Mastering Common Programming Interview Pitfalls
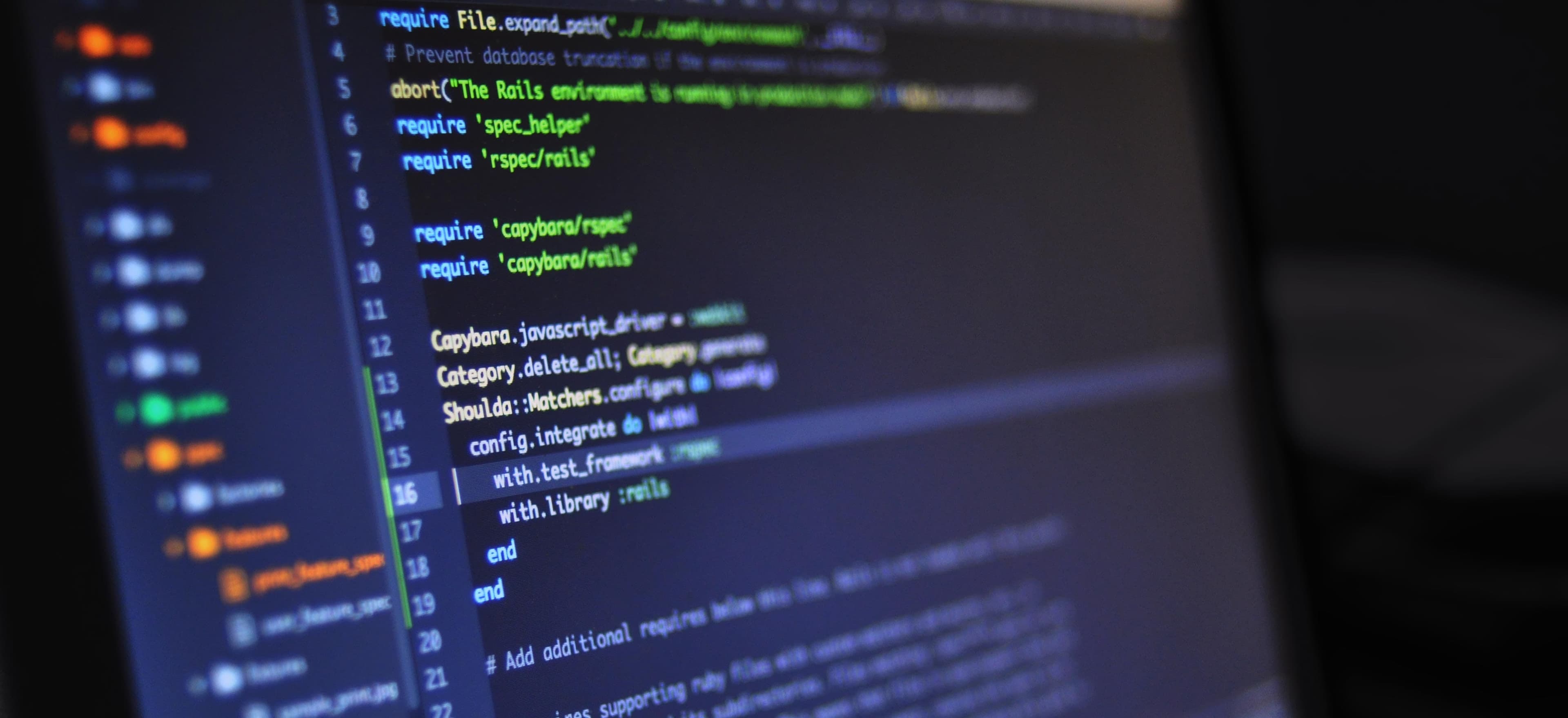
- Published on
Mastering Common Programming Interview Pitfalls
In today’s technology-driven world, programming interviews can be both exhilarating and daunting. For many candidates, the ability to demonstrate technical skills effectively determines success. However, various pitfalls can undermine even the most qualified candidates. Here, we dissect common interview pitfalls programmers face and provide strategies to conquer these challenges.
Understanding Programming Interviews
Before we dive into the pitfalls, let’s briefly review what a programming interview typically entails:
- Algorithm testing: You'll need to write code that can solve specific problems, usually within a set timeframe.
- Technical questions: Expect questions around data structures, complexities, and design patterns.
- System design: Larger interviews may involve designing systems that meet certain requirements.
With this overview established, let’s explore the most common pitfalls and how to master them.
Pitfall 1: Lack of Preparation
Why It Matters
Preparation is essential in any field, especially in programming interviews. A candidate’s confidence can be greatly enhanced through practice and familiarity with problem-solving techniques.
Solution: Practice Regularly
Engaging in mock interviews or joining coding communities can elevate your skills. Websites like LeetCode and HackerRank provide ample programming challenges across varying difficulty levels.
Example Scenario
Suppose the interviewer asks you to solve the following problem:
Problem: Given an array of integers, find two numbers such that they add up to a specific target number.
Code Snippet
import java.util.HashMap;
public class TwoSum {
public static int[] findTwoSum(int[] nums, int target) {
HashMap<Integer, Integer> map = new HashMap<>(); // To store the numbers and their indices
for (int i = 0; i < nums.length; i++) {
int complement = target - nums[i]; // Calculate the required complement
if (map.containsKey(complement)) {
return new int[] { map.get(complement), i }; // Found the two indices
}
map.put(nums[i], i); // Store the current number and its index
}
throw new IllegalArgumentException("No two sum solution");
}
}
Commentary
In this example, utilizing a HashMap
allows us to achieve a time complexity of O(n). Instead of a brute-force O(n^2) approach with nested loops, we can effectively reduce our operations by storing past values in the map (which operates on average in O(1) time complexity).
Pitfall 2: Overcomplicating Solutions
Why It Matters
Complexity can lead to confusion. Many candidates attempt to rewrite existing algorithms instead of applying well-known methods, leading to ineffective solutions.
Solution: Keep It Simple
Strive for simplicity first. If a problem can be solved using established paradigms, embrace them. Familiarity with fundamental concepts can save a lot of time.
Example Scenario
Problem: You need to check if a string is a palindrome, reading the same forwards and backward.
Code Snippet
public class PalindromeCheck {
public static boolean isPalindrome(String str) {
if (str == null) return false; // Handle null input
int left = 0, right = str.length() - 1;
while (left < right) {
if (str.charAt(left) != str.charAt(right)) return false; // Compare characters
left++;
right--;
}
return true; // The string is a palindrome
}
}
Commentary
This straightforward O(n) solution checks characters from both ends until the middle is reached. Clear logic helps in maintaining focus during the interview without overwhelming the interviewer with unnecessary complicated procedures.
Pitfall 3: Poor Communication Skills
Why It Matters
Your ability to think aloud is crucial in interviews. Silence during problem-solving may create the impression of uncertainty or lack of knowledge.
Solution: Verbalize Your Thought Process
Practice explaining your rationale clearly—what you're doing and why. Provide context and express potential edge cases.
Example Scenario
Imagine you're asked to implement a method for sorting an array. Don’t just code. Discuss your choice of sorting algorithm; for instance, say:
"I'll use quicksort here due to its average performance of O(n log n). I’ll also need to ensure we handle duplicates effectively."
Key Aspects to Discuss
- Why you selected a specific algorithm
- How the algorithm works
- The time and space complexity of your solution
Pitfall 4: Ignoring Edge Cases
Why It Matters
Neglecting edge cases can lead to failures in real-world applications. Interviewers often use edge cases to test the robustness of a solution.
Solution: Test Against Edge Cases
Before finalizing your code, consider and write test cases for:
- Empty inputs
- Maximum and minimum values
- Null values
Code Snippet
public class ArraySum {
public static int sum(int[] array) {
if (array == null || array.length == 0) return 0; // Handle null or empty array
int sum = 0;
for (int num : array) {
sum += num; // Sum the numbers
}
return sum;
}
}
Commentary
In this snippet, the method first checks for null or empty input, a crucial step often omitted by candidates. Testing against edge cases demonstrates thoroughness and anticipates potential pitfalls.
Final Thoughts
Mastering programming interview pitfalls requires an amalgamation of solid preparation, clear communication, and strategic thinking. It’s essential to practice regularly, focus on simplicity, and be candid and engaging during the interview.
For deeper insights into analyzing data structures and optimizing algorithms, consider exploring resources like GeeksforGeeks or refining your system design skills through targeted learning.
Ultimately, every interviewer seeks a blend of technical skill and the capacity for logical, clear communication. By avoiding these common pitfalls, you can present yourself confidently as a capable programmer ready to tackle any challenge.
Arming yourself with these insights will not only help in interviews but also enhance your overall programming acumen. Good luck!
Checkout our other articles