Fixing Rogue Layouts: A Java Developer's Guide to Anomalies
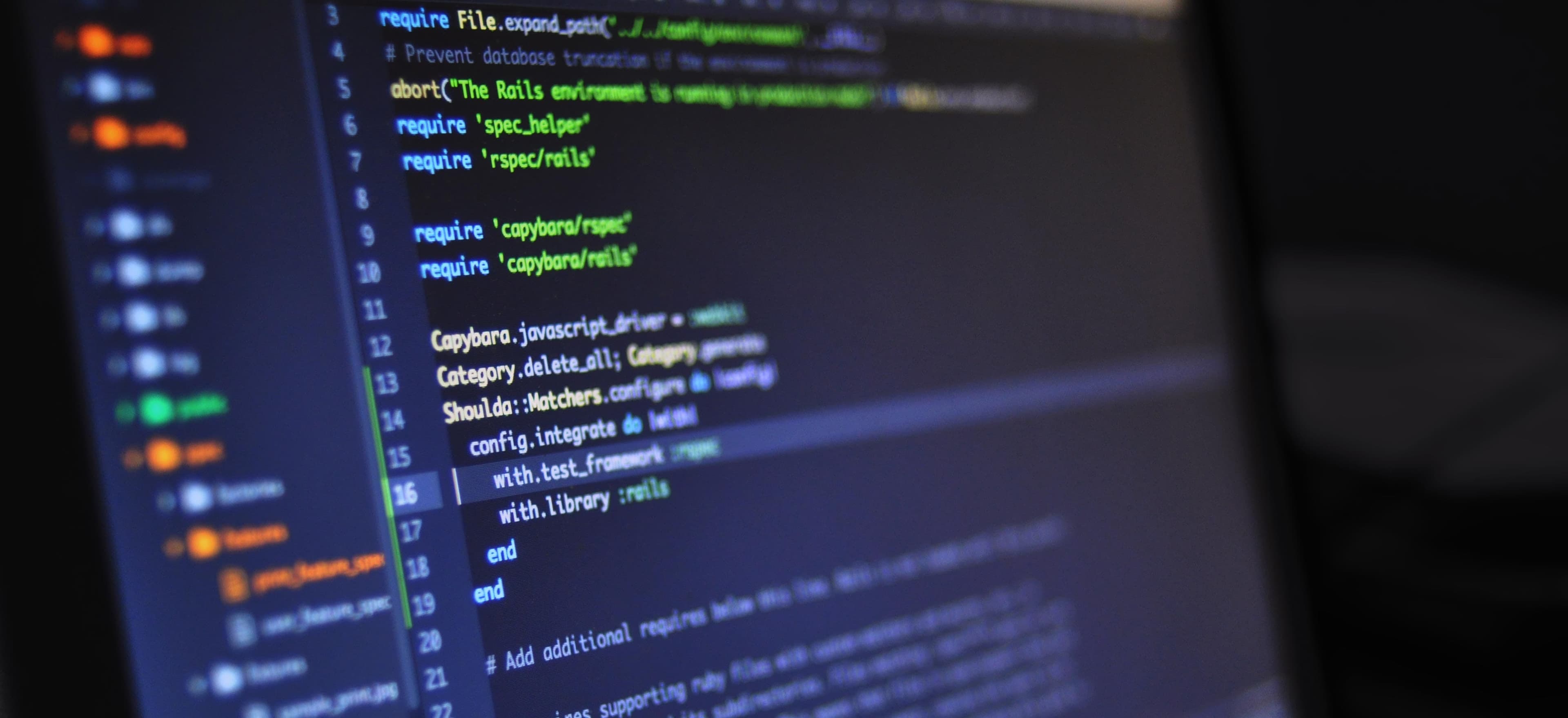
- Published on
Fixing Rogue Layouts: A Java Developer's Guide to Anomalies
In the world of web and application development, layout anomalies can disrupt user experience, leading to frustration and a lack of trust in your application. Whether you're a seasoned Java developer or just starting out, understanding how to diagnose and fix rogue layouts is essential. This guide will explore common layout issues in Java applications, while we'll draw on relevant strategies discussed in the article Spotting Anomalies: Fixing Layout Elements Gone Rogue.
Understanding Layout Anomalies
Layout anomalies can occur for many reasons, including:
- Incorrect CSS Styles: Misconfigured style sheets can throw off alignment.
- Browser Compatibility Issues: Different browsers may render elements differently.
- Java Container Mismanagement: Improper usage of Java frameworks and libraries.
- Dynamic Content Changes: Content that loads asynchronously often leads to layout shifts.
By pinpointing the root causes of layout issues, we can apply targeted strategies to resolve them. It's crucial to regularly test your layout in various environments and across different browsers to identify these anomalies early.
Common Layout Anomalies and Solutions
1. Misaligned Elements
Misalignment is one of the most common layout anomalies. This often arises from incorrect CSS properties or container configurations.
Solution: Use Flexbox or Grid layout systems. Here’s a simple example using JavaFX for aligning components.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.HBox;
import javafx.scene.control.Button;
import javafx.stage.Stage;
public class LayoutExample extends Application {
@Override
public void start(Stage primaryStage) {
HBox hBox = new HBox();
hBox.setSpacing(10); // Set space between elements
Button button1 = new Button("Button 1");
Button button2 = new Button("Button 2");
hBox.getChildren().addAll(button1, button2);
Scene scene = new Scene(hBox, 300, 100);
primaryStage.setScene(scene);
primaryStage.setTitle("Misaligned Elements Fixed");
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Why? Using layout containers like HBox ensures that your components are automatically managed. This reduces the risk of misalignment due to hardcoded sizes or positions.
2. Content Overflow
Dynamic content, particularly when it comes from APIs, can often cause layout overflow. Ensure you properly manage overflow properties within your CSS files.
Solution: Utilize overflow
properties in your stylesheets.
.container {
width: 100%;
height: 400px;
overflow: auto; /* Add scroll bars as needed */
}
Why? The overflow
property helps in keeping your UI organized, preventing content from spilling outside its boundaries. A cluttered interface not only looks bad but also hinders usability.
3. Element Blockages
When elements overlap or block each other, it can lead directly to a poor user experience. This typically originates from improper z-index management or unnecessarily positioned elements.
Solution: Always set z-index for layered components.
.header {
position: relative;
z-index: 10; /* Ensure it sits above other components */
}
.content {
position: relative;
z-index: 5; /* Below the header */
}
Why? Using z-index correctly allows you full control over your element layering, ensuring that important elements are always accessible.
Debugging Your Layouts
So, how do you go about debugging layout issues? Here are some steps:
- Inspect Element: Use your browser’s developer tools to inspect each component on the page.
- Console Logs: Java's
System.out.println
can help you track component sizes and positions. - Test Responsiveness: Resize your browser or use a tool like BrowserStack to check how your app renders on different devices.
Example Debugging Output
System.out.println("Button Size: " + button.getWidth() + " x " + button.getHeight());
Why? Debugging output helps in understanding how layouts respond to changes, enabling you to catch anomalies before they reach the end user.
Recommendations for Preventing Future Anomalies
- Regular Updates: Always keep Java and any frameworks you are using updated to the latest versions.
- Responsive Design: Adopt a mobile-first approach. This typically ensures that your layouts stay functional on all screen sizes.
- Consistent Testing: Establish a routine for testing across different browsers and devices.
Key Takeaways
Layout anomalies can be a developer's nightmare, but with the right strategies, these issues can be mitigated effectively. Leveraging Java's capabilities in conjunction with thoughtful design principles will lead to smooth and coherent user interfaces.
If you want to dive deeper into spotting and fixing rogue layout elements, I encourage you check out the article Spotting Anomalies: Fixing Layout Elements Gone Rogue. Here, you will find additional tips and tricks that align with what we've discussed.
By mastering these skills, you will see a marked improvement in your application's layout and, consequently, user satisfaction. Happy coding!
Checkout our other articles