Mastering Generic Types for Cleaner Java API Responses
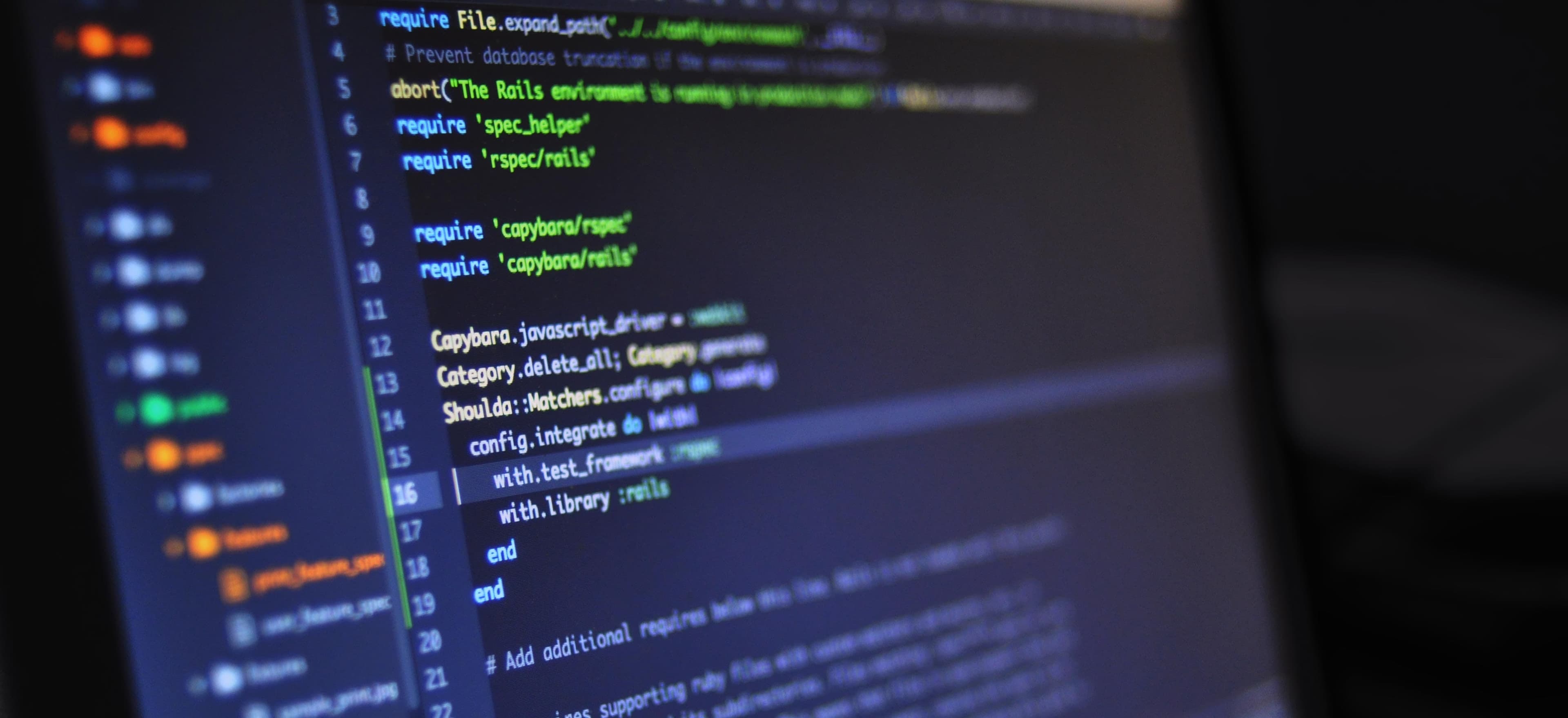
- Published on
Mastering Generic Types for Cleaner Java API Responses
When it comes to Java development, especially when working with APIs, clarity and reusability of code are crucial. Generic types allow us to achieve that by creating flexible and reusable code templates. In this post, we will dive deep into mastering generic types to enhance our Java API responses, ensuring that your code is not only cleaner but also more maintainable.
What Are Generic Types?
Generic types are a powerful feature in Java that allow you to define classes, interfaces, and methods with a placeholder for the type of data they operate on. This enables you to implement algorithms and data structures in a type-safe manner without sacrificing performance.
For instance, when building a simple API, you might want to return a list of user objects. Instead of specifying a concrete type, you can leverage generics to create a method that can handle various data types.
Basic Syntax of Generics
Here’s the basic syntax for defining a generic class:
public class Box<T> {
private T item;
public void set(T item) {
this.item = item;
}
public T get() {
return item;
}
}
In this example, Box<T>
is a generic class. The type parameter <T>
enables the Box
class to hold any type of object. This is the foundation of using generics effectively in your code.
Why Use Generics?
- Type Safety: It ensures that you always deal with the right type of objects which helps you catch errors at compile time.
- Code Reusability: You can create methods and classes that operate on various data types, reducing redundant code.
- Cleaner Code: Generics can help to avoid typecasting and generics make code more readable.
The Role of Generics in API Responses
As APIs often need to handle differing data types, generics are essential in crafting cleaner and more manageable API responses. When you define your API methods to return generic types, they become more versatile and easier to maintain.
Example: A Generic API Response Wrapper
Often, API responses include additional metadata, like success status or error messages, alongside the actual data. A common practice is to use a generic response wrapper. Here's a simple implementation:
public class ApiResponse<T> {
private String message;
private boolean success;
private T data;
public ApiResponse(String message, boolean success, T data) {
this.message = message;
this.success = success;
this.data = data;
}
public String getMessage() {
return message;
}
public boolean isSuccess() {
return success;
}
public T getData() {
return data;
}
}
How to Use Generic API Response
Now, imagine we have a user and product service. Here's how we might utilize our ApiResponse
class:
@RestController
@RequestMapping("/api")
public class UserController {
@GetMapping("/user/{id}")
public ApiResponse<User> getUser(@PathVariable String id) {
User user = userService.findUserById(id);
if (user == null) {
return new ApiResponse<>("User not found", false, null);
}
return new ApiResponse<>("User found", true, user);
}
}
Explanation
- Creating Flexibility: The
ApiResponse<T>
allows the return type to vary based on what is being requested. In this case, for thegetUser
method, we returnApiResponse<User>
. - Maintaining Consistency: Regardless of the endpoint accessed, the structure of the response remains consistent, making it easier for clients to handle API responses without unnecessary complexity.
For further reading on handling generic types and structuring API responses, check out Struggling with Generic Types in API Responses? Here's Help!.
Handling Collections with Generics
When returning lists or collections, generics ensure that our API stays type-safe. Here’s how to return a list of users:
@GetMapping("/users")
public ApiResponse<List<User>> getAllUsers() {
List<User> users = userService.findAllUsers();
return new ApiResponse<>("Users retrieved", true, users);
}
In this case, ApiResponse<List<User>>
allows clients to receive a list of users while keeping the response’s integrity intact. Each entry is guaranteed to be of type User
, reinforcing the benefits of type safety.
Best Practices for Using Generics
-
Favor Specificity: Specify your types correctly to maximize clarity. Using raw types can lead to confusing code.
-
Avoid Unnecessary Complexity: Keep your generic types simple. Overuse of generics can make your code complicated and harder to read.
-
Use Bounded Type Parameters: If your method expects certain capabilities from a type, consider using bounded type parameters:
public <T extends Number> void processNumbers(List<T> numbers) {
// Process numbers knowing they are of type Number or its subclasses
}
- Document Your Code: Always provide clear documentation for your generic types, as they may not be immediately intuitive to others reading or using your code.
My Closing Thoughts on the Matter
Mastering generic types in Java can significantly streamline the development of clean, reliable API responses. By using generics effectively, you create code that is not only reusable and easy to maintain but also enhances type safety.
Remember, the next time you're designing an API, think about incorporating generics into your response structures. This small shift can lead to cleaner code and a better developer experience.
For more insights on working with generic types in API responses, visit the article Struggling with Generic Types in API Responses? Here's Help!. Happy coding!
Checkout our other articles