Common Pitfalls When Mapping Java Enums to PostgreSQL
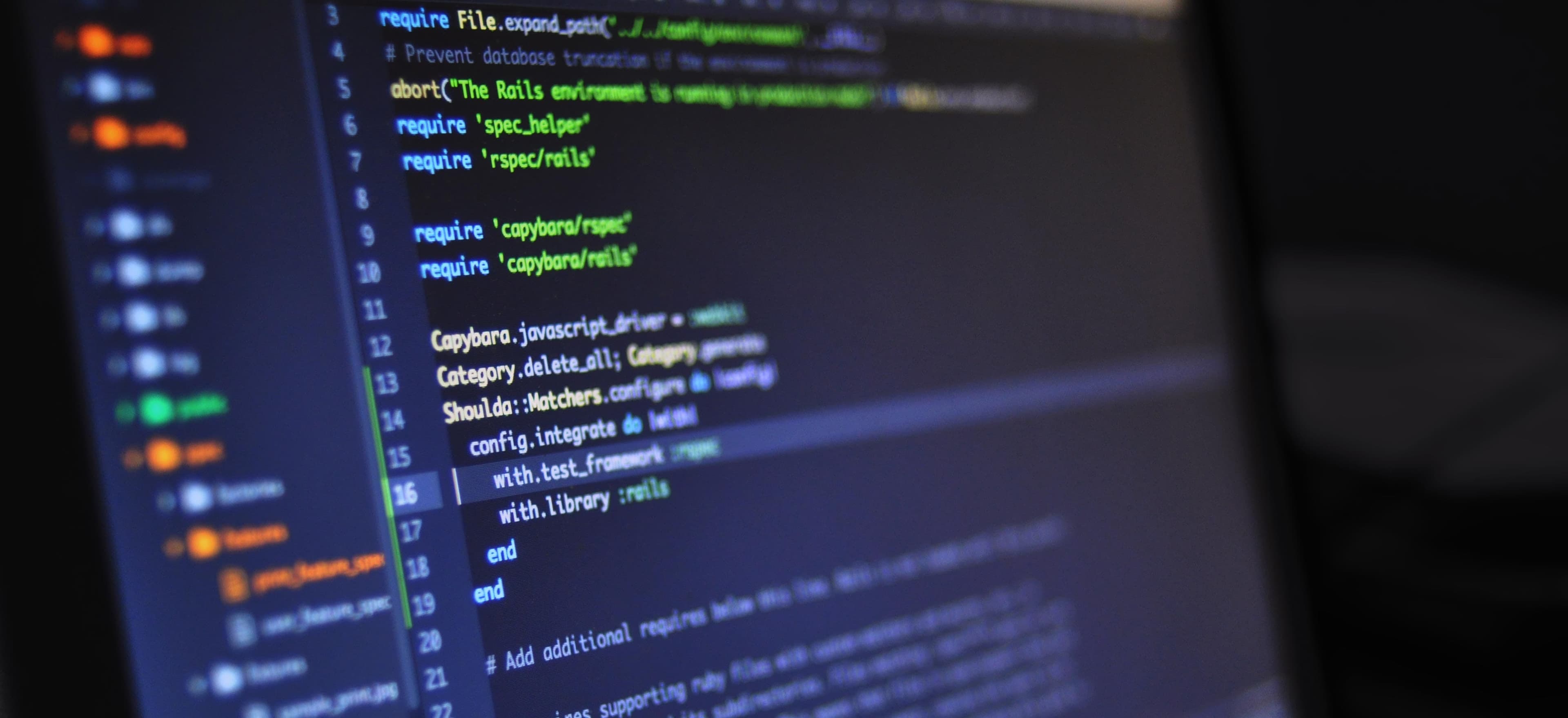
- Published on
Common Pitfalls When Mapping Java Enums to PostgreSQL
In the world of application development, efficient data handling is paramount, especially when you're working with databases. One area that often presents challenges is the mapping of Java Enums to PostgreSQL. While Enums in Java add type safety and improve code readability, their integration with PostgreSQL can lead to various pitfalls if not handled properly. This blog post will explore these common pitfalls and how to avoid them.
Understanding Java Enums
Java Enums are a special data type that represents a group of constants. They enable you to define a variable that can hold a set of predefined constants, which improves code clarity and safety:
public enum OrderStatus {
PENDING,
SHIPPED,
DELIVERED,
CANCELED;
}
In the example above, we have defined an Enum named OrderStatus
with four constants. The benefit here is the ability to use OrderStatus
data type for any variable that needs to represent an order's status.
The PostgreSQL Enum Type
PostgreSQL has a native Enum type that provides a similar functionality. However, Java Enums and PostgreSQL Enums do not directly match. In Java, the Enum constant is a reference type, while PostgreSQL treats Enum as a database type.
To define an Enum in PostgreSQL, you can run the following SQL command:
CREATE TYPE order_status AS ENUM ('pending', 'shipped', 'delivered', 'canceled');
Pitfall 1: Mismatched Naming Conventions
Sometimes, developers might forget to maintain consistency in naming conventions between Java Enums and PostgreSQL Enum types. For example, in the OrderStatus
Enum, you might have constants that are UPPER_SNAKE_CASE
. However, if the corresponding PostgreSQL Enum type uses a different format (like lower_case), you have a mismatch.
How to Avoid This:
- Stick to a consistent naming convention. Either use all uppercase letters with underscores in both Java and SQL or a camel case approach to ensure uniformity.
CREATE TYPE order_status AS ENUM ('PENDING', 'SHIPPED', 'DELIVERED', 'CANCELED');
Pitfall 2: Using Java Enum Values Instead of String
When persisting Java Enums into the database, the common approach is to use the enum’s string name. Using the Java Enum values directly can lead to issues. For instance, consider the following code to save an Enum value:
OrderStatus status = OrderStatus.SHIPPED;
preparedStatement.setString(1, status.name());
This snippet is effective, but if the PostgreSQL ENUM is defined differently, it may not map correctly—leading to data inconsistency or unexpected crashes.
How to Avoid This:
- Always convert your Java Enum into a string format that directly maps to the PostgreSQL Enum. You can also create a method inside your Enum class to handle this:
public String toPostgresValue() {
return name().toLowerCase();
}
// Usage
preparedStatement.setString(1, status.toPostgresValue());
Pitfall 3: Enum Value Changes
Adding, removing, or renaming Enum constants in Java can lead to significant issues if the corresponding PostgreSQL Enum type is not updated. For instance, removing an enum constant called CANCELED
from OrderStatus
would lead to data inconsistencies.
How to Avoid This:
- Always keep in sync with the database when making changes to Java Enums.
- Use migration tools like Flyway or Liquibase to manage your database changes systematically.
Pitfall 4: Using JPA without Custom Converter
If you use an ORM framework like JPA (Java Persistence API), one common mistake is failing to map Java Enums to PostgreSQL Enums via a custom converter. By default, JPA might persist the Enum as an integer rather than as a string or reference it to a Java Enum.
How to Avoid This:
- Implement a custom converter by annotating your Enum in the entity class. Here’s how to do it:
import javax.persistence.AttributeConverter;
import javax.persistence.Converter;
@Converter(autoApply = true)
public class OrderStatusConverter implements AttributeConverter<OrderStatus, String> {
@Override
public String convertToDatabaseColumn(OrderStatus orderStatus) {
return orderStatus == null ? null : orderStatus.toPostgresValue();
}
@Override
public OrderStatus convertToEntityAttribute(String dbData) {
return dbData == null ? null : OrderStatus.valueOf(dbData.toUpperCase());
}
}
This ensures that your Enum is appropriately converted to the required database format and retrieves the appropriate Enum from the database without hassle.
Pitfall 5: PostgreSQL Version Compatibility
PostgreSQL has undergone several updates. Developers may work with different versions, which sometimes leads to unexpected behavior with Enums. Features introduced in later versions may not function the same way in older ones, causing discrepancies and bugs.
How to Avoid This:
- Make sure to test your application across different PostgreSQL versions if you expect to support multiple environments. Keep an eye on the PostgreSQL documentation for the version you are using to grasp potential Enum behaviors.
Closing Remarks
Mapping Java Enums to PostgreSQL can undoubtedly be tricky due to the differences in type handling and representation. The pitfalls discussed in this blog post—ranging from mismatched naming conventions to database compatibility—highlight the essential practices that can help mitigate potential issues.
Utilizing well-defined strategies, such as using custom converters, ensuring naming conventions are consistent, and keeping both systems (Java and PostgreSQL) synchronized, can significantly enhance the integrity and reliability of your application’s data handling.
To further explore Java Enums and PostgreSQL data handling, you might find the following resources useful:
By keeping these considerations in mind, you can seamlessly work with Java Enums and PostgreSQL, ensuring your application remains robust and efficient. Happy coding!
Checkout our other articles