Why Ignoring Trust Boundaries Can Derail Your Product
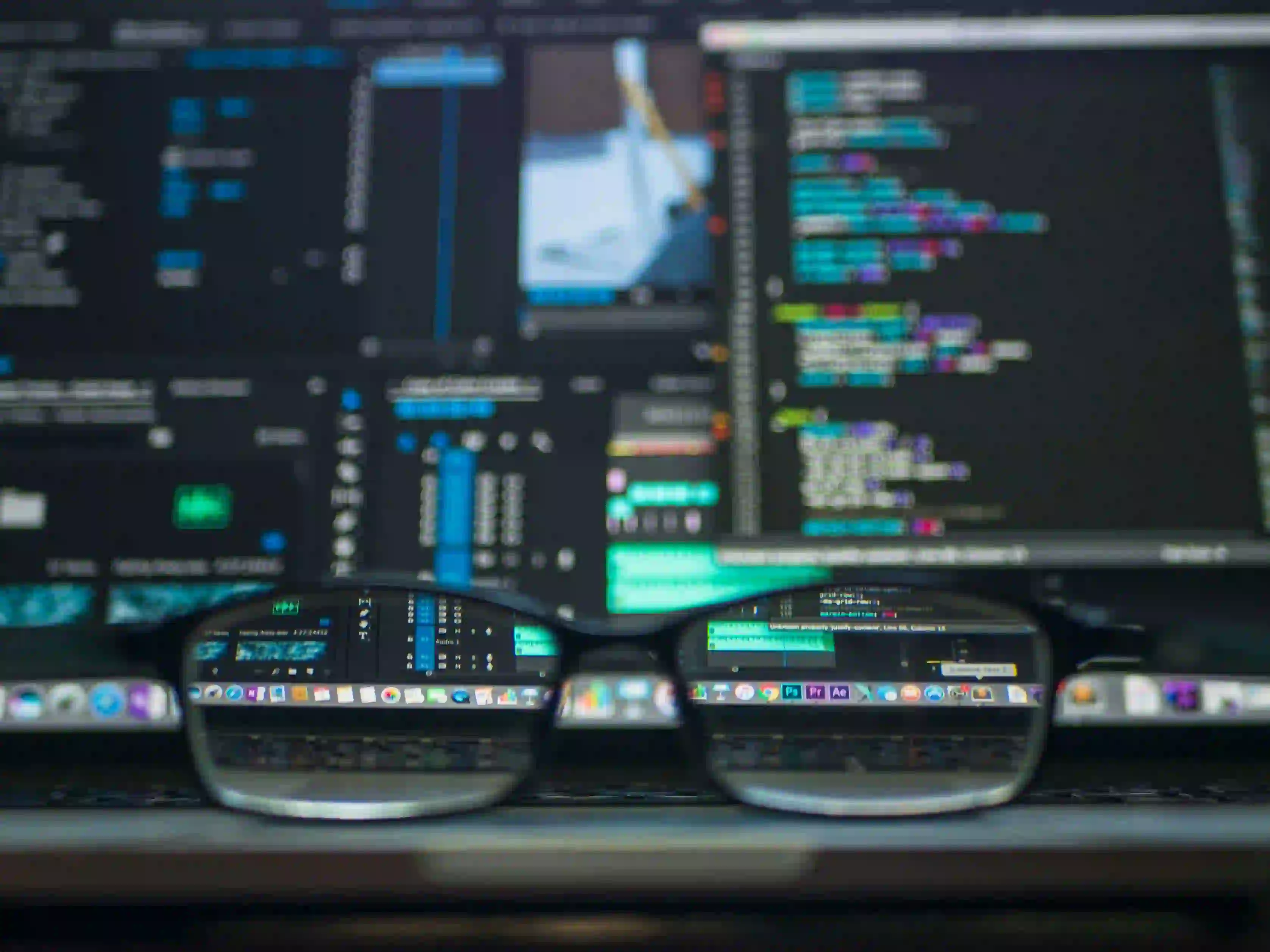
Why Ignoring Trust Boundaries Can Derail Your Product
In the realm of software development, the concept of trust boundaries is pivotal but often overlooked. Trust boundaries define the limits within which certain assumptions can be made regarding data and user actions. They specify the level of trust you can place in the systems or components interacting with each other. Ignoring these boundaries can lead to security vulnerabilities, data breaches, and ultimately, the failure of your product. In this blog post, we will delve deep into the significance of trust boundaries and provide actionable insights to ensure you never fall prey to the pitfalls of neglecting them.
What Are Trust Boundaries?
Trust boundaries are the points in your application architecture where the trust level changes. For example, data moving from a user interface (UI) to a backend server is subject to a trust boundary. The UI is generally more vulnerable to manipulation than the server, where data validation and security protocols should be more stringent.
A clear understanding of these boundaries is crucial. If you treat all components in your application with the same level of trust, you expose yourself to risks.
Why Trust Boundaries Matter
-
Security: Trust boundaries help identify where security measures must be tighter. When user input reaches your backend, it must be validated and sanitized—since users can easily manipulate data sent from the UI.
-
Reliability: Systems that operate across different trust zones require robust checks. If a reliable system isn’t implemented, it can lead to unexpected failures and degraded user experience.
-
Scalability: As your product scales, so does its complexity. Trust boundaries ensure that components are independently tested, allowing for a scalable architecture that doesn’t compromise security.
-
Maintainability: Clear trust boundaries foster better documentation and understanding amongst team members, making the system easier to maintain over time.
How to Define Trust Boundaries in Your Application
1. Identify Components and Data Flow
The first step in managing trust boundaries is to pinpoint where data is flowing in your application. Understanding how components interact and their roles is pivotal.
Code Example
class UserInput {
private String data;
public void setData(String input) {
this.data = input;
}
public String getData() {
return data;
}
}
This simplistic UserInput
class demonstrates a basic encapsulation. However, it is crucial to enforce validations to ensure any data processed via this component meets security standards.
2. Define Trust Levels
Once you've identified the components and their interactions, classify them based on their trust levels:
- Trusted: Back-end services and databases.
- Untrusted: User behavior on the front-end and incoming network data.
Discussing Trust Levels
Why this matters is quite clear: trusted components should handle data differently compared to untrusted ones. For instance, while user input needs thorough validation, backend services may only process data after ensuring its integrity.
3. Implement Data Validation and Sanitization
Data validation is your first line of defense against threats. Always sanitize user input and validate the data before it enters your trusted zones.
Code Example
class DataHandler {
public void processUserData(UserInput userInput) {
String userInputData = userInput.getData();
if (isValid(userInputData)) {
// Process the valid data
} else {
// Log and reject invalid data
}
}
private boolean isValid(String data) {
return data != null && data.matches("[a-zA-Z0-9 ]*"); // Example validation
}
}
In the above snippet, the isValid
method checks if the user input is safe before processing it. This validation serves as a practical defense against attacks like SQL injection or cross-site scripting (XSS).
4. Monitor and Audit Interactions Across Boundaries
Whether it’s logging suspicious activities or auditing data transactions, maintaining a clear record of what crosses trust boundaries can provide insights into potential vulnerabilities.
Code Example
import java.util.logging.Logger;
class SecureDataHandler {
private static final Logger logger = Logger.getLogger(SecureDataHandler.class.getName());
public void processUserData(UserInput userInput) {
String userInputData = userInput.getData();
if (isValid(userInputData)) {
logger.info("Valid data received: " + userInputData);
// Process the valid data
} else {
logger.warning("Invalid data received: " + userInputData);
}
}
// isValid method remains unchanged
}
Here, we have introduced logging to keep track of user input. This practice helps significantly in auditing and monitoring the trust boundary’s activities.
Common Mistakes When Overlooking Trust Boundaries
-
Underestimating User Input: Many developers assume user input is harmless, leading to security gaps.
-
Single-Level Trust: Treating all interactions as equally trustworthy often results in unintended vulnerabilities.
-
Ignoring External Libraries: Relying on libraries without scrutinizing their interaction with your system can result in weaknesses.
-
Skipping Reviews: Regular code reviews focusing on trust boundaries help catch issues at an early stage.
In Conclusion, Here is What Matters
Ignoring trust boundaries can prove detrimental to your product. Understanding and implementing effective trust boundary management ensures a robust, secure, and maintainable application. By defining your system's trust levels, enforcing data validation, and continuously monitoring interactions, you're not just protecting your product, but also your users.
For more information on secure coding practices, consider visiting OWASP and exploring their vast resources on web application security.
Finally, embrace the concept of defense in depth—and make trust boundaries a cornerstone of your development process. The moment you decide to ignore them is the moment your product's integrity and success comes under threat.
By being proactive about trust boundaries, you’re ensuring that your product remains not just functional, but secure and resilient in an ever-evolving digital landscape.