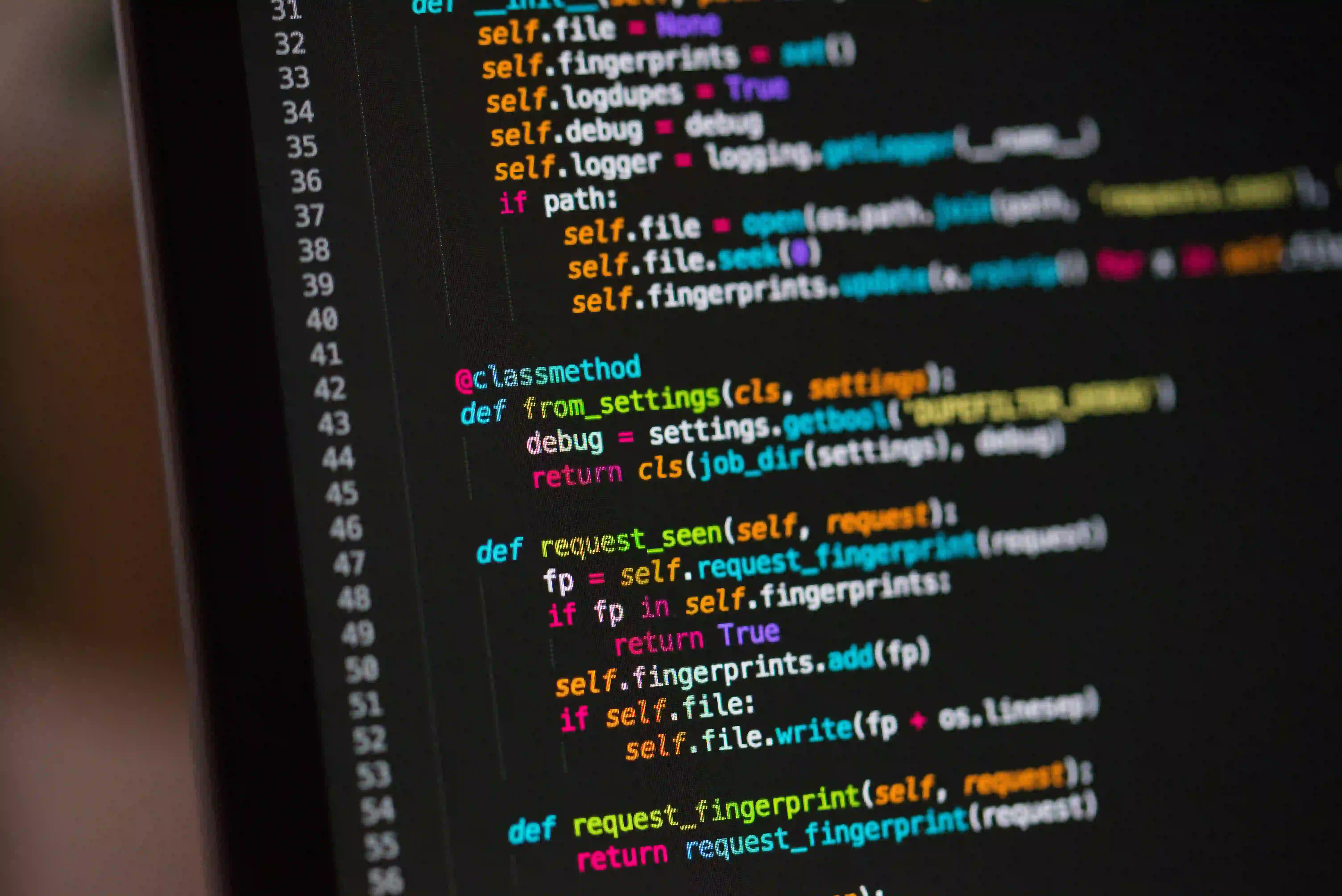
Unlocking the Power of Data Over Database Management
In today's digital ecosystem, businesses generate an immense amount of data every second. The way organizations capture, manage, and leverage this data can significantly influence their success. Enter Data Over Database Management, or DoD. This concept transcends traditional database management by focusing on the actual value derived from data rather than just storing it.
Understanding Data Over Database Management
Data Over Database Management is a paradigm shift. Instead of merely managing databases, organizations are learning to harness the power of data itself. This approach prioritizes data analytics, data quality, and data accessibility over the structures and systems used to manage databases.
The reason for this shift? Traditional database management does not provide the flexibility and insights that today’s businesses need. In a world where real-time data analysis can lead to informed decision-making, organizations must think beyond the confines of their databases.
The Value of Data
Why is data so valuable? Here are a few reasons:
- Decision Making: Data-driven decisions are often more robust and reliable than gut feelings or intuition.
- Customer Insights: Understanding customer behavior helps businesses tailor their offerings more effectively.
- Trend Analysis: Real-time data can identify trends that help companies stay ahead of the competition.
Focusing on Data Quality
When shifting to a DoD model, the focus on data quality cannot be overstated. Quality data ensures accurate analysis. If the raw data is filled with inaccuracies, the insights derived from it will be misleading.
Here is a simple Java code snippet demonstrating how to check for null or empty values in your data:
public class DataValidator {
public static boolean isValidData(String data) {
return data != null && !data.trim().isEmpty();
}
public static void main(String[] args) {
String testData = " ";
if (isValidData(testData)) {
System.out.println("Data is valid.");
} else {
System.out.println("Data is invalid.");
}
}
}
Explanation of the Code:
- Why Validate Data?: Validating data ensures quality and relevancy before using it in decision-making processes. In this example, the method
isValidData
checks for null or empty strings, confirming that only meaningful data is considered.
Utilizing Data Analytics
Data analytics converts raw data into meaningful insights. By implementing analytics tools, organizations can derive actionable intelligence from their data.
One popular library for data processing in Java is Apache Spark. It allows you to process large datasets quickly and efficiently. This can be illustrated with the following sample code:
import org.apache.spark.api.java.JavaRDD;
import org.apache.spark.api.java.JavaSparkContext;
import org.apache.spark.SparkConf;
public class DataAnalyzer {
public static void main(String[] args) {
SparkConf conf = new SparkConf().setAppName("Data Analyzer").setMaster("local");
JavaSparkContext sc = new JavaSparkContext(conf);
JavaRDD<Integer> data = sc.parallelize(Arrays.asList(1, 2, 3, 4, 5));
int sum = data.reduce((x, y) -> x + y);
System.out.println("The sum of data is: " + sum);
sc.close();
}
}
Explanation of the Code:
- What is Apache Spark?: Spark is a powerful big data processing tool that enables fast computations.
- Why Use It?: The
reduce
method takes a collection of integers and returns their sum, demonstrating how simple operations can yield rich insights. The scalability of Spark makes it suitable for enterprises dealing with big data.
Making Data Accessible
Accessibility is a core principle of Data Over Database Management. Organizations need to ensure that relevant stakeholders have access to meaningful data in real time. This can be achieved through cloud solutions and APIs.
Example of Building a Simple REST API in Java
To make your data accessible, you can create a RESTful service using Spring Boot. This enables stakeholders to retrieve data through web requests. Here’s a minimal setup for a REST API:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@SpringBootApplication
public class DataServiceApplication {
public static void main(String[] args) {
SpringApplication.run(DataServiceApplication.class, args);
}
}
@RestController
class DataController {
@GetMapping("/data")
public String getData() {
return "Here is your data!";
}
}
Explanation of the Code:
- Why Use Spring Boot?: It simplifies the setup of Spring applications and allows you to create stand-alone, production-grade applications easily.
- REST API Endpoint: The
@GetMapping
annotation sets up a simple endpoint that returns a string when accessed. This allows users to access data over HTTP easily.
The Role of Machine Learning
Incorporating machine learning into your data strategy can further enhance the power of data. Machine learning algorithms can analyze large datasets to uncover patterns and make predictions.
A Simple Machine Learning Example in Java
Utilizing the Weka library, one can implement a basic classification model:
import weka.classifiers.trees.J48;
import weka.core.Instances;
import weka.core.SerializationHelper;
public class DecisionTree {
public void buildModel(Instances trainingData) throws Exception {
J48 tree = new J48();
tree.buildClassifier(trainingData);
SerializationHelper.write("decisionTree.model", tree);
}
}
Explanation of the Code:
- What is Weka?: Weka is a popular suite of machine learning software written in Java.
- Why Build a Model?: The
J48
class creates a decision tree, which is a valuable tool for classification tasks. This shows how data can be used for predictive analysis.
Data Governance
Shifting the focus to Data Over Database Management also necessitates stringent data governance practices. It becomes imperative for organizations to establish policies concerning data management, quality control, and compliance with regulations such as GDPR.
Key Elements of Data Governance:
- Data Stewardship: Assign roles to ensure data is managed appropriately.
- Data Quality Metrics: Develop metrics to assess data quality continually.
- Security Protocols: Ensure data is secure, protecting it from unauthorized access.
In Conclusion, Here is What Matters
Embracing Data Over Database Management is a strategic move for organizations navigating today’s data-driven landscape. By prioritizing data quality, accessibility, analytics, and governance, businesses can leverage their data to derive actionable insights that drive success.
By implementing the practices discussed in this article, such as utilizing Java tools like Apache Spark and Spring Boot, your organization can unlock the true power of data. As you move forward, remember that the ultimate aim is not just to store data, but to transform it into meaningful, actionable insights for informed decision-making.
For further reading, check out Data Science for Business and Understanding Data Governance. These resources provide an in-depth exploration of how data governance and analytics fit into your overall data strategy.