JavaFX vs. CSS: Styling Buttons for Desktop Applications
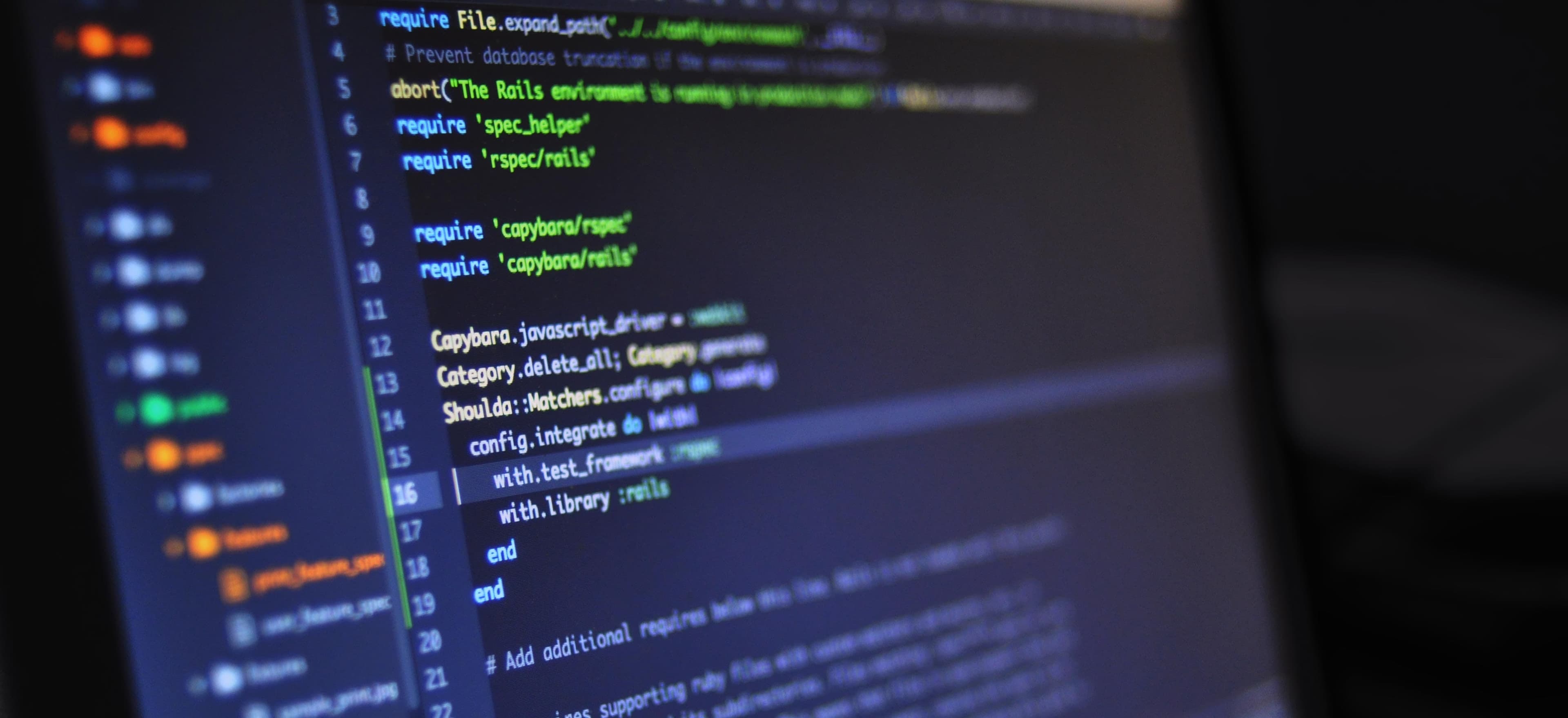
- Published on
JavaFX vs. CSS: Styling Buttons for Desktop Applications
In the world of desktop application development, user interface (UI) design is crucial. The buttons are often the most significant interaction point for users, so styling them correctly enhances user experience. In this blog post, we will explore the dynamics between JavaFX and CSS for styling buttons in desktop applications, guiding you toward creating visually appealing interfaces.
Understanding JavaFX and Its Benefits
JavaFX is a powerful framework for building rich desktop applications in Java. It allows developers to design sophisticated UIs with a flexible component architecture and provides a powerful set of APIs for graphics, media, and effects.
Key Advantages of JavaFX
- Rich API: JavaFX comes with a comprehensive set of libraries and tools, making it easier to create intricate UI designs.
- FXML for UI Design: You can separate your UI from your application logic using FXML, allowing for cleaner code and easier maintenance.
- Hardware Acceleration: The framework uses hardware acceleration to deliver smooth graphics performance, benefiting applications with rich media.
Why CSS for Styling JavaFX Buttons?
While JavaFX provides its own methods for styling, incorporating CSS can take your application's appearance to the next level. CSS allows developers to define styles outside of the Java code, promoting separation of concerns.
Benefits of Using CSS in JavaFX
- Ease of Maintenance: Changes to the style can be made without altering the underlying code.
- Consistency Across Elements: CSS allows for a uniform look and feel across all components, enhancing the user experience.
- Dynamic Styling: With CSS, you can quickly change styles based on user interactions or other events.
Styling Buttons: A Comparison
When styling buttons in JavaFX, you can utilize either JavaFX-specific properties or CSS styles. Let's compare the two approaches.
Using JavaFX API
Here is how you can style a button purely using the JavaFX API:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.paint.Color;
import javafx.scene.text.Font;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class JavaFXButtonExample extends Application {
@Override
public void start(Stage primaryStage) {
Button button = new Button("Click Me");
// Setting button styles programmatically
button.setFont(Font.font("Arial", 20));
button.setTextFill(Color.WHITE);
button.setStyle("-fx-background-color: #0078D7; -fx-padding: 10;");
StackPane layout = new StackPane();
layout.getChildren().add(button);
Scene scene = new Scene(layout, 400, 300);
primaryStage.setScene(scene);
primaryStage.setTitle("JavaFX Button Styling Example");
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Commentary on JavaFX API Approach
Using the JavaFX API gives you direct control over button styles within your code. However, it can lead to cluttered code when multiple properties need to be set.
Styling with CSS
Now, let's see how CSS can simplify the process.
- Create the CSS File
Here is a sample CSS file (buttonStyle.css
):
.button {
-fx-background-color: #0078D7;
-fx-padding: 10;
-fx-font-size: 20px;
-fx-text-fill: white;
}
- Modify the JavaFX Application to Use CSS
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class CSSButtonExample extends Application {
@Override
public void start(Stage primaryStage) {
Button button = new Button("Click Me");
button.getStyleClass().add("button"); // Adding the CSS class
StackPane layout = new StackPane();
layout.getChildren().add(button);
Scene scene = new Scene(layout, 400, 300);
scene.getStylesheets().add("buttonStyle.css"); // Applying the CSS stylesheet
primaryStage.setScene(scene);
primaryStage.setTitle("JavaFX Button Styling Example with CSS");
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Commentary on CSS Approach
This method utilizes a separate CSS file to manage styles, resulting in cleaner code and easier updates. The CSS approach also allows for the reuse of styles across multiple components, making it superior in terms of maintainability.
Best Practices for Styling Buttons in JavaFX
- Use Semantic Naming: Name your CSS classes meaningfully for better readability.
- Avoid Inline Styles: Keep your styles in separate CSS files to enhance maintainability.
- Test Across Platforms: Ensure that your button styles appear consistent across different operating systems.
Real-world Application
A practical scenario for these styling techniques can be drawn from the article titled Styling Woes: Mastering CSS for Perfect Buttons. This article provides insights into creating visually appealing buttons using CSS, which can be adapted for JavaFX applications.
Lessons Learned
Choosing between JavaFX's built-in styling capabilities and CSS ultimately depends on your project's needs. For complex applications requiring easy maintenance and a cohesive design approach, CSS might be the way to go. Conversely, for simple projects where quick adjustments are necessary, the JavaFX API can be sufficient.
Whichever method you choose, ensure that your buttons are not just functional but also visually appealing. Your users will appreciate the attention to detail, leading to a better overall experience with your application.
Further Reading
To dive deeper into the intricacies of styling with CSS, check out resources like the W3Schools documentation on CSS Buttons. Happy coding!
Checkout our other articles