Master Java Session Management for Effective Cookie Persistence
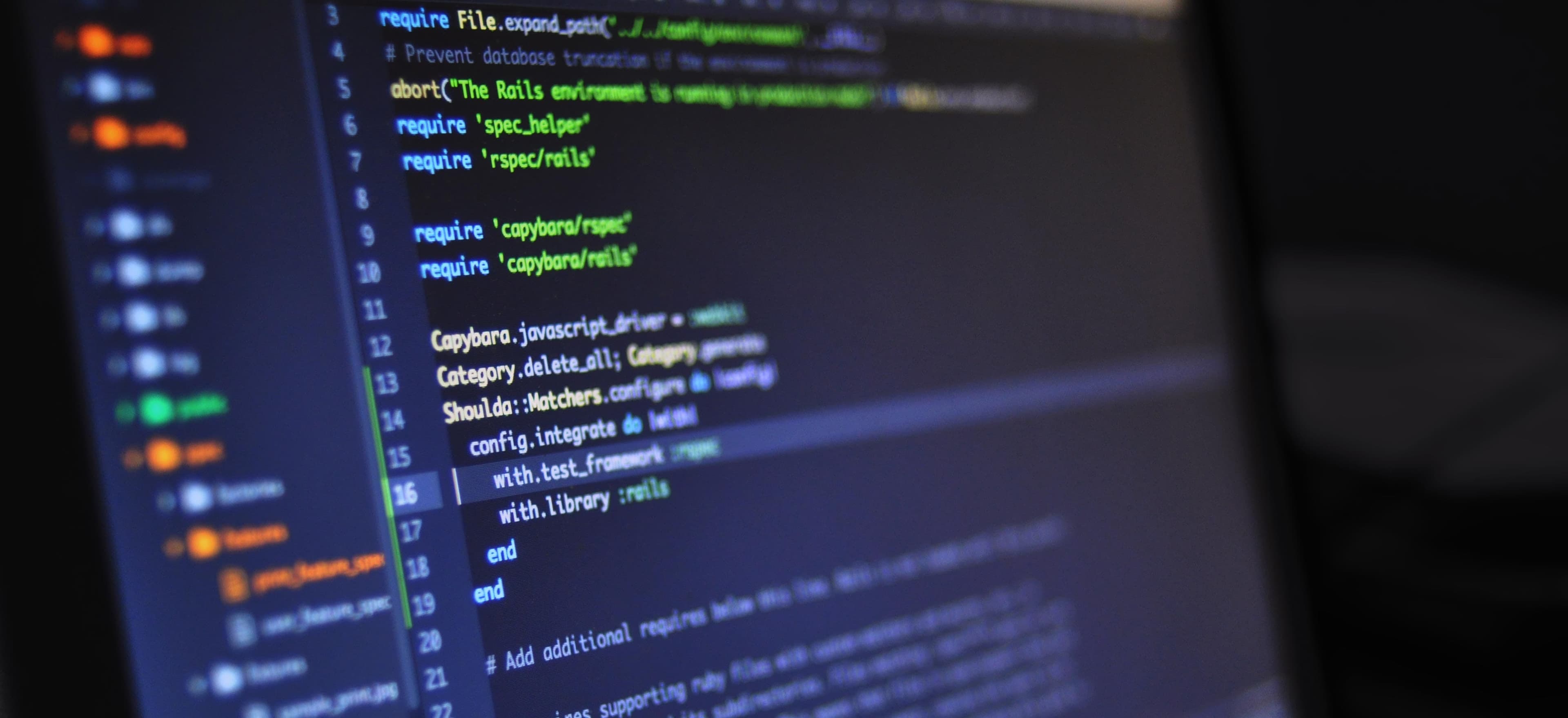
- Published on
Master Java Session Management for Effective Cookie Persistence
When developing web applications, one of the key challenges developers face is managing user sessions effectively. Understanding the concept of session management, particularly through cookies, can greatly enhance user experience. This article explores session management in Java, particularly focusing on cookie persistence. We’ll also draw parallels with the handling of cookie persistence in jQuery UI Tabs, as discussed in the article Handling Cookie Persistence in jQuery UI Tabs.
What is Session Management?
Session management refers to the ability to track user interactions with a web application across multiple requests. A session allows a server to maintain state information, such as user preferences, authentication status, and more. In contrast to a stateless architecture, session management ensures a personalized experience for users.
Why Use Cookies?
Cookies are small pieces of data that a server sends to a user's browser. The browser may store these cookies and send them back with subsequent requests to the server. They facilitate session management by enabling persistent storage of user data across sessions.
Java and Cookies: A Quick Overview
In Java, managing cookies typically involves the javax.servlet.http.Cookie
class. This class provides several methods to create, manipulate, and read cookies. Here's a basic example of how to create a cookie in a Java servlet:
import javax.servlet.http.Cookie;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
// Example method to create a cookie
public void createCookie(HttpServletRequest request, HttpServletResponse response) {
Cookie cookie = new Cookie("username", "JohnDoe"); // Create a cookie
cookie.setMaxAge(60 * 60); // Set cookie to expire in one hour
response.addCookie(cookie); // Add cookie to response
}
Why Use the Above Code?
- Cookie Creation: We create a cookie named "username" with a value of "JohnDoe".
- Setting Expiration: Using
setMaxAge(int seconds)
allows us to define how long the cookie should persist. - Sending the Cookie: With
response.addCookie(cookie)
, we send the cookie to the user's browser.
Reading Cookies in Java
To read cookies from a request, you can utilize the getCookies()
method from the HttpServletRequest
object. Here is a simple method to read cookies:
import javax.servlet.http.Cookie;
import javax.servlet.http.HttpServletRequest;
// Example method to read cookies
public void readCookies(HttpServletRequest request) {
Cookie[] cookies = request.getCookies(); // Retrieve cookies
if (cookies != null) {
for (Cookie cookie : cookies) {
if ("username".equals(cookie.getName())) {
System.out.println("Username: " + cookie.getValue()); // Output the cookie's value
}
}
}
}
Why Is This Important?
- Retrieval: We efficiently check if the "username" cookie exists.
- Value Extraction: By printing its value, we can use it for logic in our application, such as personalizing the user experience.
Implementing Cookie Persistence
Cookie persistence is the ability to maintain a user's data across browser sessions. To implement cookie persistence effectively, consider the following strategies:
Expiration Date
Always set an expiration date on cookies to control how long they are persisted. Utilizing session cookies (which expire once the browser is closed) versus persistent cookies (which remain until a specific date) can determine user experience.
cookie.setMaxAge(60 * 60 * 24 * 30); // Expires in 30 days
Security Considerations
When managing cookies, especially those that contain sensitive information, you should consider the following:
- Secure Flag: Ensure that cookies are only sent over HTTPS connections.
- HttpOnly Flag: Mitigate risks of cross-site scripting (XSS) by making cookies inaccessible to JavaScript.
Example of secure cookie creation:
cookie.setSecure(true); // Cookie is only sent over HTTPS
cookie.setHttpOnly(true); // Can't be accessed via JavaScript
Understanding Cookie Scope
Cookies have different scopes depending on the domain and path specified when they are set. For example, a cookie set for /app
will not be sent to /app/settings
. Make sure to set the correct path to enhance accessibility.
cookie.setPath("/app"); // Cookie will be sent to /app and all sub-paths
Java Code Summarization
To summarize, the following code shows how to create, read, and manage cookie persistence in Java:
public void manageCookies(HttpServletRequest request, HttpServletResponse response) {
// Create a new cookie
Cookie cookie = new Cookie("username", "JohnDoe");
cookie.setMaxAge(60 * 60 * 24 * 30); // Persistent for 30 days
cookie.setSecure(true);
cookie.setHttpOnly(true);
cookie.setPath("/app"); // Set path for proper scope
response.addCookie(cookie); // Send cookie to user
// Read cookies
Cookie[] cookies = request.getCookies();
if (cookies != null) {
for (Cookie c : cookies) {
if ("username".equals(c.getName())) {
System.out.println("Welcome back, " + c.getValue());
}
}
}
}
Incorporating with jQuery UI Tabs
In the context of Java web applications utilizing jQuery UI Tabs, implementing cookie persistence can significantly enhance user experience. The aforementioned article, Handling Cookie Persistence in jQuery UI Tabs, discusses how to maintain the state of tabs using cookies.
By adopting similar principles in Java, developers can ensure that users return to the tabs they last visited. Here’s a brief idea of how you could combine both frameworks:
- When a user interacts with a tab, JavaScript can store the last active tab in a cookie.
- On page load, the servlet can retrieve the cookie and render the corresponding tab as active.
Final Considerations
Mastering session management and cookie persistence in Java is crucial for building robust web applications. By integrating the strategies discussed in this article, you can provide a seamless user experience.
The interplay between server-side cookie management with Java and client-side state management with jQuery can lead to efficient applications tailored to user preferences.
By controlling cookie attributes like expiration, path, and security, you will not only improve user experience but also ensure a secure application environment.
Leverage these practices in your projects to enhance accessibility and maintain user engagement. Happy coding!