Optimizing Java Performance with React's useMemo and useCallback
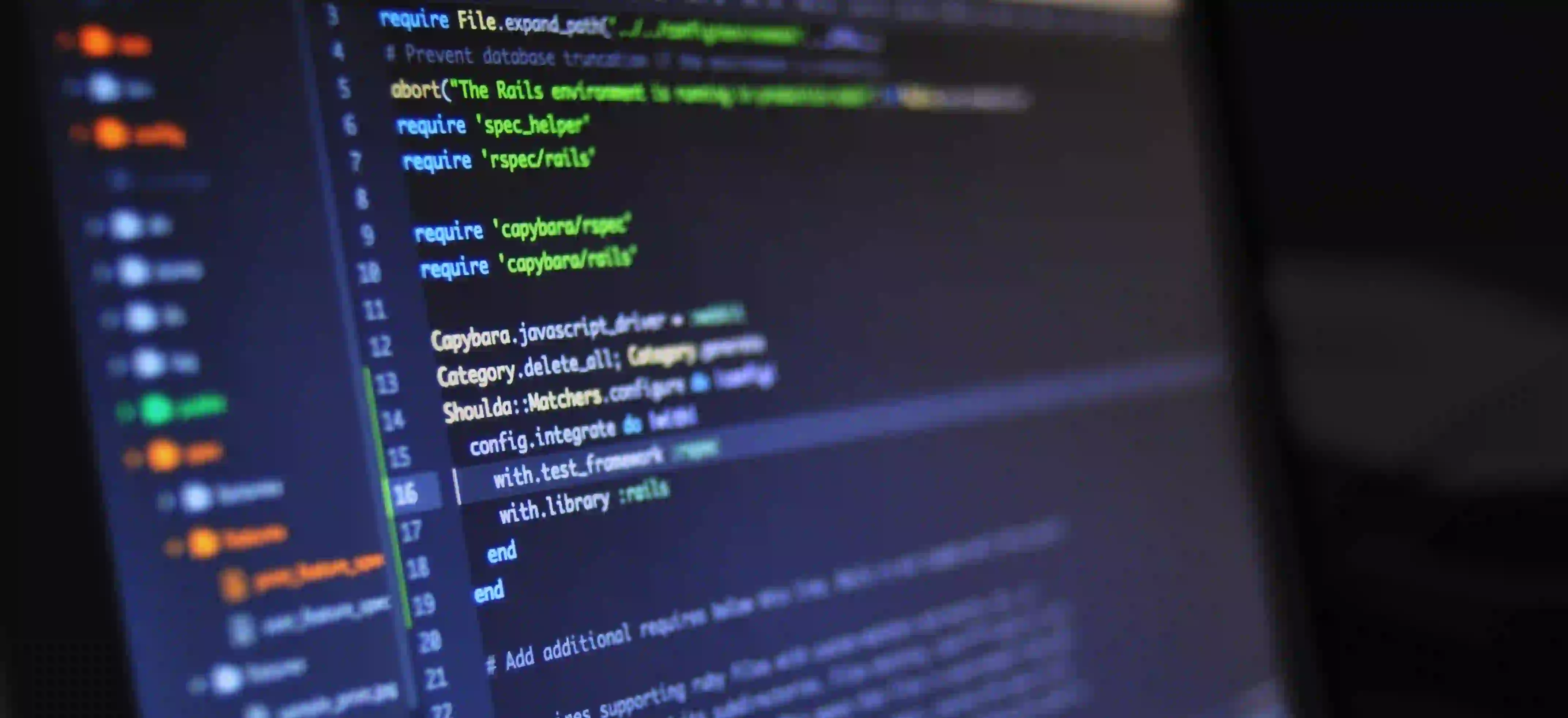
Optimizing Java Performance with React's useMemo and useCallback
In the fast-paced world of software development, performance optimization is crucial. While Java has long been a reliable choice for enterprise applications, front-end frameworks like React can introduce complexities, especially in performance management. This blog will explore how Java developers can leverage React's useMemo
and useCallback
hooks to enhance performance when building applications that interface with Java backends.
The Overlap of Java and React
Java is a powerful backend language renowned for its stability and scalability. Meanwhile, React has transformed the frontend development landscape with its component-based architecture. The challenge arises when integrating the two, particularly when ensuring that the React application communicates efficiently with Java services.
In this context, understanding and utilizing the performance optimizations provided by React is essential to maintaining a smooth user experience.
Understanding useMemo and useCallback
Before we dive into optimization strategies, let’s clarify what useMemo
and useCallback
do:
-
useMemo: This hook is used to memoize expensive calculations. By caching the result of a computation, React can skip recalculating it unless its dependencies change.
-
useCallback: This hook memoizes functions, which helps in preventing unnecessary re-creations of the function during re-renders. This is particularly beneficial when passing functions down as props to child components, potentially preventing unnecessary renders.
Why Are These Hooks Important?
When React re-renders, it typically re-evaluates all components, which can be costly if components execute heavy calculations or expensive renders. In applications where React interacts closely with Java (like fetching data), optimizing this part of the stack can markedly improve performance.
Efficient State Management with useMemo
Imagine you have a component that processes large datasets fetched from the Java backend. Each time the component re-renders, you do not want to reprocess the same data unless it has changed.
Here’s an exemplary usage of useMemo
:
import React, { useMemo } from 'react';
function DataDisplay({ data }) {
const processedData = useMemo(() => {
// Simulating an expensive calculation
return data.map(item => ({
...item,
computedValue: heavyCalculation(item.value)
}));
}, [data]);
return (
<div>
{processedData.map((item, index) => (
<div key={index}>{item.computedValue}</div>
))}
</div>
);
}
Commentary on the Code Above
-
heavyCalculation(item.value)
simulates a resource-intensive operation. Instead of running this on every render,useMemo
only recalculates whendata
changes, preserving performance. -
The dependencies array (
[data]
) ensures that the computation is only re-executed when the data array changes.
Managing Function References with useCallback
Similarly, you may need to manage function references effectively. If a function does not change, it doesn’t need to be recreated unnecessarily. This is especially important in situations where a function is passed down to child components that depend on it.
Here’s how you can use useCallback
:
import React, { useCallback } from 'react';
function ListComponent({ items, onItemClick }) {
return (
<ul>
{items.map(item => (
<li key={item.id} onClick={() => onItemClick(item)}>
{item.name}
</li>
))}
</ul>
);
}
function ParentComponent() {
const handleItemClick = useCallback((item) => {
console.log(item);
}, []);
return <ListComponent items={itemData} onItemClick={handleItemClick} />;
}
Explanation of the Code Snippet
-
handleItemClick
is wrapped inuseCallback
, which prevents its re-creation unless its dependencies change, thus enhancing the efficiency of theListComponent
. -
By ensuring that
onItemClick
never changes, we help React skip rendering that might otherwise happen in the child component when its props are unchanged.
Combining useMemo and useCallback for Maximum Efficiency
By combining both hooks, you can create strategies that optimize React applications interacting with Java backends. Here’s a more integrated example:
function Dashboard({ apiData }) {
const processedData = useMemo(() => {
return apiData.map(item => item.value * 2);
}, [apiData]);
const handleDataChange = useCallback((newData) => {
// Logic to handle data change and maybe notify the Java backend
console.log(newData);
}, []);
return (
<div>
{processedData.map((data, index) => (
<button key={index} onClick={() => handleDataChange(data)}>
{data}
</button>
))}
</div>
);
}
Breaking Down the Combined Use
-
processedData
is computed only whenapiData
changes, leveraging the benefits ofuseMemo
. -
handleDataChange
is a stable function reference, ensuring that events triggered from the button clicks do not cause unnecessary re-renders.
Benefits for Java Developers
For Java developers, understanding React's caching mechanisms helps improve the way you design APIs and optimize data management in applications. Here are some key advantages:
-
Reduced Load on the Backend: Efficient data handling on the frontend minimizes the number of requests to the Java backend, enhancing overall application performance.
-
Improved User Experience: Smooth interactions lead to higher user satisfaction. Users experience faster response times, which is critical for modern web applications.
-
Cleaner and More Maintainable Code: Using
useMemo
anduseCallback
encourages clear and maintainable logic in React components, making it easier for Java developers transitioning to full-stack development.
Additional Resources
For a more exhaustive exploration of performance optimizations in React, you may refer to the article titled Mastering useMemo and useCallback for Efficient React Caching. This article elaborates further on the theory and practical applications of these hooks in performance-driven environments.
Closing the Chapter
Performance optimization is an ongoing necessity in software development, especially when merging technologies from different domains. React's useMemo
and useCallback
are essential tools for Java developers working in the React ecosystem. By judiciously applying these tools, you can build more efficient front-end applications that work seamlessly with Java backends.
With a solid understanding of these hooks, you can elevate your code’s performance and ensure that both your Java and React components work harmoniously together. Remember, a clear separation of concerns and keeping your component logic memoized is key to maintaining an efficient application.
Keep experimenting and optimizing! Happy coding!