Mastering API Gateway Caching in Your Java Application
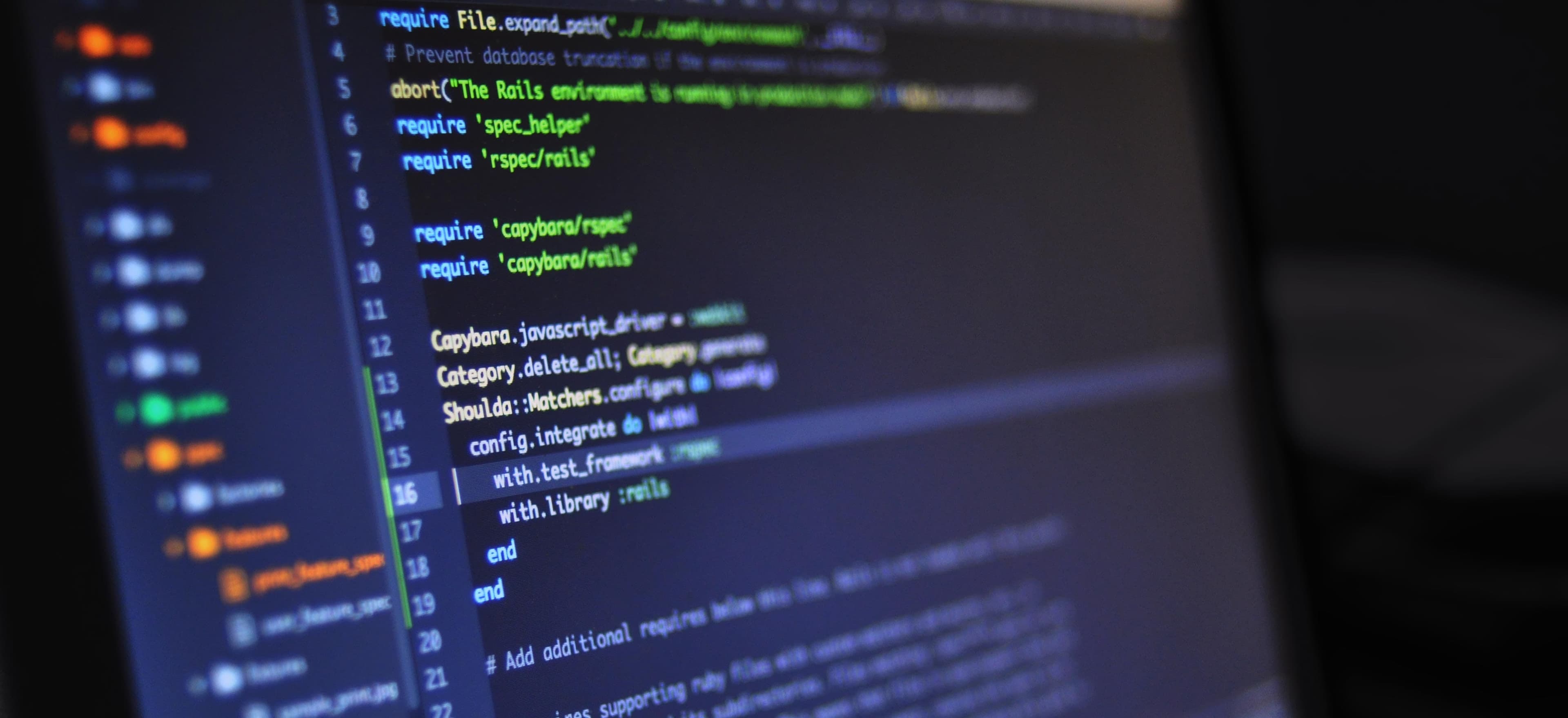
- Published on
Mastering API Gateway Caching in Your Java Application
Caching is a critical performance optimization technique that can drastically improve the efficiency of an API gateway. In the fast-paced world of software development, particularly with microservices architectures, understanding how to leverage caching can reduce latency, minimize backend load, and enhance user experience. If you're struggling with API gateway caching, you might find insights in our previously published article, Struggling with API Gateway Caching? Here's Your Fix!.
What is API Gateway Caching?
API Gateway Caching is a mechanism that stores copies of responses for requests at the API gateway level. This means that when a client makes a request for the same resource, the gateway can return a cached response rather than forwarding the request to the backend service. This results in faster response times and less load on backend services.
Benefits of API Gateway Caching
- Reduced Latency: Cached responses can be delivered more quickly than responses retrieved from backend services.
- Lower Backend Load: Caching minimizes the number of requests that hit your services, reducing CPU and memory resource usage.
- Improved Scalability: By offloading a portion of the requests to the cache, your API can handle increased traffic more effectively.
Enabling Caching in Your API Gateway
While the specifics can vary depending on the gateway you are using—be it AWS API Gateway, Nginx, or Kong, for example—the general principles remain similar. We'll illustrate how to implement caching in a Java application using Spring Cloud Gateway, a popular choice for building API gateways in Java.
Step 1: Setting Up Spring Cloud Gateway
To get started, create a new Spring Boot project. You can use Spring Initializr to bootstrap your project by adding dependencies for Spring Web and Spring Cloud Gateway.
Example Dependency in pom.xml
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-gateway</artifactId>
</dependency>
Step 2: Configuring Caching
In order to configure caching in your Spring Cloud Gateway application, you can leverage the CacheFilter
interceptor, which allows us to set cache-related headers.
Setting Up Cache Configuration
Add the following configuration to your application.yml
file:
spring:
cloud:
gateway:
routes:
- id: my_service_route
uri: http://localhost:8081 # Your backend service
predicates:
- Path=/api/v1/resource # The path to cache
filters:
- name: AddResponseHeader
args:
name: Cache-Control
value: public, max-age=3600 # Cache for 1 hour
Commentary on Cache-Control Header
The Cache-Control: public, max-age=3600
header instructs the browser and intermediary proxies to cache the response for one hour. This helps improve performance by minimizing redundant requests to your backend service.
Step 3: Testing the Configuration
After configuring caching, you can test your setup by making requests to your API route. You should observe that subsequent requests within the specified cache duration return faster responses as they come from the cache.
Example Testing with Curl
curl -v http://localhost:8080/api/v1/resource
You will see headers in the response indicating whether the response is coming from cache or if it hit the backend:
HTTP/1.1 200 OK
Cache-Control: public, max-age=3600
...
This confirmation means that your caching is working correctly.
Caching Strategies
When implementing caching, it's essential to choose the right caching strategy for your application needs. Below are a few common strategies:
-
Cache Aside: Load data into the cache on demand. When the application needs data, it checks the cache first; if missed, it fetches it from the data source and updates the cache.
-
Cache Invalidation: Implement mechanisms to invalidate or refresh the cache when the data changes. This is crucial for maintaining the accuracy of the cached data.
-
TTL (Time-to-Live): Set time limits on how long data can stay in the cache. This conclusion helps keep stale data from being served to users.
Managing Cache Invalidation in Java
Invalidating or updating the cache is crucial for keeping the data accurate in your application. You can set cache invalidation logic based on your domain model.
Example Cache Invalidation Logic
Let’s assume you have a service that handles user data:
@Service
public class UserService {
private final Cache<User> userCache = ...; // Initialize your caching mechanism
public User getUser(String userId) {
User cachedUser = userCache.get(userId);
if (cachedUser != null) {
return cachedUser; // Return cached user
}
User user = fetchFromDatabase(userId); // Fetch from database
userCache.put(userId, user); // Cache the newly fetched user
return user;
}
public void updateUser(User user) {
updateDatabase(user); // Presume we update DB first
userCache.evict(user.getId()); // Invalidate the cache
}
}
Commentary on Updating Cache
In this design, we first check if the user exists in the cache and return it if available. On updating user data, we first ensure the database is updated and then invalidate the cache for that user. This approach ensures that users receive updated data without old cache conflicts.
Monitoring API Gateway Performance
Once caching is implemented, monitoring its effectiveness is crucial. Tools like Spring Boot Actuator can help in monitoring your application's health and metrics.
Adding Metrics in Your Application
Add the Actuator dependency:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
This enables you to access valuable metrics such as requests per minute, cache hit ratios, and response times.
Accessing Metrics
You can access Actuator metrics by visiting:
http://localhost:8080/actuator/metrics
This endpoint will give you insight into cache performance, helping you make data-driven decisions about the effectiveness of your caching configuration.
My Closing Thoughts on the Matter
API Gateway caching is an essential technique that can dramatically enhance your Java application's performance. Through configuring caching in Spring Cloud Gateway, understanding caching strategies, and managing cache lifecycle effectively, you can ensure scalable and responsive API services.
Remember to regularly monitor your caching strategies and adapt them according to your application's usage patterns. For in-depth discussions around API Gateway Caching challenges, be sure to check out Struggling with API Gateway Caching? Here's Your Fix!.
Incorporating these practices not only makes your application faster but also provides a better experience for your users. Happy coding!
Checkout our other articles