Java Frameworks for Building Dynamic Expand/Collapse Menus
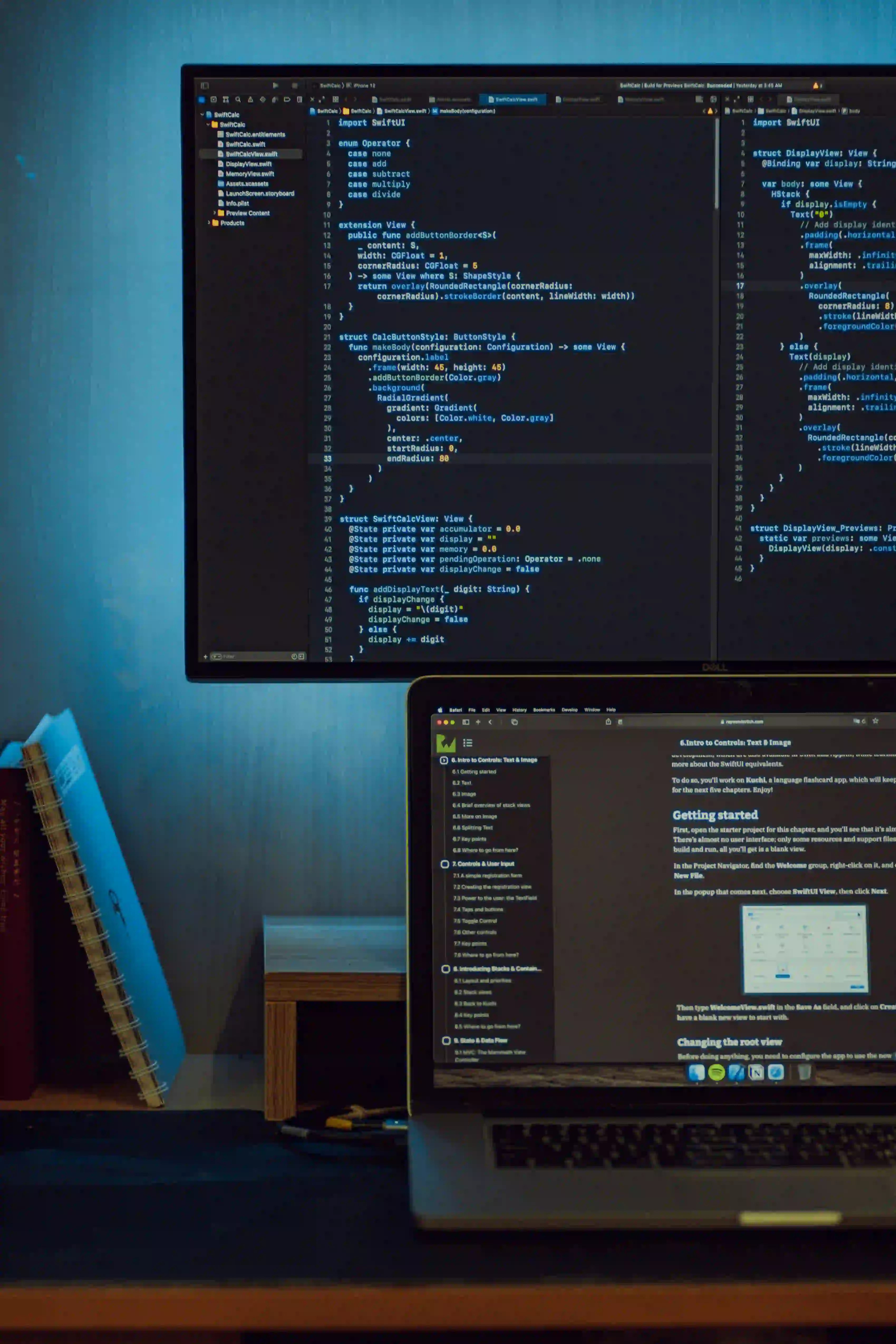
Java Frameworks for Building Dynamic Expand/Collapse Menus
In web development, user interface (UI) design plays a crucial role in providing an engaging experience. A clean and interactive UI keeps users on the site longer, enhancing usability and functionality. One common pattern in modern web applications is the expand/collapse menu. This dynamic mechanism helps manage space and group content efficiently.
In this blog post, we'll explore popular Java frameworks ideal for building such menus dynamically. We will also provide insights and examples that highlight not only how to implement these menus but also the reasons behind the coding practices.
Table of Contents
- Understanding Expand/Collapse Menus
- Java Frameworks for Dynamic Menus
- Spring Boot
- JavaServer Faces (JSF)
- Vaadin
- Sample Implementation
- Best Practices
- Conclusion
- References
Understanding Expand/Collapse Menus
An expand/collapse menu allows users to hide or show additional content, which is especially useful in navigation menus or content-heavy applications. It condenses information into manageable chunks, providing users a cleaner interface. The effectiveness of this design was discussed in detail in the article Mastering CSS: Create the Perfect Expand/Collapse Menu, which outlines how CSS can enhance the visual aspect. However, for our purposes, we will delve into how Java frameworks can bring these menus to life dynamically.
Java Frameworks for Dynamic Menus
Spring Boot
Spring Boot is a widely adopted framework for creating stand-alone, production-grade Spring-based applications. Its ability to manage dependencies and streamline application setup makes it a popular choice for developers.
Key Features:
- Microservice architecture support
- Extensive set of integrated tools and libraries
- Simplified configuration
Implementation Example
Here is a sample code snippet using Spring Boot to create a basic expand/collapse menu:
@RestController
@RequestMapping("/menu")
public class MenuController {
@GetMapping
public Menu getMenu() {
List<SubMenu> subMenus = Arrays.asList(
new SubMenu("SubMenu 1", "Content for submenu 1"),
new SubMenu("SubMenu 2", "Content for submenu 2")
);
return new Menu("Main Menu", subMenus);
}
}
Why this code?
- We create a REST endpoint to serve our menu dynamically. This method encapsulates all the menu data on the server side, facilitating a seamless UI update on the client side via AJAX calls.
JavaServer Faces (JSF)
JavaServer Faces (JSF) is a component-based UI framework that simplifies the development of user interfaces for Java web applications. It offers built-in support for managing the state of UI components.
Key Features:
- Rich component set
- Event-driven programming model
- In-built support for AJAX
Implementation Example
Here's an example of how you might create a dynamic expand/collapse menu using JSF.
<h:panelGroup>
<h:commandButton value="Toggle Menu"
action="#{menuBean.toggle}"
update="menuPanel" />
<h:panelGroup id="menuPanel" rendered="#{menuBean.isExpanded}">
<ul>
<li>SubMenu 1</li>
<li>SubMenu 2</li>
</ul>
</h:panelGroup>
</h:panelGroup>
Why this code?
- We use
h:commandButton
to toggle the visibility of our menu. Therendered
attribute conditionally displays the submenu based on the state managed bymenuBean
, demonstrating JSF's power in state management.
Vaadin
Vaadin is another renowned Java framework for building modern web applications. It enables developers to create a rich user interface entirely in Java, abstracting away the complexities of HTML, CSS, and JavaScript.
Key Features:
- Write UIs entirely in Java
- Strong integration with Java backend
- Responsive by default
Implementation Example
The following snippet demonstrates how to build an expand/collapse menu using Vaadin:
VerticalLayout layout = new VerticalLayout();
Button expandButton = new Button("Expand/Collapse");
Accordion accordion = new Accordion();
accordion.add("Sub Menu 1", new Label("Content for submenu 1"));
accordion.add("Sub Menu 2", new Label("Content for submenu 2"));
expandButton.addClickListener(event -> {
accordion.setVisible(!accordion.isVisible());
});
layout.add(expandButton, accordion);
setContent(layout);
Why this code?
- Vaadin’s
Accordion
component provides a ready-made solution for creating expandable menus, allowing developers to focus purely on the functionality without getting tangled in the details of raw HTML/CSS.
Sample Implementation
Combining the frameworks, we can create a basic web application that features a dynamic expand/collapse menu. Ensure you've integrated Spring Boot with either JSF or Vaadin, as per your project requirements.
- Pom.xml Configuration: For Spring Boot with JSF, the dependencies in
pom.xml
must be correctly configured.
<dependency>
<groupId>com.sun.faces</groupId>
<artifactId>jsf-impl</artifactId>
<version>2.3.9</version>
</dependency>
-
Launch your Application: After you complete the setup, run your application and access your menu via a web browser.
-
Testing the Menu: Click to expand or collapse the options to ensure the interaction works as intended. It’s essential to test various aspects like responsiveness, state management, and integration.
Best Practices
- Accessibility: Ensure that your expand/collapse menus are accessible. Implementing ARIA attributes can greatly enhance accessibility.
- Performance: Load menus dynamically as users interact with them to optimize load times and performance.
- State Management: Use a robust state management system, especially when working with frameworks like JSF and Vaadin.
- Responsiveness: Ensure your menus are responsive across devices. Testing on multiple devices will help iron out any issues early in the design phase.
Final Considerations
Creating dynamic expand/collapse menus is not just about coding; it's about enhancing user interaction and making content more accessible. Java frameworks like Spring Boot, JSF, and Vaadin offer developers diverse and powerful tools to build such effective web components. Each of these frameworks has its strengths, and your choice might vary based on project requirements, team skillsets, and future scalability.
For more context on similar UI components and styles, feel free to check the article Mastering CSS: Create the Perfect Expand/Collapse Menu.
Happy coding, and may your menus always be dynamic!