Conquering Creative Blocks in Java Game Development
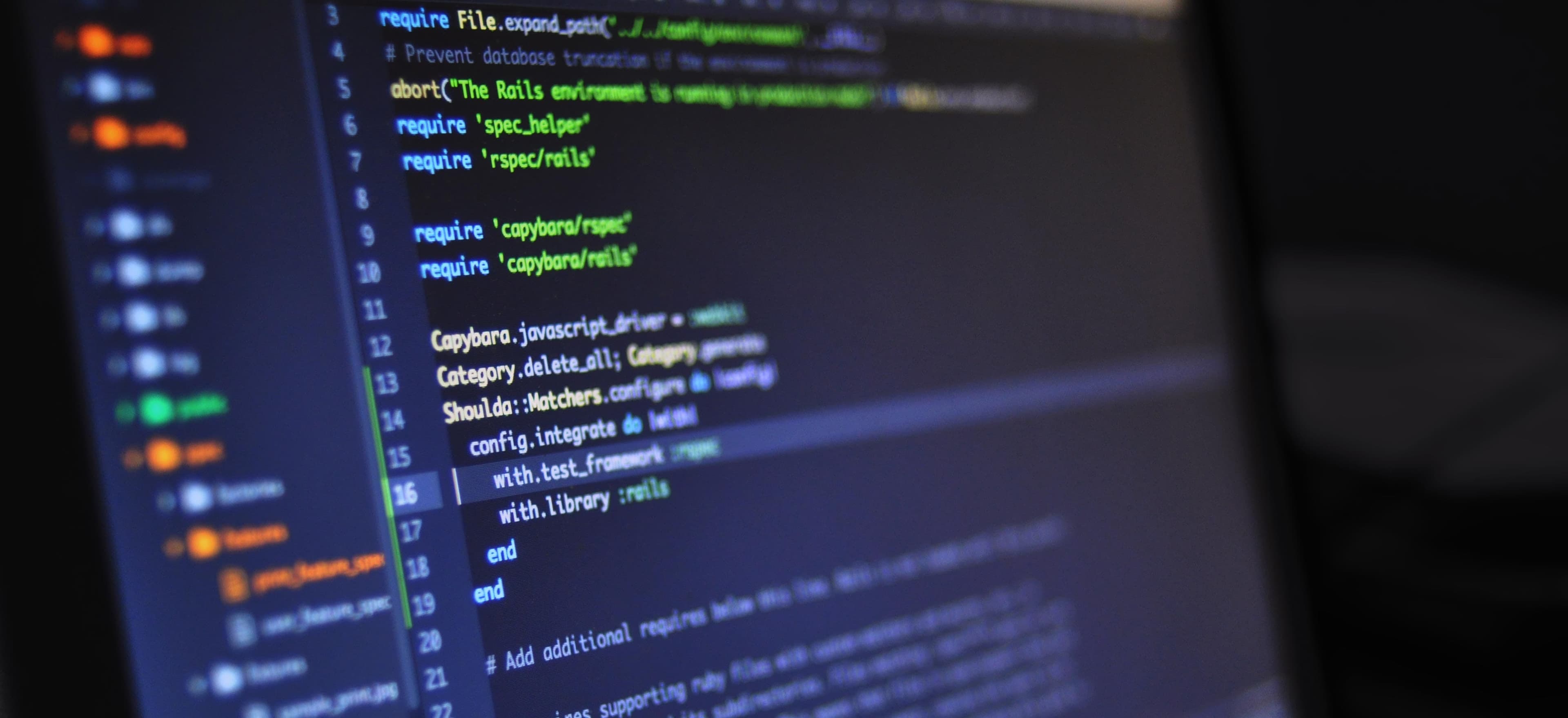
- Published on
Conquering Creative Blocks in Java Game Development
Java has established itself as a powerful tool for game development, with robust libraries, cross-platform capabilities, and a supportive community. However, even the most seasoned developers can face a creative block, halting the flow of innovative ideas. In this blog post, we will explore how to overcome creative blocks specifically in Java game development, enriched with best practices, code snippets, and links to resources.
Understanding Creative Blocks
Before diving into solutions, let’s define what a creative block is. It is a period when a developer feels incapable of producing new work or ideas. This can result from various factors like stress, lack of inspiration, or even technical challenges. Acknowledging and understanding your block is the first step in overcoming it.
The article How to Overcome Creative Block in Small Game Development delves into the small game development side of this issue. This article complements our discussion by providing broader insights that can be applied to Java game projects.
Getting Unstuck: Techniques to Overcome Creative Blocks
1. Revisit Your Project Fundamentals
Sometimes, stepping back and revisiting your project’s fundamentals can ignite your creative spark. This involves reviewing your game idea, core mechanics, and objectives.
Example Code: Let's define the main structure of a simple Java game.
public class MyGame {
public static void main(String[] args) {
Game game = new Game("My Awesome Game");
game.start();
}
}
Why this matters: A straightforward main structure provides clarity and focus. Clarity can guide your creative process and help you see the broader picture, nudging your mind toward creative solutions.
2. Break Down Tasks
Creative blocks often arise from feeling overwhelmed by a large project. Breaking down tasks into smaller, actionable pieces can make the work seem less daunting.
Example Code: Here’s how you might effectively manage a to-do list for game development tasks.
import java.util.*;
public class TaskManager {
private List<String> tasks;
public TaskManager() {
tasks = new ArrayList<>();
}
public void addTask(String task) {
tasks.add(task);
}
public void showTasks() {
for (String task : tasks) {
System.out.println(task);
}
}
public static void main(String[] args) {
TaskManager manager = new TaskManager();
manager.addTask("Design main character");
manager.addTask("Implement jump mechanics");
manager.showTasks();
}
}
Why this matters: This code introduces a straightforward method to track tasks. When you declutter your workload, your mind can focus better, promoting creativity.
3. Draw Inspiration from Others
Don't hesitate to seek inspiration from fellow developers. Online communities, game jams, and forums can provide fresh ideas. Websites like GitHub offer countless repositories of Java games, and browsing through them can spark new concepts.
4. Implement More Prototypes
Prototyping is a powerful way to explore ideas without a long-term commitment. It enables you to experiment with various game mechanics and visuals quickly.
Example Code: Let’s create a simple prototype for a character movement mechanism.
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class SimpleGame extends JPanel implements ActionListener {
private int x = 50, y = 50;
private Timer timer;
public SimpleGame() {
timer = new Timer(100, this);
timer.start();
setFocusable(true);
addKeyListener(new KeyAdapter() {
public void keyPressed(KeyEvent e) {
if (e.getKeyCode() == KeyEvent.VK_UP) {
y -= 5;
} else if (e.getKeyCode() == KeyEvent.VK_DOWN) {
y += 5;
} else if (e.getKeyCode() == KeyEvent.VK_LEFT) {
x -= 5;
} else if (e.getKeyCode() == KeyEvent.VK_RIGHT) {
x += 5;
}
repaint();
}
});
}
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
g.fillRect(x, y, 30, 30); // Draw the character
}
public void actionPerformed(ActionEvent e) {
repaint();
}
public static void main(String[] args) {
JFrame frame = new JFrame("Simple Game");
SimpleGame game = new SimpleGame();
frame.add(game);
frame.setSize(400, 400);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
}
Why this matters: This simple implementation demonstrates character movement, a core element of most games. Prototyping helps in validating or rejecting ideas swiftly, keeping the creative juices flowing.
5. Mix and Match Game Genres
Experimenting with different genres can lead to unexpected and exciting innovations. Do you love platformers but want to add an RPG element? Don’t be afraid to explore this.
6. Take Breaks and Pursue Other Interests
Sometimes the best ideas arise when you stop thinking about your project. Engage in other hobbies, exercise, or take a walk to give your mind a fresh perspective. This break can help reset your brain’s creative pathways.
7. Return to Basic Game Dev Principles
Revisiting the basics and established principles of game design may reignite your passion for your project. Key principles such as balance, feedback, and progression can offer fresh ideas.
Utilizing Libraries and Frameworks
Leveraging libraries can often lead to increased productivity and creativity. Libraries like LibGDX and JavaFX offer ready-made functionalities that can help stimulate creative thinking.
For example, let’s integrate a simple physics engine using LibGDX, which can provide you with an immediate boost in creativity.
import com.badlogic.gdx.ApplicationAdapter;
import com.badlogic.gdx.Gdx;
import com.badlogic.gdx.graphics.GL20;
import com.badlogic.gdx.graphics.g2d.SpriteBatch;
public class PhysicsExample extends ApplicationAdapter {
SpriteBatch batch;
@Override
public void create() {
batch = new SpriteBatch();
}
@Override
public void render() {
Gdx.gl.glClearColor(1, 0, 0, 1);
Gdx.gl.glClear(GL20.GL_COLOR_BUFFER_BIT);
batch.begin();
// Draw and manage physics here
batch.end();
}
}
Why this matters: This simple setup allows you to work on game graphics and physics simultaneously, maximizing your focus on creativity rather than on foundational setups.
Final Thoughts
Creative blocks are an integral part of the development process, but they can be overcome. The essential takeaways include revisiting project fundamentals, breaking down tasks, drawing inspiration, rapid prototyping, embracing genre-fusion, taking regular breaks, and leveraging existing libraries.
Java game development, with its powerful features, provides numerous tools and techniques to keep your creative momentum alive. For additional guidance, refer to the article How to Overcome Creative Block in Small Game Development, which explores more strategies applicable to various facets of game development.
Persist through the blocks, explore, collaborate, and remember that every creative pursuit has its ebbs and flows. Happy coding!
Checkout our other articles