Why Your Production Profiling is Failing and How to Fix It
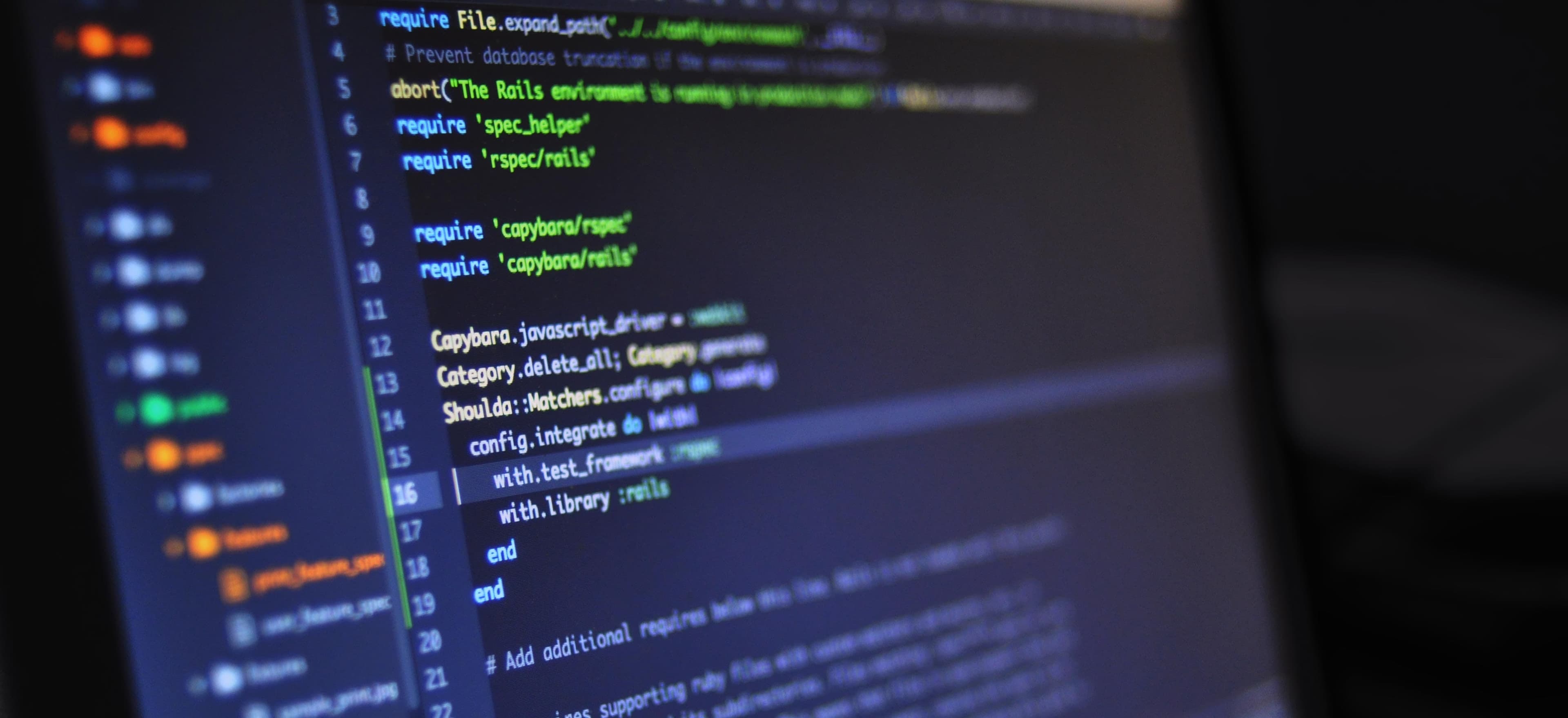
- Published on
Why Your Production Profiling is Failing and How to Fix It
In the fast-paced world of software development, performance profiling is often overlooked, yet it should not be. Profiling helps identify bottlenecks in your application, allowing for efficient resource utilization and improved user experience. However, many developers encounter difficulties in effectively profiling production systems. In this blog post, we will discuss why your production profiling might be failing and provide actionable insights to overcome these challenges.
Understanding Production Profiling
What is Production Profiling?
Production profiling involves analyzing the performance of an application while it's running in a live environment. This analysis is crucial because performance bottlenecks can differ significantly between development and production due to variations in load, user behavior, and operational constraints.
Why Conduct Production Profiling?
- Identify Bottlenecks: It helps in pinpointing issues that bottleneck the performance.
- Resource Allocation: Allows optimal resource utilization, ensuring that servers can handle requests efficiently.
- User Experience: Enhances overall user experience by reducing response times and improving application reliability.
Common Pitfalls in Production Profiling
Despite its advantages, there are several reasons why production profiling fails. Let's examine these once-by-once.
1. Lack of Realistic Testing Scenarios
One of the primary reasons profiling fails is due to inadequate simulation of real-world usage. Developers often depend on simulated environments that do not reflect actual usage conditions, leading to misleading profiling outputs.
How to Fix:
Implement load testing tools in pre-production to simulate real-world scenarios. Tools like JMeter or Gatling can help recreate user behaviors.
// Example JMeter configuration
ThreadGroup threadGroup = new ThreadGroup();
threadGroup.setName("User Load");
threadGroup.setNumberOfThreads(100); // Simulating 100 users
// Add the Thread Group to the Test Plan
TestPlan testPlan = new TestPlan();
testPlan.addThreadGroup(threadGroup);
2. Insufficient Monitoring and Instrumentation
Without proper monitoring, it can be difficult to ascertain where the performance issues lie. Many applications lack sufficient instrumentation, making it challenging to gather actionable data.
How to Fix:
Incorporate application performance monitoring (APM) tools like New Relic or Grafana. These tools provide valuable insights into application performance and resource utilization.
Example:
Using Micrometer with Spring Boot can help in setting up monitoring:
import io.micrometer.core.instrument.MeterRegistry;
@RestController
public class MetricsController {
private final MeterRegistry meterRegistry;
public MetricsController(MeterRegistry meterRegistry) {
this.meterRegistry = meterRegistry;
}
@GetMapping("/api/data")
public ResponseEntity<?> getData() {
meterRegistry.counter("api.calls").increment();
return ResponseEntity.ok("Data");
}
}
By recording API call metrics, you can identify which endpoints are frequently accessed and which ones may need optimization.
3. Ignoring External Dependencies
External systems, like databases or microservices, may contribute to latency. Yet, many developers focus solely on their own code without assessing how these dependencies impact application performance.
How to Fix:
Utilize profiling tools that can monitor external calls, such as DependencyTrack or Zipkin. Here’s a simple example with Spring Boot for tracking external service calls:
import org.springframework.web.client.RestTemplate;
import io.micrometer.core.annotation.Timed;
@Service
public class ExternalService {
private final RestTemplate restTemplate;
public ExternalService(RestTemplate restTemplate) {
this.restTemplate = restTemplate;
}
@Timed(value = "external.service.call.time")
public String callExternalService(String url) {
return restTemplate.getForObject(url, String.class);
}
}
4. Not Capturing Enough Data
Profiling might fail if you're not capturing enough relevant data during runtime. Focusing solely on CPU and memory usage can hide deeper issues.
How to Fix:
Expand the metrics scope to gather more data points, such as:
- Response times
- Database query performance
- Third-party service latency
This comprehensive approach will give better insights into overall application performance. For databases, consider using SQL query profiling to analyze slow queries.
5. Ineffective Communication Among Teams
Production profiling is often a team effort. Lack of communication between development, operations, and QA teams can lead to missed opportunities for identifying and resolving performance issues.
How to Fix:
Foster a culture of collaboration. Use tools such as Slack or Microsoft Teams for better communication. Hold regular performance meetings to discuss profiling results and actionable insights.
Best Practices for Effective Production Profiling
1. Automate Monitoring
Set up automatic monitoring whenever it is feasible. Automation allows for continuous data collection without manual intervention.
2. Continuous Improvement
Allow profiling and monitoring to be part of your continuous integration and continuous deployment (CI/CD) pipelines. This ensures performance issues are caught before they reach production.
3. Regularly Review and Optimize
Profoundly analyze profiling data on a regular basis, and prioritize optimizations that will yield the maximum impact.
4. Establish a Baseline
Establish baseline performance metrics to compare against during profiling. This will help you quickly identify deviations that could indicate problems.
Closing the Chapter
In summary, effective production profiling is essential for maintaining a high-performing application. By recognizing the common pitfalls such as unrealistic testing scenarios, insufficient monitoring, external dependencies, lack of data collection, and ineffective team communication, you can enhance your profiling processes. Implement the best practices we discussed to ensure continuous monitoring and improvement.
For further reading on performance optimization, check out the extensive Java Performance Tuning Guide and Spring’s official documentation on Metrics. Take the time to refine your approach to profiling, and watch as your applications reach new heights.
Feel free to leave any questions or observations in the comments below! Happy coding!