Choosing Between Spring Boot Test and WebMvcTest: A Guide
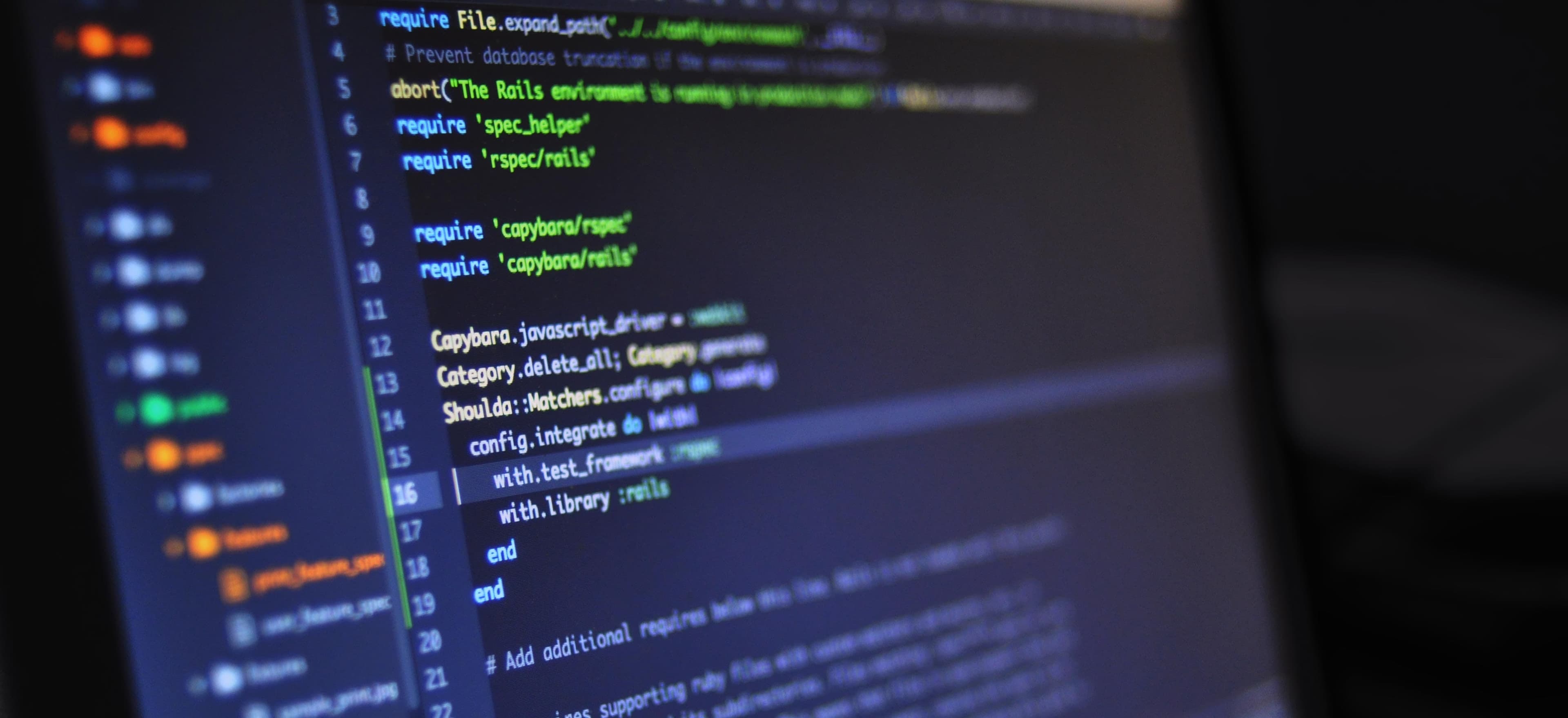
- Published on
Choosing Between Spring Boot Test and WebMvcTest: A Guide
In the realm of Java web development, Spring Boot has revolutionized the way developers build and maintain applications. With its emphasis on convention over configuration, developers can sweat less over boilerplate code and focus more on business logic. However, a critical part of developing robust applications is ensuring that your code is well-tested. In this guide, we will explore two vital testing annotations in Spring Boot: @SpringBootTest
and @WebMvcTest
. We’ll delve into their use-cases, advantages, and when to utilize each in your development projects.
Table of Contents
- Understanding Spring Boot Testing
- What is @SpringBootTest?
- What is @WebMvcTest?
- When to Use @SpringBootTest
- When to Use @WebMvcTest
- Example of @SpringBootTest
- Example of @WebMvcTest
- Conclusion
Understanding Spring Boot Testing
Writing tests for your application is essential. It not only validates your code but also ensures that your service runs as intended after every change. Spring Boot provides several testing tools that make it easier for you to write tests. The two most commonly used annotations are @SpringBootTest
and @WebMvcTest
.
@SpringBootTest
More suited for integration testing, @SpringBootTest
offers a complete context loading. This means that all the beans in the application context will be loaded. Useful for verifying that the system works as a whole, it is ideal for integration tests where multiple components need to be wired together.
@WebMvcTest
On the other hand, @WebMvcTest
is tailored specifically for testing the web layer of your application—the controllers. It only loads the beans essential for MVC components and does not load other beans, such as service or repository beans. This is advantageous for isolating web layer tests, making it faster and less resource-intensive.
What is @SpringBootTest?
@SpringBootTest
is an annotation that tells Spring Boot to look for a main configuration class (one annotated with @SpringBootApplication
) and load the entire application context. This feature makes it suitable for integration tests where various components interact with each other.
Key Features:
- Loads the full application context.
- Can be configured to run on a specific port.
- Enables testing of both the servlet environment and the web layer.
Example of @SpringBootTest
@RunWith(SpringRunner.class)
@SpringBootTest
public class MyApplicationTests {
@Autowired
private MyService myService;
@Test
public void contextLoads() {
assertNotNull(myService);
}
}
In this example, we are testing that the Spring application context loads successfully and that the MyService
bean is correctly instantiated. The context will load all the beans in the application, thus providing a comprehensive test.
What is @WebMvcTest?
@WebMvcTest
focuses primarily on the controller aspect of the application. It only loads the relevant components for the MVC layer, excluding services and repositories by default. This limitation allows for faster execution of your tests since less overhead is involved.
Key Features:
- Loads only web-related components (controllers, filters).
- Does not start the full application context.
- Facilitates mocking of service layers for isolated testing.
Example of @WebMvcTest
@RunWith(SpringRunner.class)
@WebMvcTest(MyController.class)
public class MyControllerTests {
@Autowired
private MockMvc mockMvc;
@MockBean
private MyService myService;
@Test
public void testGetHello() throws Exception {
Mockito.when(myService.getGreeting()).thenReturn("Hello, World!");
mockMvc.perform(get("/greet"))
.andExpect(status().isOk())
.andExpect(content().string("Hello, World!"));
}
}
In this example, the @WebMvcTest
annotation only loads the MyController
and configures a MockMvc
instance to simulate HTTP requests. We also mock the MyService
bean to isolate our tests against the service layer. This keeps our tests fast and focused precisely on the web layer.
When to Use @SpringBootTest
You should opt for @SpringBootTest
when:
- You need to test the interaction between multiple components.
- You are working on integration tests where the full application context is necessary.
- You want to ensure that all beans, including configurations, services, and repositories, are instantiated correctly.
When to Use @WebMvcTest
You should consider using @WebMvcTest
when:
- You are focusing solely on the controller layer.
- You want to achieve faster test execution without loading the full application context.
- You need to isolate your controller's behavior without the side effects of other beans.
To Wrap Things Up
Testing is a crucial aspect of software development, especially in a complex web application. Choosing the right testing approach is essential to ensure the robustness of your application. While @SpringBootTest
provides a comprehensive test of the entire application context, @WebMvcTest
allows for quicker, more focused testing of the web layer. Depending on the use-case, both annotations serve their own unique purpose in ensuring that your Spring Boot application is functioning as intended.
By understanding when to use @SpringBootTest
and @WebMvcTest
, you can create a solid testing strategy that aligns with your project's requirements. The goal is to write meaningful tests that add value and confidence to your codebase. Happy testing!
For more detailed documentation on Spring Testing, please visit the Spring Boot Testing documentation.
Checkout our other articles