Boost Java Performance: Java Caching Techniques Uncovered
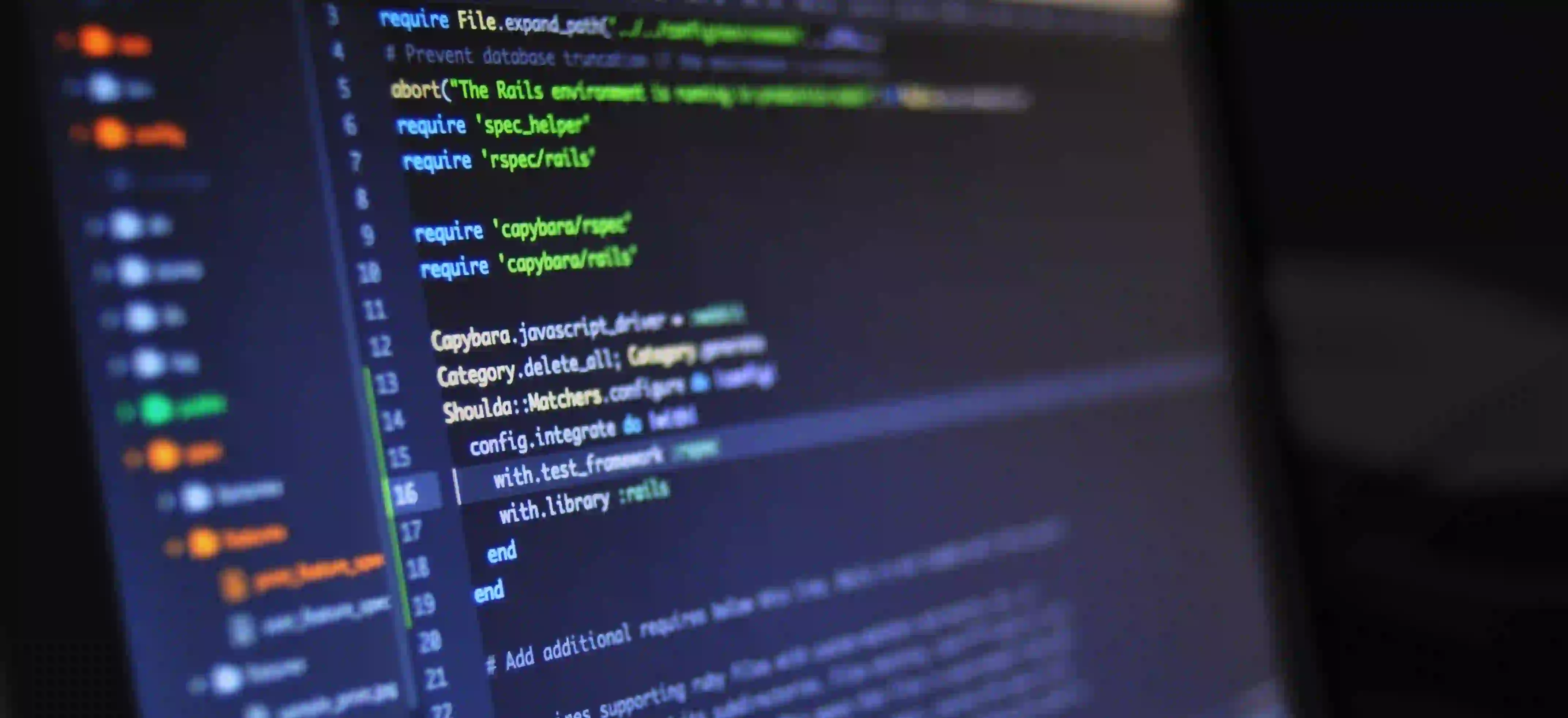
Boost Java Performance: Java Caching Techniques Uncovered
Java is a powerful and widely-used programming language that excels in building scalable applications. However, as applications grow, performance issues can arise. One of the most effective ways to tackle these issues is through caching. In this post, we’ll dive deep into Java caching techniques that can optimize performance, ensuring your applications run smoothly and efficiently.
What is Caching?
Caching is the process of storing frequently accessed data in a temporary storage area called a cache. This allows for quicker access to data and reduces the time taken in fetching it from the primary data source, such as a database or an API.
By storing copies of costly operations/results, you save time in future requests. Implementing caching can lead to significant performance gains, particularly in read-heavy applications.
Why is Caching Important?
- Performance: Faster response times can dramatically improve user experience.
- Scalability: Reducing the load on the database or external API allows for more simultaneous users.
- Cost-Efficiency: Reducing server load can cut down on cloud service costs.
Types of Caching in Java
Several caching strategies can be used, each suited for different scenarios:
- In-Memory Caching
- Distributed Caching
- Local Caching
1. In-Memory Caching
In-memory caching stores data in the memory of the application server. This technique is incredibly fast since accessing data in RAM is significantly quicker than hitting the database.
Example: Using ConcurrentHashMap for In-Memory Caching
Here’s a simple implementation of an in-memory cache using Java’s ConcurrentHashMap
:
import java.util.concurrent.ConcurrentHashMap;
public class InMemoryCache {
private final ConcurrentHashMap<String, String> cache = new ConcurrentHashMap<>();
// Fetches data from cache
public String get(String key) {
return cache.get(key);
}
// Stores data in cache
public void put(String key, String value) {
cache.put(key, value);
}
}
Why Use ConcurrentHashMap?
ConcurrentHashMap
provides concurrency at scale, allowing multiple threads to read and write without impacting performance.
2. Distributed Caching
In a distributed system, data is often shared across multiple instances of applications. A distributed cache can cache data across different nodes to ensure consistency and scalability.
Example: Using Redis as a Distributed Cache
Redis is one of the most popular tools for distributed caching. Here’s an example of using Redis to cache the results of an expensive operation:
import redis.clients.jedis.Jedis;
public class DistributedCache {
private Jedis jedis;
public DistributedCache() {
this.jedis = new Jedis("localhost"); // Adjust for your setup
}
public String getData(String key) {
String value = jedis.get(key);
if (value == null) {
value = expensiveOperation(); // Placeholder for costly computation
jedis.set(key, value);
}
return value;
}
private String expensiveOperation() {
// Simulate a delay for fetching data
try {
Thread.sleep(1000); // Simulating slow operation
} catch (InterruptedException e) {
e.printStackTrace();
}
return "Fetched Data";
}
}
Why Use Redis?
Redis is an in-memory data structure store that is fast, efficient, and supports various data structures. Its high availability and scalability make it ideal for large applications.
3. Local Caching
Local caching keeps data within the application instance. Although not suitable for every situation, it can work well in single-instance applications.
Example: Caffeine Caching Library
Caffeine is a high-performance caching library in Java. Here’s how to implement a simple local cache using Caffeine:
import com.github.benmanes.caffeine.cache.Cache;
import com.github.benmanes.caffeine.cache.Caffeine;
import java.util.concurrent.TimeUnit;
public class CaffeineCache {
private final Cache<String, String> cache;
public CaffeineCache() {
this.cache = Caffeine.newBuilder()
.expireAfterWrite(10, TimeUnit.MINUTES) // Cache expiration policy
.maximumSize(1000) // Max entries
.build();
}
public String get(String key) {
return cache.get(key, k -> expensiveOperation(k)); // Loads from cache or computes
}
private String expensiveOperation(String key) {
// Simulating a costly operation
return "Value for " + key;
}
}
Why Use Caffeine?
Caffeine provides efficient caching and is highly configurable. It also utilizes an eviction policy that is automatic and dynamic based on the application's usage pattern.
Best Practices for Java Caching
- Choose the Right Strategy: Depending on the use case, select between In-Memory, Distributed, or Local Caching.
- Set Expiration Policies: Cache entries should not live indefinitely. Setting expiration policies ensures that stale data does not persist in the cache.
- Utilize Cache Hits and Misses: Monitor the cache for efficiency. Understanding cache hits vs. misses can uncover optimization opportunities.
- Keep Cache Size Manageable: Limit the cache size to avoid memory overflow and ensure performance doesn’t degrade.
Final Thoughts
Caching can significantly enhance the performance of your Java applications, but it requires thoughtful implementation and monitoring. By understanding the various caching mechanisms and selecting the most suitable strategy, you can ensure that your applications remain responsive and efficient as they scale.
For deeper insights on caching in Java and similar performance techniques, check out Mastering useMemo and useCallback for Efficient React Caching, where caching principles are also heavily emphasized in the context of UI frameworks.
In the world of Java, caching is not merely an optimization tool; it is a necessity for creating performant applications. So, whether you opt for In-Memory, Distributed, or Local caching, make sure you integrate these techniques into your development cycle to unlock the full potential of your Java applications.