Java Solutions for Cross-Browser Compatibility Challenges
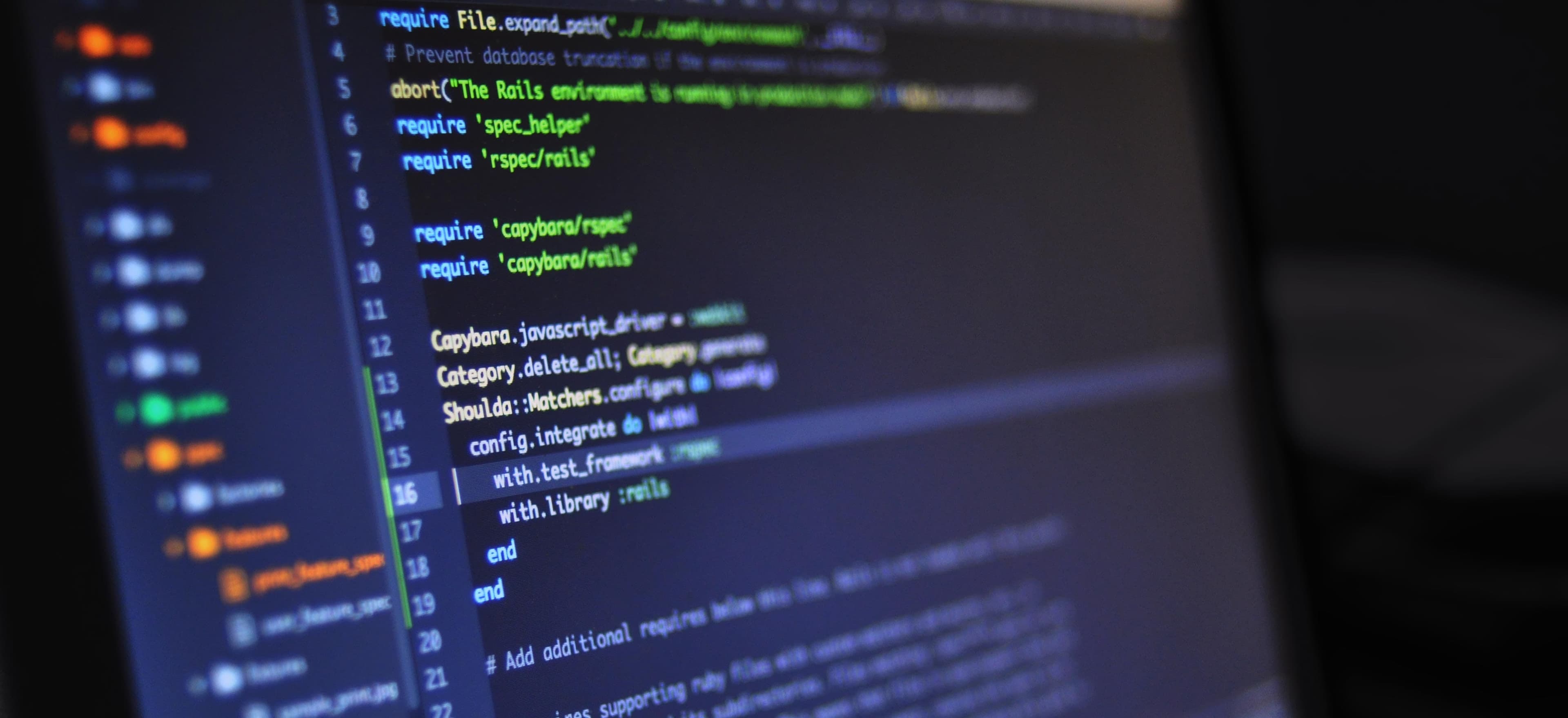
- Published on
Java Solutions for Cross-Browser Compatibility Challenges
In the digital age, ensuring that applications run seamlessly across various web browsers can be a daunting challenge. With the rise of diverse browsers, developers face the task of delivering a consistent user experience. If your application targets multiple browsers, leveraging Java frameworks can significantly ease this process.
In this blog post, we will explore the cross-browser compatibility challenges faced by developers, discuss why some browsers like Internet Explorer continue to present issues, and provide Java-based solutions to tackle these challenges effectively.
Understanding Cross-Browser Compatibility
Cross-browser compatibility refers to the ability of a website or web application to function correctly across different web browsers. The leading browsers include Google Chrome, Mozilla Firefox, Safari, and the often problematic Internet Explorer.
The Significance of Compatibility
Inconsistent rendering and functionality across browsers can lead to poor user experience, loss of engagement, and—ultimately—a decrease in conversions. According to a study by Quirk, about 90% of users will abandon a website if they encounter issues.
Common Cross-Browser Issues
-
CSS and HTML Rendering: Different browsers interpret CSS and HTML specifications in varying ways. What looks perfect in one may appear broken in another.
-
JavaScript Execution: JavaScript can behave unpredictably across browsers. For example, features supported in modern browsers may not be available in Internet Explorer.
-
Font Rendering: Fonts display differently, leading to discrepancies in layout.
-
Event Handling: With different implementations of event listeners, user interactions might break.
-
API Support: Not all APIs are supported across each browser, which can limit functionality or lead to errors.
You may want to read the article titled Why Some Versions of Internet Explorer Still Have Bugs for a closer look at browser inconsistencies and issues that specifically plague Internet Explorer.
Java Solutions for Cross-Browser Compatibility
Java serves as a powerful tool in creating web applications that need to function across multiple browsers. Here are four effective Java solutions to address these compatibility challenges.
1. Using Java-based Frameworks
Java frameworks like Spring MVC, JSF (JavaServer Faces), and Vaadin enable developers to craft responsive applications that can adapt to various browser behaviors.
Example: Spring MVC
Spring MVC can abstract complexities in rendering different views based on browser types, thus enabling more manageable code.
@Controller
public class MyController {
@RequestMapping(value = "/home", method = RequestMethod.GET)
public String showHome(HttpServletRequest request, Model model) {
String browserType = getBrowserType(request);
model.addAttribute("browserType", browserType);
return "home";
}
private String getBrowserType(HttpServletRequest request) {
String userAgent = request.getHeader("User-Agent");
if (userAgent.contains("MSIE")) {
return "Internet Explorer";
} else if (userAgent.contains("Firefox")) {
return "Firefox";
} else {
return "Unknown browser";
}
}
}
Why Use This Approach?
This method allows you to gain insight into the user's browser, tailoring the response based on its type. By detecting the user's browser, you can optimize rendering or provide specific content to cater to that browser's quirks.
2. JavaScript Fallbacks in Java
For Java web applications, handling JavaScript inconsistencies across browsers is crucial. Implementing fallbacks can ensure that your application remains functional regardless of the browser.
Example: Using a Fallback
if (typeof fetch === "undefined") {
alert("Your browser does not support Fetch API. Please upgrade.");
// Fallback code
}
Why This Matters?
When certain JavaScript features are unsupported, informative alerts can guide users to take necessary actions, improving usability and the overall experience.
3. Responsive Web Design Using Java
Java can assist in creating responsive applications that adapt to different devices and screen sizes. Using frameworks like Bootstrap with Java backends ensures a fluid user experience.
Example: Bootstrap Integration
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css">
</head>
<body>
<div class="container">
<h1 class="text-center">Welcome to Our Application</h1>
<div class="row">
<div class="col-md-6">
<p>Your first half-column goes here.</p>
</div>
<div class="col-md-6">
<p>Your second half-column goes here.</p>
</div>
</div>
</div>
</body>
</html>
Why This Matters?
Utilizing responsive design practices means fewer discrepancies across devices. A layout that adjusts smoothly will minimize browser rendering issues and provides a better user experience.
4. Using Java for API Compatibility
Java’s strong typing and rich set of libraries make it favorable for handling cross-browser API compatibility. Tools like Apache HTTPClient can abstract some of the backend complexities.
Example: API Consumption
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
public class ApiFetcher {
public void fetchData(String apiUrl) {
try (CloseableHttpClient client = HttpClients.createDefault()) {
HttpGet request = new HttpGet(apiUrl);
CloseableHttpResponse response = client.execute(request);
// Process response
} catch (Exception e) {
e.printStackTrace();
}
}
}
Why This Matters?
By utilizing an abstraction for HTTP calls, it becomes easier to manage the way data is retrieved or sent across different browsers. This is fundamental in ensuring no functionality is lost due to how different browsers handle API calls.
Closing Remarks
Navigating the landscape of cross-browser compatibility can be intricate. However, Java provides robust solutions to tackle these challenges. By leveraging frameworks, implementing responsive designs, managing JavaScript fallbacks, and employing precise API handling, developers can significantly diminish compatibility issues.
If you want to delve deeper into the issue of browser bugs, do check out the existing article on Why Some Versions of Internet Explorer Still Have Bugs.
Maintaining a consistent user experience across all platforms is crucial. With an arsenal of Java solutions, developers can create adaptable and resilient web applications that transcend the limitations of various browsers. Happy coding!