Debugging Java UI: Tackling Rogue Layout Anomalies
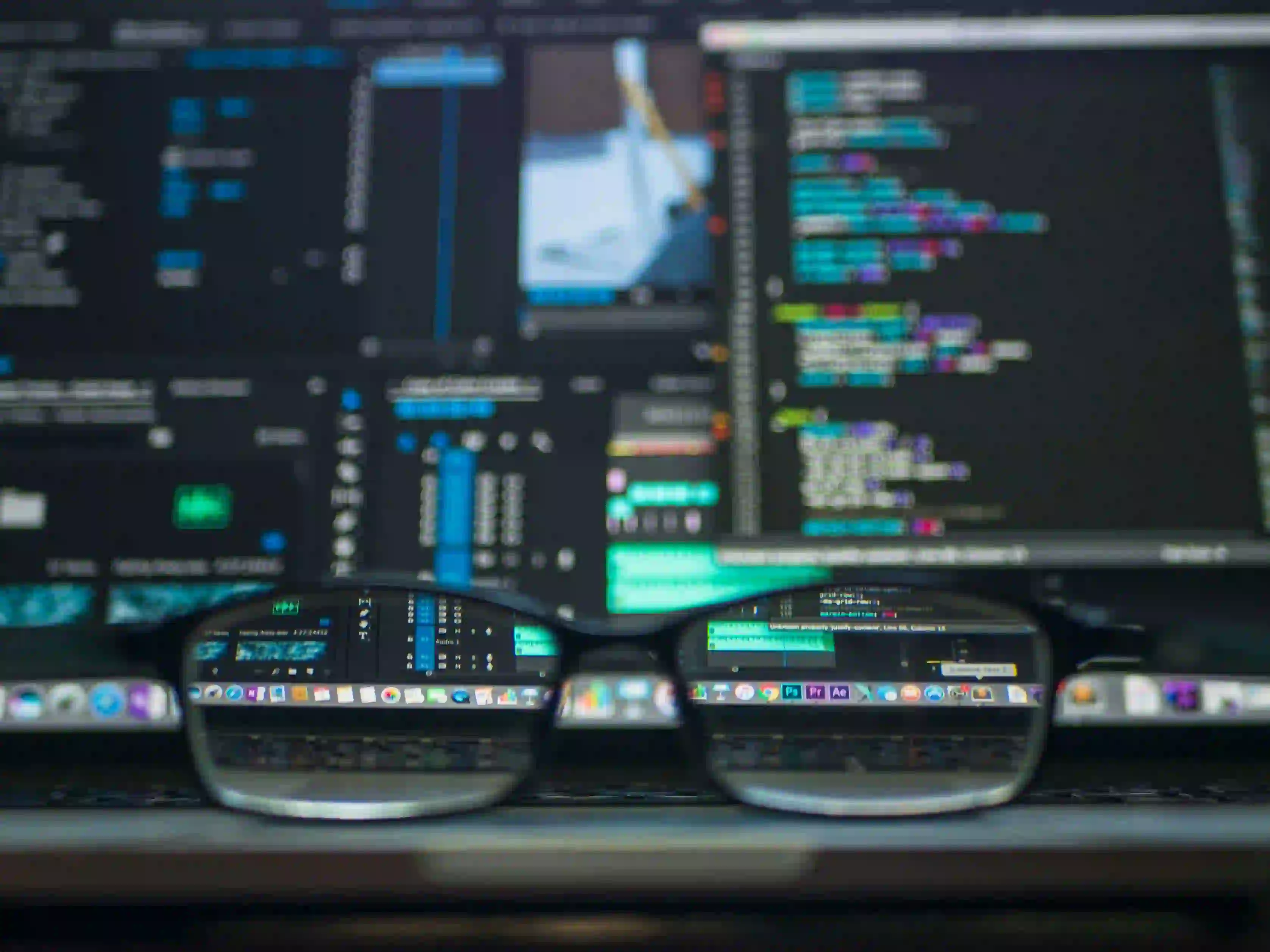
Debugging Java UI: Tackling Rogue Layout Anomalies
Java is a robust platform favored for its versatility in creating user interfaces (UIs). However, even seasoned developers can find themselves ensnared by rogue layout anomalies—unexpected behaviors that disrupt the intended visual and functional aspects of an application. In this post, we will delve into effective strategies for debugging these UI issues while linking to an insightful resource: Spotting Anomalies: Fixing Layout Elements Gone Rogue.
Understanding Layouts in Java
Java provides various layout managers, each designed for specific tasks. These enable the arrangement of components in user interfaces. Key layouts include:
- FlowLayout: Arranges components in a line.
- BorderLayout: Divides the space into five regions.
- GridLayout: Displays items in a grid format.
However, selecting the wrong layout or misconfiguring properties can lead to rogue anomalies. Understanding these layouts forms the foundation of effective debugging.
Example: FlowLayout
In a FlowLayout, components are arranged sequentially. Here's a basic implementation:
import javax.swing.*;
import java.awt.*;
public class FlowLayoutExample {
public static void main(String[] args) {
JFrame frame = new JFrame("FlowLayout Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setLayout(new FlowLayout());
JButton button1 = new JButton("Button 1");
JButton button2 = new JButton("Button 2");
JButton button3 = new JButton("Button 3");
frame.add(button1);
frame.add(button2);
frame.add(button3);
frame.setSize(300, 200);
frame.setVisible(true);
}
}
Why Use FlowLayout?
FlowLayout is straightforward. It's best for when you want components to wrap to a new line automatically. But beware—it can lead to rogue elements if you are embedding it within more complex layouts.
Diagnosing Layout Issues
When a layout doesn’t behave as expected, immediate troubleshooting is essential. Here’s a process to pinpoint issues:
- Examine Component Sizes: Often, rogue elements arise from incorrect sizes. If buttons overlap or overflow, check their preferred size.
- Inspect Layout Constraints: Ensure that any constraints defined for the layout manager are in place. In BorderLayout, forgetting a component placement can lead to unexpected results.
- Debug Mode: Utilize Java’s built-in debugging features to step through UI rendering.
Example: GridLayout
Here's a snippet demonstrating a GridLayout that can run into issues if not configured properly:
import javax.swing.*;
import java.awt.*;
public class GridLayoutExample {
public static void main(String[] args) {
JFrame frame = new JFrame("GridLayout Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setLayout(new GridLayout(2, 2));
JButton button1 = new JButton("Button 1");
JButton button2 = new JButton("Button 2");
JButton button3 = new JButton("Button 3");
JButton button4 = new JButton("Button 4");
frame.add(button1);
frame.add(button2);
frame.add(button3);
frame.add(button4);
frame.setSize(300, 300);
frame.setVisible(true);
}
}
Why Use GridLayout?
This layout neatly organizes components into a grid format. Any rogue element here may be a result of mismatch in intended rows or columns. Always verify intentionality behind Rows and Columns used.
Techniques for Fixing Rogue Layouts
Once anomalies are identified, implement specific techniques to resolve these issues.
Component Alignment
Correctly aligning components can often mitigate rogue behavior. For instance, if buttons are not distributing properly in a BorderLayout:
import javax.swing.*;
import java.awt.*;
public class BorderLayoutExample {
public static void main(String[] args) {
JFrame frame = new JFrame("BorderLayout Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setLayout(new BorderLayout());
JButton buttonNorth = new JButton("North");
JButton buttonSouth = new JButton("South");
JButton buttonEast = new JButton("East");
JButton buttonWest = new JButton("West");
JButton buttonCenter = new JButton("Center");
frame.add(buttonNorth, BorderLayout.NORTH);
frame.add(buttonSouth, BorderLayout.SOUTH);
frame.add(buttonEast, BorderLayout.EAST);
frame.add(buttonWest, BorderLayout.WEST);
frame.add(buttonCenter, BorderLayout.CENTER);
frame.setSize(300, 300);
frame.setVisible(true);
}
}
Why BorderLayout?
In this layout, components fill specific regions. An unintentional overlap can happen if placements are mishandled. Always ensure these constraints reflect your design.
Use of Insets and Padding
Sometimes, rogue layouts stem from insufficient spacing between components. Utilizing insets can provide the necessary gaps.
public class InsetExample {
public static void main(String[] args) {
JFrame frame = new JFrame("Inset Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setLayout(new FlowLayout(FlowLayout.CENTER, 10, 10)); // Horizontal and vertical gaps of 10
JButton button1 = new JButton("Button with Insets");
JButton button2 = new JButton("Another Button");
frame.add(button1);
frame.add(button2);
frame.setSize(250, 200);
frame.setVisible(true);
}
}
Why Insets?
Insets help in avoiding collisions and ensure a polished look. They are crucial in creating a user-friendly interface.
Tools for Debugging
In addition to addressing anomalies through programming, tools exist to aid in this process. Java IDEs like Eclipse or IntelliJ IDEA come equipped with features for layout previews and run-time inspections. Tools such as JProfiler or profiling candidates provide insights into component rendering characteristics that may unveil hidden issues.
Final Thoughts
Debugging rogue layout anomalies in Java isn’t merely about fixing visible glitches. It's an interplay of understanding layout managers, addressing component properties, applying best practices, and utilizing appropriate tools.
For further detailed insights into layout issues and methods to effectively fix rogue elements, I recommend reading the article titled Spotting Anomalies: Fixing Layout Elements Gone Rogue.
In summary, mastering Java UI debugging enriches your development capacity, elevating user experience. Always keep your code modular and clean and watch the beauty and functionality of your UI flourish. Happy coding!