Java Framework Solutions for jQuery GalleryView Problems
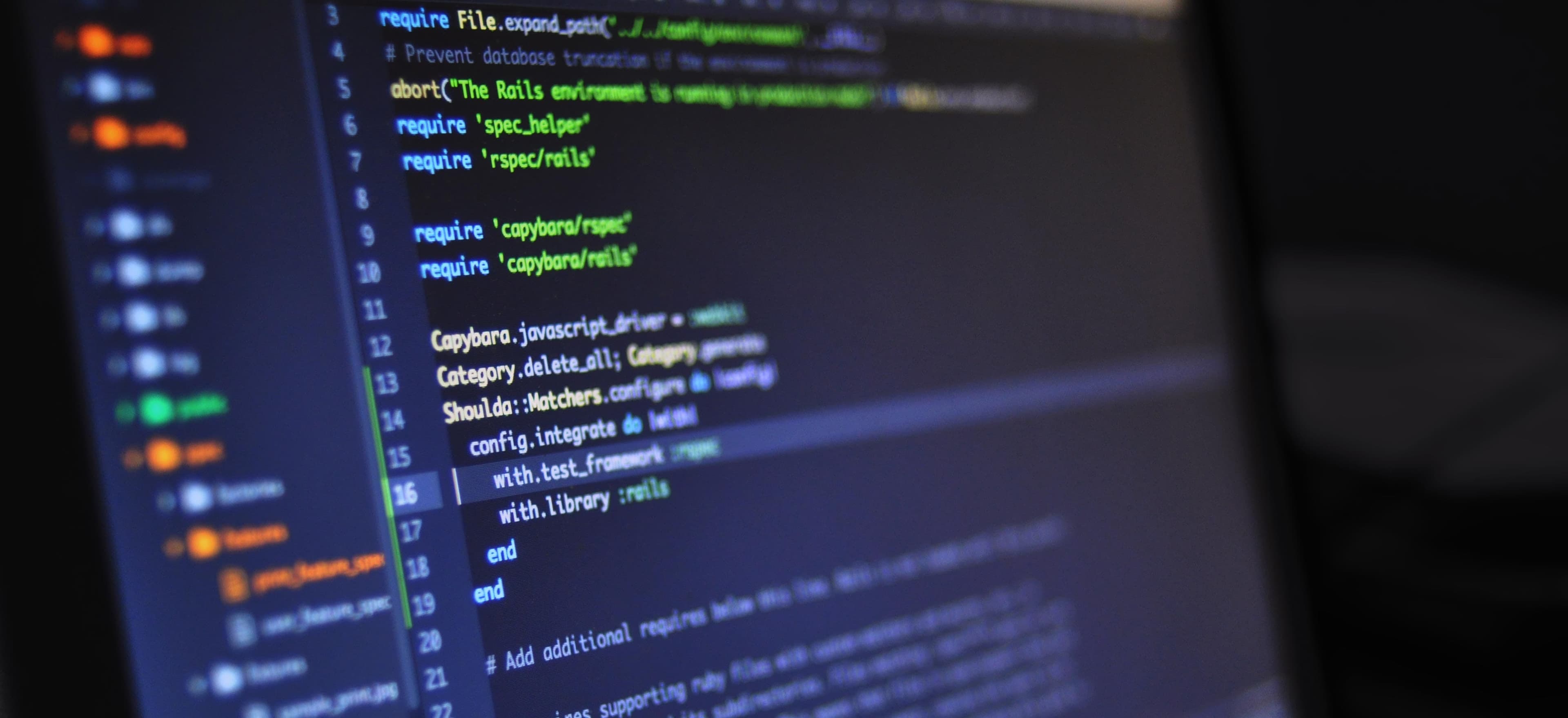
- Published on
Java Framework Solutions for jQuery GalleryView Problems
When you're working on web development, particularly with Java-based backend technologies, it's common to encounter issues with frontend frameworks like jQuery GalleryView. When these problems arise, Java frameworks can provide effective solutions that not only enhance functionality but also improve user experience. In this blog post, we will explore how Java frameworks can help revitalize your site’s jQuery GalleryView functionality.
If you’re facing challenges, you're not alone. I highly recommend checking out the article Revive Your Site: Solving jQuery GalleryView Issues for additional insights. But for now, let's dive deeper into some effective Java-based solutions to ensure your gallery runs smoothly.
Understanding jQuery GalleryView Issues
Before we delve into solutions, it is important to understand the common issues faced with jQuery GalleryView:
- Delayed Loading of Images: Users often encounter slow-loading galleries that deter engagement.
- Compatibility Issues: Different browsers may render the gallery inconsistently, causing frustrations.
- Unresponsive Designs: Many gallery implementations do not adapt to various screen sizes, particularly on mobile devices.
These issues are not insurmountable. By incorporating Java frameworks such as Spring, Hibernate, and JavaServer Faces (JSF), developers can streamline their web applications, creating a seamless user experience.
Why Use Java Frameworks?
Java frameworks provide several benefits:
- Seamless Integration: Frameworks like Spring can easily connect with JavaScript libraries, including jQuery.
- Robustness and Stability: Java is known for its robustness, making it suitable for enterprise-level applications.
- Scalability: With Java-based solutions, your application can handle increasing loads without compromising performance.
Solution 1: Using Spring Boot for Backend Enhancements
Spring Boot is a powerful Java framework that simplifies backend development. It can optimize image loading and manage server-side processes effectively.
Example Code: Spring Boot Controller
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.bind.annotation.ResponseBody;
@RestController
public class GalleryController {
@GetMapping("/gallery")
public @ResponseBody List<Image> getImages() {
// Fetch images from the database
return imageService.findAllImages();
}
}
Why This Works: By utilizing a RESTful controller, you can fetch images efficiently. This allows for asynchronous loading, meaning the gallery can populate images as they are retrieved rather than waiting for all resources, addressing the delayed loading issue.
Example Code: Frontend Integration
In your jQuery script, you can retrieveand render images like this:
$(document).ready(function(){
$.ajax({
url: '/gallery',
method: 'GET',
success: function(data){
data.forEach(function(image) {
$('#galleryContainer').append(`<img src="${image.url}" alt="${image.alt}"/>`);
});
},
error: function() {
alert("Error fetching images");
}
});
});
Why This Works: This AJAX call enables the gallery to load images dynamically, keeping the user engaged while images appear progressively rather than all at once.
Solution 2: Hibernate for Efficient Data Handling
Hibernate, a Java ORM (Object-Relational Mapping) framework, can enhance database interactions effectively.
Example Code: Hibernate Entity
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.Id;
@Entity
public class Image {
@Id
@GeneratedValue
private Long id;
private String url;
private String alt;
// Getters and Setters
}
Why This Works: Hibernate helps in reducing boilerplate code by managing database operations like queries and transactions. As a result, it allows you to focus more on the application logic without worrying about persistent data handling intricacies.
Solution 3: JavaServer Faces (JSF) for Dynamic Interfaces
JavaServer Faces (JSF) is another framework that can facilitate dynamic user interfaces while improving gallery responsiveness.
Example Code: JSF Managed Bean
import javax.faces.bean.ManagedBean;
import javax.faces.bean.ViewScoped;
@ManagedBean
@ViewScoped
public class GalleryView {
private List<Image> images;
@PostConstruct
public void init() {
images = imageService.findAllImages();
}
public List<Image> getImages() {
return images;
}
}
Why This Works: With JSF, you harness the power of Java on the server side, dynamically rendering UI components based on user interactions. This enables features like client-side pagination and filtering, thereby enhancing user experience for your gallery.
Addressing Compatibility Issues
While Java frameworks help streamline the backend processes, ensuring your gallery functions consistently across multiple browsers requires thoughtful consideration of your frontend code.
Best Practice: Use Feature Detection Libraries
Using libraries like Modernizr can help detect browser capabilities, allowing you to implement fallback methods for unsupported features.
Example Code: Feature Detection
<script src="https://cdnjs.cloudflare.com/ajax/libs/modernizr/2.8.3/modernizr.min.js"></script>
<script>
if (Modernizr.boxshadow) {
// Apply box shadow styles
} else {
// Fallback styles for older browsers
}
</script>
Why This Works: This ensures that users on varying devices still receive a polished experience regardless of the browser specifications.
To Wrap Things Up
By leveraging the capabilities of Java frameworks such as Spring, Hibernate, and JSF, you can significantly enhance the functionality of your jQuery GalleryView. Whether it is improving load times, managing database interactions better, or ensuring a responsive design, Java provides a solid foundation to work with.
Remember, integrating these technologies not only solves existing issues but also paves the way for scalability in your web application. For those interested in further reading on jQuery GalleryView issues, revisit the valuable insights provided in the article Revive Your Site: Solving jQuery GalleryView Issues.
Feel free to apply these concepts in your next project and elevate the user experience on your web applications. Happy coding!