How the Proxy Pattern Can Simplify Your Code Architecture
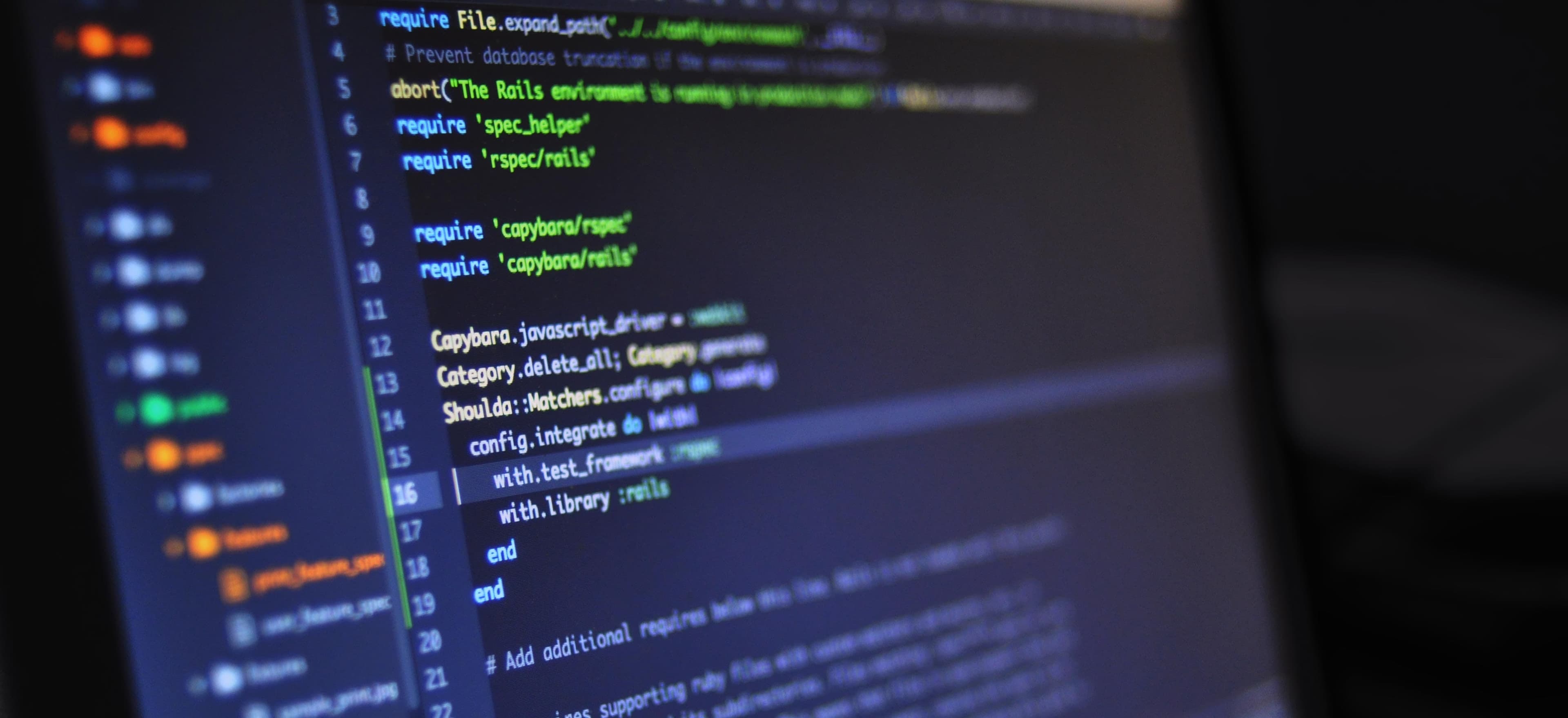
- Published on
How the Proxy Pattern Can Simplify Your Code Architecture
In the vast landscape of software development, design patterns serve as tried-and-tested solutions to common problems. One such pattern that often garners attention is the Proxy Pattern. In this blog post, we will delve into its significance, discuss its various types, illustrate the implementation through Java code snippets, and explain how it can simplify your code architecture.
Understanding the Proxy Pattern
At its core, the Proxy Pattern provides a placeholder or surrogate for another object to control access to it. It serves as an intermediary, allowing you to add functionality or control access without changing the original object. The design pattern is especially useful in scenarios where an object is expensive to create, manage, or interact with.
Why Use the Proxy Pattern?
- Lazy Initialization: It allows for delayed object creation until it is needed, saving resources.
- Access Control: It can manage permission levels, ensuring that only certain clients can access specific objects.
- Logging and Monitoring: It can serve as a logging mechanism, tracking requests made to the real object.
- Remote Proxy Capabilities: It can facilitate remote method invocation when dealing with distributed systems.
With a solid understanding of its purpose, let's explore the types of proxies commonly utilized.
Types of Proxies
- Virtual Proxy: Delays the creation and initialization of an object until it is actually needed.
- Protection Proxy: Controls access and permissions for the original object, enhancing security.
- Remote Proxy: Facilitates communication between different systems, almost acting like a local representation of a remote object.
Implementing the Proxy Pattern in Java
Step 1: Define the Subject Interface
The first step in implementing the Proxy Pattern is to define an interface that both the RealSubject and Proxy classes will share.
public interface Image {
void display();
}
Why this matters: By defining an interface, you create a contract that both the subject and proxy adhere to. This ensures that both the RealSubject and Proxy can be used interchangeably, promoting flexibility.
Step 2: Create the RealSubject
The RealSubject class implements the Image interface and embodies the actual functionality.
public class RealImage implements Image {
private String filename;
public RealImage(String filename) {
this.filename = filename;
loadFromDisk();
}
private void loadFromDisk() {
System.out.println("Loading " + filename);
}
@Override
public void display() {
System.out.println("Displaying " + filename);
}
}
Why use a RealSubject?: It encapsulates the actual implementation that can be resource-intensive. In this case, loading an image onto memory is simulated by printing statements.
Step 3: Create the Proxy Class
Now, we can implement the Proxy that controls access to the RealImage.
public class ProxyImage implements Image {
private RealImage realImage;
private String filename;
public ProxyImage(String filename) {
this.filename = filename;
}
@Override
public void display() {
if (realImage == null) {
realImage = new RealImage(filename);
}
realImage.display();
}
}
Why the Proxy?: The Proxy class ensures that RealImage is created only when needed. This is particularly important if the loading of the image consumes significant resources.
Step 4: Using the Proxy
With our classes defined, we can now utilize our Proxy in a client scenario.
public class Client {
public static void main(String[] args) {
Image image1 = new ProxyImage("test_image_1.jpg");
Image image2 = new ProxyImage("test_image_2.jpg");
// Image will be loaded from disk
image1.display();
// Image will not be loaded from disk this time
image1.display();
// Image will be loaded from disk
image2.display();
}
}
Why a Client?: This demonstrates how the user interacts with the proxy without needing to understand the instantiation of RealImage. The Proxy manages resource allocation effectively.
Benefits of Simplifying Your Code Architecture with the Proxy Pattern
-
Decoupling: By using a proxy, you decouple the client code from the implementation details of the RealSubject. This reduces dependencies and enhances code maintainability.
-
Easier Testing: Proxies can facilitate testing by allowing you to mock or stub interactions with the RealSubject. This enables developers to isolate functionality during unit testing.
-
Enhanced Performance: In scenarios where resources are costly to manage, lazy initialization ensures your application runs more efficiently, only utilizing memory and processing power as needed.
-
Controlled Access: The Proxy Pattern provides a structure to enforce access controls cleanly, allowing only authorized users or systems to interact with essential resources.
The Closing Argument
The Proxy Pattern is a powerful design pattern that simplifies your code architecture by acting as an intermediary between the client and the actual object. It enhances resource management, access control, and system performance, all while maintaining a clean separation of concerns.
To learn more about design patterns in Java, consider checking out The Java™ Tutorials and Refactoring Guru, which provide comprehensive guides and examples to further deepen your understanding.
By integrating the Proxy Pattern into your software development toolkit, you can write clearer, more maintainable code that is both efficient and user-friendly. Happy coding!
Checkout our other articles