Java Solutions for Managing Food Hydration in Apps
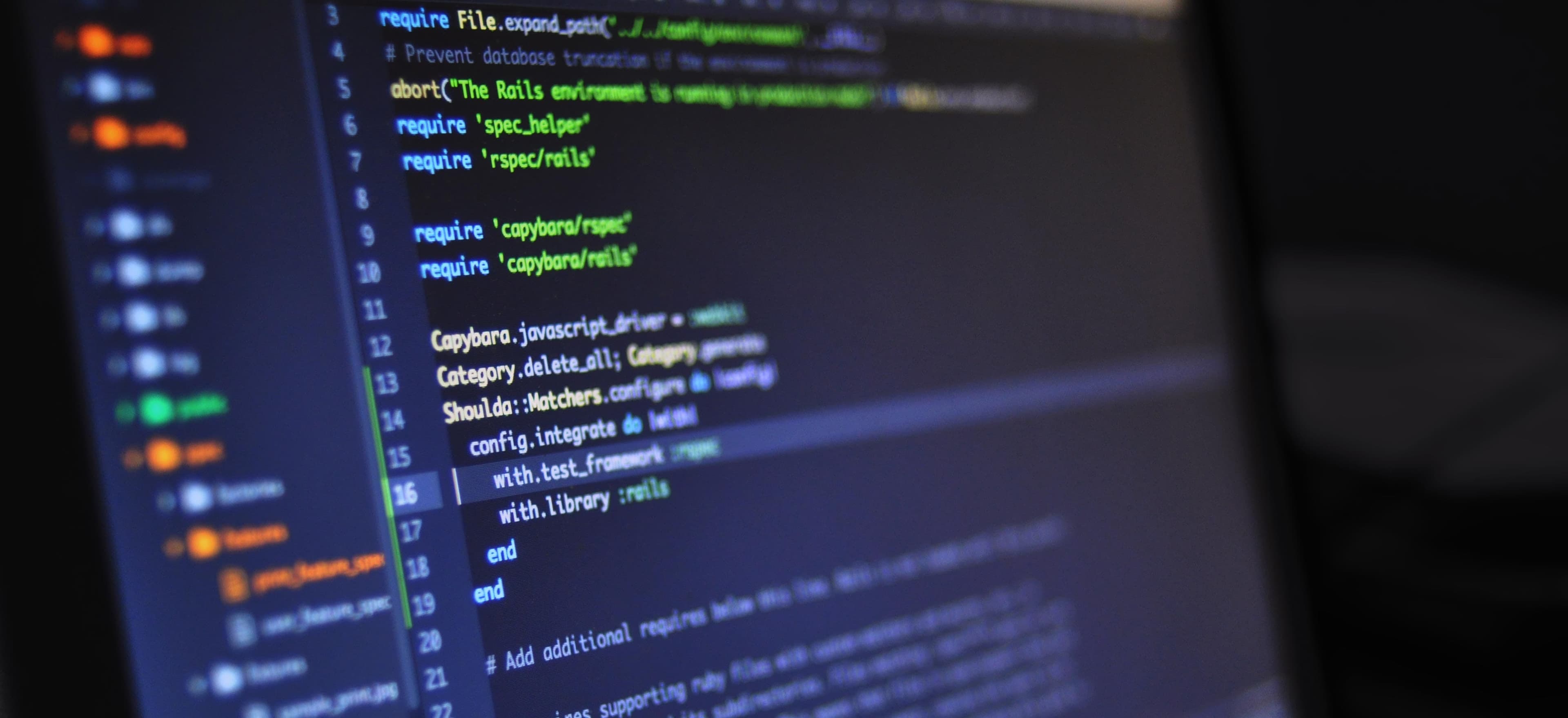
- Published on
Java Solutions for Managing Food Hydration in Apps
In today's tech-driven world, the intersection of health and technology is becoming increasingly important. One area where this is particularly relevant is food hydration. As detailed in the article titled "Hydrating Right: Water Needs for Freeze-Dried vs. Dehydrated Foods" (which you can read here), understanding how to correctly hydrate food can greatly influence nutrition and preparation methods. This post delves into how to develop a Java application that helps users manage food hydration needs effectively.
Why Focus on Hydration?
Before diving into the code, let's discuss why food hydration matters. Proper hydration is crucial for the quality, flavor, and nutritional value of food. Freeze-dried foods often need different water requirements than dehydrated ones. Incorrect hydration can lead to inconsistency and dissatisfaction in meal preparation.
Now, let's explore how we can create Java solutions to help users determine the right amount of water for their freeze-dried and dehydrated foods.
Setting Up Your Java Environment
Ensure you have a Java environment set up. For beginners, the Java Development Kit (JDK) and an Integrated Development Environment (IDE) such as IntelliJ IDEA or Eclipse will suffice.
Defining the Problem
The core challenge is to create a Java application that can:
- Allow users to input the type of food (freeze-dried or dehydrated).
- Accept the weight of the food item.
- Calculate the required water for hydration based on predefined ratios.
Code Structure
Here is a simple structure to start building the application in Java.
import java.util.Scanner;
public class FoodHydrationApp {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Welcome to the Food Hydration Manager!");
// Collect user input
System.out.print("Enter the type of food (freeze-dried/dehydrated): ");
String foodType = scanner.nextLine();
System.out.print("Enter the weight of the food in grams: ");
double weight = scanner.nextDouble();
// Calculate required water
double waterNeeded = calculateWaterNeed(foodType.toLowerCase(), weight);
// Output the result
if (waterNeeded == -1) {
System.out.println("Invalid food type entered. Please use freeze-dried or dehydrated.");
} else {
System.out.printf("For %.2f grams of %s food, you need %.2f liters of water.%n",
weight, foodType, waterNeeded);
}
scanner.close();
}
private static double calculateWaterNeed(String foodType, double weight) {
// Define water ratios
final double FREEZE_DRIED_RATIO = 0.25; // 1:4 ratio
final double DEHYDRATED_RATIO = 0.5; // 1:2 ratio
switch (foodType) {
case "freeze-dried":
return weight * FREEZE_DRIED_RATIO;
case "dehydrated":
return weight * DEHYDRATED_RATIO;
default:
return -1; // Invalid input
}
}
}
Code Explanation
- User Input: The program prompts the user to enter the type of food and its weight.
- Water Calculation: It uses a method
calculateWaterNeed
to compute the required water based on the defined ratios:- For freeze-dried foods, it requires 1 part water for every 4 parts of food (0.25).
- For dehydrated foods, it requires 1 part water for every 2 parts of food (0.5).
- Error Handling: If the food type entered does not match expected values, the program returns an error message.
Expanding Functionality
To make this application more robust, consider adding features like:
- A database: Store food hydration information and allow users to add entries.
- A GUI: Create a graphical interface using JavaFX or Swing for a user-friendly experience.
- Additional food types: Allow the application to handle a variety of food types and hydration requirements.
Sample GUI Implementation
To provide a graphical user experience, we can use JavaFX. Below is a basic implementation of a GUI for our hydration calculator.
import javafx.application.Application;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.layout.GridPane;
import javafx.stage.Stage;
public class FoodHydrationGUI extends Application {
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("Food Hydration Manager");
// Create the input fields
TextField weightField = new TextField();
ComboBox<String> foodTypeBox = new ComboBox<>();
foodTypeBox.getItems().addAll("freeze-dried", "dehydrated");
Button calculateButton = new Button("Calculate");
Label resultLabel = new Label();
// Define the layout
GridPane grid = new GridPane();
grid.setPadding(new Insets(10));
grid.setVgap(8);
grid.setHgap(10);
// Positioning the nodes
grid.add(new Label("Food Type:"), 0, 0);
grid.add(foodTypeBox, 1, 0);
grid.add(new Label("Weight (grams):"), 0, 1);
grid.add(weightField, 1, 1);
grid.add(calculateButton, 1, 2);
grid.add(resultLabel, 1, 3);
// Button action listener
calculateButton.setOnAction(e -> {
String foodType = foodTypeBox.getValue();
double weight = Double.parseDouble(weightField.getText());
double waterNeeded = calculateWaterNeed(foodType.toLowerCase(), weight);
if (waterNeeded == -1) {
resultLabel.setText("Invalid food type!");
} else {
resultLabel.setText(String.format("You need %.2f liters of water.", waterNeeded));
}
});
// Set scene and show
Scene scene = new Scene(grid, 300, 200);
primaryStage.setScene(scene);
primaryStage.show();
}
private double calculateWaterNeed(String foodType, double weight) {
final double FREEZE_DRIED_RATIO = 0.25;
final double DEHYDRATED_RATIO = 0.5;
switch (foodType) {
case "freeze-dried":
return weight * FREEZE_DRIED_RATIO;
case "dehydrated":
return weight * DEHYDRATED_RATIO;
default:
return -1;
}
}
public static void main(String[] args) {
launch(args);
}
}
GUI Explanation
- Input Fields: This version uses
TextField
for weight input andComboBox
for food type selection. - Button Action: Clicking the calculate button computes the water needed and displays the result in a
Label
. - Error Handling: Similar to the console application, it manages invalid inputs gracefully.
My Closing Thoughts on the Matter
Creating a Java application to manage food hydration can be straightforward and beneficial. As outlined, the program allows users to input food types and weight, helping them understand how much water is necessary for efficient hydration. By leveraging Java's object-oriented capabilities, we can expand upon this basic application, integrating more complex features such as databases, advanced user interfaces, and additional food types.
As highlighted in this discussion, managing hydration is vital for those relying on various food preservation methods. Implementing a Java solution not only serves users practically but also reinforces the critical health aspect of food management. For more on hydration needs, check out the article "Hydrating Right: Water Needs for Freeze-Dried vs. Dehydrated Foods" at youvswild.com.
Happy coding and healthy eating!
Checkout our other articles