Harnessing Java to Overcome jQuery GalleryView Challenges
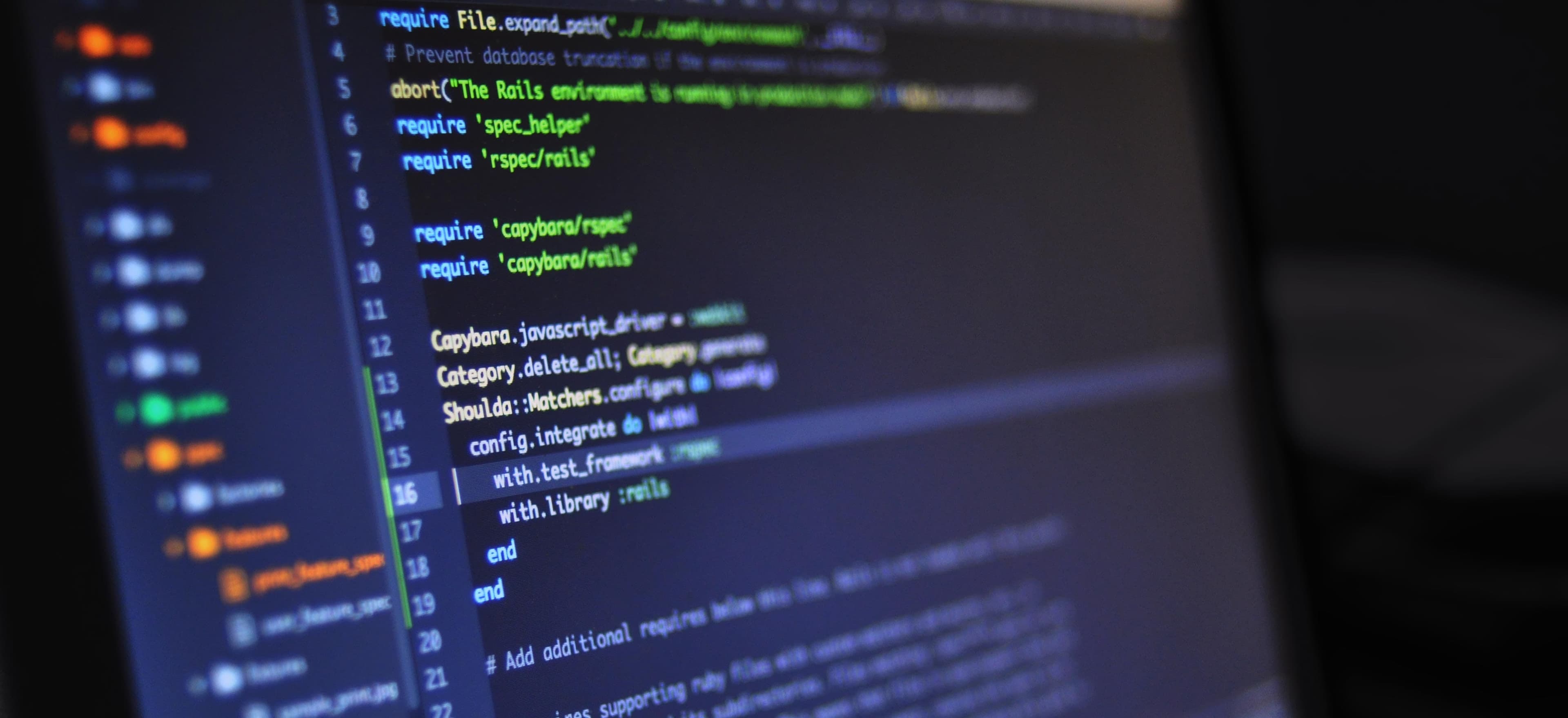
- Published on
Harnessing Java to Overcome jQuery GalleryView Challenges
As we traverse the intricacies of web development, encountering challenges in a framework or library is a common experience. One such instance can arise with jQuery's GalleryView, where developers often find themselves entangled in issues that hinder performance and user experience. However, what if we told you that Java could be a viable ally in solving these problems? In this blog post, we will explore how Java can be utilized to complement jQuery frameworks, streamline operations, and enhance the GalleryView experience.
Understanding jQuery GalleryView Struggles
jQuery GalleryView is a popular plugin that facilitates image galleries on websites. While convenient, developers frequently run into problems like performance lag, poor responsiveness, and bugs during transitions. To read more about these issues and potential fixes, you can check out the article Revive Your Site: Solving jQuery GalleryView Issues.
Given that the jQuery syntax can sometimes be entangled and less performant for large datasets, incorporating Java can make a substantial difference. Java, being strongly typed and object-oriented, allows for better structure, high performance, and easy integration with modern web technology.
Why Use Java Alongside jQuery?
-
Performance Optimization: Java is compiled, leading to faster execution times. It can handle heavy data processing tasks before the data hits the client-side, reducing load times.
-
Robustness: With Java, the code is less prone to runtime errors due to its strong typing system, which can enhance the quality of your application.
-
Backend Processing: Java can seamlessly serve as a backend technology alongside jQuery's frontend capabilities. This allows for efficient data handling, business logic implementation, and server-side rendering.
-
Scalability: As your client base grows, utilizing Java backend services can help scale your application more effectively compared to relying solely on jQuery.
Integrating Java with jQuery GalleryView
Let's examine a simple integration where Java processes and serves images to a jQuery GalleryView plugin. We will create a barebones Java servlet to handle requests and return image data in a JSON format, which jQuery can easily consume.
1. Setting Up Java Servlet
To create a servlet, you will need a Java web server (e.g., Apache Tomcat) and an IDE like Eclipse or IntelliJ IDEA.
Here’s a simple servlet to fetch images:
import javax.servlet.*;
import javax.servlet.http.*;
import java.io.*;
import org.json.*;
public class ImageServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// Sample data retrieval (In a real-world project, this would likely be from a database)
String[] imageUrls = {
"http://example.com/image1.jpg",
"http://example.com/image2.jpg",
"http://example.com/image3.jpg"
};
// Convert to JSON
JSONArray jsonImages = new JSONArray();
for (String url : imageUrls) {
jsonImages.put(url);
}
// Set response type to JSON
response.setContentType("application/json");
PrintWriter out = response.getWriter();
out.print(jsonImages.toString());
out.flush();
}
}
Code Explanation
-
Servlet Setup: We extend
HttpServlet
to create a custom servlet. ThedoGet
method handles GET requests made to this servlet. -
Data Retrieval: In this example, we're simply using hardcoded URLs. For a production app, you would likely pull this data from a database.
-
JSON Conversion: We use the
org.json
library to create a JSONArray of image URLs. This method simplifies how data is structured for the frontend. -
JSON Response: By specifying
response.setContentType("application/json")
, we inform the client that it can expect a JSON response.
2. Fetching Images with jQuery
Now, let’s see how we can fetch this image data using jQuery and display it in our GalleryView.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Dynamic Gallery</title>
<link rel="stylesheet" href="styles/gallery.css">
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="path/to/jquery.galleryview.js"></script>
</head>
<body>
<div id="gallery"></div>
<script>
$(document).ready(function() {
// Fetch images via AJAX
$.getJSON('ImageServlet', function(data) {
var images = [];
$.each(data, function(index, url) {
images.push('<img src="' + url + '" alt="Image ' + (index + 1) + '" />');
});
// Append images to the gallery div
$('#gallery').html(images.join(''));
// Initialize jQuery GalleryView
$('#gallery').galleryView();
});
});
</script>
</body>
</html>
Code Explanation
-
jQuery AJAX Call: We employ
$.getJSON
to fetch image data from the Java servlet we set up earlier. If you are using a different URL structure, make sure to adjust accordingly. -
Dynamic Image Loading: We loop over the returned data, creating image elements dynamically and appending them to the gallery div.
-
GalleryView Initialization: After the images are appended to the DOM, we call
$('#gallery').galleryView()
to initialize the GalleryView plugin, which should now populate with our images.
In Conclusion, Here is What Matters
Incorporating Java into your web applications can considerably increase robustness, performance, and data handling capabilities, especially for those situations where jQuery alone presents challenges. Utilizing Java servlets for backend processing can help alleviate common issues faced by developers working with the jQuery GalleryView.
By combining these technologies, you create a scalable architecture ready to handle the demands of modern web applications. For further detailed analysis of typical issues faced with jQuery GalleryView, feel free to reference the article Revive Your Site: Solving jQuery GalleryView Issues.
Whether you are a seasoned developer or just beginning your journey, the harmonious integration of Java and jQuery may very well be the solution to your next web development challenge.